JavaScript .filter() Method: Array Manipulation Guide
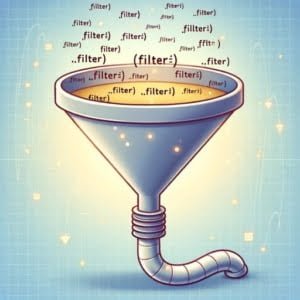
Are you finding it challenging to filter out elements in a JavaScript array? You’re not alone. Many developers find themselves in a similar situation, but there’s a method that can make this process a breeze.
Think of the JavaScript filter method as a sieve, it can help you sift through an array and keep only what you need. It’s a powerful tool that can streamline your code and make your data manipulation tasks more efficient.
This guide will walk you through the use of the JavaScript filter method, from basic usage to advanced techniques. We’ll explore the core functionality of the filter method, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the JavaScript filter method!
TL;DR: How Do I Use the Filter Method in JavaScript?
The filter method in JavaScript is used to create a new array with all elements that pass a test implemented by a provided function, used with the syntax
let newArray = arrayName.filter('filterCode')
. It’s a powerful tool for data manipulation and can be used in a variety of scenarios.
Here’s a simple example:
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers);
// Output:
// [2, 4]
In this example, we’ve used the filter
method to create a new array (evenNumbers
) from numbers
. The filter
method tests each element in the numbers
array with the provided function (number => number % 2 === 0
), and only elements that pass the test (i.e., even numbers) are included in the new array.
This is just a basic way to use the filter method in JavaScript, but there’s much more to learn about this versatile method. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of JavaScript Filter Method
- Advanced JavaScript Filter Techniques
- Exploring Alternative Array Filtering Techniques in JavaScript
- Troubleshooting Common Issues with JavaScript Filter Method
- Understanding JavaScript Arrays and Callback Functions
- The Relevance of JavaScript Filter Method in Programming
- Wrapping Up: JavaScript .filter() Method
Understanding the Basics of JavaScript Filter Method
The JavaScript filter method is a built-in array function that filters out elements in an array based on a test function, creating a new array with the elements that pass the test. This method does not mutate the original array, which is a key advantage when you want to avoid side effects in your code.
Let’s look at a simple code example to understand how this works:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
let filteredNumbers = numbers.filter(number => number > 5);
console.log(filteredNumbers);
// Output:
// [6, 7, 8, 9, 10]
In this example, we have an array of numbers from 1 to 10. We want to filter out numbers that are greater than 5. To do this, we use the filter
method with a test function number => number > 5
. This function checks each element in the numbers
array, and if the element is greater than 5, it passes the test and is included in the filteredNumbers
array. The result is a new array with only the numbers greater than 5.
Advantages of the Filter Method
The filter method is a powerful tool for data manipulation in JavaScript. It allows you to create a new array based on a condition, without modifying the original array. This is particularly useful when you want to preserve the original data while creating a subset of it.
Potential Pitfalls of the Filter Method
While the filter method is incredibly useful, it’s important to be aware of its potential pitfalls. One common issue is that if no elements pass the test function, the filter method will return an empty array. This is not necessarily a problem, but it’s something you should be aware of when using this method.
Advanced JavaScript Filter Techniques
As you become more comfortable with the JavaScript filter method, you can start to unlock its full potential by combining it with other array methods. Two such methods that work well with filter are map
and reduce
.
Combining Filter with Map
The map
method creates a new array populated with the results of calling a provided function on every element in the array. When used in conjunction with the filter
method, you can filter an array and then transform the filtered elements.
Let’s look at an example:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
let filteredAndMappedNumbers = numbers.filter(number => number > 5).map(number => number * 2);
console.log(filteredAndMappedNumbers);
// Output:
// [12, 14, 16, 18, 20]
In this example, we first filter the numbers
array to get numbers greater than 5 and then use the map
method to multiply each of these numbers by 2. The result is a new array with the filtered and transformed numbers.
Combining Filter with Reduce
The reduce
method applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single output value. It can be used with the filter
method to reduce the filtered array to a single value.
Here’s an example:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
let sumOfFilteredNumbers = numbers.filter(number => number > 5).reduce((sum, number) => sum + number, 0);
console.log(sumOfFilteredNumbers);
// Output:
// 40
In this code, we first filter the numbers
array to get numbers greater than 5. We then use the reduce
method to calculate the sum of these numbers. The result is the sum of all numbers in the array that are greater than 5.
By combining the filter
method with other array methods like map
and reduce
, you can perform complex data manipulation tasks in a clean and efficient way.
Exploring Alternative Array Filtering Techniques in JavaScript
While the filter
method is a go-to choice for array filtering in JavaScript, it’s not the only tool at your disposal. There are other techniques you can use to filter arrays, such as using the reduce
method or a for
loop. Let’s explore these alternatives.
Filtering with Reduce Method
The reduce
method is primarily used to reduce the elements of an array to a single output value. However, with a little creativity, you can also use it for filtering. Here’s how:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
let filteredNumbers = numbers.reduce((accumulator, number) => {
if (number > 5) {
accumulator.push(number);
}
return accumulator;
}, []);
console.log(filteredNumbers);
// Output:
// [6, 7, 8, 9, 10]
In this example, we’re using reduce
to filter out numbers greater than 5. The accumulator
starts as an empty array, and we push numbers that pass our test into it. The result is similar to what we’d get with filter
.
Filtering with a For Loop
A for
loop is a more manual, but still effective way to filter an array. Here’s an example:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
let filteredNumbers = [];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] > 5) {
filteredNumbers.push(numbers[i]);
}
}
console.log(filteredNumbers);
// Output:
// [6, 7, 8, 9, 10]
In this code, we iterate over the numbers
array with a for
loop, and push numbers that pass our test into the filteredNumbers
array. While this method requires more code than filter
, it offers more control over the filtering process.
Choosing the Right Method
Each of these methods has its advantages and disadvantages. The filter
method is simple and declarative, but it may not offer the control you need for more complex tasks. The reduce
method offers more flexibility, but it can be harder to read. A for
loop offers the most control, but it requires more code and can be prone to errors. Choose the method that best fits your needs and coding style.
Troubleshooting Common Issues with JavaScript Filter Method
While the JavaScript filter method is a powerful tool, it’s not without its challenges. Here, we’ll discuss some common issues you may encounter when using this method, along with their solutions and workarounds.
Understanding the Callback Function
One common issue that developers face when using the filter
method is understanding the callback function. The callback function is the function that filter
uses to test each element in the array. It should return true
for elements that should be included in the new array, and false
for those that shouldn’t.
If your filter
method isn’t working as expected, check your callback function. Make sure it’s correctly implemented and returning the expected results.
Dealing with Empty Arrays
Another common issue is dealing with empty arrays. If no elements in the array pass the test implemented by the provided function, filter
will return an empty array. This isn’t necessarily a problem, but it’s something you should be aware of.
If you’re getting an empty array and you’re not sure why, check your test function and your input array. Make sure your test function is correctly identifying the elements you want to include, and that your input array actually contains elements that should pass the test.
Here’s an example of how you can handle empty arrays:
let numbers = [1, 2, 3, 4, 5];
let filteredNumbers = numbers.filter(number => number > 10);
if (filteredNumbers.length === 0) {
console.log('No numbers passed the test.');
} else {
console.log(filteredNumbers);
}
// Output:
// 'No numbers passed the test.'
In this example, we’re trying to filter numbers greater than 10 from the numbers
array. Since no numbers pass this test, filter
returns an empty array. We then check the length of the filteredNumbers
array, and log a message if it’s empty.
By understanding these common issues and how to handle them, you can use the JavaScript filter method more effectively and avoid potential pitfalls.
Understanding JavaScript Arrays and Callback Functions
To fully grasp the JavaScript filter method, it’s essential to understand the basics of JavaScript arrays and callback functions.
JavaScript Arrays
An array in JavaScript is a single variable used to store different elements. It’s an ordered collection where each element can be accessed by its index (its position in the array).
let fruits = ['apple', 'banana', 'cherry'];
console.log(fruits[0]);
// Output:
// 'apple'
In this example, we created an array fruits
and accessed the first element using its index 0
.
JavaScript Callback Functions
In JavaScript, functions are objects, meaning they can be passed as arguments to other functions. A function that is passed as an argument to another function is known as a callback function.
function greet(name, callback) {
console.log('Hello ' + name);
callback();
}
function sayGoodbye() {
console.log('Goodbye!');
}
greet('John', sayGoodbye);
// Output:
// 'Hello John'
// 'Goodbye!'
In this example, sayGoodbye
is a callback function. It’s passed as an argument to the greet
function and is called within the greet
function.
Understanding these fundamentals will help you better understand how the filter
method works in JavaScript. The filter
method creates a new array by testing each element in the original array with a callback function. Only elements that pass the test (i.e., the callback function returns true
) are included in the new array.
The Relevance of JavaScript Filter Method in Programming
The JavaScript filter
method is more than just a tool for manipulating arrays. It’s part of a larger programming paradigm known as functional programming, which emphasizes the use of pure functions and avoids changing-state and mutable data.
Data Manipulation with Filter Method
In data-focused applications, the filter
method is invaluable. It allows you to sift through large amounts of data and extract what you need without altering the original data source. This is particularly useful in scenarios where data integrity is crucial.
let users = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 }
];
let youngUsers = users.filter(user => user.age < 30);
console.log(youngUsers);
// Output:
// [ { name: 'Alice', age: 25 } ]
In this code block, we have an array of user objects. We use the filter
method to create a new array that only includes users who are younger than 30. This is a simple example of how the filter
method can be used for data manipulation.
The Filter Method and Functional Programming
The filter
method is a staple in functional programming. By avoiding changes to the original array and returning a new array, it adheres to the principles of immutability and pure functions. This can lead to code that is easier to understand, test, and debug.
Further Resources for Mastering JavaScript .filter()
To deepen your understanding of the JavaScript filter
method and its applications, check out these resources:
- MDN Web Docs: Array.prototype.filter(): A comprehensive guide to the
filter
method from the official Mozilla Developer Network. - JavaScript.info: Array methods: An in-depth tutorial on array methods, including
filter
. - Eloquent JavaScript: Higher-Order Functions: A chapter from Eloquent JavaScript that covers higher-order functions, including
filter
.
Wrapping Up: JavaScript .filter() Method
In this comprehensive guide, we’ve journeyed through the powerful JavaScript filter
method, a versatile tool for array manipulation.
We began with the basics, understanding how to use the filter
method to create a new array from an existing one, based on a test function. We then explored more advanced techniques, such as combining filter
with other array methods like map
and reduce
for more complex data manipulation tasks.
We also tackled common issues that you might encounter when using the filter
method, such as understanding the callback function and dealing with empty arrays. We provided solutions and workarounds for each issue to help you overcome these challenges.
In addition, we looked at alternative approaches to array filtering in JavaScript, such as using the reduce
method or a for
loop. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Filter | Simple, declarative, doesn’t mutate original array | Returns an empty array if no elements pass the test |
Reduce | More flexible, can be used for filtering and other tasks | More complex, can be harder to read |
For Loop | Offers the most control | Requires more code, can be prone to errors |
Whether you’re just starting out with the JavaScript filter
method or you’re looking to level up your array manipulation skills, we hope this guide has given you a deeper understanding of the filter
method and its capabilities.
With its balance of simplicity, flexibility, and control, the JavaScript filter
method is a powerful tool for array manipulation. Now, you’re well equipped to enjoy those benefits. Happy coding!