The Complete Guide to Linux Git Commands
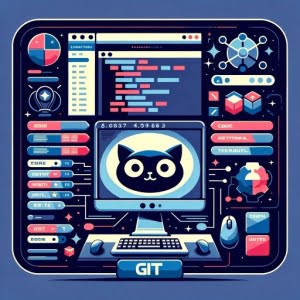
Are you finding it challenging to use Git commands in Linux? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Think of ‘git’ as your personal time machine, allowing you to track and revert changes in your code. It’s a powerful utility that can seamlessly manage your code changes in a Linux environment.
This guide will walk you through the essentials of using Git commands in a Linux environment, from basic to advanced usage. We’ll explore Git’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Git commands in Linux!
TL;DR: How Do I Use Git Commands in Linux?
git
commands in Linux are used to manage and track changes in your code. The most commongit
command isgit clone https://github.com/user/repo.git
, which clones a repository into a new directory.
Here’s a simple example:
# Clone a repository into a new directory named 'my-repo'
git clone https://github.com/user/repo.git my-repo
In this example, we use the git clone
command to clone a repository from a given URL into a new directory named ‘my-repo’. This is a basic Git command that you’ll use frequently when working with Git in Linux.
But there’s so much more to Git than just cloning repositories. Continue reading for a comprehensive guide on using Git commands in Linux, from basic to advanced usage.
Table of Contents
- Getting Started with Git Commands in Linux
- Advanced Git Commands in Linux: Branching, Merging, Rebasing, and More
- Exploring Alternative Approaches to Git Usage
- Troubleshooting Common Git Issues in Linux
- Understanding Version Control Systems
- Diving into Git’s Architecture and Workflow
- Exploring the Relevance of Git Commands in Larger Projects and Team Collaboration
- Wrapping Up: Mastering Git Commands in Linux
Getting Started with Git Commands in Linux
Initializing a Git Repository
The first step in using Git in Linux is to initialize a Git repository. This can be done using the git init
command. Here’s an example:
cd /path/to/your/project
git init
# Output:
# Initialized empty Git repository in /path/to/your/project/.git/
In this example, we navigate to our project directory using the cd
command, then run git init
. This initializes a new Git repository in our project directory.
Staging Changes
Once you’ve made some changes to your code, you’ll want to stage those changes. This can be done using the git add
command. Here’s an example:
git add .
# Output:
# Changes to be committed:
# new file: example.txt
In this example, git add .
stages all changes in the current directory. The output shows that our new file example.txt
has been staged.
Committing Changes
After staging your changes, you’ll want to commit them. This can be done using the git commit
command. Here’s an example:
git commit -m 'Add example.txt'
# Output:
# [master (root-commit) 0a1b2c3] Add example.txt
# 1 file changed, 1 insertion(+)
# create mode 100644 example.txt
In this example, git commit -m 'Add example.txt'
commits our staged changes with a message describing what we’ve done.
Pushing Changes to a Remote Repository
Finally, you’ll want to push your changes to a remote repository. This can be done using the git push
command. Here’s an example:
git push origin master
# Output:
# Counting objects: 3, done.
# Writing objects: 100% (3/3), 218 bytes | 218.00 KiB/s, done.
# Total 3 (delta 0), reused 0 (delta 0)
# To github.com:user/repo.git
# * [new branch] master -> master
In this example, git push origin master
pushes our changes to the ‘master’ branch of our remote repository ‘origin’. The output shows that our changes have been successfully pushed.
That’s it for the basics of using Git commands in Linux! Stay tuned for our discussion on more advanced Git commands.
Advanced Git Commands in Linux: Branching, Merging, Rebasing, and More
As you become more proficient with Git, you’ll discover that it’s more than just a tool for tracking changes – it’s a powerful system for managing your codebase. This section will delve into some of the more advanced Git commands in Linux, such as branching, merging, rebasing, and resolving merge conflicts.
Before we dive into these advanced commands, let’s familiarize ourselves with some of the command-line arguments or flags that can modify the behavior of the Git command. Here’s a table with some of the most commonly used Git arguments.
Argument | Description | Example |
---|---|---|
-a | Tells the command to consider all files. | git add -a |
-m | Specifies a commit message. | git commit -m 'Add feature' |
-u | Updates or stages only the tracked files. | git add -u |
-v | Verbose mode, shows the diff in the commit message screen. | git commit -v |
-n | Dry run, show what would be done but do not execute anything. | git push -n |
--amend | Amend the last commit. | git commit --amend |
-d | Delete a branch. | git branch -d my-branch |
-D | Force delete a branch. | git branch -D my-branch |
--no-ff | Creates a new commit when merging. | git merge --no-ff my-branch |
--rebase | Reapply commits on top of another base tip. | git rebase master |
Now that we have a basic understanding of Git command line arguments, let’s dive deeper into the advanced use of Git.
Exploring Alternative Approaches to Git Usage
Embracing Git GUIs and Other Tools
While the command-line interface of Git provides powerful functionality, it can sometimes be complex and intimidating, especially for beginners. This is where Git GUIs and other tools come into play. These tools provide a more interactive and user-friendly interface for managing your Git repositories, making it easier to visualize changes and manage your code.
Here’s an example using GitKraken, a popular Git GUI:
# Open GitKraken and clone a repository
# File > Clone Repo > Clone
# Enter URL: https://github.com/user/repo.git
# Set local path: /path/to/your/project
# Click 'Clone the repo!'
In this example, we open GitKraken, navigate to ‘File > Clone Repo’, enter the URL of our repository, set our local path, and click ‘Clone the repo!’. This clones our repository into a new directory, just like the git clone
command we discussed earlier.
Command-line Git vs. GUI Git: A Comparison
Command-line Git and GUI Git each have their own strengths and weaknesses. The command-line interface offers more control and flexibility, allowing you to execute complex commands and scripts. On the other hand, GUI tools provide a more intuitive interface, making it easier to visualize changes and navigate your repository.
Here’s a quick comparison of command-line Git and GUI Git:
Feature | Command-line Git | GUI Git |
---|---|---|
Control and Flexibility | High | Medium |
Ease of Use | Medium | High |
Visualization | Low | High |
Learning Curve | Steep | Gentle |
Whether you prefer command-line Git or GUI Git ultimately depends on your needs and preferences. If you’re comfortable with the command line and need the flexibility it offers, command-line Git is the way to go. If you prefer a more user-friendly interface and don’t mind sacrificing some control, GUI Git might be a better fit for you.
Troubleshooting Common Git Issues in Linux
Resolving Merge Conflicts
One common issue when using Git is encountering a merge conflict. This happens when Git is unable to automatically merge changes from different branches. Here’s an example of a merge conflict and how to resolve it:
git merge feature-branch
# Output:
# Auto-merging example.txt
# CONFLICT (content): Merge conflict in example.txt
# Automatic merge failed; fix conflicts and then commit the result.
In this example, Git is unable to merge ‘feature-branch’ into our current branch due to a conflict in ‘example.txt’. To resolve this conflict, we need to manually edit ‘example.txt’, remove the conflict markers, and make the necessary changes.
Dealing with ‘detached HEAD’ State
Another common issue is ending up in a ‘detached HEAD’ state. This happens when you check out a commit instead of a branch. Here’s an example of how to resolve a ‘detached HEAD’ state:
git checkout 0a1b2c3
# Output:
# You are in 'detached HEAD' state. You can look around, make experimental
# changes and commit them, and you can discard any commits you make in this
# state without impacting any branches by performing another checkout.
In this example, we’ve checked out a commit, which puts us in a ‘detached HEAD’ state. To resolve this, we can simply check out a branch using git checkout master
.
Recovering Lost Commits
Sometimes, you might accidentally lose commits, either by using git reset
or by other means. Here’s an example of how to recover lost commits using git reflog
and git cherry-pick
:
git reflog
# Output:
# 0a1b2c3 HEAD@{0}: commit: Add feature
# ...
git cherry-pick 0a1b2c3
# Output:
# [master 0a1b2c3] Add feature
# 1 file changed, 1 insertion(+)
In this example, we use git reflog
to find the commit hash of our lost commit, then use git cherry-pick
to apply that commit to our current branch.
These are just a few examples of the issues you might encounter when using Git commands in Linux. Remember, Git is a powerful tool, but with that power comes complexity. Don’t be discouraged if you run into issues – with patience and practice, you’ll become a Git master in no time!
Understanding Version Control Systems
Version Control Systems (VCS) serve as the backbone for effective code management. They track changes to a file or set of files over time, allowing you to revisit specific versions later. There are two types of VCS: Centralized Version Control Systems (CVCS) and Distributed Version Control Systems (DVCS). Git falls into the latter category, providing each user a full-fledged copy of the entire codebase.
# Clone a repository with Git
git clone https://github.com/user/repo.git
# Output:
# Cloning into 'repo'...
# remote: Enumerating objects: 13, done.
# remote: Total 13 (delta 0), reused 0 (delta 0), pack-reused 13
# Unpacking objects: 100% (13/13), done.
In this example, the git clone
command is used to clone a repository, which is essentially creating a complete copy of the entire codebase on your local machine.
Diving into Git’s Architecture and Workflow
Git’s architecture can be broken down into three main areas: the Working Directory, the Staging Area, and the Git Directory (repository).
- Working Directory: This is where you’ll be doing all your work: creating, editing, deleting and organizing files.
Staging Area: Also known as the ‘Index’, this is an area where Git tracks and saves changes that happen in the Working Directory. It’s a preparatory space for changes that will be committed.
Git Directory (Repository): This is where Git stores the metadata and object database for your project. It’s the most integral part of Git and it’s what is copied when you clone a repository from another computer.
The basic Git workflow goes something like this:
- Modify files in your working directory.
- Stage the files, adding snapshots of them to your staging area.
- Perform a commit, which takes the files as they are in the staging area and stores that snapshot permanently to your Git directory.
# Modify a file
echo 'Hello, World!' > hello.txt
# Stage the file
git add hello.txt
# Commit the file
git commit -m 'Add hello.txt'
# Output:
# [master (root-commit) 0a1b2c3] Add hello.txt
# 1 file changed, 1 insertion(+)
# create mode 100644 hello.txt
In this example, we create a new file ‘hello.txt’, stage it with git add
, and commit it with git commit
. The output shows that our new file has been successfully committed.
Exploring the Relevance of Git Commands in Larger Projects and Team Collaboration
Git is not just a tool for individual developers. It’s a powerful system for team collaboration, enabling multiple developers to work on the same project without stepping on each other’s toes. This is made possible by Git’s branch-based workflow, which allows each developer to work on their own branch without affecting the main codebase.
# Create a new branch for a feature
git checkout -b feature-branch
# Output:
# Switched to a new branch 'feature-branch'
In this example, we use the git checkout -b
command to create a new branch for our feature. This allows us to work on our feature without affecting the ‘master’ branch.
Git commands are also relevant in the context of Continuous Integration/Continuous Delivery (CI/CD), a practice that involves regularly integrating code changes and delivering them to production quickly and reliably. Git plays a crucial role in CI/CD pipelines, allowing developers to manage and track their code changes effectively.
Diving Deeper into GitHub and GitLab
GitHub and GitLab are two popular platforms that provide a web-based interface for managing Git repositories. They offer additional features like issue tracking, code reviews, and more, making them invaluable tools for team collaboration.
# Push changes to GitHub or GitLab
git push origin feature-branch
# Output:
# Counting objects: 3, done.
# Writing objects: 100% (3/3), 218 bytes | 218.00 KiB/s, done.
# Total 3 (delta 0), reused 0 (delta 0)
# To github.com:user/repo.git
# * [new branch] feature-branch -> feature-branch
In this example, we use the git push
command to push our changes to our ‘feature-branch’ on GitHub or GitLab. This allows our team to review our changes and merge them into the main codebase when they’re ready.
Further Resources for Mastering Git Commands
If you’re interested in learning more about Git commands in Linux, here are some resources that you might find helpful:
- Pro Git Book – This is an in-depth guide to Git, available for free online.
Git Documentation – The official Git documentation is a comprehensive resource for all things Git.
Atlassian Git Tutorial – Atlassian, the company behind Bitbucket, offers a series of Git tutorials that cover everything from the basics to advanced topics.
Wrapping Up: Mastering Git Commands in Linux
In this comprehensive guide, we’ve journeyed through the essentials of using Git commands in Linux, from basic to advanced usage. We’ve explored Git’s core functionality, delved into its advanced features, and discussed common issues and their solutions.
We began with the basics, learning how to initialize a Git repository, stage changes, commit changes, and push changes to a remote repository. We then ventured into more advanced territory, exploring Git commands such as branching, merging, rebasing, and resolving merge conflicts. Along the way, we tackled common challenges you might face when using Git commands in Linux, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to Git usage, introducing Git GUIs and other tools that can simplify Git usage. We compared command-line Git and GUI Git, giving you a sense of the broader landscape of tools for managing your Git repositories. Here’s a quick comparison of these approaches:
Approach | Control and Flexibility | Ease of Use | Visualization |
---|---|---|---|
Command-line Git | High | Medium | Low |
GUI Git | Medium | High | High |
Whether you’re just starting out with Git or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Git commands in Linux and their capabilities.
With its balance of control, flexibility, and ease of use, Git is a powerful tool for managing your codebase in a Linux environment. Happy coding!