Docker Run Bash: How to Run a Bash Shell in a Container
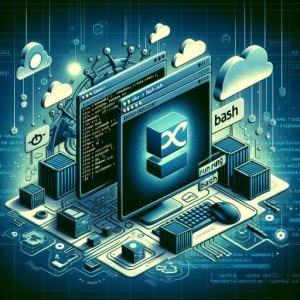
Are you finding it difficult to run a bash shell within a Docker container? You’re not alone. Many developers find themselves grappling with this, but there’s a command that can make this process a breeze.
Bash commands can run on any system, even those without Docker installed. However for this purpose, you can think of Docker as a shipping container for your code, and ‘docker run bash’ as the crane that places it where it needs to go.
This guide will walk you through the ins and outs of using ‘docker run bash’, from the basics to more advanced scenarios. We’ll explore Docker’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Bash shells and Docker!
TL;DR: How Do I Use ‘docker run bash’?
The
'docker run bash'
command is used to start a new Docker container and run a Bash shell inside it. It can also be used with flags, such asdocker run -it ubuntu bash
. This command allows you to interact with the container in real-time, making it a powerful tool for debugging and development.
Here’s a simple example:
docker run -it ubuntu bash
# Output:
# root@container_id:/#
In this example, we use the ‘docker run bash’ command to start a new container from the ‘ubuntu’ image and run a Bash shell inside it. The -it
flag tells Docker to run the container in interactive mode, and ubuntu
is the name of the Docker image we’re using. The bash
command at the end starts a Bash shell inside the new container.
This is just a basic way to use ‘docker run bash’, but there’s much more to learn about Docker and its powerful command-line interface. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Docker Run Bash: A Beginner’s Guide
- Intermediate Level: Running Scripts and Applications
- Exploring Alternative Approaches to Docker Interactions
- Common Issues and Solutions with Docker Run Bash
- Understanding Docker and Bash Fundamentals
- Docker Run Bash: Integrating into Larger Workflows
- Wrapping Up: Mastering Docker Run Bash
Docker Run Bash: A Beginner’s Guide
The ‘docker run bash’ command is a combination of several elements, each with its own purpose. Let’s break down what each part of the command does.
docker
: This is the base command for the Docker CLI (Command Line Interface).run
: This is a subcommand ofdocker
. Therun
command creates a new Docker container from an image.-it
: This is a combination of two options. The-i
(or--interactive
) option keeps STDIN open even if not attached, allowing you to interact with the container. The-t
(or--tty
) option allocates a pseudo-TTY, making the container’s shell interactive.ubuntu
: This is the name of the Docker image from which the container is created. In this case, it’s the official image for Ubuntu, a popular Linux distribution.bash
: This is the command that is run inside the new Docker container. In this case, it starts a new Bash shell.
Now, let’s see a simple example of how to use the ‘docker run bash’ command and understand its output.
docker run -it debian bash
# Output:
# root@container_id:/#
In this example, we’ve replaced ubuntu
with debian
, another popular Linux distribution. Like before, the docker run bash
command starts a new Docker container from the Debian image and runs a Bash shell inside it. The output root@container_id:/#
is the command prompt of the new Bash shell, indicating that you’re now inside the Docker container and ready to run commands.
Intermediate Level: Running Scripts and Applications
As you become more comfortable with the ‘docker run bash’ command, you can start to explore its more advanced uses. One common use case is running scripts or applications inside the Docker container.
Let’s say you have a Python script that you want to run inside the container. You can do this by including the script in the ‘docker run bash’ command. Here’s how:
docker run -it python:3.7 bash -c "echo 'print("Hello, Docker!")' > script.py && python script.py"
# Output:
# Hello, Docker!
In this example, we’re using the ‘docker run bash’ command to start a new Docker container from the Python 3.7 image. The -c
option allows us to run multiple commands in the Bash shell. We’re using it to create a Python script called script.py
and then run that script using Python.
The output Hello, Docker!
is the result of running the Python script inside the Docker container. This demonstrates how you can use ‘docker run bash’ to run scripts or applications inside Docker containers, enabling a wide range of development and testing scenarios.
Exploring Alternative Approaches to Docker Interactions
While ‘docker run bash’ is a powerful command, it’s not the only way to interact with Docker containers. Two other commands, ‘docker exec’ and ‘docker attach’, offer alternative methods of interaction.
The ‘docker exec’ Command
The ‘docker exec’ command allows you to run a command in a running Docker container. This is useful when you want to inspect a running container or debug a problem.
Here’s an example of how to use the ‘docker exec’ command:
docker run -d --name my_container ubuntu sleep infinity
# Output:
# container_id
docker exec -it my_container bash
# Output:
# root@container_id:/#
In this example, we first start a new Docker container called ‘my_container’ using the ‘docker run’ command. The -d
option tells Docker to run the container in detached mode, meaning it runs in the background. The sleep infinity
command keeps the container running indefinitely.
We then use the ‘docker exec’ command to start a Bash shell in the running container. The output root@container_id:/#
indicates that we’re inside the Docker container.
The ‘docker attach’ Command
The ‘docker attach’ command allows you to attach your terminal’s input, output, and error streams to a running Docker container. This is useful when you want to view the output of a running container or interact with it.
Here’s an example of how to use the ‘docker attach’ command:
docker run -d --name my_container ubuntu bash -c "while true; do echo Hello, Docker!; sleep 1; done"
# Output:
# container_id
docker attach my_container
# Output:
# Hello, Docker!
# Hello, Docker!
# ...
In this example, we start a new Docker container called ‘my_container’ that prints ‘Hello, Docker!’ every second. We then use the ‘docker attach’ command to attach our terminal to the running container. The output shows the ‘Hello, Docker!’ messages being printed by the container.
Comparing the Approaches
While all three commands are useful, they each have their pros and cons. The ‘docker run bash’ command is great for starting a new container and interacting with it immediately. The ‘docker exec’ command is useful for running a command in a running container, and the ‘docker attach’ command is great for viewing the output of a running container or interacting with it.
Ultimately, the best command to use depends on your specific needs and the situation at hand.
Common Issues and Solutions with Docker Run Bash
While ‘docker run bash’ is a powerful command, it’s not without its potential issues. Let’s discuss some common problems you might encounter and how to solve them.
Networking Problems
One common issue when using Docker is networking problems. For example, you might have trouble connecting to the internet from inside a Docker container. This can be caused by a variety of factors, such as misconfigured network settings or firewall rules.
Here’s an example of how you might troubleshoot a networking issue:
docker run -it ubuntu bash -c "ping -c 4 google.com"
# Output:
# PING google.com (172.217.164.174) 56(84) bytes of data.
# From 172.17.0.2 icmp_seq=1 Destination Host Unreachable
# ...
In this example, we’re trying to ping Google’s servers from inside a Docker container. If you see a message like ‘Destination Host Unreachable’, it means that the Docker container is having trouble connecting to the internet.
One possible solution is to check your Docker network settings and make sure they’re configured correctly. You might also need to check your firewall rules to make sure they’re not blocking traffic to or from Docker containers.
File Permission Issues
Another common issue when using ‘docker run bash’ is file permission problems. For example, you might have trouble reading or writing files from inside a Docker container. This can be caused by a variety of factors, such as incorrect file permissions or ownership.
Here’s an example of how you might troubleshoot a file permission issue:
docker run -it ubuntu bash -c "echo 'Hello, Docker!' > file.txt && cat file.txt"
# Output:
# bash: file.txt: Permission denied
In this example, we’re trying to write to a file and then read from it inside a Docker container. If you see a message like ‘Permission denied’, it means that the Docker container is having trouble accessing the file.
One possible solution is to check the file permissions and ownership and make sure they’re set correctly. You might also need to use the chown
or chmod
commands to change the file’s ownership or permissions.
Remember, when troubleshooting, it’s important to understand the problem fully before trying to fix it. Always start with the basics, and don’t be afraid to ask for help if you need it.
Understanding Docker and Bash Fundamentals
Before we dive deeper into the ‘docker run bash’ command, let’s take a step back and understand the basics of Docker and Bash.
Docker: The Basics
Docker is an open-source platform designed to make it easier to create, deploy, and run applications by using containers. Containers allow a developer to package up an application with all the parts it needs, such as libraries and other dependencies, and ship it all out as one package.
docker run hello-world
# Output:
# Hello from Docker!
# This message shows that your installation appears to be working correctly.
In the example above, we run the ‘hello-world’ Docker image, which is a simple image that tests your Docker installation and runs a message in the console. The output verifies that Docker is installed and working correctly on your system.
Bash: The Basics
Bash, or the Bourne Again SHell, is a command interpreter and a well-known Unix shell. Bash supports a command-line editor, job control, command-line completion, and unlimited size command history.
echo 'Hello, Bash!'
# Output:
# Hello, Bash!
In the example above, we use the ‘echo’ command in Bash to print a simple message. The output shows the message ‘Hello, Bash!’, demonstrating a basic use of the Bash shell.
Docker and Bash: A Powerful Combination
Running a Bash shell inside a Docker container allows you to interact with the container in real time, making it a powerful tool for debugging and development. You can run commands, inspect the filesystem, and even install new software inside the container, all from the comfort of your Bash shell.
docker run -it ubuntu bash -c "ls -l"
# Output:
# total 64
# drwxr-xr-x 2 root root 4096 Apr 24 2018 bin
# drwxr-xr-x 2 root root 4096 Apr 24 2018 boot
# ...
In the example above, we use the ‘docker run bash’ command to start a new Docker container and run the ‘ls -l’ command inside it. The output shows the contents of the root directory inside the Docker container, demonstrating how you can use a Bash shell to interact with a Docker container.
Docker Run Bash: Integrating into Larger Workflows
While mastering ‘docker run bash’ is a significant step, it’s essential to understand how this command fits into larger development and deployment workflows. Docker is not just about running containers; it’s about building, shipping, and running applications in a consistent and repeatable manner.
Dockerfile Creation
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. Using docker build
, you can create an automated build that executes several command-line instructions in succession.
echo -e 'FROM ubuntu:18.04
RUN apt-get update && apt-get install -y curl' > Dockerfile
docker build -t my_image .
docker run -it my_image bash -c "curl --version"
# Output:
# curl 7.58.0 (x86_64-pc-linux-gnu) libcurl/7.58.0 OpenSSL/1.1.1 zlib/1.2.11 libidn2/2.0.4 libpsl/0.19.1 (+libidn2/2.0.4) nghttp2/1.30.0 librtmp/2.3
In this example, we create a Dockerfile that starts with the Ubuntu 18.04 image and installs curl. We then build a new Docker image using this Dockerfile and run a new container from this image. The output shows the version of curl installed in the container.
Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. With Compose, you use a YAML file to configure your application’s services. Then, with a single command, you create and start all the services from your configuration.
echo -e 'version: "3"
services:
web:
image: nginx:alpine
ports:
- "80:80"' > docker-compose.yml
docker-compose up -d
curl localhost
# Output:
# <!DOCTYPE html>
# <html>
# <head>
# <title>Welcome to nginx!</title>
# ...
In this example, we create a docker-compose.yml file that defines a single service running the nginx:alpine image. We then use the ‘docker-compose up’ command to start the service. The output shows the default welcome page served by Nginx.
Kubernetes
Kubernetes, also known as K8s, is an open-source system for automating deployment, scaling, and management of containerized applications. It groups containers that make up an application into logical units for easy management and discovery.
echo -e 'apiVersion: v1
kind: Pod
metadata:
name: my_pod
spec:
containers:
- name: my_container
image: ubuntu
command: ["bash", "-c", "echo Hello, Kubernetes! && sleep infinity"]' > pod.yml
kubectl apply -f pod.yml
kubectl logs my_pod
# Output:
# Hello, Kubernetes!
In this example, we create a pod.yml file that defines a single Kubernetes Pod running the Ubuntu image. We then use the ‘kubectl apply’ command to create the Pod. The output shows the message printed by the Pod.
Further Resources for Docker Mastery
To further explore Docker and its related technologies, check out the following resources:
- Docker’s official documentation
- Kubernetes’ official documentation
- Docker Compose’s official documentation
Wrapping Up: Mastering Docker Run Bash
This comprehensive guide has provided a deep dive into the ‘docker run bash’ command, a vital tool for Docker users. We’ve explored its usage, from the basic to the advanced, and how it fits into the broader Docker ecosystem.
We began with the basics, learning how to use ‘docker run bash’ to start a new Docker container and run a Bash shell inside it. We then progressed to more advanced scenarios, such as running scripts or applications inside the container. Along the way, we discussed common issues and their solutions, providing a roadmap to navigate potential challenges.
We also explored alternative approaches to interacting with Docker containers, such as the ‘docker exec’ and ‘docker attach’ commands. These alternatives offer different ways to interact with Docker containers, broadening your Docker toolbox.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Pros | Cons |
---|---|---|---|
docker run bash | Starting a new container and running a Bash shell inside it | Powerful and flexible | May require troubleshooting for some scenarios |
docker exec | Running a command in a running container | Useful for inspecting a running container | Requires a running container |
docker attach | Attaching to a running container | Great for viewing the output of a running container | Only works with running containers |
Whether you’re just starting out with Docker or you’re an experienced user looking to deepen your understanding, we hope this guide has provided valuable insights into the ‘docker run bash’ command and its alternatives.
Mastering ‘docker run bash’ is a significant step towards becoming a Docker power user. With this command and its alternatives in your toolbox, you’re well equipped to handle a wide range of Docker tasks. Happy Dockering!