Characters in Java: Working with Char Data Type and Class
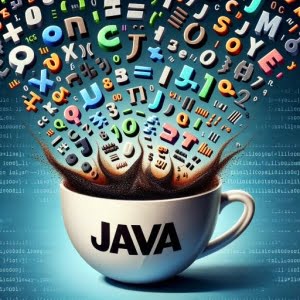
Are you finding it challenging to work with characters in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling characters in Java, but we’re here to help.
Think of Java’s character handling as a linguist’s toolkit – allowing us to manipulate and utilize characters in a variety of ways, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with characters in Java, from their declaration, manipulation, and usage. We’ll cover everything from the basics of character handling to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Work with Characters in Java?
In Java, you can declare and manipulate characters using the
char
keyword and theCharacter
class,char letter = 'A';
. Here’s a simple example of declaring a character:
char letter = 'A';
System.out.println(letter);
// Output:
// 'A'
In this example, we declare a character variable letter
and assign it the value ‘A’. We then print the value of letter
, which outputs ‘A’.
This is just the beginning of what you can do with characters in Java. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Understanding the char Data Type in Java
- Diving Deeper: Character Encoding and Decoding
- Leveraging the Character Class
- Handling Unicode Characters
- Exploring Alternative Approaches to Character Handling
- Troubleshooting Common Issues with Characters in Java
- Deep Dive into Java Characters: Unicode, ASCII, and More
- Exploring Relevance of Character Handling in Java
- Further Resources for Mastering Java Characters
- Wrapping Up: Characters in Java
Understanding the char
Data Type in Java
In Java, the char
keyword is used to declare a character variable. This data type can store a single 16-bit Unicode character.
Here’s how you can declare a character in Java:
char letter = 'B';
System.out.println(letter);
// Output:
// 'B'
In this example, we declare a character variable letter
and assign it the value ‘B’. We then print the value of letter
, which outputs ‘B’.
Basic Operations: Comparison and Conversion to Integer
Java allows you to perform various operations on characters, including comparison and conversion to integers.
Character Comparison
You can compare characters in Java using relational operators like “, ==
, and !=
. Here’s an example:
char letter1 = 'A';
char letter2 = 'B';
System.out.println(letter1 < letter2);
// Output:
// true
In this example, we compare two characters letter1
and letter2
. Since ‘A’ comes before ‘B’ in the Unicode table, letter1 < letter2
returns true.
Converting Characters to Integers
You can convert a character to its corresponding integer value in the Unicode table using a simple casting operation:
char letter = 'A';
int letterValue = (int) letter;
System.out.println(letterValue);
// Output:
// 65
In this example, we convert the character ‘A’ to its corresponding integer value in the Unicode table, which is 65.
Diving Deeper: Character Encoding and Decoding
As you advance in Java, you’ll encounter more complex operations involving characters. One such operation is character encoding and decoding. In Java, you can encode a character into its corresponding Unicode value and decode a Unicode value back into its corresponding character.
Here’s an example of character encoding:
char letter = 'A';
int unicodeValue = (int) letter;
System.out.println(unicodeValue);
// Output:
// 65
In this example, we encode the character ‘A’ into its corresponding Unicode value, which is 65.
And here’s an example of character decoding:
int unicodeValue = 65;
char letter = (char) unicodeValue;
System.out.println(letter);
// Output:
// 'A'
In this example, we decode the Unicode value 65 back into its corresponding character, which is ‘A’.
Leveraging the Character
Class
Java provides a Character
class that offers various methods for manipulating characters. For instance, you can use the Character
class to convert a character to uppercase or lowercase.
Here’s an example:
char letter = 'a';
char upperCaseLetter = Character.toUpperCase(letter);
System.out.println(upperCaseLetter);
// Output:
// 'A'
In this example, we use the Character.toUpperCase()
method to convert the lowercase character ‘a’ to its uppercase equivalent ‘A’.
Handling Unicode Characters
Java’s char
data type and Character
class support Unicode characters, allowing you to work with a wide range of characters from various languages.
Here’s an example of declaring a Unicode character:
char letter = 'A';
System.out.println(letter);
// Output:
// 'A'
In this example, we declare a character variable letter
and assign it the Unicode value for ‘A’ (which is 0041 in hexadecimal). We then print the value of letter
, which outputs ‘A’.
Exploring Alternative Approaches to Character Handling
While the char
data type and the Character
class are the primary tools for handling characters in Java, there are alternative approaches that you can use, especially when dealing with more complex scenarios.
Using the String
Class
The String
class in Java can be used to handle characters. This approach is particularly useful when you need to manipulate a sequence of characters.
Here’s an example of using the String
class to convert a character to uppercase:
String letter = "a";
String upperCaseLetter = letter.toUpperCase();
System.out.println(upperCaseLetter);
// Output:
// 'A'
In this example, we use the toUpperCase()
method of the String
class to convert the lowercase character ‘a’ to its uppercase equivalent ‘A’.
Leveraging Third-Party Libraries
There are also third-party libraries available that offer advanced character handling capabilities. For instance, Apache Commons Lang provides a variety of utilities for manipulating characters and strings.
Here’s an example of using Apache Commons Lang to swap the case of a character:
import org.apache.commons.lang3.StringUtils;
String letter = "a";
String swappedCaseLetter = StringUtils.swapCase(letter);
System.out.println(swappedCaseLetter);
// Output:
// 'A'
In this example, we use the swapCase()
method of Apache Commons Lang to swap the case of the character ‘a’, turning it into ‘A’.
While these alternative approaches offer additional flexibility, they come with their own trade-offs. For instance, using the String
class or third-party libraries might be overkill for simple character handling tasks. On the other hand, they can be quite handy for more complex tasks, such as string manipulation or handling special characters. Therefore, the choice of approach depends on the specific requirements of your task.
Troubleshooting Common Issues with Characters in Java
While working with characters in Java, you might encounter several common issues. Understanding these issues and knowing how to troubleshoot them can save you a lot of time and frustration.
Handling Encoding Errors
One common issue when working with characters in Java is encoding errors. These errors occur when you try to interpret a byte sequence as a character sequence using an incorrect character encoding.
Here’s an example of how an encoding error might occur:
String text = "Javä";
byte[] bytes = text.getBytes("ISO-8859-1");
String newText = new String(bytes, "UTF-8");
System.out.println(newText);
// Output:
// 'Jav?'
In this example, we encode a string containing a special character (‘ä’) using the ISO-8859-1 encoding and then try to decode it using the UTF-8 encoding. This results in an incorrect output, as the two encodings handle special characters differently.
The solution to this issue is to ensure that you’re using the correct character encoding when converting between byte sequences and character sequences.
Dealing with Special Characters
Another common issue when working with characters in Java is handling special characters, such as newline (\n
), tab (\t
), and backslash (\\
).
Here’s an example of how to escape a special character in Java:
String text = "Java \n 101";
System.out.println(text);
// Output:
// Java
// 101
In this example, we use the newline character (\n
) in a string. When we print the string, \n
creates a new line, resulting in the ‘101’ appearing on the line below ‘Java’.
By understanding these common issues and their solutions, you can work more effectively with characters in Java and avoid common pitfalls.
Deep Dive into Java Characters: Unicode, ASCII, and More
To effectively work with characters in Java, it’s crucial to understand the underlying concepts, such as Unicode and ASCII, and the difference between char
and Character
.
Unicode and ASCII: What’s the Difference?
Unicode and ASCII are both character encoding standards. ASCII, an acronym for American Standard Code for Information Interchange, was one of the first character encoding standards. It uses 7 bits to represent a character, allowing it to represent 128 different characters.
Unicode, on the other hand, is a more modern and comprehensive character encoding standard. It uses 16 bits to represent a character, allowing it to represent over 65,000 different characters. This wide range makes Unicode capable of representing almost every character in almost every language in the world.
Here’s an example of a Unicode character in Java:
char letter = '\u0041';
System.out.println(letter);
// Output:
// 'A'
In this example, we declare a character variable letter
and assign it the Unicode value for ‘A’ (which is 0041 in hexadecimal). We then print the value of letter
, which outputs ‘A’.
char
vs. Character
in Java
In Java, char
is a primitive data type, while Character
is a wrapper class. The char
data type can store a single 16-bit Unicode character, while the Character
class provides a number of methods for manipulating characters.
Here’s an example of using the Character
class in Java:
Character letter = new Character('A');
System.out.println(Character.isUpperCase(letter));
// Output:
// true
In this example, we create a Character
object letter
and assign it the value ‘A’. We then use the Character.isUpperCase()
method to check if letter
is an uppercase character, which returns true.
Understanding these concepts is fundamental to mastering character handling in Java.
Exploring Relevance of Character Handling in Java
Working with characters in Java is not just confined to basic programming tasks. It plays a crucial role in several other areas, including file I/O, networking applications, and more.
Character Handling in File I/O
When reading from or writing to a file, you often deal with characters. Understanding how to handle characters in Java can help you effectively read data from files or write data to files.
Networking Applications
In networking applications, data is often transmitted as sequences of characters. Being able to manipulate and interpret these characters is key to developing successful networking applications in Java.
Exploring Related Concepts
Mastering character handling in Java opens the door to understanding related concepts, such as string manipulation and regular expressions. These concepts are fundamental to many programming tasks in Java, from data validation to text processing.
Further Resources for Mastering Java Characters
To deepen your understanding of characters in Java, consider exploring the following resources:
- Use Cases of Primitive Data Types in Java – Understand the impact of variable scope on primitive data types in Java.
Byte Data Type in Java – Understand the range and usage of bytes in Java programming.
Char Data Type in Java – Learn about Unicode encoding and the range of characters supported by char.
Oracle Java Documentation is a comprehensive resource that covers all aspects of Java, including characters.
Baeldung’s Guide to Java Characters provides a deep dive into how to use the
char
data type and theCharacter
class.GeeksforGeeks’ Java Character Class Article offers a detailed look at the
Character
class in Java and its various methods.
Wrapping Up: Characters in Java
In this comprehensive guide, we’ve delved into the world of characters in Java, exploring everything from the basics to more advanced techniques.
We started with the basics, learning how to declare and manipulate characters using the char
keyword and the Character
class. We then delved into more complex operations, such as character encoding and decoding, and the handling of Unicode characters. Along the way, we also explored alternative approaches to character handling, including the use of the String
class and third-party libraries.
We tackled common issues you might encounter when working with characters in Java, such as encoding errors and issues with special characters, providing solutions to these challenges. We also took a deep dive into the underlying concepts, such as Unicode and ASCII, and the difference between char
and Character
.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
char Keyword | Simple and straightforward | Limited functionality |
Character Class | Provides various methods for character manipulation | More complex than using the char keyword |
String Class | Useful for manipulating a sequence of characters | Can be overkill for simple character handling tasks |
Third-Party Libraries | Offer advanced character handling capabilities | May require additional setup and learning |
Whether you’re a beginner just starting out with characters in Java or an experienced developer looking to enhance your skills, we hope this guide has provided you with a deeper understanding of characters in Java and how to work with them effectively.
Understanding how to work with characters in Java is a fundamental skill for any Java developer. With this guide, you’re now better equipped to handle characters in Java. Happy coding!