‘-z’ Flag in Bash Script | Checking For Null or Empty Strings
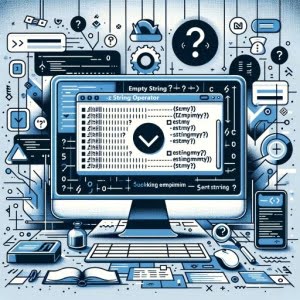
Are you finding it challenging to understand the ‘-z’ option in bash scripting? You’re not alone. Many developers find themselves puzzled when it comes to handling this particular option in bash scripting. Think of the ‘-z’ option as a vigilant gatekeeper, checking if a string is null or empty. It’s a powerful tool that can help you prevent errors and make your scripts more robust.
In this guide, we’ll walk you through the process of using the -z option in bash scripting, from the basics to more advanced techniques. We’ll cover everything from simple checks for null or empty strings to complex scenarios where the -z option can come in handy.
Let’s dive in and start mastering the -z option in bash scripting!
TL;DR: What Does -z Do in Bash?
In bash scripting, the
-z
option checks if a string is null or empty. It can be used in anif
statement, with the syntaxif [ -z "$variable" ]
. This option can help prevents errors in scripts and strings.
Here’s a simple example:
variable=""
if [ -z "$variable" ]
then
echo "Variable is empty"
fi
# Output:
# Variable is empty
In this example, we’ve declared a variable and assigned it an empty string. Then, we used the -z option in an if statement to check if the variable is empty. Since the variable is indeed empty, the script prints ‘Variable is empty’.
This is just a basic way to use the -z option in bash, but there’s much more to learn about handling null or empty strings in bash scripting. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of -z in Bash Scripting
The -z option in bash scripting is a test operator that checks if a string is null. In simpler terms, it checks if the string is empty or not. It’s often used in conditional statements like if, while, or until to control the flow of the script based on whether a string is empty.
Here’s a code example demonstrating the basic use of -z:
name=""
if [ -z "$name" ]
then
echo "Name is empty"
else
echo "Name is not empty"
fi
# Output:
# Name is empty
In this example, we’ve declared a variable ‘name’ and assigned it an empty string. We then used the -z option in an if statement to check if the variable ‘name’ is empty. As ‘name’ is indeed empty, the script prints ‘Name is empty’.
The advantage of using the -z option is that it allows you to handle situations where a variable might not have a value. For instance, if a user forgets to enter their name in a script that requires it, the -z option can detect this and allow the script to handle it gracefully.
However, one potential pitfall to be aware of is that the -z option only checks if the string is null or empty. It doesn’t check for other types of values, such as integers or arrays. Therefore, it’s important to ensure that the variable you’re checking with -z is indeed expected to be a string.
Advanced Use of -z in Bash Scripting
As you become more familiar with bash scripting, you’ll find that the -z option can be used in more complex scenarios in conjunction with other options and commands.
One such scenario is when you need to check multiple conditions using logical operators. For instance, you might want to check if two strings are empty. Here’s how you can do it:
name=""
address=""
if [ -z "$name" ] && [ -z "$address" ]
then
echo "Name and address are empty"
else
echo "At least one of name or address is not empty"
fi
# Output:
# Name and address are empty
In this example, we’re using the logical AND operator (&&) to check if both ‘name’ and ‘address’ are empty. If both are empty, the script will echo ‘Name and address are empty’. If at least one of them is not empty, it will echo ‘At least one of name or address is not empty’.
This demonstrates how the -z option can be used in combination with other options and commands to create more complex conditional statements in bash scripting. It’s a powerful tool that can significantly increase the robustness and flexibility of your scripts.
Exploring Alternatives to -z in Bash
While the -z option is a powerful tool in bash scripting, it’s not the only way to check if a string is null or empty. There are other methods you can use, each with its own advantages and disadvantages.
Using -n Option
The -n option is essentially the opposite of the -z option. While -z checks if a string is null or empty, -n checks if a string is not null. Here’s an example:
name="John"
if [ -n "$name" ]
then
echo "Name is not empty"
else
echo "Name is empty"
fi
# Output:
# Name is not empty
In this example, we’re checking if the variable ‘name’ is not null using the -n option. Since ‘name’ is indeed not empty, the script echoes ‘Name is not empty’.
The advantage of using -n is that it allows you to write conditions in a more intuitive way when you’re specifically looking for non-empty strings. However, like -z, it only checks for null or empty strings and doesn’t check for other types of values.
Using String Comparison Operator
Another way to check if a string is null or empty in bash scripting is to use the string comparison operator. Here’s how you can do it:
name=""
if [ "$name" = "" ]
then
echo "Name is empty"
else
echo "Name is not empty"
fi
# Output:
# Name is empty
In this example, we’re comparing the variable ‘name’ to an empty string. If ‘name’ is indeed empty, the script will echo ‘Name is empty’.
The advantage of this method is that it’s very explicit and intuitive, even for beginners. However, it’s slightly more verbose than using -z or -n, and like them, it doesn’t check for other types of values.
In conclusion, while -z is a powerful option for checking if a string is null or empty in bash scripting, there are other methods you can use depending on your specific needs and preferences. It’s always a good idea to know multiple ways to accomplish a task in bash scripting, as it increases your flexibility and allows you to write scripts that are more robust and easier to understand.
Troubleshooting -z in Bash Scripting
While the -z option is a valuable tool in bash scripting, it’s not without its quirks. Here we’ll discuss some common issues you may encounter when using the -z option, along with solutions and workarounds.
Handling Whitespace Characters
One common issue is the unexpected behavior when dealing with whitespace characters. For instance, you might expect a string containing only whitespace characters to be considered empty, but that’s not the case with the -z option.
Here’s an example:
name=" "
if [ -z "$name" ]
then
echo "Name is empty"
else
echo "Name is not empty"
fi
# Output:
# Name is not empty
In this example, we’ve assigned a string containing a single space to the variable ‘name’. However, the -z option considers this string as not empty, thus the script prints ‘Name is not empty’.
This happens because the -z option checks if the string is null or has zero length. A string containing whitespace characters is not null and its length is not zero, so -z considers it as not empty.
To handle this, you can use the trim functionality to remove leading and trailing whitespace characters before checking with -z.
name=" "
name=$(echo "$name" | tr -d ' ')
if [ -z "$name" ]
then
echo "Name is empty"
else
echo "Name is not empty"
fi
# Output:
# Name is empty
In this modified script, we’re using the tr command to remove spaces from the ‘name’ variable before checking it with -z. Now the script correctly identifies the ‘name’ variable as empty.
This is just one example of the potential issues you might encounter when using the -z option in bash scripting. Always be aware of the specific behavior of the -z option and other bash commands, and don’t hesitate to test your scripts thoroughly to ensure they behave as expected.
Bash Scripting and Condition Testing
Bash scripting is a powerful tool for automating tasks in Unix-like operating systems. It allows you to write scripts that can execute multiple commands, control the flow of execution, and manipulate data.
One of the key features of bash scripting is the ability to test conditions using different options. These options can be used in conditional statements like if, while, or until to control the flow of the script based on certain conditions.
Here’s a basic example of a conditional statement in bash scripting:
number=5
if [ $number -gt 3 ]
then
echo "Number is greater than 3"
else
echo "Number is not greater than 3"
fi
# Output:
# Number is greater than 3
In this script, we’re using the -gt (greater than) option to check if the variable ‘number’ is greater than 3. If it is, the script echoes ‘Number is greater than 3’. If it’s not, it echoes ‘Number is not greater than 3’.
Importance of Empty String Handling
Handling null or empty strings is a common requirement in bash scripting. For instance, you might have a script that asks the user to input their name, and you want to check if the user actually entered a name before proceeding.
This is where the -z option comes in handy. By using the -z option, you can check if a string is null or empty, and handle the situation accordingly. It’s a simple yet powerful tool that can help you make your bash scripts more robust and reliable.
Remember, the key to effective bash scripting is understanding the different options and how to use them to test conditions. Whether it’s checking if a string is null with -z, or performing more complex checks with other options, mastering condition testing is essential to becoming proficient in bash scripting.
Exploring -z in Larger Bash Scripts
The -z option is not just a tool for simple bash scripts. It plays a crucial role in larger scripts and projects, where handling null or empty strings becomes increasingly important. Whether it’s validating user input, managing configuration options, or controlling the flow of complex scripts, the -z option is an indispensable tool in your bash scripting toolkit.
Expanding Your Bash Scripting Skills
While mastering the -z option is a significant step, it’s just one part of the wider world of bash scripting. There are many other concepts and techniques to explore, such as conditional statements, loops, and other bash scripting fundamentals.
For instance, you might want to learn more about how to use logical operators in conjunction with the -z option to create more complex conditions. Or perhaps you’re interested in how to handle arrays in bash scripting, or how to read and write files.
The more you learn about bash scripting, the more powerful and flexible your scripts can become. So don’t stop at -z. Continue to explore, experiment, and expand your bash scripting skills.
Further Resources for Mastering Bash Scripting
To help you on your journey, here are some resources that provide more in-depth information and tutorials on bash scripting:
- Advanced Bash-Scripting Guide: This is a comprehensive guide to bash scripting that covers everything from basic to advanced topics.
Bash Academy: This is an interactive learning platform that teaches bash scripting through hands-on exercises and quizzes.
Bash Scripting Tutorial: This tutorial covers all the basics of bash scripting, including conditional statements, loops, and functions.
Remember, mastering bash scripting is a journey. Don’t be afraid to experiment, make mistakes, and learn from them. Happy scripting!
Recap: Checking Bash Strings with -z
In this comprehensive guide, we’ve delved into the world of bash scripting with a focus on the -z option, a powerful tool for checking if a string is null or empty.
We began with the basics, understanding what the -z option does and how to use it in simple scenarios. We then moved on to more advanced uses, demonstrating how the -z option can be used in conjunction with other options and commands for more complex scenarios.
Along the journey, we encountered some common issues that may arise when using the -z option, such as handling whitespace characters, and provided solutions and workarounds for each. We also explored alternative approaches to checking if a string is null or empty in bash scripting, including the -n option and the string comparison operator.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
-z option | Checks if string is null or empty | Doesn’t check for other types of values |
-n option | Checks if string is not null | Doesn’t check for other types of values |
String Comparison Operator | Explicit and intuitive | More verbose than using -z or -n |
Whether you’re just starting out with bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the -z option and its role in handling null or empty strings.
The ability to accurately check for null or empty strings is a key skill in bash scripting, and mastering the -z option is a significant step towards that. Happy scripting!