jq Guide: How to Remove Quotes from JSON
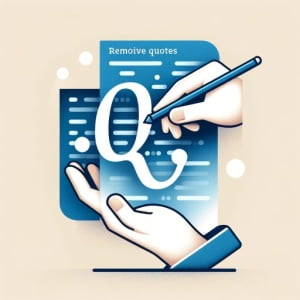
Are you finding it challenging to remove quotes from your JSON data using jq? You’re not alone. Many developers find themselves in a bind when it comes to handling quotes in JSON data, but we’re here to help.
Think of jq as a Swiss Army knife – a versatile tool that can help you manipulate JSON data with ease. Whether it’s trimming off unnecessary quotes or transforming data, jq has got you covered.
In this guide, we’ll walk you through the process of using jq to remove quotes from JSON data, from the basics to more advanced techniques. We’ll cover everything from simple usage of jq, handling complex JSON structures, to alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering jq!
TL;DR: How Do I Remove Quotes from JSON Data Using jq?
To remove quotes from JSON data using jq, you can use the
'-r'
or'--raw-output'
option, with the syntax,jq -r '.keyToOutput'
. This option instructs jq to output raw strings instead of JSON-encoded strings.
Here’s a simple example:
echo '{"name": "John Doe"}' | jq -r '.name'
# Output:
# John Doe
In this example, we’re using the echo command to create a simple JSON object with a single key-value pair. The key is ‘name’ and the value is ‘John Doe’. We then pipe this JSON object to jq, which uses the ‘-r’ option to output the value of the ‘name’ key without quotes.
This is just a basic way to use jq to remove quotes from JSON data, but there’s much more to learn about manipulating JSON data with jq. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Simplifying JSON with jq: The ‘-r’ Option
The ‘-r’ or ‘–raw-output’ option in jq is a powerful tool when it comes to removing quotes from JSON data. It’s straightforward to use and can simplify your JSON data manipulation tasks significantly.
Let’s look at a code example to understand how the ‘-r’ option works:
echo '{"city": "New York", "country": "USA"}' | jq -r '.city'
# Output:
# New York
In this example, we’re creating a JSON object with two key-value pairs: ‘city’ with the value ‘New York’ and ‘country’ with the value ‘USA’. We’re then using the ‘-r’ option in jq to output the value of the ‘city’ key without quotes.
The ‘-r’ option is telling jq to output raw strings, not JSON-encoded strings. This is why the output ‘New York’ doesn’t have quotes around it.
Using the ‘-r’ option is a great way to simplify your JSON data, but it’s not without its pitfalls. One thing to keep in mind is that if your JSON data contains special characters, the ‘-r’ option might not behave as expected. We’ll cover how to handle this scenario in the ‘Troubleshooting and Considerations’ section of this guide.
Tackling Complex JSON Structures with jq
While the ‘-r’ option is great for simple JSON data, what happens when we have more complex structures like nested objects or arrays? The good news is that jq can handle these scenarios as well.
Let’s explore a more complex JSON data structure:
echo '{"person": {"name": "John Doe", "address": {"city": "New York", "country": "USA"}}}' | jq -r '.person.address.city'
# Output:
# New York
In this example, we have a JSON object that contains another object (the ‘person’), which in turn contains another object (the ‘address’). We’re using jq to access the ‘city’ field in the ‘address’ object, and the ‘-r’ option is removing the quotes from the output.
But what about arrays? jq can handle those too:
echo '{"cities": ["New York", "Los Angeles", "Chicago"]}' | jq -r '.cities[]'
# Output:
# New York
# Los Angeles
# Chicago
In this example, we have a JSON object with a key ‘cities’ whose value is an array of city names. We’re using jq to access each element in the array, and again, the ‘-r’ option is removing the quotes from the output.
These examples illustrate how jq can handle more complex JSON data structures, making it a versatile tool for any JSON data manipulation task.
Exploring Alternative Methods for JSON Manipulation
While jq is a versatile tool for manipulating JSON data, it’s not the only one out there. There are other command-line tools and programming languages that can be used to remove quotes from JSON data. Let’s explore some of these alternatives.
Using Python’s json Module
Python is a powerful language that comes with a built-in module for handling JSON data. Here’s how you can use Python to remove quotes from JSON data:
import json
json_data = '{"name": "John Doe"}'
# Load the JSON data
data = json.loads(json_data)
# Access the 'name' key and print its value
print(data['name'])
# Output:
# John Doe
In this example, we’re using Python’s json module to load the JSON data. We then access the ‘name’ key and print its value, which is output without quotes.
Using the sed Command
The sed command is a stream editor for filtering and transforming text. It can also be used to remove quotes from JSON data:
echo '{"name": "John Doe"}' | sed -e 's/[{}"]//g' -e 's/: /
/g'
# Output:
# name: John Doe
In this example, we’re using the echo command to create a simple JSON object. We then pipe this object to sed, which uses a series of expressions to remove the quotes and brackets and replace the colon with a newline character.
Each of these methods has its advantages and disadvantages. jq is a versatile tool specifically designed for manipulating JSON data, making it a great choice for complex tasks. Python, on the other hand, is a powerful language that can handle a wide range of tasks, not just JSON manipulation. The sed command is a handy tool for simple text transformations, but it can be tricky to use for more complex tasks.
Ultimately, the best method depends on your specific needs and the complexity of your JSON data.
Overcoming Challenges with jq
As with any tool, you might encounter some challenges when using jq to remove quotes from JSON data. These challenges can range from syntax errors to handling special characters. Let’s discuss some common issues and their solutions.
Syntax Errors
One common issue is syntax errors. These can occur if you’re not familiar with jq’s syntax or if you make a typo. For example, forgetting to include the ‘.’ before the key name in the jq command will result in a syntax error:
echo '{"name": "John Doe"}' | jq -r 'name'
# Output:
# jq: error: syntax error, unexpected IDENT, expecting $end (Unix shell quoting issues?) at <top-level>, line 1:
# name
# jq: 1 compile error
The solution is to ensure you’re using the correct syntax. In this case, you should include the ‘.’ before the key name:
echo '{"name": "John Doe"}' | jq -r '.name'
# Output:
# John Doe
Handling Special Characters
Another common issue is handling special characters in JSON data. For example, if your JSON data contains a backslash (), you’ll need to escape it using another backslash:
echo '{"path": "C:\\Users\\John Doe"}' | jq -r '.path'
# Output:
# C:\Users\John Doe
In this example, we’re escaping each backslash in the JSON data with another backslash. This allows jq to interpret the backslashes correctly and remove the quotes from the output.
These are just a few examples of the challenges you might face when using jq to remove quotes from JSON data. However, with a good understanding of jq’s syntax and a bit of practice, you’ll be able to overcome these challenges and manipulate JSON data with ease.
Understanding JSON and jq Fundamentals
To effectively use jq for manipulating JSON data, it’s essential to understand the basics of both JSON and jq.
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
A basic JSON structure looks like this:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
In this example, we have a JSON object with three key-value pairs: ‘name’, ‘age’, and ‘city’. The keys are strings, and the values can be strings, numbers, objects, arrays, or boolean values.
What is jq?
jq is a lightweight and flexible command-line JSON processor. It’s like sed for JSON data – you can use it to slice, filter, map, and transform structured data.
Here’s a simple example of using jq to filter JSON data:
echo '{"name": "John Doe", "age": 30, "city": "New York"}' | jq '.name'
# Output:
# "John Doe"
In this example, we’re using jq to filter the ‘name’ key from the JSON data. The output is the value of the ‘name’ key, including quotes.
By understanding the fundamentals of JSON and jq, you can better understand the process of removing quotes from JSON data using jq.
Expanding Your JSON Data Processing Skills
Removing quotes from JSON data using jq is just the beginning. The ability to manipulate JSON data is a crucial skill in data processing, scripting, and many other areas of software development.
Exploring Related Concepts
Once you’ve mastered the basics of using jq to remove quotes from JSON data, you might want to explore related concepts like JSON parsing and data transformation. JSON parsing involves converting JSON data into a format that can be used and understood by your programming language. Data transformation, on the other hand, involves changing the format, structure, or values of data to meet specific requirements.
Here’s a simple example of using jq for data transformation:
echo '{"name": "John Doe", "age": 30, "city": "New York"}' | jq '{fullName: .name, age: .age}'
# Output:
# {
# "fullName": "John Doe",
# "age": 30
# }
In this example, we’re using jq to transform the JSON data. We’re changing the ‘name’ key to ‘fullName’ and keeping only the ‘fullName’ and ‘age’ keys in the output.
Further Resources for Mastering JSON Manipulation
If you’re interested in learning more about JSON manipulation and jq, here are some resources you might find helpful:
- jq Manual: The official manual for jq. It provides a comprehensive overview of jq’s features and how to use them.
Learning JSON with W3 Schools: A free online resource that offers tutorials on JSON, including its syntax, data types, and how to work with JSON in different programming languages.
JSON Processing with jq: An in-depth tutorial on processing JSON data with jq. It covers everything from installing jq to filtering, transforming, and querying JSON data.
Mastering jq and JSON manipulation can open up a world of possibilities for data processing, scripting, and more. So, keep learning and exploring!
Wrapping Up: Mastering the Art of Removing Quotes with jq
In this comprehensive guide, we’ve delved into the process of removing quotes from JSON data using jq. From understanding the basics of jq and JSON to exploring advanced usage and alternative approaches, we’ve covered a wide spectrum of knowledge to equip you with the skills needed to handle JSON data effectively.
We embarked on this journey with an introduction to jq and JSON, setting a solid foundation for all the learning that followed. We then dove into the basic use of jq, where we learned how to use the ‘-r’ option to remove quotes from simple JSON data. As we ventured further, we tackled more complex JSON structures like nested objects and arrays, demonstrating jq’s versatility.
In our quest for mastery, we also explored alternative approaches for handling JSON data, including Python’s json module and the sed command. Each method has its own strengths and weaknesses, and understanding these can help you choose the right tool for your specific needs.
Method | Pros | Cons |
---|---|---|
jq | Versatile, designed for JSON | May require troubleshooting for special characters |
Python’s json module | Powerful, part of a full-fledged programming language | Overkill for simple tasks |
sed command | Handy for simple text transformations | Tricky for complex JSON data |
Lastly, we discussed common issues you might encounter when using jq, such as syntax errors and handling special characters, and provided solutions for each. This troubleshooting guide will be a handy reference as you continue your journey with jq and JSON data manipulation.
Whether you’re a beginner just starting out with jq or an experienced developer looking for a refresher, we hope this guide has been a valuable resource. The ability to manipulate JSON data effectively is an essential skill in today’s data-driven world, and you’re now well-equipped to handle it. Happy coding!