Python Decorator | Usage Guide (With Examples)
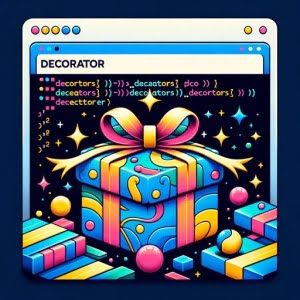
Are you finding it challenging to simplify your Python code and make it more readable? You’re not alone. Many developers grapple with this task, but Python decorators, like a magic wand, can help you achieve this.
Python decorators are powerful tools that can help streamline your code, making it more efficient and easier to read. They can be a bit tricky to understand at first, but once you get the hang of them, they can significantly enhance your coding process.
This guide will walk you through the ins and outs of Python decorators, from basic usage to advanced techniques. Whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you.
So, let’s get started and master Python decorators!
TL;DR: What is a Python Decorator?
A Python decorator is a function that modifies the behavior of another function. It allows you to wrap a function or method in order to extend or change its behavior without permanently modifying it.
Here’s a simple example:
def my_decorator(func):
def wrapper():
print('Before function execution')
func()
print('After function execution')
return wrapper
@my_decorator
def say_hello():
print('Hello!')
say_hello()
# Output:
# Before function execution
# Hello!
# After function execution
In this example, we’ve created a decorator named my_decorator
. This decorator wraps the say_hello
function and modifies its behavior by printing ‘Before function execution’ and ‘After function execution’ before and after the function execution, respectively.
This is a basic way to use Python decorators, but there’s much more to learn about their creation and manipulation. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Creating and Using a Basic Python Decorator
- Advanced Python Decorator Techniques
- Built-In Python Decorators:
- Troubleshooting Python Decorators: Common Issues and Solutions
- Python Decorators: Understanding the Fundamentals
- Python Decorators in Web Development and Beyond
- Wrapping Up: Mastering Python Decorators
Creating and Using a Basic Python Decorator
Python decorators are a powerful tool that can make your code more readable and maintainable. They allow you to wrap a function, extending or modifying its behavior. This allows you to apply boilerplate code to functions while adhering to the “DRY” principle (don’t repeat yourself).
Let’s start with a simple example:
def my_decorator(func):
def wrapper():
print('Before function execution')
func()
print('After function execution')
return wrapper
@my_decorator
def say_hello():
print('Hello!')
say_hello()
# Output:
# Before function execution
# Hello!
# After function execution
In this example, my_decorator
is a Python decorator. It takes a function func
as an argument and defines a new function wrapper
that modifies the behavior of func
. The wrapper
function is then returned and replaces the original func
when say_hello
is called.
The @my_decorator
syntax is just an easier way of saying say_hello = my_decorator(say_hello)
. It’s a Pythonic way to apply a decorator to a function.
Benefits of Using Python Decorators
Python decorators can greatly enhance your code by providing a simple syntax for calling higher-order functions. They can help reduce code duplication and make your code more expressive and easier to read.
Pitfalls to Watch Out For
While decorators can be powerful, they can also be misused. Overusing decorators can make your code harder to understand and debug, as the logic can become obscured. It’s important to use them judiciously and always consider whether a decorator is the best solution for your needs.
Advanced Python Decorator Techniques
As you become more comfortable using Python decorators, you can start to explore more advanced techniques. These include using decorators with parameters, class methods, and even applying multiple decorators to a single function.
Decorators with Parameters
Sometimes, you might want to pass additional parameters to your decorator. Here’s how you can do that:
def decorator_with_args(decorator_arg1, decorator_arg2):
def my_decorator(func):
def wrapper(*args, **kwargs):
print(f'Before function execution, Arg1: {decorator_arg1}, Arg2: {decorator_arg2}')
func(*args, **kwargs)
print('After function execution')
return wrapper
return my_decorator
@decorator_with_args('Hello', 'World')
def say_hello(name):
print(f'Hello, {name}!')
say_hello('Alice')
# Output:
# Before function execution, Arg1: Hello, Arg2: World
# Hello, Alice!
# After function execution
In this example, the decorator decorator_with_args
accepts two arguments. It returns the my_decorator
function, which in turn takes a function func
and returns the wrapper
function. The wrapper
function finally executes the function func
along with its arguments.
Decorators with Class Methods
Decorators can also be used with class methods. Here’s an example:
class MyDecorator:
def __init__(self, func):
self.func = func
def __call__(self, *args, **kwargs):
print('Before function execution')
self.func(*args, **kwargs)
print('After function execution')
@MyDecorator
def say_hello(name):
print(f'Hello, {name}!')
say_hello('Alice')
# Output:
# Before function execution
# Hello, Alice!
# After function execution
In this example, MyDecorator
is a class that acts as a decorator. The __init__
method stores the function, and the __call__
method is used to make the class instance callable.
Multiple Decorators
You can also use multiple decorators on a single function:
def decorator1(func):
def wrapper():
print('Decorator 1')
func()
return wrapper
def decorator2(func):
def wrapper():
print('Decorator 2')
func()
return wrapper
@decorator1
@decorator2
def say_hello():
print('Hello!')
say_hello()
# Output:
# Decorator 1
# Decorator 2
# Hello!
In this example, when say_hello
is called, it’s first passed to decorator2
, and the result is then passed to decorator1
. So, the output of decorator2
becomes the input for decorator1
.
These advanced techniques can help you make the most out of Python decorators. However, remember that with great power comes great responsibility. Overusing decorators or using them inappropriately can make your code harder to understand and debug, so use them judiciously.
Built-In Python Decorators:
While creating your own decorators can be powerful, Python also provides several built-in decorators that can be used to modify the behavior of your methods. These include @staticmethod
, @classmethod
, and @property
.
The @staticmethod Decorator
The @staticmethod
decorator is used to define a method within a class that doesn’t access any instance or class-specific data.
class MyClass:
@staticmethod
def my_method():
return 'Hello, World!'
print(MyClass.my_method())
# Output:
# Hello, World!
In this example, my_method
is a static method, meaning it can be called on the class itself, without creating an instance of the class.
The @classmethod Decorator
The @classmethod
decorator is used to define a method within a class that has access to class-specific data and methods.
class MyClass:
greeting = 'Hello, World!'
@classmethod
def my_method(cls):
return cls.greeting
print(MyClass.my_method())
# Output:
# Hello, World!
In this example, my_method
is a class method, meaning it has access to the greeting
class variable.
The @property Decorator
The @property
decorator is used to define methods in a class that should be accessed like attributes, without needing to call them like a method.
class MyClass:
def __init__(self):
self._greeting = 'Hello, World!'
@property
def greeting(self):
return self._greeting
my_instance = MyClass()
print(my_instance.greeting)
# Output:
# Hello, World!
In this example, greeting
is a property, meaning it can be accessed like an attribute on instances of MyClass
, even though it’s defined as a method.
These built-in decorators can be powerful tools for organizing your code and controlling how your classes and methods behave. However, they should be used judiciously, as overuse can lead to code that is difficult to understand and maintain.
Troubleshooting Python Decorators: Common Issues and Solutions
While Python decorators are a powerful tool, they can sometimes lead to unexpected issues. Here are some common problems you might encounter and how to resolve them.
Maintaining the Original Function’s Metadata
When you wrap a function with a decorator, the original function’s metadata (like its name, docstring, etc.) gets hidden. Here’s an example:
def my_decorator(func):
def wrapper():
"""This is the wrapper function"""
func()
return wrapper
@my_decorator
def say_hello():
"""This is the say_hello function"""
print('Hello!')
print(say_hello.__doc__)
# Output:
# This is the wrapper function
As you can see, the say_hello
function’s docstring is replaced by the wrapper
function’s docstring. This can be problematic when you’re trying to debug your code or when you’re using tools that auto-generate documentation.
Solution: The functools.wraps Decorator
To solve this issue, Python provides a built-in decorator functools.wraps
that helps to preserve the original function’s metadata:
from functools import wraps
def my_decorator(func):
@wraps(func)
def wrapper():
"""This is the wrapper function"""
func()
return wrapper
@my_decorator
def say_hello():
"""This is the say_hello function"""
print('Hello!')
print(say_hello.__doc__)
# Output:
# This is the say_hello function
In this example, the @wraps(func)
decorator is used inside the my_decorator
function to preserve the say_hello
function’s metadata.
Remember, understanding and troubleshooting these common issues can help you use Python decorators more effectively and efficiently. Happy coding!
Python Decorators: Understanding the Fundamentals
To truly master Python decorators, it’s essential to understand the core concepts they’re built upon: functions, closures, and the decorator pattern. Let’s dive into each of these.
Python Functions: The Building Blocks
In Python, functions are first-class objects. This means that functions in Python can be passed around and used as arguments, just like any other object (string, int, float, list, etc.). Here’s an example:
def greet(name):
return f'Hello, {name}!'
def welcome(greet, name):
return greet(name)
print(welcome(greet, 'Alice'))
# Output:
# Hello, Alice!
In this example, the greet
function is passed as an argument to the welcome
function.
Closures in Python: Remembering the Values
A closure in Python is a function object that has access to variables from its enclosing lexical scope, even when the function is called outside that scope. This means that it remembers the values of those variables, even if they’re not present in memory.
def outer_func(x):
def inner_func(y):
return x + y
return inner_func
closure = outer_func(10)
print(closure(5))
# Output:
# 15
In this example, inner_func
is a closure that remembers the value of x
, even when it’s called outside outer_func
.
The Decorator Pattern: Modifying Function Behavior
The decorator pattern is a design pattern in Python that allows you to wrap a function or method, thereby extending or modifying its behavior. In Python, decorators are a specific application of this pattern. Here’s a simple example of a decorator that modifies the behavior of the say_hello
function:
def my_decorator(func):
def wrapper():
print('Before function execution')
func()
print('After function execution')
return wrapper
@my_decorator
def say_hello():
print('Hello!')
say_hello()
# Output:
# Before function execution
# Hello!
# After function execution
In this example, my_decorator
is a decorator that modifies the behavior of say_hello
by printing ‘Before function execution’ and ‘After function execution’ before and after the function execution, respectively.
By understanding these core concepts, you’ll have a solid foundation to understand Python decorators and how they can be used to make your code more efficient and organized.
Python Decorators in Web Development and Beyond
Python decorators are not just limited to simple Python scripts; they play a crucial role in web development frameworks like Flask and Django. They also pave the way to understanding more advanced Python concepts like context managers and metaclasses.
Python Decorators in Flask and Django
In Flask, decorators are used extensively for route handlers. Here’s a simple example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
In this example, @app.route('/')
is a decorator that tells Flask what URL should trigger the hello_world
function.
Similarly, Django uses decorators to manage user permissions and to create view functions.
Exploring Related Concepts: Context Managers and Metaclasses
Once you’re comfortable with decorators, you might want to explore related advanced Python concepts like context managers and metaclasses.
- Context managers allow you to manage resources efficiently. They are created using
@contextlib.contextmanager
decorator andwith
statement. Metaclasses are ‘classes of a class’ that can customize class creation. They are an advanced topic and not commonly used, but can be powerful when used correctly.
Further Resources for Mastering Python Decorators
If you’re interested in diving deeper into Python decorators, here are some additional resources that you might find helpful:
- Python Decorators – A Gentle Introduction – This guide provides a simple introduction to Python’s decorators.
Primer on Python Decorators – Another comprehensive guide to decorators, with plenty of examples and explanations.
Python Decorators in Web Frameworks – A guide to using decorators in Flask, one of the most popular Python web frameworks.
Remember, mastering Python decorators takes practice. So don’t get discouraged if you don’t understand everything at first. Keep learning and coding, and you’ll get there!
Wrapping Up: Mastering Python Decorators
In this comprehensive guide, we’ve delved deep into the world of Python decorators, a powerful tool for enhancing the functionality of your Python code.
We began with the basics, understanding what Python decorators are and how to use them at a beginner level. We then explored more advanced techniques, such as using decorators with parameters, class methods, and even applying multiple decorators to a single function. Along the way, we tackled common issues that you might encounter when using decorators, such as maintaining the original function’s metadata, and provided solutions to help you overcome these challenges.
We also looked at built-in Python decorators like @staticmethod
, @classmethod
, and @property
and discussed how they can be used as alternatives to custom decorators. We even ventured into the realm of web development, discussing the relevance of Python decorators in web development frameworks like Flask and Django.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Custom Decorators | Highly customizable, can modify any function | Can be complex, may obscure logic |
Built-in Decorators | Easy to use, no need to write extra code | Limited functionality |
Whether you’re a beginner just starting out with Python decorators or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python decorators and their capabilities.
With practice, you’ll be able to use decorators to write cleaner, more efficient Python code. Happy coding!