Using BASH IF / ELSE Statements: Shell Script Conditionals Explained
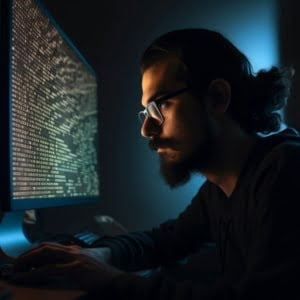
Have you ever wondered how to make your Bash scripts more powerful and flexible? One of the key skills you need to achieve this is mastering conditional statements, specifically bash if, elif, and else statements. By the end of this blog post, you’ll be well-equipped to handle these conditionals with ease, making your scripts more efficient and versatile.
Whether you’re a systems administrator or are building your own internet application, understanding server-side scripting is essential for automating routine server tasks. At IOFLOOD we love to automate routine sysadmin tasks to make sure our dedicated servers stay in tip top shape. Knowing how to use If, Elif, and Else statements in Bash scripting can add powerful capabilities to your shell scripts, and make your life a whole lot easier.
Table of Contents
What is a bash if / then / else statement?
BASH IF / THEN / ELSE are commands usable in Linux Shell scrips. As a “conditional branch”, the code evaluates IF some condition is true, and THEN will execute a set of commands. Alternately, ELSE specifies different commands to run if the “IF” condition is not satisfied.
In some programming languages, the keyword “THEN” keyword is implied, but in BASH it is a required part of the syntax.
Syntax of If, Elif, and Else in Bash
Before diving into the examples, let’s first take a look at the general syntax of If, Elif, and Else statements in Bash. Familiarizing yourself with the basic structure will make it easier to understand and apply the examples that follow.
General syntax explanation:
An If statement in Bash is written like this:
if [condition]; then commands fi
If the condition is true, the commands within the If statement will be executed. The statement ends with the fi keyword.
An Elif (short for “else if”) statement can be used to test multiple conditions:
if [condition1]; then commands1 elif [condition2]; then commands2 else commands3 fi
In this case, if condition1 is true, commands1 will be executed; if condition1 is false, AND condition2 is true, commands2 will be executed. If neither condition is true, the commands3 under the Else statement will be executed.
Double bracket syntax:
In some cases, you may see double brackets [[ … ]] used instead of single brackets [ … ] in the condition. This is a newer syntax with some advantages, such as better support for string comparisons and logical operators. You can use double brackets interchangeably with single brackets in most cases.
Formatting and aesthetics:
To improve the readability of your Bash scripts, you can use indentation and whitespace. While not required, proper formatting will make your code easier to understand and maintain.
Now that you have a basic understanding of the syntax, let’s dive into some specific examples to see how you can use If, Elif, and Else statements in your Bash scripts.
Specific Examples
Now that you know the basic syntax, let’s go over all the great things you can do with BASH IF statements.
String equality:
To check if two strings are equal, you can use the == operator:
string1="hello" string2="world" if [[ $string1 == $string2 ]]; then echo "Strings are equal." else echo "Strings are not equal." fi
In this example, the script will output “Strings are not equal,” since the values of string1 and string2 are different.
Number equality:
To compare numbers, you can use the -eq operator:
number1=5 number2=10 if [[ $number1 -eq $number2 ]]; then echo "Numbers are equal." else echo "Numbers are not equal." fi
Here, the script will output “Numbers are not equal,” as number1 and number2 have different values.
Less than or greater than a number:
You can use the -lt operator to check if one number is less than another, or -gt to check if one number is greater than another. Here is the -lt example:
number1=5 number2=10 if [[ $number1 -lt $number2 ]]; then echo "Number1 is less than Number2." else echo "Number1 is not less than Number2." fi
In this case, the script will output “Number1 is less than Number2,” since 5 is less than 10.
Logical AND operator:
To check if two conditions are both true, you can use the && operator:
number=15 if [[ $number -gt 10 && $number -lt 20 ]]; then echo "Number is between 10 and 20." else echo "Number is not between 10 and 20." fi
This script will output “Number is between 10 and 20,” since 15 is greater than 10 and less than 20.
Logical OR operator:
To check if at least one of two conditions is true, you can use the || operator:
number=5 if [[ $number -lt 10 || $number -gt 20 ]]; then echo "Number is either less than 10 or greater than 20." else echo "Number is between 10 and 20." fi
In this example, the script will output “Number is either less than 10 or greater than 20,” since 5 is less than 10.
Elif condition:
Elif, spoken as “else if”, is a command that will perform another comparison, if the previous comparison evaluated as false. You can use an Elif statement to test multiple conditions, as shown in this example:
number=25 if [[ $number -lt 10 ]]; then echo "Number is less than 10." elif [[ $number -gt 20 ]]; then echo "Number is greater than 20." else echo "Number is between 10 and 20." fi
The script will output “Number is greater than 20,” since 25 is greater than 20.
If Else statement:
Here’s an example of using an If Else statement with a simple condition:
is_raining=true if [[ $is_raining == true ]]; then echo "It's raining, bring an umbrella!" else echo "It's not raining, enjoy the sunshine!" fi
This script will output “It’s raining, bring an umbrella!” because the value of is_raining is true.
Extra Tips and Tricks
While you now have a strong grasp of If, Elif, and Else statements, there are a few more tips and tricks to keep in mind as you continue your Bash scripting journey. These extra insights can help you optimize your scripts and avoid potential pitfalls.
Nested If statements:
Sometimes, you may need to use nested If statements to test multiple conditions:
number=35 if [[ $number -gt 20 ]]; then if [[ $number -lt 40 ]]; then echo "Number is between 20 and 40." else echo "Number is greater than 40." fi else echo "Number is less than or equal to 20." fi
In this example, the script will output “Number is between 20 and 40,” since 35 is greater than 20 and less than 40.
Using test command:
You can use the test command as an alternative to the [ … ] or [[ … ]] syntax:
number1=5 number2=10 if test $number1 -eq $number2; then echo "Numbers are equal." else echo "Numbers are not equal." fi
This script will output “Numbers are not equal,” as number1 and number2 have different values.
Exit status of commands:
You can use the exit status of a command as a condition in an If statement:
filename="example.txt" if touch "$filename"; then echo "File created successfully." else echo "Failed to create file." fi
The touch command creates an empty file and returns an exit status of 0 if successful or non-zero if it fails. In this case, the script will output “File created successfully.”
Using arithmetic expressions:
You can use the (( … )) syntax to perform arithmetic operations and comparisons:
number=42 if (( number % 2 == 0 )); then echo "Number is even." else echo "Number is odd." fi
This script will output “Number is even,” as 42 is an even number.
Combining conditions:
You can combine multiple conditions using -a (AND) and -o (OR) operators within single brackets:
number=15 if [ $number -gt 10 -a $number -lt 20 ]; then echo "Number is between 10 and 20." else echo "Number is not between 10 and 20." fi
This script will output “Number is between 10 and 20,” since 15 is greater than 10 and less than 20.
Conclusion
Congratulations! You’ve now learned the basics of If, Elif, and Else statements in Bash scripting, and you’ve seen some practical examples that demonstrate their power and flexibility. As you work on your server-side scripting, you’ll find that mastering these conditional statements will make your scripts more efficient and versatile.
Now that you have a solid foundation, you can continue to expand your Bash scripting skills. There are plenty of resources available online to help you along the way. Don’t forget to experiment and practice, and feel free to share your experiences and tips in the comments section below. Happy scripting!
Do you love servers?
We Do! Whether you’re just getting an app off the ground or you’re a seasoned sysadmin at a hosting provider, I think we can all appreciate the fun of putting together a quick bash script. At IOFLOOD, we would be happy to help you with any dedicated server hosting needs. Email us at sales[at]ioflood.com or visit our website at https://ioflood.com to learn how our dedicated server offerings can help you with your hosting needs.