jq Commands in Linux | Code Examples for JSON Parsing
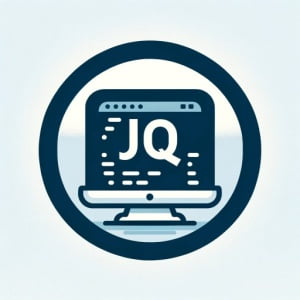
Are you finding it challenging to parse JSON data from the command line? You’re not alone. Many developers find themselves in a similar predicament, but there’s a tool that can make this process a breeze.
Like a Swiss Army knife, the jq command in Linux can slice and dice JSON data with ease. This powerful tool can transform your JSON handling experience, making it an essential part of your Linux toolkit.
In this guide, we’ll walk you through the ins and outs of using the jq command in Linux, from basic usage to advanced techniques. We’ll cover everything from simple parsing tasks to complex data manipulation, as well as troubleshooting common issues.
So, let’s dive in and start mastering the jq command!
TL;DR: How Do I Use jq Commands in Linux?
The
jq
command in Linux is a powerful tool for parsing and manipulating JSON data directly from the command line. To utilize, you must first create JSON Objects with the echo command,echo '{"name":"John"}'
. Once created you can access jq commands with the syntax,| jq [command]
. Here’s an example of how you can use it:
echo '{"name":"John"}' | jq '.name'
# Output:
# "John"
In this example, we’re using the echo command to create a simple JSON object with a single property: 'name'
with a value of 'John'
. This JSON object is then piped into the jq command, which extracts the value of the ‘name’ property and prints it to the console.
This is just a basic usage of the jq command. There’s so much more you can do with it, including filtering, mapping, and reducing data. Continue reading for more detailed usage scenarios and advanced techniques.
Table of Contents
- Getting Started with jq Command
- Diving Deeper: Advanced Usage of jq Command
- Exploring Alternatives: Other Tools for Parsing JSON
- Resolving Common jq Command Issues
- Understanding JSON: The Backbone of Data Exchange
- The Need for Tools like jq
- Basics of the Linux Command Line
- Real-World Applications of jq Command
- Wrapping Up: jq Command in Linux
Getting Started with jq Command
The jq command in Linux is a versatile tool that allows you to parse and manipulate JSON data right from your command line. It’s a handy tool that can simplify working with JSON data.
Let’s look at a simple example of how you can use the jq command to parse JSON data.
echo '{"name":"John", "age":30}' | jq '.age'
# Output:
# 30
In this example, we’re using the echo command to create a simple JSON object with two properties: ‘name’ with a value of ‘John’ and ‘age’ with a value of 30. This JSON object is then piped into the jq command, which extracts the value of the ‘age’ property and prints it to the console.
Advantages of Using jq
The jq command offers several advantages. It’s lightweight, easy to use, and can handle complex JSON data. It can also be combined with other Linux commands to create powerful data processing pipelines.
Potential Pitfalls
While jq is a powerful tool, it’s not without its potential pitfalls. If not used properly, you can end up with syntax errors or unexpected output. It’s important to understand the syntax and usage of the jq command to avoid these issues. In the upcoming sections, we’ll cover some common issues and how to troubleshoot them.
Diving Deeper: Advanced Usage of jq Command
As you become more comfortable with the basic usage of the jq command, you’ll find that its true power lies in its advanced features. The jq command’s flexibility allows it to handle more complex JSON manipulation tasks, such as filtering, mapping, and reducing data. Let’s explore some of these advanced uses.
Before we dive into the advanced usage of jq, let’s familiarize ourselves with some of the command-line arguments or flags that can modify the behavior of the jq command. Here’s a table with some of the most commonly used jq arguments.
Argument | Description | Example |
---|---|---|
-r | Outputs raw strings instead of JSON-encoded strings. | echo '{"name":"John"}' | jq -r '.name' |
-c | Compact output: instead of pretty-printing, jq outputs the JSON in compact form. | echo '{"name":"John"}' | jq -c |
-n | Allows jq to process a non-JSON input. | jq -n '{foo:1}' |
-e | Sets the exit status to 0 if the filter’s result is neither false nor null. | echo '{"name":"John"}' | jq -e '.name' |
-s | Reads (slurps) all input into an array. | echo -e '{"name":"John"}{"name":"Doe"}' | jq -s |
-f | Takes a filename as the argument and uses the file content as the filter. | jq -f filter.jq file.json |
-M | Monochrome output: disables colorized output. | echo '{"name":"John"}' | jq -M |
-C | Colorize output. | echo '{"name":"John"}' | jq -C |
-S | Sorts keys of objects on output. | echo '{"b":1, "a":2}' | jq -S |
-R | Reads raw input instead of parsing input as JSON. | jq -R '.' file.txt |
--arg | Passes a value to the jq script. | jq --arg key value -n '$key' |
Now that we have a basic understanding of jq command line arguments, let’s dive deeper into the advanced use of jq.
Filtering Data with jq
One of the most common advanced uses of jq is filtering data. You can filter data based on certain conditions. Here’s an example:
echo '[{"name":"John", "age":30}, {"name":"Doe", "age":20}]' | jq '.[] | select(.age > 25)'
# Output:
# {"name":"John", "age":30}
In this example, we’re using the jq command to filter out objects where the ‘age’ property is greater than 25. As you can see, only the object with ‘name’ as ‘John’ and ‘age’ as 30 is returned.
Mapping Data with jq
Another advanced use of jq is mapping data. You can transform the data into a new format. Here’s an example:
echo '[{"name":"John", "age":30}, {"name":"Doe", "age":20}]' | jq 'map({name, old: .age > 25})'
# Output:
# [{"name":"John", "old":true}, {"name":"Doe", "old":false}]
In this example, we’re using the jq command to transform the input array into a new format. We’re creating a new property ‘old’ which is true if the ‘age’ property is greater than 25.
Reducing Data with jq
You can also use jq to reduce an array of objects to a single value. Here’s an example:
echo '[{"name":"John", "age":30}, {"name":"Doe", "age":20}]' | jq 'map(.age) | add'
# Output:
# 50
In this example, we’re using the jq command to add up the ‘age’ properties of all objects in the array. The output is the total age, which is 50.
Transforming JSON Data with jq
jq can be used to transform JSON data into a different format. Let’s say we have a JSON object with ‘name’ and ‘age’ fields, but we want to transform it into an object with ‘firstName’ and ‘yearOfBirth’ fields.
Here’s how you can do that with jq:
echo '{"name": "John", "age": 30}' | jq '{firstName: .name, yearOfBirth: (2022 - .age)}'
# Output:
# {"firstName": "John", "yearOfBirth": 1982}
In this example, we’re transforming the original JSON object into a new one. We’re keeping the ‘name’ field but renaming it to ‘firstName’, and we’re converting the ‘age’ field into a ‘yearOfBirth’ field.
Chaining Operations with jq
jq also allows you to chain multiple operations together. This can be incredibly powerful when you’re working with complex JSON data. Here’s an example:
echo '[{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]' | jq 'map(.name)'
# Output:
# ["John", "Jane"]
In this example, we’re using the ‘map’ function to extract the ‘name’ field from each object in the array. The result is a new array that contains only the names.
The advanced use of the jq command can greatly simplify the process of handling JSON data in Linux. However, it’s important to be aware of potential issues, such as syntax errors or unexpected output, and how to troubleshoot them. In the next section, we’ll cover some common issues and their solutions.
Exploring Alternatives: Other Tools for Parsing JSON
While the jq command is a powerful tool for parsing JSON data in Linux, it’s not the only option available. There are other tools and methods you can use to parse JSON data from the command line. Let’s explore some of these alternatives.
Python’s JSON Module
Python, a popular high-level programming language, has a built-in module for handling JSON data. This module can be used to parse JSON data from the command line. Here’s an example:
import json
json_string = '{"name":"John", "age":30}'
data = json.loads(json_string)
print(data['age'])
# Output:
# 30
In this example, we’re using Python’s json module to parse a JSON string and extract the value of the ‘age’ property. This method is straightforward and doesn’t require any additional tools if you have Python installed.
However, Python’s JSON module is not as flexible as the jq command when it comes to filtering, mapping, and reducing data. It’s also slower and consumes more memory, which can be an issue when dealing with large JSON data.
JavaScript’s JSON.parse Method
JavaScript, the language of the web, also has a built-in method for parsing JSON data: JSON.parse. This method can be used in a Node.js environment to parse JSON data from the command line. Here’s an example:
const json_string = '{"name":"John", "age":30}';
const data = JSON.parse(json_string);
console.log(data.age);
# Output:
# 30
In this example, we’re using JavaScript’s JSON.parse method to parse a JSON string and log the value of the ‘age’ property to the console. This method is also straightforward and doesn’t require any additional tools if you have Node.js installed.
However, like Python’s JSON module, JavaScript’s JSON.parse method is not as powerful as the jq command when it comes to advanced data manipulation tasks. It’s also slower and consumes more memory.
In conclusion, while there are alternative methods for parsing JSON data from the command line, the jq command in Linux offers a balance of power, flexibility, and performance that makes it an excellent choice for most JSON parsing tasks.
Resolving Common jq Command Issues
The jq command, while powerful and flexible, can sometimes present challenges, especially when dealing with complex JSON data structures. Here, we’ll discuss some of the common issues you might encounter when using the jq command and provide some practical solutions and workarounds.
Syntax Errors
One of the most common issues when using the jq command is syntax errors. These can occur if you make a mistake in your jq filter expression. For instance, forgetting to close a bracket or misusing a jq function can lead to a syntax error.
echo '{"name":"John", "age":30}' | jq '.name
# Output:
# jq: error: syntax error, unexpected INVALID_CHARACTER, expecting $end (Unix shell quoting issues?) at <top-level>, line 1:
# .name
# jq: 1 compile error
In the example above, we forgot to close the single quote after the jq filter expression, leading to a syntax error. Always ensure your jq filter expressions are correctly formatted to avoid such errors.
Unexpected Output
Another common issue is getting unexpected output from your jq command. This can happen if your JSON data doesn’t have the structure you assumed, or if your jq filter expression doesn’t do what you thought it would.
echo '{"name":"John", "age":30}' | jq '.height'
# Output:
# null
In the example above, we’re trying to extract the ‘height’ property from our JSON data. However, our JSON data doesn’t have a ‘height’ property, so the jq command returns ‘null’. Always ensure you understand the structure of your JSON data and what your jq filter expression does to avoid such issues.
Tips for Using jq Command
Here are some tips to help you avoid common issues when using the jq command:
- Always double-check your jq filter expressions for syntax errors.
- Understand the structure of your JSON data before writing your jq filter expressions.
- Use the
-C
option to colorize your jq output. This can make it easier to understand the structure of your JSON data. - Use the
-r
option to output raw strings instead of JSON-encoded strings when necessary. - If you’re unsure about a jq filter expression, test it with simple JSON data first before using it with complex JSON data.
By following these tips, you can avoid many common issues when using the jq command and make your JSON parsing tasks easier and more efficient.
Understanding JSON: The Backbone of Data Exchange
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of JavaScript Programming Language, Standard ECMA-262 3rd Edition – December 1999.
JSON is a text format that is completely language-independent but uses conventions familiar to programmers of the C family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
Here’s a simple example of JSON data:
{
"name": "John",
"age": 30,
"city": "New York"
}
This JSON data represents an object with three properties: ‘name’, ‘age’, and ‘city’. The values of these properties are ‘John’, 30, and ‘New York’ respectively.
The Need for Tools like jq
While JSON is easy to read and write, it can be challenging to manipulate, especially from the command line. This is where tools like jq come in. The jq command in Linux allows you to parse and manipulate JSON data right from your command line, making it an invaluable tool for developers and system administrators.
Basics of the Linux Command Line
The Linux command line, also known as the terminal or shell, is a powerful tool that allows you to interact directly with your operating system. By typing commands into the terminal, you can perform tasks that would normally require a graphical user interface.
Here’s a simple example of a Linux command:
echo 'Hello, world!'
# Output:
# Hello, world!
In this example, we’re using the echo command to print the string ‘Hello, world!’ to the console. The Linux command line is filled with commands like echo, each with its own set of options and behaviors.
The jq command is one of these commands, and it’s specifically designed to handle JSON data. By understanding the basics of JSON and the Linux command line, you’ll be better equipped to use the jq command to its full potential.
Real-World Applications of jq Command
The jq command in Linux is not just a tool for parsing JSON data; it’s a versatile utility that can greatly simplify many real-world tasks.
jq in API Interaction
When interacting with APIs, you often need to deal with JSON data. Whether you’re sending a request to an API or receiving a response, the jq command can be incredibly useful. You can use it to extract specific data from a JSON response or format JSON data before sending a request.
curl 'https://api.github.com/users/octocat' | jq '.public_repos'
# Output:
# 8
In this example, we’re using the curl command to send a GET request to the GitHub API. The response is piped into the jq command, which extracts the number of public repositories.
jq in Data Analysis
The jq command can also be used in data analysis. With its filtering, mapping, and reducing capabilities, you can transform and analyze JSON data in various ways.
echo '[{"name":"John", "age":30}, {"name":"Doe", "age":20}]' | jq 'map(.age) | add / length'
# Output:
# 25
In this example, we’re using the jq command to calculate the average age of the objects in the JSON array.
jq in Automation
Automation scripts often need to handle JSON data. Whether you’re writing a script to automate API interaction or to process JSON logs, the jq command can make your job easier.
echo '{"name":"John", "age":30}' > data.json
jq '.age += 1' data.json > data.json.tmp && mv data.json.tmp data.json
# Output in data.json:
# {"name":"John", "age":31}
In this example, we’re using the jq command in a bash script to increment the ‘age’ property of a JSON object stored in a file.
Further Resources for Mastering jq Command
If you’re interested in learning more about the jq command and its capabilities, here are some resources that you might find useful:
- jq Manual: The official manual for the jq command. It provides a comprehensive overview of the command and its various options and usages.
- jq Tutorial by Tutorialspoint: A detailed tutorial that covers the basics and advanced usages of the jq command.
- Learning jq by Example: A practical guide that teaches you how to use the jq command by working with real-world examples.
By exploring these resources and practicing with different JSON data, you can become a master of the jq command and leverage its power in your everyday tasks.
Wrapping Up: jq Command in Linux
In this comprehensive guide, we’ve journeyed through the world of jq command in Linux, a versatile tool for parsing and manipulating JSON data from the command line.
We began with the basics, learning how to use jq for simple tasks like parsing JSON data. We then ventured into more advanced territory, exploring complex tasks like filtering, mapping, and reducing JSON data using jq. Along the way, we tackled common challenges you might face when using jq, such as syntax errors and unexpected output, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to parsing JSON data from the command line, comparing jq with other tools like Python’s json module and JavaScript’s JSON.parse method. Here’s a quick comparison of these methods:
Method | Flexibility | Performance | Ease of Use |
---|---|---|---|
jq | High | High | Moderate |
Python’s json module | Moderate | Moderate | High |
JavaScript’s JSON.parse | Moderate | Moderate | High |
Whether you’re just starting out with jq or you’re looking to level up your JSON parsing skills, we hope this guide has given you a deeper understanding of jq and its capabilities.
With its balance of flexibility, performance, and ease of use, jq is a powerful tool for handling JSON data in Linux. Happy coding!