npm concurrently: Run Scripts, Commands Simultaneously
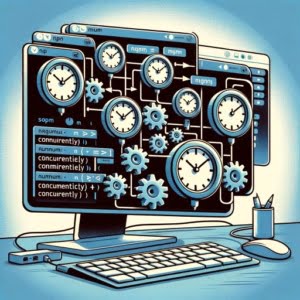
Are you finding it challenging to manage multiple npm scripts simultaneously? If so, you’re not alone. Many developers face this hurdle, but the ‘npm concurrently’ tool can help. Today’s article will walk you through its usage.
When developing software for IOFLOOD executing multiple NPM tasks is crucial for our processses, especially when working on large numbers of servers. We’ve explored the ‘npm concurrently’ package as a solution to help speed up the devlopment workflow, and have gathered our findings into this comprehensive article. We hope that this info can assist our bare metal server customers and other developers facing similar hurdles.
This guide will walk you through the npm ‘concurrently’ package, from basic setup to advanced usage, making your development workflow smoother and more efficient. By the end of this guide, you’ll be equipped with the knowledge to implement ‘npm concurrently’ in your projects, enhancing productivity and optimizing task management.
Let’s dive into the world of ‘npm concurrently’ and discover how it can allowg you to run multiple NPM commands with ease.
TL;DR: How Do I Run Multiple NPM Commands Simultaneously?
You can run multiple npm commands simultaneously with the
npm concurrently
package. To use it, install withnpm install concurrently -g
ornpm install concurrently --save-dev
. You can then define the scripts you want to run concurrently within yourpackage.json
file.
Here’s a quick start example:
npm install concurrently --save-dev
npm run concurrently "npm:watch-*"
# Output:
# [All scripts starting with 'watch-' run simultaneously]
In this example, we first install ‘npm concurrently’ as a development dependency. Then, by running npm run concurrently "npm:watch-*"
, we execute all scripts that start with ‘watch-‘ in parallel. This is particularly useful for tasks like watching files for changes while running a server, compiling code, or running tests concurrently.
For a deeper dive into ‘npm concurrently’, including more detailed instructions, advanced tips, and troubleshooting advice, keep reading. This guide will equip you with everything you need to know to master concurrent tasks in Node.js using npm concurrently.
Table of Contents
Getting Started with NPM Concurrently
Your First Concurrent Scripts
Embarking on the journey of mastering concurrent tasks in Node.js begins with a simple yet powerful step: installing ‘npm concurrently’. This package is your gateway to running multiple npm scripts at once, a common necessity in modern development workflows. Let’s dive into the basic setup and your first concurrent scripts execution.
First, ensure you have ‘npm concurrently’ installed in your project. If not, here’s how you can add it as a development dependency:
npm install concurrently --save-dev
With ‘npm concurrently’ now part of your project, let’s proceed to run two scripts concurrently. Imagine you have two scripts in your package.json
: script1
and script2
. Here’s how you can execute them at the same time:
npx concurrently "npm run script1" "npm run script2"
# Output:
# [Both script1 and script2 running concurrently, their outputs intermingled]
In this code block, npx concurrently
is used to run script1
and script2
concurrently. The beauty of ‘npm concurrently’ is in its simplicity and power. By running scripts in parallel, you not only save time but also streamline your development process. This approach is particularly beneficial when you need to watch files for changes and serve your application simultaneously, among other use cases.
The syntax of ‘npm concurrently’ is straightforward. Each script you wish to run concurrently is enclosed in quotes and separated by a space. This method allows for easy addition of more scripts as your project grows. The expected output shows both scripts running at the same time, demonstrating the package’s ability to manage multiple processes efficiently.
By incorporating ‘npm concurrently’ into your development workflow, you embrace a more efficient and productive approach to managing tasks. This beginner-level guide sets the foundation for exploring more advanced features and optimizations, paving the way for a smoother development experience.
Advanced npm concurrently
Tips
Custom Prefixes for Clarity
As you delve deeper into using ‘npm concurrently’, you’ll discover features that not only enhance efficiency but also improve the readability of your output. One such feature is the ability to add custom prefixes to your script outputs. This is invaluable for quickly identifying which output belongs to which script, especially when running several tasks simultaneously.
Imagine you have three scripts: build
, test
, and lint
. Here’s how you can run them with custom prefixes for easier log identification:
npx concurrently --names "BUILD,TEST,LINT" "npm run build" "npm run test" "npm run lint"
# Output:
# [BUILD] Output from build script
# [TEST] Output from test script
# [LINT] Output from lint script
In this example, --names
is used to assign custom prefixes (BUILD, TEST, LINT) to each script’s output. This makes it significantly easier to follow the logs and understand which process they belong to at a glance. The importance of this feature grows with the complexity of your project, making your development process more manageable.
Utilizing Wildcards for Efficiency
Another powerful feature of ‘npm concurrently’ is the use of wildcards to run multiple scripts that match a pattern. This is particularly useful for projects with numerous scripts that you might want to run in groups.
For instance, if you have several watch tasks named watch-js
, watch-css
, and watch-html
, you can run them all concurrently with a single command:
npx concurrently "npm run watch-*"
# Output:
# [watch-js] Watching .js files...
# [watch-css] Watching .css files...
# [watch-html] Watching .html files...
By using the wildcard pattern watch-*
, ‘npm concurrently’ intelligently runs all matching scripts. This not only saves time but also simplifies your command structure, making your workflow more efficient.
These intermediate-level techniques demonstrate the versatility and power of ‘npm concurrently’. By mastering these features, you can significantly optimize your development workflow, making it smoother and more productive. The ability to easily identify script outputs and efficiently run groups of tasks empowers developers to manage complex projects with ease.
Alternative Concurrency Methods
Embracing Node.js Child Processes
While ‘npm concurrently’ is a powerful tool for managing concurrent tasks, the Node.js ecosystem offers alternative methods that can achieve similar results. One such approach involves leveraging Node.js child processes in combination with Promise.all
to run multiple tasks simultaneously. This method provides a more programmatic way to handle concurrency.
Consider this example where we run two scripts, script1.js
and script2.js
, using Node.js child processes:
const { exec } = require('child_process');
Promise.all([
new Promise((resolve) => exec('node script1.js', (err, stdout) => resolve(stdout))),
new Promise((resolve) => exec('node script2.js', (err, stdout) => resolve(stdout)))
]).then((results) => {
console.log('script1 output:', results[0]);
console.log('script2 output:', results[1]);
});
# Output:
# script1 output: [Output from script1.js]
# script2 output: [Output from script2.js]
In the code block above, exec
from Node.js’s child_process
module is used to execute two scripts. Promise.all
is then employed to run these commands concurrently, waiting for both to complete before logging their outputs. This approach not only demonstrates the flexibility of Node.js for concurrent operations but also highlights the power of promises for managing asynchronous tasks.
Utilizing Shell Operators for Simplicity
Another alternative to ‘npm concurrently’ involves using native shell operators. For Unix-like systems, the ampersand (&
) operator allows you to run commands in the background, achieving concurrency with minimal effort.
Here’s a simple example:
node script1.js & node script2.js
# Output:
# [Both scripts run in the background concurrently]
Using the &
operator, both script1.js
and script2.js
are executed in the background simultaneously. This method is straightforward and requires no additional packages, making it an attractive option for simpler tasks.
Comparing Alternatives
Both the Node.js child processes with Promise.all
and shell operators offer viable alternatives to ‘npm concurrently’, each with its own set of benefits and drawbacks. The Node.js approach provides more control and is platform-independent, making it suitable for complex applications. On the other hand, shell operators offer a quick and easy solution for simpler use cases, though they are limited by the operating system.
In conclusion, while ‘npm concurrently’ remains a robust tool for managing concurrent tasks, exploring these alternative approaches can enrich your development toolkit, offering flexibility to choose the right solution for your project’s needs.
Troubleshooting NPM Concurrently
Handling Script Exit Codes
One of the nuances of using ‘npm concurrently’ involves managing the exit codes of concurrently run scripts. When one script fails (exits with a non-zero status), it’s crucial to ensure your workflow can handle this scenario gracefully.
Consider a scenario where you’re running two scripts, and one fails:
npx concurrently "exit 1" "echo 'Script 2 Running'"
# Output:
# [error] Command "exit 1" exited with 1.
# Script 2 Running
In the example above, the first command intentionally fails with exit 1
. By default, ‘npm concurrently’ will continue running the other scripts. This behavior might be desirable or not, depending on your workflow. To stop all scripts when one fails, you can use the --kill-others
flag. Understanding and controlling these behaviors is key to managing complex workflows.
Managing Output Clutter
When running multiple scripts concurrently, the output can become cluttered, making it hard to follow. ‘npm concurrently’ offers the --prefix
option to label each script’s output, as discussed in the advanced usage section. Additionally, consider structuring your scripts to minimize unnecessary output.
Ensuring Cross-Platform Compatibility
Another consideration is ensuring that your use of ‘npm concurrently’ and associated scripts are cross-platform compatible, especially when using shell-specific commands. For example, using UNIX-specific commands without alternatives or wrappers can cause scripts to fail on Windows.
To address this, you can use cross-platform packages like shx
for UNIX-like commands on non-UNIX systems or ensure your scripts are written in a way that is compatible across the board.
npx concurrently "shx echo 'Cross-platform echo'"
# Output:
# Cross-platform echo
In the snippet above, shx
is used to make the echo
command cross-platform compatible. This approach ensures that the script runs smoothly regardless of the operating system.
Conclusion
Mastering ‘npm concurrently’ involves not just understanding how to run tasks concurrently but also managing the nuances and potential pitfalls effectively. By addressing common issues such as script exit codes, output clutter, and cross-platform compatibility, you can leverage ‘npm concurrently’ to its fullest, making your development process more efficient and robust.
Role of Concurrency in Development
The Heart of Node.js: Asynchronous Operations
Before diving into the practical use of ‘npm concurrently’, it’s crucial to grasp the concept of concurrency in software development. Concurrency involves executing multiple sequences of operations in overlapping periods, allowing for more efficient use of resources and faster completion of tasks.
Node.js, at its core, is designed around asynchronous event-driven architecture. This means that operations in Node.js don’t block the main thread; instead, they run in parallel where possible, making efficient use of system resources. Here’s a basic example of asynchronous code in Node.js:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
# Output:
# Content of example.txt
In this snippet, fs.readFile
is used to read the content of a file asynchronously. The callback function is executed once the file reading is completed, printing the content to the console. This non-blocking nature allows Node.js applications to handle multiple operations concurrently, significantly improving performance.
The Role of NPM Scripts
NPM scripts play a pivotal role in Node.js projects, automating tasks like building, testing, and deployment. These scripts are defined in the package.json
file of a project, providing a convenient way to run custom commands.
For example, a simple NPM script to run a test could look like this:
"scripts": {
"test": "echo 'Running tests...' && exit 0"
}
Running npm run test
would execute the command defined in the script, displaying ‘Running tests…’ in the console. While this example is straightforward, real-world projects often require running multiple scripts concurrently — for tasks such as watching file changes while serving the application.
This is where ‘npm concurrently’ comes into play, allowing multiple NPM scripts to be executed at the same time, harnessing the power of Node.js’s asynchronous nature and optimizing the development workflow.
In conclusion, understanding the fundamentals of concurrency and the asynchronous capabilities of Node.js, along with the role of NPM scripts, lays the groundwork for effectively using ‘npm concurrently’. This knowledge not only aids in grasping the utility of ‘npm concurrently’ but also in appreciating the broader context of its application in modern web development.
Optimizing NPM Concurrently
Integrating with Build Tools
As your projects grow in complexity, integrating ‘npm concurrently’ with build tools can significantly enhance your development workflow. Tools like Webpack or Gulp often require running multiple tasks simultaneously—such as watching files, starting a development server, and compiling assets. ‘npm concurrently’ seamlessly fits into this process, allowing you to automate and simplify these tasks.
For instance, integrating ‘npm concurrently’ with Webpack might look like this:
"scripts": {
"dev": "concurrently \"webpack --watch\" \"npm run serve\""
}
# Output:
# Webpack is watching the files…
# Project is served at http://localhost:3000
In the script above, webpack --watch
and npm run serve
are run concurrently, ensuring that your assets are recompiled on-the-fly while the development server is refreshed automatically. This setup not only saves time but also eliminates the need for manually restarting tasks.
Automating Development Tasks
Automation is key to efficient development practices, especially in larger projects. ‘npm concurrently’ can be part of a larger automation setup, running alongside other scripts to perform tasks like linting, testing, and deployment. This holistic approach to automation ensures consistency across development environments and can significantly reduce errors.
npx concurrently "npm run lint" "npm test" "npm run deploy"
# Output:
# Linting code...
# Running tests...
# Deploying application...
This command demonstrates how ‘npm concurrently’ can be used to run linting, testing, and deployment scripts in parallel, streamlining the development process and making it more efficient.
Further Resources for Mastering NPM Concurrently
To deepen your understanding of ‘npm concurrently’ and explore more advanced use cases, consider the following resources:
- The npm concurrently GitHub page offers comprehensive documentation, including all options and configurations.
- Node.js official documentation provides insights into Node.js’s asynchronous nature and how it complements tools like ‘npm concurrently’.
- JavaScript Info offers tutorials on advanced JavaScript concepts, including concurrency and event loops, which are fundamental for using ‘npm concurrently’ effectively.
These resources will equip you with the knowledge to leverage ‘npm concurrently’ in your projects, encouraging you to explore broader development practices and tools that complement its functionality.
Recap: Mastering NPM Concurrently
In this comprehensive guide, we’ve explored the power and flexibility of ‘npm concurrently’ for managing concurrent tasks in Node.js projects. From the basic setup to advanced usage, this tool streamlines your development workflow, making it more efficient and manageable.
We began with the essentials, demonstrating how to install ‘npm concurrently’ and execute your first concurrent scripts. This foundational knowledge sets the stage for more complex operations, allowing you to run multiple npm scripts simultaneously with ease. Next, we delved into advanced features, such as custom prefixes and wildcards, which further optimize your workflow and clarify your output.
Exploring alternative approaches, we compared ‘npm concurrently’ with native Node.js child processes and shell operators, providing you with a broader perspective on concurrency management. This comparison not only highlighted the versatility of ‘npm concurrently’ but also showcased the rich ecosystem of tools available in Node.js for achieving similar outcomes.
Approach | Flexibility | Ease of Use | Cross-Platform Compatibility |
---|---|---|---|
NPM Concurrently | High | High | High |
Node.js Child Processes | High | Moderate | High |
Shell Operators | Low | High | Low |
Whether you’re just starting out with ‘npm concurrently’ or looking to refine your concurrent task management skills, we hope this guide has provided you with valuable insights and practical knowledge. ‘Npm concurrently’ not only simplifies the execution of multiple tasks but also enhances the overall development experience by reducing complexity and saving time.
With its balance of power, flexibility, and ease of use, ‘npm concurrently’ stands as a vital tool in the modern developer’s toolkit. We encourage you to experiment with ‘npm concurrently’ in your projects and explore further resources to continue mastering concurrent tasks in Node.js. Happy coding!