npm Reference Guide | All Essential Developer Commands
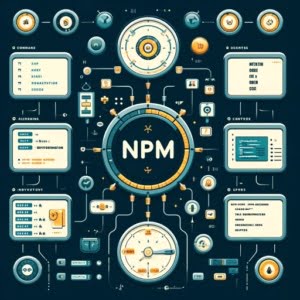
At IOFLOOD, utilizing npm commands correctly helps with efficient development and collaboration. We understand that the multitude of npm commands can be cumbersome, especially when starting Node.js development. To support other developers in enhancing their project workflows, we’ve gathered our information into this comprehensive guide on npm commands.
This guide is your beacon through the vast sea of NPM commands. It’s designed to illuminate the path from beginner to pro, ensuring you have the tools and knowledge to harness the full power of NPM. Whether you’re managing dependencies, executing scripts, or configuring your project settings, understanding NPM commands is crucial for any Node.js developer.
Let’s embark on this journey together, and explore the uses of NPM commands.
TL;DR: What Are the Essential NPM Commands?
Essential npm commands include
npm install
for adding packages,npm start
to run your application, andnpm test
for executing tests.
Here’s a quick example of using npm install
:
npm install express
# Output:
# + [email protected]
# added 50 packages in 1.265s
In this example, we use npm install
to add the Express package to our project. This command fetches the Express package from the npm registry and adds it to the project’s dependencies, making it ready for use. This is just the tip of the iceberg when it comes to npm commands, but it’s a crucial step for any Node.js project.
Ready to dive deeper? Keep reading for a comprehensive guide to mastering npm commands, from basic usage to advanced techniques.
Table of Contents
Kickstart Your Node.js Journey
Understanding Basic NPM Commands
NPM commands are the backbone of managing Node.js applications. They allow developers to efficiently manage packages, which are essentially bits of reusable code. At the beginner level, three primary commands you’ll frequently use are for installing, updating, and removing packages. Let’s dive into each of these commands with simple code examples to guide you through.
Installing Packages with NPM
To add a new package to your project, you use the npm install
command. Here’s how to install a specific version of a package, which is particularly useful when you need to ensure compatibility across different environments:
npm install [email protected]
# Output:
# + [email protected]
# added 1 package in 0.596s
In this example, we installed version 4.17.15 of the lodash package. Lodash is a popular utility library that provides a lot of helpful functions for working with arrays, numbers, objects, strings, etc. Ensuring you have the right version of a package can be crucial for project stability.
Updating Packages
Keeping your packages up-to-date is essential for security and performance. To update a package, use the npm update
command. This command checks for newer versions and updates the package accordingly:
npm update lodash
# Output:
# updated 1 package in 0.432s
This command updates lodash to the latest version available. Regularly updating your packages helps protect your project from vulnerabilities found in older versions.
Removing Packages
There might be instances where a package is no longer needed. To remove a package from your project, use the npm uninstall
command:
npm uninstall lodash
# Output:
# removed 1 package in 0.317s
Removing unneeded packages keeps your project clean and reduces its size, making it easier to maintain and faster to deploy.
Through these basic npm commands, you’re taking the first steps towards effective Node.js project management. These commands lay the foundation for more complex operations you’ll encounter as you delve deeper into the world of Node.js development.
A Deep Dive into NPM Commands
NPM, the default package manager for Node.js, offers a plethora of commands designed to streamline the development process. Whether you’re initializing a new project, managing dependencies, or publishing your own packages, understanding these commands can significantly improve your workflow. Below is a comprehensive table showcasing 20 essential NPM commands, their purposes, best usage practices, and examples.
Command | Description | Best Use | Example |
---|---|---|---|
npm init | Initializes a new Node.js project. | To create a new project with a package.json file. | npm init -y |
npm install | Installs a package. | To add a dependency to your project. | npm install express |
npm update | Updates all packages. | To keep your dependencies up-to-date. | npm update |
npm uninstall | Removes a package. | To clean up unused dependencies. | npm uninstall lodash |
npm list | Lists installed packages. | To view current project dependencies. | npm list --depth=0 |
npm audit | Scans for security vulnerabilities. | To improve project security. | npm audit |
npm run | Runs a script defined in package.json . | To execute custom scripts. | npm run start |
npm test | Runs tests using the script named “test”. | To execute test scripts. | npm test |
npm publish | Publishes a package to npm registry. | For sharing your package with the world. | npm publish |
npm cache clean | Clears the npm cache. | To solve issues related to package installation. | npm cache clean --force |
npm version | Bumps the version of your project. | To update project version before publishing. | npm version patch |
npm ci | Clean install based on package.json . | For consistent installs, ideal in CI/CD pipelines. | npm ci |
npm link | Symlinks a package for local development. | To work with local versions of a package. | npm link ../my-package |
npm search | Searches for packages in the npm registry. | To find new packages or specific versions. | npm search express |
npm logout | Logs out of the npm registry. | To switch between npm accounts. | npm logout |
npm whoami | Displays the npm username. | To check which account you’re logged into. | npm whoami |
npm config | Manages npm configuration settings. | To customize npm behavior. | npm config list |
npm help | Displays help on npm commands. | To get more information about specific commands. | npm help install |
After exploring the table above, it’s clear that npm commands cover a wide range of functionalities, from project initialization to security auditing. These commands are the tools that enable developers to manage their Node.js projects efficiently.
Spotlight on Key Commands
npm init: Starting Fresh
The npm init
command is your first step towards creating a new Node.js project. It prompts you to fill in details that will populate your package.json
file, a crucial component for managing project dependencies, scripts, and more. Here’s a simple example:
npm init -y
# Output:
# Wrote to /path/to/your/project/package.json:
# {...}
This command creates a package.json
file with default values, speeding up the project setup process.
npm install: Adding Dependencies
npm install
is perhaps the most used npm command. It allows you to add packages to your project, thus enabling the use of external libraries and frameworks. Here’s how you can add React to your project:
npm install react
# Output:
# + [email protected]
# added 1 package in 2.145s
This command adds React to your project’s dependencies, making it ready for use in your applications.
npm audit: Enhancing Security
Security is paramount, and npm audit
helps identify and fix vulnerabilities within your project’s dependencies. By scanning your project and providing insights into potential security issues, it plays a critical role in maintaining the integrity of your applications:
npm audit
# Output:
# found 0 vulnerabilities
This command reassures you that your project is free of known vulnerabilities, allowing you to focus on development with peace of mind.
Elevate Your NPM Skills
Advanced NPM Commands for Development
As you become more comfortable with the basics of npm, it’s time to delve into more advanced commands that can significantly enhance your workflow. Two such commands are npm link
and npm ci
, each serving a unique purpose in the development process. Let’s explore these commands with practical examples.
Local Package Development with npm link
When developing a package, you may want to test it within another local project before publishing. This is where npm link
becomes invaluable. It creates a symlink in the global folder for the package, which can then be linked to any project on your local machine.
# In your package directory
npm link
# In the project where you want to use the package
npm link <package-name>
# Output:
# /usr/local/lib/node_modules/<package-name> -> /path/to/your/package
This command sets up a symbolic link between your development package and the consuming project, allowing for real-time testing and development. It’s a powerful way to iterate on your package with immediate feedback from a real project environment.
Clean, Consistent Installs with npm ci
The npm ci
command is designed for automated environments such as continuous integration pipelines. It provides a faster, more reliable install by using the package-lock.json
or npm-shrinkwrap.json
file, rather than the package.json
to install dependencies, ensuring consistency across installations.
npm ci
# Output:
# added 204 packages in 4.506s
This command deletes your node_modules directory and reinstalls all dependencies, ensuring that you have a clean, up-to-date installation that matches your lock file exactly. It’s especially useful for avoiding discrepancies between development environments and production or between team members’ environments.
Why These Commands Matter
Both npm link
and npm ci
address specific challenges in Node.js development. npm link
facilitates the development and testing of local packages without the need to publish them to the npm registry. On the other hand, npm ci
ensures that your project dependencies are installed exactly as specified, without any unexpected updates or modifications. Mastering these commands can lead to a more efficient and reliable development process.
Beyond NPM: Yarn and PNPM
Exploring Yarn and PNPM
While npm commands are a staple in Node.js development, exploring alternative package managers like Yarn and PNPM can offer unique advantages. Both tools aim to address certain limitations of npm, providing developers with options tailored to different project needs. Let’s delve into these alternatives, highlighting their benefits and when to consider using them.
Yarn: Speed and Security
Yarn was introduced by Facebook to address performance and security concerns with npm at the time. One of its standout features is deterministic package installation, ensuring that the same dependencies are installed in the same way across all environments.
yarn add lodash
# Output:
# success Saved 1 new dependency.
# info Direct dependencies
# └─ [email protected]
# info All dependencies
# └─ [email protected]
In this example, we added the lodash package using Yarn. The command is similar to npm install
, but Yarn’s lock file mechanism ensures that the exact version installed is consistent across all team members’ environments, enhancing project stability.
PNPM: Efficiency and Disk Space
PNPM addresses another common concern with npm: disk space usage. It achieves this by hard linking packages from a single content-addressable storage to the node_modules
directories where they are needed, significantly reducing disk space usage.
pnpm add lodash
# Output:
# Packages: +1
# +
# Resolving: total 1, reused 1, downloaded 0, done
# [email protected] added to the project
This command adds lodash to your project using PNPM. Notice how the output highlights the efficiency of the operation, with minimal data needing to be downloaded or copied. This makes PNPM an excellent choice for developers working with limited disk space or those who value efficiency.
Decision-Making Considerations
When deciding between npm, Yarn, and PNPM, consider your project’s specific needs. Yarn is a strong choice for projects where consistent dependency resolution is critical, while PNPM shines in scenarios where disk space and efficiency are paramount. Regardless of your choice, understanding the nuances of each tool will empower you to make informed decisions, ensuring your development process is as effective as possible.
Tackling Common NPM Issues
Navigating Dependency Conflicts
Dependency conflicts can be a significant hurdle in Node.js projects, potentially leading to failed installations or runtime errors. Understanding how to resolve these conflicts is crucial for maintaining a healthy project. One effective strategy is using the npm ls
command to identify conflicting dependencies.
npm ls lodash
# Output:
# [email protected]
# └── [email protected]
# └── UNMET PEER DEPENDENCY lodash@^5.0.0
This output indicates that the project requires lodash version 4.17.15, but there’s an unmet peer dependency expecting lodash version ^5.0.0. To resolve this, you might need to update the package causing the conflict or find a version of lodash that satisfies all dependencies.
Speeding Up Installation Times
Slow npm installation times can be frustrating, especially when working on large projects or when internet connectivity is limited. Utilizing npm’s cache can significantly reduce installation times. The npm cache verify
command ensures your cache is in good condition, potentially speeding up future installations.
npm cache verify
# Output:
# Cache verified: 254 MB
# Content verified: 0
# Missing: 0
# Total files: 18923
This command checks the integrity of your npm cache, ensuring that it’s not corrupted and is ready to speed up your installations. It’s a simple yet effective way to optimize npm’s performance.
Best Practices for Package Management
Adopting best practices in package management is essential for efficient and trouble-free development. Here are a few tips:
- Regularly Audit Your Dependencies: Use
npm audit
to identify and resolve security vulnerabilities within your dependencies. - Keep Your
package-lock.json
Updated: This file locks down the versions of each package, ensuring consistent installations across environments. - Optimize Your
package.json
: Specify exact versions rather than ranges for dependencies to avoid unexpected updates.
By implementing these strategies, you can mitigate common npm issues, improve your project’s stability, and enhance your development experience.
Understanding NPM Fundamentals
The Heart of Node.js Development
NPM, standing for Node Package Manager, is the lifeline of the Node.js ecosystem, serving as a public repository for Node.js packages and a command-line utility for interacting with said repository. It facilitates the process of installing, updating, managing, and publishing packages. Understanding the role of npm is crucial for any Node.js developer aiming to leverage the full potential of npm commands.
To grasp the importance of npm, consider the npm init
command. Unlike the npm install
command mentioned earlier, npm init
lays the groundwork for a new Node.js project. Here’s an example:
npm init
# Output:
# This utility will walk you through creating a package.json file.
# It only covers the most common items, and tries to guess sensible defaults.
The npm init
command prompts you to answer a series of questions to create a package.json
file, which is pivotal for managing project dependencies. This file contains metadata about the project, such as its name, version, and dependencies, serving as a blueprint for npm on how to handle these packages.
Why NPM Commands Matter
NPM commands streamline the development process by automating tasks that would otherwise be tedious and time-consuming. For instance, managing dependencies manually in large projects can be overwhelming and prone to errors. NPM commands mitigate these issues, allowing developers to focus more on writing code and less on managing project dependencies.
Moreover, the npm ecosystem is vast, with hundreds of thousands of packages available for use. This abundance means that developers can easily find packages that suit their project’s needs, significantly speeding up the development process. However, with great power comes great responsibility. Developers must be diligent in selecting and updating packages to maintain the security and efficiency of their applications.
In summary, understanding the fundamentals of npm and its commands is not just about learning how to execute tasks; it’s about grasping the broader impact of these commands on the Node.js development workflow. This knowledge is essential for mastering npm and becoming an efficient Node.js developer.
Large-Scale Projects Uses: NPM
Streamlining Development with Scripts and Automation
As your Node.js projects grow in complexity and size, leveraging npm to automate and streamline your development process becomes increasingly important. NPM scripts can serve as a powerful tool for automating repetitive tasks such as testing, building, and deploying your applications. Let’s explore how you can use npm scripts to enhance your development workflow.
Consider the scenario where you want to automate the process of minifying JavaScript files. You can achieve this by adding a script in your package.json
file:
"scripts": {
"minify": "uglifyjs --compress --mangle -- output.min.js < input.js"
}
To run this script, you would execute the following command:
npm run minify
# Output:
# Successfully minified input.js to output.min.js
This command triggers the minification of JavaScript files, simplifying the process and saving valuable development time. NPM scripts are not limited to minification; they can be used for a wide range of tasks, providing a versatile solution to automate your development pipeline.
Further Resources for Mastering NPM Commands
To deepen your understanding and mastery of npm commands, consider exploring the following resources:
- NPM Documentation: The official npm documentation is an invaluable resource for developers, offering comprehensive guides on npm commands, packages, and best practices.
Node.js Guides: This collection of guides from the official Node.js website covers a wide range of topics, including npm and package management.
The Node.js Handbook: This handbook by freeCodeCamp offers a thorough exploration of Node.js, including detailed sections on npm and its commands.
By taking advantage of npm scripts and exploring these resources, you can significantly enhance your Node.js development experience. Whether you’re working on small projects or large-scale applications, understanding and utilizing npm commands is key to efficient and effective development.
Wrapping Up: NPM Command Guide
In this comprehensive guide, we’ve navigated through the essential landscape of NPM commands, a cornerstone for any Node.js developer. From the basic to the advanced, these commands not only streamline your development process but also empower you to manage your projects with greater efficiency and control.
We began with the fundamentals, understanding the pivotal role of NPM in the Node.js ecosystem and how simple commands like npm init
and npm install
lay the groundwork for project management. We then progressed to exploring a comprehensive list of commands, each tailored for specific tasks within your development workflow.
Our journey took us further into the realm of advanced usage, where we delved into commands like npm link
for local package development and npm ci
for clean, consistent installs. The exploration of alternative package managers like Yarn and PNPM provided insights into the broader ecosystem, offering tools for different project needs.
To aid in navigating these commands, here’s a quick reference table:
Command | Use Case |
---|---|
npm init | Initialize a new Node.js project |
npm install | Add a package to your project |
npm update | Update all packages |
npm uninstall | Remove a package |
npm link | Test local packages |
npm ci | Clean, consistent installs |
Whether you’re just starting out with NPM or looking to refine your command over its capabilities, we hope this guide has provided you with the knowledge to navigate the complexities of package management in Node.js.
The mastery of NPM commands is more than just memorizing syntax; it’s about understanding their impact on your development process and how they can be leveraged to enhance productivity. As you continue to develop your Node.js projects, remember that these commands are tools at your disposal, designed to facilitate a more efficient and streamlined development experience. Happy coding!