Bash Script How-to | Check If String Contains a Substring
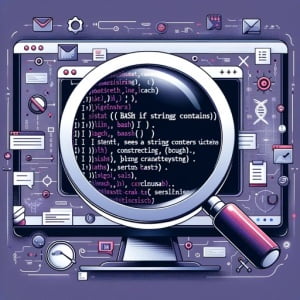
Are you finding it challenging to check if a string contains a substring in Bash? You’re not alone. Many developers find this task a bit tricky, but there’s a way to make it straightforward.
Think of Bash as a detective, capable of searching for clues within strings. It can sift through the characters and find the exact match you’re looking for, making it a powerful tool for string manipulation.
This guide will walk you through the process of checking if a string contains a substring in Bash, from basic use to more advanced techniques. We’ll cover everything from the simple syntax to handling special characters and case sensitivity, as well as alternative approaches.
So, let’s dive in and start mastering string manipulation in Bash!
TL;DR: How Do I Check if a String Contains a Substring in Bash?
In Bash, you can check if a string contains a substring using the
[[ $string = *substring* ]]
syntax. This command allows Bash to search within the string for the specified substring.
Here’s a simple example:
string='Hello, world!'
if [[ $string = *'world'* ]]; then
echo 'Found!'
fi
# Output:
# 'Found!'
In this example, we have a string ‘Hello, world!’ and we’re checking if it contains the substring ‘world’. The [[ $string = *substring* ]]
syntax is used to perform this check. If the substring is found within the string, the echo command prints ‘Found!’.
This is a basic way to check if a string contains a substring in Bash, but there’s much more to learn about string manipulation in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started: Basic Use of [[ $string = *substring* ]] Syntax in Bash
- Dealing with Case Sensitivity and Special Characters in Bash
- Exploring Alternatives: grep Command and case Statement
- Troubleshooting Common Issues in Bash String Checks
- Understanding Strings and Substrings in Bash
- The Power of String Manipulation in Bash Scripting
- Wrapping Up: Mastering String Manipulation in Bash
Getting Started: Basic Use of [[ $string = *substring* ]]
Syntax in Bash
If you’re new to Bash scripting or just starting to explore string manipulation, this section will guide you through the basic use of the [[ $string = *substring* ]]
syntax. This syntax is fundamental to checking if a string contains a substring in Bash.
Consider this example:
string='Welcome to the world of Bash scripting!'
if [[ $string = *'Bash scripting'* ]]; then
echo 'Substring found!'
else
echo 'Substring not found!'
fi
# Output:
# 'Substring found!'
In this example, we have a string ‘Welcome to the world of Bash scripting!’ and we’re checking if it contains the substring ‘Bash scripting’. If the substring is found within the string, the echo command prints ‘Substring found!’, otherwise, it prints ‘Substring not found!’.
The [[ $string = *substring* ]]
syntax works by comparing the string with a pattern. The asterisks (*) around the ‘substring’ act as wildcards, allowing any characters to be present before or after the ‘substring’. If the ‘substring’ is found anywhere within the ‘string’, the condition becomes true.
This syntax is simple and easy to use, making it a great starting point for beginners. However, it’s important to note that this syntax is case-sensitive and doesn’t handle special characters well. These issues will be addressed in the following sections.
Dealing with Case Sensitivity and Special Characters in Bash
As you delve deeper into Bash scripting, you’ll encounter scenarios where you need to handle case sensitivity and special characters. This section will guide you through these more advanced aspects of checking if a string contains a substring in Bash.
Case Sensitivity
By default, the [[ $string = *substring* ]]
syntax is case-sensitive. This means ‘BASH SCRIPTING’ and ‘Bash scripting’ are considered different. If you want to perform a case-insensitive search, you can convert both the string and the substring to the same case.
Here’s an example:
string='Welcome to the world of BASH scripting!'
substring='bash scripting'
if [[ ${string,,} = *${substring,,}* ]]; then
echo 'Substring found!'
else
echo 'Substring not found!'
fi
# Output:
# 'Substring found!'
In this example, both the string and the substring are converted to lowercase using the ${variable,,}
syntax before performing the check. This ensures that the check is not case-sensitive.
Handling Special Characters
Special characters in Bash are characters that have a specific meaning. If your substring contains special characters, the [[ $string = *substring* ]]
syntax might not work as expected. In this case, you can use the grep
command with the -q
(quiet) option.
Consider this example:
string='Welcome to the world of Bash scripting!'
substring='$world'
if echo "$string" | grep -q "$substring"; then
echo 'Substring found!'
else
echo 'Substring not found!'
fi
# Output:
# 'Substring not found!'
In this example, the substring ‘$world’ contains a special character ‘$’. The grep -q "$substring"
command is used to search the string for the substring. The ‘-q’ option suppresses the output of grep
and only returns an exit status.
These techniques allow you to handle case sensitivity and special characters when checking if a string contains a substring in Bash, expanding your scripting capabilities.
Exploring Alternatives: grep
Command and case
Statement
While the [[ $string = *substring* ]]
syntax is a common method for checking if a string contains a substring in Bash, there are alternative approaches that provide more flexibility and control. In this section, we’ll explore two of these alternatives: the grep
command and the case
statement.
Using grep
Command
The grep
command is a powerful tool for pattern searching in Bash. It allows you to search within a string for a substring, even if the substring contains regular expressions or special characters.
Here’s an example:
string='Welcome to the world of Bash scripting!'
substring='Bash.*scripting'
if echo "$string" | grep -q -E "$substring"; then
echo 'Substring found!'
else
echo 'Substring not found!'
fi
# Output:
# 'Substring found!'
In this example, we’re using the grep -E
command to search for a substring that contains a regular expression. The -E
option enables extended regular expression syntax, allowing the use of special characters in the substring.
While grep
provides powerful pattern matching capabilities, it can be slower than the [[ $string = *substring* ]]
syntax for large strings or files.
Employing case
Statement
The case
statement in Bash can also be used to check if a string contains a substring. It provides a clean syntax and allows you to handle multiple patterns.
Here’s an example:
string='Welcome to the world of Bash scripting!'
substring='Bash'
case "$string" in
*"$substring"*) echo 'Substring found!' ;;
*) echo 'Substring not found!' ;;
esac
# Output:
# 'Substring found!'
In this example, the case
statement checks if the string contains the substring. If it does, it echoes ‘Substring found!’. If it doesn’t, it echoes ‘Substring not found!’.
The case
statement is a good option for checking multiple patterns, but it can be more verbose than the [[ $string = *substring* ]]
syntax or grep
command for simple checks.
In conclusion, while the [[ $string = *substring* ]]
syntax is a straightforward method for checking if a string contains a substring in Bash, the grep
command and case
statement provide alternative approaches that offer more flexibility. The best method to use depends on your specific needs and the complexity of your script.
Troubleshooting Common Issues in Bash String Checks
While Bash scripting can be incredibly powerful, it’s not without its quirks. When checking if a string contains a substring in Bash, you may encounter some common issues. Let’s discuss these potential pitfalls and how to navigate around them.
Dealing with Whitespace
Whitespace characters like spaces, tabs, and newlines can sometimes cause unexpected behavior. For instance, a trailing space in the substring can lead to a mismatch even if the rest of the substring is present in the string.
Consider this example:
string='Welcome to Bash'
substring='Bash '
if [[ $string = *$substring* ]]; then
echo 'Substring found!'
else
echo 'Substring not found!'
fi
# Output:
# 'Substring not found!'
In this example, the substring ‘Bash ‘ includes a trailing space, which is not present in the string ‘Welcome to Bash’. As a result, the substring is not found.
To avoid this issue, ensure that the substring does not contain any leading or trailing whitespace. If necessary, you can use the trim
command to remove whitespace from the substring.
Handling Special Characters
As discussed in the ‘Advanced Use’ section, special characters can cause issues with the [[ $string = *substring* ]]
syntax. If your substring contains special characters, consider using the grep
command or escaping the special characters with a backslash (\
).
Here’s an example of escaping special characters:
string='Welcome to $Bash'
substring='$Bash'
if [[ $string = *${substring//\/\\}* ]]; then
echo 'Substring found!'
else
echo 'Substring not found!'
fi
# Output:
# 'Substring found!'
In this example, the substring ‘$Bash’ contains a special character ‘$’. We escape the special character with a backslash (\
) using the ${variable//\/\\}
syntax, which replaces all occurrences of ‘\’ with ‘\’ in the variable.
By being aware of these common issues and knowing how to handle them, you can ensure that your Bash scripts work as expected and handle strings and substrings accurately.
Understanding Strings and Substrings in Bash
Before we delve deeper into checking if a string contains a substring in Bash, it’s crucial to understand the fundamentals of strings and substrings in Bash. This knowledge will help you grasp the underlying concepts of the operation.
What are Strings in Bash?
In Bash, a string is a sequence of characters. It can include letters, numbers, special characters, and even whitespace. A string can be any length, from a single character to thousands of characters.
You can create a string in Bash by assigning a sequence of characters to a variable. Here’s an example:
string='Hello, Bash!'
echo $string
# Output:
# 'Hello, Bash!'
In this example, we’ve created a string ‘Hello, Bash!’ and assigned it to the variable ‘string’. We then print the string using the echo
command.
What are Substrings in Bash?
A substring is a part of a string. It can be any length, from a single character to the entire string. For example, in the string ‘Hello, Bash!’, ‘Bash’ is a substring.
Bash provides various ways to extract a substring from a string. One common method is using the ${string:start:length}
syntax, where ‘start’ is the starting index and ‘length’ is the number of characters to extract. Here’s an example:
string='Hello, Bash!'
substring=${string:7:4}
echo $substring
# Output:
# 'Bash'
In this example, we’re extracting a substring from the string ‘Hello, Bash!’. The substring starts at index 7 and is 4 characters long, resulting in the substring ‘Bash’.
Understanding these basics of strings and substrings in Bash is essential for manipulating strings and checking if a string contains a substring.
The Power of String Manipulation in Bash Scripting
String manipulation is a fundamental skill in Bash scripting that extends far beyond checking if a string contains a substring. It plays a crucial role in various aspects of scripting, such as file handling and text processing.
File Handling with Bash
When working with files in Bash, string manipulation can be used to extract file paths, file names, and file extensions. For instance, you might need to check if a file path contains a specific directory or if a file name contains a specific pattern.
Text Processing with Bash
In text processing, string manipulation is used to search and replace text, format text, and more. For instance, you might need to check if a line of text contains a specific word or phrase.
Exploring Related Concepts
If you’re interested in digging deeper into string manipulation in Bash, there are several related concepts worth exploring, such as regular expressions and pattern matching.
- Regular expressions are a powerful tool for pattern matching in strings. They allow you to search for complex patterns within a string, making them a valuable skill for any Bash scripter.
Pattern matching in Bash is a broader concept that includes not only regular expressions but also glob patterns and other forms of pattern matching. Understanding pattern matching in Bash can open up a whole new world of possibilities for your scripts.
Further Resources for Bash Scripting Mastery
If you’re eager to learn more about string manipulation and Bash scripting in general, here are some external resources that offer in-depth tutorials and guides:
- Bash Guide for Beginners: This guide provides a comprehensive introduction to Bash scripting, including string manipulation.
Advanced Bash-Scripting Guide: For those who are already familiar with the basics, this guide delves into more advanced topics in Bash scripting.
Bash String Manipulation Examples: This article provides several practical examples of string manipulation in Bash, including checking if a string contains a substring.
Wrapping Up: Mastering String Manipulation in Bash
In this comprehensive guide, we’ve delved into the intricacies of checking if a string contains a substring in Bash. We’ve explored the syntax, usage, and potential pitfalls, providing you with a thorough understanding of this essential operation in Bash scripting.
We began with the basics, learning how to use the [[ $string = *substring* ]]
syntax to perform a simple check. We then progressed to more advanced topics, such as handling case sensitivity and special characters. Along the way, we tackled common issues you might encounter, such as dealing with whitespace and special characters, providing you with solutions and workarounds for each issue.
We also ventured into alternative approaches to checking if a string contains a substring in Bash, exploring the grep
command and the case
statement. Each of these methods has its advantages and disadvantages, and the best one to use depends on your specific needs and the complexity of your script.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Handling Special Characters |
---|---|---|---|
[[ $string = *substring* ]] syntax | Moderate | Low | Poor |
grep command | High | Moderate | Excellent |
case statement | Moderate | High | Good |
Whether you’re just starting out with Bash scripting or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of checking if a string contains a substring in Bash.
With this knowledge, you’re well-equipped to handle a wide range of string manipulation tasks in Bash. Happy scripting!