NPM Dotenv Guide | Setup Node.js Environment Variables
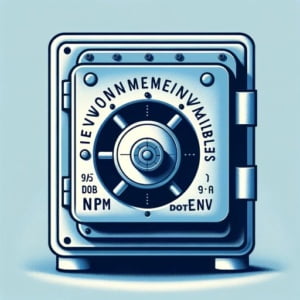
Managing environment variables in Node.js projects, especially when working on software at IOFLOOD, can be complex and error-prone. In our experience, using the dotenv npm package has proven to be a reliable solution for keeping sensitive info secure and managing configurations. Seeing as this can be useful to our dedicated server customers utilizing Node.js, we’ve gathered our tips and tricks into this comprehensive article.
This guide will walk you through the basics to advanced usage of dotenv, making your development process smoother and more secure. By the end of this journey, you’ll have a solid understanding of how to implement npm dotenv in your Node.js projects, enhancing both security and efficiency.
Let’s unlock the potential of environment variable management together!
TL;DR: How Do I Use npm dotenv for Managing Environment Variables in Node.js?
To manage environment variables in Node.js using npm dotenv, first install dotenv via npm, then create a
.env
file in your project root, and finally load the variables usingrequire('dotenv').config();
.
Here’s a quick example:
require('dotenv').config();
console.log(process.env.YOUR_VARIABLE);
# Output:
# Your variable value
In this example, we demonstrate how to load environment variables from a .env
file into your Node.js application. By using require('dotenv').config();
, the dotenv package reads the .env
file, parses the variables, and adds them to process.env
, making them accessible throughout your application.
Keen to dive deeper? Continue reading for more detailed instructions, advanced tips, and troubleshooting advice.
Table of Contents
Getting Started with npm dotenv
Installation and Setup
Embarking on the journey of managing environment variables in your Node.js project begins with a simple step: installing the dotenv package. This process is straightforward and requires only a single command. Open your terminal and run:
npm install dotenv
This command fetches and installs the dotenv package from npm, adding it to your project’s dependencies. Once installed, you’re ready to take the next step: creating your .env
file.
Creating Your .env
File
The .env
file serves as the vault for your environment variables, safely storing sensitive information outside your source code. Here’s how to create one:
- In your project root, create a new file named
.env
. - Add environment variables in the format
VARIABLE_NAME=value
.
For example:
API_KEY=12345abcde
DATABASE_PASSWORD=securepassword
These variables are now ready to be accessed by your Node.js application.
Accessing Environment Variables
With your .env
file in place, accessing the stored variables in your application is simple. Here’s a basic example of how to load and use an environment variable:
require('dotenv').config();
console.log(`Your API Key is: ${process.env.API_KEY}`);
# Output:
# Your API Key is: 12345abcde
In this example, require('dotenv').config();
loads the environment variables from the .env
file into process.env
, making them accessible throughout your application. By doing so, you can easily manage sensitive information without hardcoding it into your source code, enhancing both security and flexibility.
Understanding and implementing these basic steps are crucial for securely managing environment variables in your Node.js projects. By following this guide, you’ve taken a significant step towards more secure and efficient project configuration.
Elevating Your npm dotenv Skills
Customizing the .env
File Path
As your Node.js project grows, you might find yourself needing to organize your environment variables in different .env
files. This could be for various environments like development, testing, or production. npm dotenv allows you to customize the path to your .env
file, making it flexible to fit your project’s structure.
To specify a custom path for your .env
file, you can modify the config
method like so:
require('dotenv').config({ path: './config/custom.env' });
This tells dotenv to load the environment variables from ./config/custom.env
instead of the default .env
file in the project root. It’s a simple yet powerful feature that enhances your project’s configuration management.
Preloading dotenv
Another advanced feature of dotenv is preloading, which allows you to load your environment variables before your application starts. This is particularly useful when you can’t use require('dotenv').config()
at the top of your main file.
Preloading dotenv is achieved by adding it as a prerequisite in your start script within your package.json
file:
"scripts": {
"start": "node -r dotenv/config your_script.js"
}
In this configuration, -r dotenv/config
preloads the dotenv before your_script.js
runs. It’s an elegant solution for applications requiring environment variables to be loaded even before the application logic begins.
Dotenv for Different Environments
Managing environment variables for different environments (e.g., development, production) is crucial for any serious project. npm dotenv facilitates this by allowing you to have separate .env
files for each environment.
A common approach is to have .env.development
, .env.test
, and .env.production
files. You can then load the appropriate file based on your current NODE_ENV setting:
const envFile = `.env.${process.env.NODE_ENV}`;
require('dotenv').config({ path: envFile });
This snippet dynamically selects the correct .env
file based on the NODE_ENV
environment variable. It’s a straightforward yet effective method to ensure your application uses the right set of environment variables for its current environment.
By mastering these advanced configurations, you significantly improve your application’s flexibility and security. Customizing the path, preloading dotenv, and using separate .env
files for different environments are key techniques in your Node.js toolbox, enhancing your project’s configuration and management.
Exploring Alternatives to npm dotenv
Node.js Built-in Support for Environment Variables
Node.js itself offers built-in support for environment variables, which can be accessed via process.env
. This method is straightforward and doesn’t require any additional packages. Here’s how you might access an environment variable directly in Node.js:
console.log(`Database URL: ${process.env.DATABASE_URL}`);
# Output:
# Database URL: your_database_url_here
This code snippet demonstrates accessing the DATABASE_URL
environment variable directly through process.env
. It’s a simple and direct approach, but lacks the organization and security features provided by dotenv, such as storing variables in a separate file and parsing them.
Other npm Packages for Environment Management
Besides npm dotenv, there are other packages available for managing environment variables in Node.js projects. Packages like env-cmd
and cross-env
offer different features and syntax for working with environment variables.
For example, env-cmd
allows you to specify different environment files for different scenarios, similar to dotenv, but with a focus on script commands. Here’s how you might use it in your package.json
:
"scripts": {
"start:dev": "env-cmd -f .env.development node app.js",
"start:prod": "env-cmd -f .env.production node app.js"
}
This configuration demonstrates using env-cmd
to load different .env
files for development and production environments. It’s a flexible approach, allowing for clear separation between environments at the script level.
Choosing the Right Tool
When comparing npm dotenv with built-in support and other packages, the choice depends on your project’s specific needs. npm dotenv is widely adopted for its simplicity and effectiveness in managing environment variables through .env
files. However, for projects that require environment variables to be set directly in scripts or that need different environment variables for different run scripts, alternatives like env-cmd
or cross-env
might be more suitable.
Understanding the strengths and limitations of each approach is key to effectively managing your Node.js project’s environment variables. Whether you choose npm dotenv for its ease of use and security features or opt for an alternative solution, the goal is to manage your environment variables in a way that best suits your project’s requirements.
Dotenv Troubleshooting Guide
Avoiding .env
in Version Control
One of the most common pitfalls with using npm dotenv is inadvertently committing the .env
file to version control. This file often contains sensitive information that should not be shared publicly. To prevent this, you can add .env
to your .gitignore
file:
# .gitignore
.env
By doing so, you ensure that your .env
file is not tracked by Git, protecting your sensitive information from being exposed.
Reloading Environment Variables
Another issue developers might face is not reloading environment variables when they change. For changes in your .env
file to take effect, you must restart your Node.js application. This ensures that the latest environment variables are loaded and used.
Handling Undefined Variables
Encountering undefined
when accessing environment variables is a common issue. This usually means the variable has not been defined in your .env
file or the application failed to load the .env
file correctly. To debug, first ensure your variable is correctly defined:
# .env example
API_SECRET=supersecret
Then, verify your application is loading the .env
file correctly. A simple console log can help identify if the variables are being loaded:
require('dotenv').config();
console.log(process.env.API_SECRET);
# Output:
# supersecret
If you see the expected output, your environment variables are being loaded correctly. If not, ensure dotenv
is installed and your .env
file is located at the root of your project or specify the path as shown in the advanced usage section.
Best Practices for Secure Dotenv Usage
- Regularly review your
.env
file to ensure it only contains necessary variables. - Use a separate
.env
file for different environments (e.g.,.env.development
,.env.production
) to prevent configuration mix-ups. - Never hardcode sensitive information in your application code, even for testing purposes.
By following these troubleshooting tips and best practices, you can avoid common pitfalls and ensure your use of npm dotenv enhances your Node.js project’s security and efficiency.
Environment Variables: The Essentials
Why Environment Variables Matter
Environment variables are a cornerstone of modern application development, acting as external data sources that influence an application’s behavior without requiring changes to the code. They are particularly crucial for managing sensitive information—such as API keys, database passwords, and configuration settings—that should not be hard-coded into application source code for security and flexibility reasons.
The Role of Dotenv
npm dotenv
plays a pivotal role in the management of these environment variables in Node.js applications. It allows developers to store environment variables in a .env
file, which is then easily loaded into the application. To illustrate, consider a scenario where we want to load a database password into our application without hardcoding it:
require('dotenv').config();
console.log(`Database password is: ${process.env.DB_PASSWORD}`);
# Output:
# Database password is: examplePassword123
In this code block, require('dotenv').config();
loads the environment variables from the .env
file. The console log then prints the database password, demonstrating how dotenv makes it accessible through process.env.DB_PASSWORD
. This approach keeps sensitive data out of the source code, enhancing security.
Dotenv Philosophy and Security
The philosophy behind npm dotenv
is straightforward yet powerful: provide a simple way to load environment variables from a .env
file into process.env
, making them accessible throughout the application. This method supports the 12-factor app methodology, which advocates for strict separation of configuration from code. By doing so, dotenv contributes to more secure, scalable, and maintainable applications.
Security best practices with dotenv include never committing the .env
file to version control, using different .env
files for different environments (development, testing, production), and regularly reviewing the .env
file to ensure it contains only necessary variables. These practices help prevent sensitive information from being exposed and ensure that your application remains secure and efficient.
Understanding the fundamentals of environment variables and the role of npm dotenv
provides a solid foundation for secure and effective application development. By leveraging dotenv, developers can manage sensitive information more securely and maintain cleaner, more flexible codebases.
Integrating Dotenv in Larger Projects
Dotenv in Deployment and CI/CD Pipelines
As your Node.js application transitions from development to production, integrating npm dotenv
into your deployment and CI/CD pipelines becomes crucial. This ensures that your environment variables are correctly set across different stages of your application lifecycle.
Consider a scenario where you’re deploying your application using a CI/CD tool like Jenkins or GitHub Actions. You can configure these tools to set environment variables directly from a .env.production
file. This approach keeps your production secrets secure while ensuring they are correctly applied during the deployment process.
# Example of setting environment variables in a CI/CD pipeline
export $(cat .env.production | xargs)
# Output:
# Environment variables are set for the deployment process
In this example, export $(cat .env.production | xargs)
reads the .env.production
file and exports each variable so they are available during the deployment process. This method streamlines setting environment variables in CI/CD pipelines, ensuring a smooth transition from development to production.
Dotenv’s Role in Project Configuration
npm dotenv
is not just about managing environment variables; it’s a tool that supports advanced project configuration strategies. By using dotenv, you can tailor your application’s behavior based on the current environment, making it adaptable and resilient.
For instance, you might have different database configurations for development, testing, and production environments. Dotenv allows you to switch seamlessly between these configurations by loading the appropriate .env
file for each environment.
Further Resources for Dotenv Mastery
To deepen your understanding of npm dotenv
and explore more advanced topics, here are three valuable resources:
- The Official npm dotenv Repository: The source of truth for dotenv, offering comprehensive documentation and usage examples.
Twelve-Factor App Methodology: A deeper dive into the principles behind environment variable management and application configuration, as advocated by dotenv.
Node.js Deployment Best Practices: Insights into deploying Node.js applications, including using Docker and other modern deployment strategies.
These resources will guide you through advanced concepts and best practices, enhancing your ability to manage environment variables effectively and securely in your Node.js projects.
Recap: Install and Use npm Dotenv
In this comprehensive guide, we’ve navigated through the essentials of using npm dotenv to manage environment variables in Node.js projects. From securely storing sensitive information to enhancing the flexibility of your project’s configuration, npm dotenv has proven to be an invaluable tool.
We began with the basics, demonstrating how to install dotenv and create a .env
file to store your environment variables. We then explored how to access these variables in your Node.js application, ensuring your project’s configuration remains both secure and easily manageable.
Moving on, we delved into advanced usage, such as customizing the path to your .env
file and preloading dotenv for different Node.js environments. These intermediate techniques offer greater control and adaptability, catering to the evolving needs of your project.
We also discussed alternative approaches for managing environment variables, comparing npm dotenv with built-in Node.js support and other npm packages. This comparison highlighted the flexibility and security benefits of dotenv, while acknowledging scenarios where alternatives might be more appropriate.
Approach | Flexibility | Security | Ease of Use |
---|---|---|---|
npm dotenv | High | High | High |
Node.js Built-in | Moderate | Moderate | High |
Other npm Packages | High | Varies | Moderate |
As we conclude, it’s clear that npm dotenv plays a critical role in the secure and efficient management of environment variables in Node.js projects. Whether you’re a beginner just starting out or an experienced developer looking for advanced configuration options, npm dotenv offers the tools you need to manage your project’s environment variables effectively.
With its balance of flexibility, security, and ease of use, npm dotenv is an essential component of modern Node.js development. As you continue to develop and deploy Node.js applications, keep these practices and considerations in mind to ensure your project’s configuration remains both secure and scalable. Happy coding!