Linux gdb: GNU Debugger Usage Guide (with Examples)
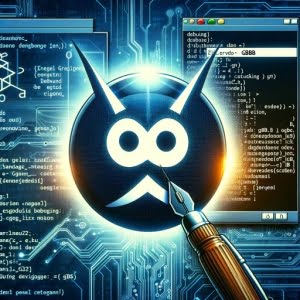
Ever felt like you’re wrestling with debugging your code in Linux? You’re not alone. Many developers find themselves puzzled when it comes to handling bugs in their Linux code, but we’re here to help.
Think of the ‘GDB’ command in Linux as a detective – a detective that can help you uncover the hidden bugs in your code. This powerful tool for debugging in Linux is a game-changer for many developers.
In this guide, we’ll walk you through the basics to advanced usage of the GDB command in Linux, from its installation, basic usage, to more advanced techniques, as well as alternative approaches.
Let’s get started and master the GDB Linux command!
TL;DR: How Do I Use the GDB Command in Linux?
To use
GDB
in Linux, you first compile your code with the-g
flag, then run it withgdb
. This allows you to debug your program and uncover any hidden bugs.
Here’s a simple example:
gcc -g myprogram.c
gdb ./a.out
In this example, we first compile the myprogram.c
file with the -g
flag using the gcc
command. This flag tells GCC to include extra debugging information in our executable. Then, we run the resulting executable (a.out
) with gdb
.
This is just a basic way to use the GDB command in Linux, but there’s much more to learn about debugging your programs effectively. Continue reading for more detailed instructions and advanced usage scenarios.
Table of Contents
The Basics: GDB Linux Command
The GDB command in Linux is a flexible and powerful tool for debugging, but like any tool, you need to know how to use it effectively.
Compiling Code with the -g Flag
Before we can debug with GDB, we need to compile our code with the -g
flag. This flag tells the compiler to include additional debugging information in the executable file, which GDB can use to help you find bugs.
Let’s take a look at an example. Suppose we have a simple C program, hello.c
, that prints ‘Hello, World!’.
gcc -g hello.c -o hello
In this example, we’re using the gcc
command to compile our hello.c
file. The -g
flag tells GCC to include debugging information, and the -o
option lets us specify the output file name.
Running Your Program with GDB
Once we’ve compiled our program with the -g
flag, we can run it with GDB. Here’s how you can do it:
gdb ./hello
This command starts GDB with our hello
program. Once GDB is running, you can use various commands to control the execution of your program and inspect its state.
Note: When you first run GDB, it might seem like nothing has happened. But don’t worry, GDB is waiting for your commands! You can start by typing
run
to start your program.
(gdb) run
# Output:
# Starting program: /path/to/hello
# Hello, World!
# [Inferior 1 (process 12345) exited normally]
In this example, we used the run
command to start our program. GDB responded by printing some information about the program’s execution, and then our program printed ‘Hello, World!’.
The GDB command in Linux is a powerful tool, but it’s also complex. It’s important to understand the basics before moving on to more advanced topics. By compiling your code with the -g
flag and running it with GDB, you’ve taken the first steps towards mastering this tool.
Advanced Features of GDB in Linux
As you get the hang of the basic functionalities of the GDB command in Linux, it’s time to explore its more advanced features. These include setting breakpoints, stepping through code, inspecting variables, and more.
Before we dive into these advanced uses, let’s familiarize ourselves with some of the command-line arguments or flags that can modify the behavior of the GDB command. Here’s a table with some of the most commonly used GDB arguments.
Argument | Description | Example |
---|---|---|
-tui | Use the Text User Interface. | gdb -tui ./hello |
-quiet | Start GDB without the introductory and copyright messages. | gdb -quiet ./hello |
-cd | Change to directory DIR. | gdb -cd /path/to/directory ./hello |
-symbols | Read symbols from file FILE. | gdb -symbols ./hello |
-write | Set writing into executable and core files. | gdb -write ./hello |
-command | Execute GDB commands from file FILE. | gdb -command commands.txt ./hello |
-core | Use file FILE as core dump. | gdb -core core.12345 ./hello |
-pid | Attach to running process PID. | gdb -pid 12345 |
-directory | Add directory DIR to the path to search for source files. | gdb -directory /path/to/directory ./hello |
-exec | Use file FILE as executable. | gdb -exec ./hello |
Now that we have a basic understanding of GDB command line arguments, let’s dive deeper into the advanced use of GDB.
Setting Breakpoints
One of the most powerful features of GDB is the ability to set breakpoints. A breakpoint is a specific point in the program where execution will stop. This allows you to inspect the state of the program at that point, which can be incredibly useful for debugging.
Here’s how you can set a breakpoint at the main function:
(gdb) break main
# Output:
# Breakpoint 1 at 0x4005f6: file hello.c, line 5.
In this example, we used the break
command to set a breakpoint at the main
function. GDB responded by telling us that it set a breakpoint at a specific address, which corresponds to the main
function in our hello.c
file.
Stepping Through Code
Once you’ve set a breakpoint, you can use the next
and step
commands to control the execution of your program. The next
command executes the next line of the program, while the step
command steps into functions.
Here’s an example of stepping through code with GDB:
(gdb) run
# Output:
# Starting program: /path/to/hello
(gdb) next
# Output:
# 6 printf("Hello, World!
");
In this example, we used the run
command to start our program, which stopped at the first line of the main
function because of our breakpoint. We then used the next
command to execute the next line of the program.
Inspecting Variables
GDB also allows you to inspect the values of variables at any point during the execution of your program. You can use the print
command to do this.
Here’s an example:
(gdb) print argc
# Output:
# $1 = 1
In this example, we used the print
command to print the value of the argc
variable, which is the number of command-line arguments. GDB responded by telling us that argc
is 1, which means our program was run without any additional command-line arguments.
These are just a few examples of the advanced features of the GDB command in Linux. By mastering these features, you can take full control of your program’s execution and find bugs more effectively.
Alternative Debugging Methods Beyond GDB
While GDB is a powerful tool for debugging in Linux, it’s not the only option. There are several alternative approaches to debugging that you might find useful, depending on your specific needs and circumstances. Let’s take a look at a couple of these alternatives: the lldb
command and third-party tools like Valgrind.
LLDB: A Modern Debugger
LLDB is a next-generation, high-performance debugger built on the LLVM framework. It offers a modern, flexible architecture and superior user experience.
Here’s how you can use LLDB to debug a simple program:
clang -g hello.c -o hello
lldb ./hello
# Output:
# (lldb) target create "/path/to/hello"
# Current executable set to '/path/to/hello' (x86_64).
In this example, we’re using the clang
compiler to compile our hello.c
file with the -g
flag, which tells the compiler to include debugging information. We then run the resulting executable (hello
) with lldb
.
Valgrind: A Suite of Debugging Tools
Valgrind is an open-source software that contains several debugging and profiling tools. One of its most popular tools is Memcheck, a memory error detector.
Here’s how you can use Valgrind to check for memory leaks in your program:
gcc hello.c -o hello
valgrind --leak-check=yes ./hello
# Output:
# ==12345== Memcheck, a memory error detector
# ==12345== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al.
# ==12345== Using Valgrind-3.13.0 and LibVEX; rerun with -h for copyright info
# ==12345== Command: ./hello
# ==12345==
# Hello, World!
# ==12345==
# ==12345== HEAP SUMMARY:
# ==12345== in use at exit: 0 bytes in 0 blocks
# ==12345== total heap usage: 1 allocs, 1 frees, 1,024 bytes allocated
# ==12345==
# ==12345== All heap blocks were freed -- no leaks are possible
# ==12345==
# ==12345== For counts of detected and suppressed errors, rerun with: -v
# ==12345== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
In this example, we’re using the gcc
compiler to compile our hello.c
file. We then run the resulting executable (hello
) with Valgrind’s --leak-check=yes
option, which checks for memory leaks.
As you can see, there’s more to debugging in Linux than just the GDB command. By exploring alternative approaches like LLDB and Valgrind, you can find the tools and techniques that work best for you.
Debugging Issues with GDB
While GDB is an extremely powerful tool, like any other software, you may encounter some issues when using it. In this section, we’ll discuss some common problems you might face while debugging with GDB, such as ‘Segmentation fault’ and ‘No symbol table’, and provide solutions and workarounds for each issue.
Dealing with Segmentation Faults
A ‘Segmentation fault’ is a specific kind of error caused by accessing memory that ‘does not belong to you’. It’s a mechanism that prevents you from corrupting the memory and introducing hard-to-debug memory bugs. Whenever you get a ‘Segmentation fault’, you know you are doing something wrong with memory – such as accessing a variable that has already been freed or writing to a read-only portion of memory.
The best way to handle a ‘Segmentation fault’ is to use GDB to figure out exactly where the segmentation fault is happening. Here’s an example:
gcc -g segfault.c -o segfault
gdb ./segfault
# Output:
# (gdb) run
# Starting program: /path/to/segfault
# Program received signal SIGSEGV, Segmentation fault.
# 0x000000000040052f in main () at segfault.c:5
# 5 *ptr = 100;
In this example, we’re using the gcc
compiler to compile our segfault.c
file with the -g
flag, which tells the compiler to include debugging information. We then run the resulting executable (segfault
) with GDB. The program crashes, and GDB tells us exactly where the segmentation fault occurred: at line 5 of segfault.c
.
No Symbol Table
Another common issue when using GDB is the ‘No symbol table’ error. This usually happens when you’re trying to debug a program without compiling it with the -g
flag.
To fix this issue, you need to recompile your program with the -g
flag. Here’s an example:
gcc -g nosymbols.c -o nosymbols
gdb ./nosymbols
# Output:
# (gdb) list
# 1 #include <stdio.h>
# 2
# 3 int main() {
# 4 printf("Hello, World!
");
# 5 return 0;
# 6 }
In this example, we’re using the gcc
compiler to compile our nosymbols.c
file with the -g
flag, which tells the compiler to include debugging information. We then run the resulting executable (nosymbols
) with GDB. The list
command shows us the source code of our program, indicating that the symbol table is indeed present.
These are just a couple of examples of the issues you might face when debugging with the GDB Linux command. Remember, the key to effective debugging is understanding your tool and knowing how to handle common issues. Happy debugging!
Understanding the Role of a Debugger
Before we delve deeper into the GDB Linux command, it’s crucial to understand the theory of debugging, the role of a debugger, and the concept of breakpoints. These fundamental concepts form the backbone of debugging in any programming environment.
The Theory of Debugging
Debugging is the process of identifying, isolating, and fixing problems or ‘bugs’ in computer code. It’s like being a detective, where the crime scene is your code, and the clues are the error messages or the incorrect behavior of your program.
While it might seem daunting at first, debugging is a skill that can be mastered with practice. The key is to be systematic and patient. Identify the symptoms, hypothesize the cause, test your hypothesis, and repeat until the bug is squashed.
The Role of a Debugger
A debugger is a tool that helps you in this debugging process. It allows you to control the execution of your program, inspect its state at any point, and change its state if necessary. In other words, a debugger gives you the superpower to manipulate time (at least, for your program).
For instance, consider this simple C program:
#include <stdio.h>
int main() {
int a = 5;
int b = 0;
int c = a / b;
printf("%d
", c);
return 0;
}
If you try to run this program, it will crash because it tries to divide by zero. But why is it crashing? Which line is causing the problem? That’s where a debugger like GDB comes in. It can tell you exactly where the program is crashing, and why.
Breakpoints and the Compilation Process
One of the key features of a debugger is the ability to set ‘breakpoints’. A breakpoint is a point in your code where the debugger will pause the execution of your program. This allows you to inspect the state of your program at that specific point, which can be incredibly useful for tracking down bugs.
To set breakpoints, you need to compile your code with debugging information. That’s where the -g
flag comes in. When you compile your code with gcc -g
, GCC includes extra information in the executable (such as line numbers and variable names) that GDB can use to help you debug.
Here’s an example:
gcc -g divide.c -o divide
gdb ./divide
# Output:
# (gdb) break main
# Breakpoint 1 at 0x40052d: file divide.c, line 5.
In this example, we’re using the gcc
compiler to compile our divide.c
file with the -g
flag, which tells the compiler to include debugging information. We then run the resulting executable (divide
) with GDB. The break main
command sets a breakpoint at the main
function.
Understanding these fundamentals of debugging can greatly enhance your ability to effectively use the GDB Linux command and other debugging tools.
Relevance and Further Exploration of GDB
GDB isn’t just a tool for simple debugging tasks; it’s a powerful ally that can make a significant difference in larger projects and in different programming languages. Its capabilities extend beyond the basics, providing you with the means to manage memory, debug multithreaded programs, and much more.
Impact on Larger Projects
In larger projects, the complexity of the codebase can increase exponentially. This is where GDB truly shines. By allowing you to set breakpoints, inspect variables, and control the execution flow, GDB helps you navigate through the labyrinth of complex code.
For instance, consider a large project with multiple files and thousands of lines of code. If your program crashes or behaves unexpectedly, it could be like finding a needle in a haystack. With GDB, you can set breakpoints in specific functions or lines of code, making it easier to isolate and identify the problematic part of your code.
Debugging in Different Programming Languages
One of the strengths of GDB is its wide language support. Apart from C and C++, GDB supports several other languages including Fortran, Go, and Python, among others. This makes GDB a versatile tool that can be used in a variety of programming environments.
For example, to debug a Python script with GDB, you can use the py
command followed by Python expressions. Here’s an example:
python -m pdb python_script.py
# Output:
# > /path/to/python_script.py(1)<module>()
# -> import sys
# (Pdb)
In this example, we’re using the Python debugger module (pdb
) to debug our python_script.py
file. The (Pdb)
prompt indicates that the debugger is waiting for our commands.
Exploring Related Concepts
Beyond basic and advanced debugging, GDB opens the door to exploring related concepts such as memory management and multithreaded debugging. Understanding these concepts can further enhance your debugging skills and make you a more proficient programmer.
For instance, GDB allows you to inspect memory directly, which can be useful for understanding how your program interacts with memory. Similarly, GDB provides commands for handling multithreaded programs, allowing you to control and inspect each thread separately.
Further Resources for Mastering GDB
To continue your journey in mastering the GDB Linux command, consider exploring the following resources:
- GNU Debugger Documentation: The official documentation for GDB, providing a comprehensive overview of its features.
Debugging with GDB – RMS’s gdb Tutorial: A detailed tutorial that covers the basics of debugging with GDB.
GDB Documentation: Sourceware’s official documentation provides a comprehensive guide to GDB (GNU Debugger).
Remember, mastering a tool like GDB takes time and practice. Don’t be discouraged if you don’t understand everything at once. Keep experimenting, keep learning, and most importantly, keep debugging!
Wrapping Up: Mastering the GDB Linux Command
In this comprehensive guide, we’ve navigated through the process of debugging with the GDB Linux command, from basic usage to advanced techniques, and even alternative approaches.
We began with the basics, understanding how to compile code with the -g
flag and running it with GDB. We then delved into the depths of GDB, exploring advanced techniques such as setting breakpoints, stepping through code, and inspecting variables. Along the way, we faced common issues like ‘Segmentation fault’ and ‘No symbol table’, and provided solutions to overcome these hurdles.
Furthermore, we ventured beyond GDB, discussing alternative debugging tools such as LLDB and Valgrind. Each tool has its unique strengths and can be a powerful ally depending on the situation and requirements.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
GDB | Powerful, supports many languages | May require troubleshooting for complex programs |
LLDB | Modern, built on LLVM framework | Less widely used than GDB |
Valgrind | Comprehensive suite of debugging tools | Higher learning curve |
Whether you’re just starting out with GDB or looking to enhance your debugging skills, we hope this guide has given you a deeper understanding of the GDB Linux command and its capabilities.
With its robust features and wide language support, GDB is an indispensable tool for debugging in Linux. Keep experimenting, keep learning, and most importantly, keep debugging!