How Can I Use Foreach Loops in Bash Script?
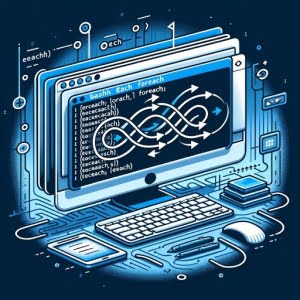
Are you finding the ‘foreach’ loop in Bash a bit challenging? You’re not alone. Many developers find themselves in a loop of confusion when it comes to using ‘foreach’ loops in Bash. But don’t worry, we’re here to help.
Think of the ‘foreach’ loop as a skilled conductor, orchestrating your code to perform tasks repetitively and efficiently. It’s a powerful tool that can significantly streamline your Bash scripts, making them more readable and maintainable.
This guide will walk you through the basics to advanced usage of the ‘foreach’ loop in Bash. We’ll cover everything from simple loops to more complex iterations, as well as alternative approaches and common issues you might encounter.
So, let’s dive in and start mastering the ‘foreach’ loop in Bash!
TL;DR: How Do I Use the ‘Foreach’ Loop in Bash?
Normally a
foreach
loop is used with the syntax,foreach n ( 1...5 )
. However, the'foreach'
loop is not supported in Bash. Due to this you must use a'for'
loop orwhile
loop.
Here’s a simple example:
for i in {1..5}; do echo $i; done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we’ve created a ‘for’ loop that acts as a ‘foreach’ loop. The loop iterates over the numbers 1 through 5, echoing each number on a new line. This is a basic way to use the ‘foreach’ loop in Bash, but there’s much more to learn about looping and iteration in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Bash Foreach Loop
- Delving into Advanced Bash Foreach Loop
- Exploring Alternative Iteration Methods in Bash
- Troubleshooting Common Issues in Bash Foreach Loops
- Understanding Loops and Iteration in Bash
- Exploring the Relevance of Bash Foreach Loops
- Wrapping Up: Mastering Bash Foreach Loops
Understanding the Basics of Bash Foreach Loop
In Bash, the ‘foreach’ loop is commonly expressed as a ‘for’ loop. The loop operates by performing a set of commands for each item in a list. This makes it an incredibly useful tool for automating repetitive tasks.
Let’s look at a simple example of a ‘for’ loop acting as a ‘foreach’ loop:
for fruit in Apple Banana Cherry; do echo "I love $fruit"; done
# Output:
# I love Apple
# I love Banana
# I love Cherry
In this example, the ‘for’ loop iterates over the list of fruits (Apple, Banana, Cherry). For each iteration, it executes the echo
command to print out a statement about each fruit. The variable fruit
takes on the value of each item in the list, one by one.
This basic use of the ‘foreach’ loop in Bash is powerful in its simplicity. It allows you to perform an operation on a series of items, automating repetitive tasks and making your scripts more efficient.
However, it’s important to be aware of potential pitfalls when using ‘for’ loops as ‘foreach’ loops in Bash. For instance, if your list items contain spaces, the loop will treat each word as a separate item. To avoid this, you’ll need to use quotes or a different method to handle items with spaces.
Delving into Advanced Bash Foreach Loop
As you become more comfortable with the basic ‘foreach’ loop in Bash, you can start exploring its more complex applications. One such application is iterating over arrays or files, which can greatly enhance the functionality of your scripts.
Iterating Over Arrays
In Bash, you can use the ‘foreach’ loop to iterate over an array. Here’s an example:
fruits=("Apple" "Banana" "Cherry")
for fruit in "${fruits[@]}"; do echo "I love $fruit"; done
# Output:
# I love Apple
# I love Banana
# I love Cherry
In this example, we first define an array of fruits. We then use a ‘for’ loop to iterate over the array, echoing a statement for each fruit. Note the use of "${fruits[@]}"
to correctly handle array items with spaces.
Iterating Over Files
You can also use a ‘foreach’ loop to iterate over files in a directory. This can be especially useful for batch processing files. Here’s an example:
for file in *.txt; do echo "Processing $file"; done
# Output (Assuming there are three .txt files: file1.txt, file2.txt, file3.txt):
# Processing file1.txt
# Processing file2.txt
# Processing file3.txt
In this example, the ‘for’ loop iterates over all .txt files in the current directory, echoing a statement for each file.
These advanced uses of the ‘foreach’ loop in Bash open up a world of possibilities for automating tasks and processing data. As you explore these techniques, remember to carefully consider the structure of your data and the desired outcomes of your scripts to select the most effective looping method.
Exploring Alternative Iteration Methods in Bash
While the ‘foreach’ loop (expressed as a ‘for’ loop in Bash) is a powerful tool for iterating over items, it’s not the only method available. Let’s explore some alternative approaches, namely the ‘while’ and ‘until’ loops.
The ‘While’ Loop
The ‘while’ loop performs a set of commands as long as a certain condition is true. Here’s an example:
count=1
while [ $count -le 5 ]; do echo "Count: $count"; ((count++)); done
# Output:
# Count: 1
# Count: 2
# Count: 3
# Count: 4
# Count: 5
In this example, the ‘while’ loop continues to echo the count as long as the count is less than or equal to 5. The ((count++))
command increments the count after each iteration.
The ‘Until’ Loop
The ‘until’ loop is similar to the ‘while’ loop, but it performs a set of commands as long as a certain condition is false. Here’s an example:
count=1
until [ $count -gt 5 ]; do echo "Count: $count"; ((count++)); done
# Output:
# Count: 1
# Count: 2
# Count: 3
# Count: 4
# Count: 5
In this example, the ‘until’ loop continues to echo the count until the count is greater than 5.
Both the ‘while’ and ‘until’ loops offer alternative ways to handle iteration in Bash. They can be more flexible than the ‘foreach’ loop in certain scenarios, as they allow for condition-based iteration rather than iterating over a fixed list. However, they can also be more complex to set up and may not be as intuitive for simple, list-based tasks.
Troubleshooting Common Issues in Bash Foreach Loops
Like any programming construct, Bash foreach loops can sometimes behave unexpectedly. Let’s delve into some common issues and their solutions.
Off-By-One Errors
Off-by-one errors occur when the loop iterates one time too many or one time too few. This often happens due to incorrect loop conditions. For example:
for i in {1..5}; do echo $i; done
# Output:
# 1
# 2
# 3
# 4
# 5
Here, the loop correctly iterates from 1 to 5. But if you wanted to iterate from 1 to 4, and mistakenly kept the upper limit as 5, you’d have an off-by-one error.
Problems with Special Characters
Special characters in list items can cause unexpected behavior in Bash foreach loops. For instance, an item with a space will be treated as two separate items. To handle this, you can use quotes:
for word in 'Hello World' 'Bash Foreach'; do echo $word; done
# Output:
# Hello World
# Bash Foreach
In this example, each phrase is treated as a single item despite containing a space.
Empty List Items
If your list contains an empty item, the loop will still iterate over it. This might cause unexpected results if your loop body doesn’t account for empty items.
for item in 'Apple' '' 'Cherry'; do echo "Item: $item"; done
# Output:
# Item: Apple
# Item:
# Item: Cherry
In this example, the loop iterates over an empty string, resulting in an empty item output.
These are just a few examples of the issues you might encounter when working with Bash foreach loops. By understanding these potential pitfalls, you can write more robust and reliable scripts.
Understanding Loops and Iteration in Bash
Before we delve deeper into the ‘foreach’ loop in Bash, let’s take a step back and understand the fundamental concepts of loops and iteration.
Loops in Bash
A loop is a programming construct that allows you to execute a block of code repeatedly. The loop continues until a certain condition is met. In Bash, there are several types of loops, including ‘for’, ‘while’, and ‘until’ loops.
The ‘foreach’ loop, expressed as a ‘for’ loop in Bash, is particularly useful for iterating over items in a list or array.
Understanding Iteration
Iteration is the process of repeating a set of operations for each item in a collection. In the context of a Bash ‘foreach’ loop, the collection could be a list of strings, numbers, or array elements.
Here’s an example of a basic Bash ‘for’ loop that prints the numbers 1 to 3:
for number in {1..3}; do echo $number; done
# Output:
# 1
# 2
# 3
In this example, the loop iterates over the numbers 1 to 3, echoing each number on a new line.
Bash and Data Types
Bash primarily deals with strings, but it can also handle numbers and arrays. When using a ‘foreach’ loop in Bash, it’s important to understand how Bash handles these data types.
For example, Bash treats each item in a list as a string by default. If you want to perform arithmetic operations, you’ll need to use specific syntax to tell Bash to treat the items as numbers.
Here’s an example of a Bash ‘for’ loop that adds 5 to each number in a list:
for number in {1..3}; do echo $((number + 5)); done
# Output:
# 6
# 7
# 8
In this example, the $(( ))
syntax tells Bash to treat number
as an integer and perform the addition operation.
Understanding these fundamental concepts will give you a solid foundation for mastering the ‘foreach’ loop in Bash.
Exploring the Relevance of Bash Foreach Loops
The ‘foreach’ loop is not just a standalone command in Bash scripting but a crucial part of larger scripts and projects. Its ability to automate repetitive tasks makes it an indispensable tool for any Bash programmer.
Bash Foreach Loops in Larger Scripts
In larger scripts, ‘foreach’ loops can be used to iterate over arrays or lists of data, process files, and automate repetitive tasks. For instance, you might use a ‘foreach’ loop to process a list of files, perform operations on each file, and generate a report.
Here’s an example of a ‘foreach’ loop in a larger script:
# Define an array of filenames
files=("file1.txt" "file2.txt" "file3.txt")
# Iterate over the array and perform operations on each file
for file in "${files[@]}"; do
# Print the filename
echo "Processing $file"
# Perform some operation on the file (e.g., count lines)
lines=$(wc -l < "$file")
echo "The file $file has $lines lines"
done
# Output (Assuming each file has 10 lines):
# Processing file1.txt
# The file file1.txt has 10 lines
# Processing file2.txt
# The file file2.txt has 10 lines
# Processing file3.txt
# The file file3.txt has 10 lines
In this example, the ‘foreach’ loop is part of a larger script that processes a list of files and performs operations on each file.
Exploring Related Bash Concepts
As you become more comfortable with ‘foreach’ loops in Bash, you might want to explore related concepts like conditional statements (if, else, case), functions, and other types of loops (while, until). These concepts can greatly enhance your Bash scripting skills and allow you to write more complex and powerful scripts.
Further Resources for Bash Foreach Mastery
For those looking to dive deeper into the world of Bash scripting and ‘foreach’ loops, here are some resources that you might find helpful:
- Advanced Bash-Scripting Guide: This comprehensive guide covers all aspects of Bash scripting, including loops, functions, and conditional statements.
- Bash ForEach Loop Examples for Linux/Unix: This Cyberciti article offers various examples and explanations of how to use the foreach loop construct in Bash.
- Bash Scripting Tutorial: A detailed tutorial covering the basics of Bash scripting, including variables, loops, and conditional statements.
Wrapping Up: Mastering Bash Foreach Loops
In this comprehensive guide, we’ve delved into the world of Bash ‘foreach’ loops, a powerful tool for automating repetitive tasks in Bash scripts.
We began with the basics, learning how to use ‘foreach’ loops in Bash to iterate over a list of items. We then moved on to more advanced usage, such as iterating over arrays and files. Along the way, we tackled common issues you might encounter when using ‘foreach’ loops in Bash, such as off-by-one errors and problems with special characters, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to iteration in Bash, including the ‘while’ and ‘until’ loops. These alternatives offer more flexibility in certain scenarios, allowing for condition-based iteration rather than iterating over a fixed list.
Here’s a quick comparison of the iteration methods we’ve discussed:
Method | Flexibility | Use Case |
---|---|---|
Foreach Loop | Moderate | Iterating over a fixed list |
While Loop | High | Condition-based iteration |
Until Loop | High | Iterating until a condition is met |
Whether you’re just starting out with Bash scripting or looking to level up your skills, we hope this guide has given you a deeper understanding of ‘foreach’ loops in Bash and their applications.
With its balance of simplicity and power, the ‘foreach’ loop is an indispensable tool in any Bash programmer’s toolkit. Happy scripting!