Manipulating JSON Arrays with jq | Example Guide
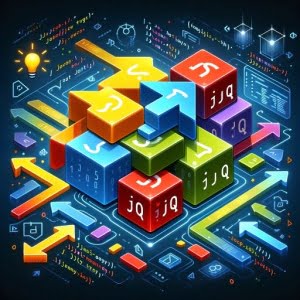
Are you finding it challenging to handle arrays in jq? You’re not alone. Many developers find themselves grappling with this task, but there’s a tool that can make this process a breeze.
Think of jq as a Swiss Army knife for JSON data, capable of slicing and dicing arrays with ease. It provides a versatile and handy tool for various tasks, from simple array manipulations to complex data transformations.
This guide will walk you through the ins and outs of working with arrays in jq, from basic operations to advanced techniques. We’ll cover everything from the creation, access, and modification of arrays to more complex operations like filtering, mapping, and reducing. We’ll also explore alternative approaches and discuss common issues and their solutions.
So, let’s dive in and start mastering arrays in jq!
TL;DR: How Do I Use jq to Work with JSON Arrays?
Working with arrays in
jq
involves creating, accessing, and modifying arrays using various commands. The commands follow common syntax such as, creating arrays and adding elements withecho '[]' | jq '. +='
, indexing with.["indexNumber"]
, modifying elements with.["indexNumber"] = "New Value"
. Here’s a simple example of creating an array:
echo '[]' | jq '. += ["element"]'
# Output:
# ["element"]
In this example, we’re using the echo
command to create an empty array, and then we’re using jq to add an element to it. The '. += ["element"]'
part of the command is where the magic happens. This is jq’s syntax for adding an element to an array. The +=
operator is used to append an element, and the element we’re appending is "element"
.
But there’s so much more to learn about handling arrays in jq. Continue reading for a more detailed guide, complete with code examples and explanations.
Table of Contents
Getting Started with Arrays in jq
jq, being a powerful command-line JSON processor, allows you to create, access, and modify arrays with ease.
Creating Arrays
Creating an array in jq is straightforward. Let’s start with a simple example:
echo '[]' | jq '. += ["Apple", "Banana", "Cherry"]'
# Output:
# ["Apple", "Banana", "Cherry"]
In this example, we use the echo
command to create an empty array, and then we use jq to add three elements to it: “Apple”, “Banana”, and “Cherry”. The '. += ["Apple", "Banana", "Cherry"]'
part of the command is jq’s syntax for adding elements to an array.
Accessing Arrays
Accessing elements in an array is also simple in jq. Let’s access the second element (“Banana”) from our array:
echo '["Apple", "Banana", "Cherry"]' | jq '.[1]'
# Output:
# "Banana"
In this command, .1
is used to access the second element of the array (remember, array indexing starts at 0).
Modifying Arrays
Modifying an array element is as simple as accessing it. Let’s change “Banana” to “Blueberry”:
echo '["Apple", "Banana", "Cherry"]' | jq '.[1]="Blueberry"'
# Output:
# ["Apple", "Blueberry", "Cherry"]
In this command, .1="Blueberry"
is used to change the second element of the array to “Blueberry”.
These basic operations are the building blocks for working with arrays in jq. However, it’s worth noting that while these commands are simple, they can be powerful when combined and used in more complex data manipulation tasks.
Advanced Array Operations in jq
As you become more comfortable with the basics of jq arrays, it’s time to explore more complex operations. We will focus on three key operations: filtering, mapping, and reducing.
Filtering Arrays
Filtering allows you to select elements from an array based on a certain condition. Here’s an example of filtering an array to select only the elements that start with the letter ‘B’:
echo '["Apple", "Banana", "Blueberry", "Cherry"]' | jq '.[] | select(startswith("B"))'
# Output:
# "Banana"
# "Blueberry"
In this command, the select(startswith("B"))
part is the filter. It selects only the elements that start with ‘B’.
Mapping Arrays
Mapping is a process of transforming each element in an array. Here’s an example of mapping an array to change all its elements to uppercase:
echo '["Apple", "Banana", "Blueberry", "Cherry"]' | jq '.[] | ascii_upcase'
# Output:
# "APPLE"
# "BANANA"
# "BLUEBERRY"
# "CHERRY"
In this command, the ascii_upcase
part is the mapping function. It transforms each element to uppercase.
Reducing Arrays
Reducing is a process of combining all elements in an array into a single value. Here’s an example of reducing an array to calculate the sum of its elements:
echo '[1, 2, 3, 4, 5]' | jq 'add'
# Output:
# 15
In this command, the add
function is used to calculate the sum of all elements in the array.
These advanced operations allow you to manipulate arrays in jq in powerful ways. They can be combined and used in complex data manipulation tasks, enabling you to handle almost any kind of JSON data.
Exploring Alternative Approaches to jq Arrays
While jq is a powerful tool for working with JSON arrays, there are alternative methods and tools that can be used to achieve similar results. Here, we’ll explore some of these alternatives, their advantages, disadvantages, and when to use them.
Using Third-Party Libraries
There are several third-party libraries available that can help simplify working with JSON arrays. One such library is underscore-cli
, a command-line utility that provides a wealth of useful functions for working with JSON data.
Here’s an example of filtering an array using underscore-cli
:
echo '["Apple", "Banana", "Blueberry", "Cherry"]' | underscore filter 'value.startsWith("B")'
# Output:
# ["Banana", "Blueberry"]
In this command, the filter 'value.startsWith("B")'
part is the filter. It selects only the elements that start with ‘B’.
Using Alternative Command-Line Tools
Another alternative is jtc
, a versatile command-line tool for JSON manipulations. It provides a rich set of features and can be a good alternative for complex JSON manipulations.
Here’s an example of mapping an array using jtc
:
echo '["Apple", "Banana", "Blueberry", "Cherry"]' | jtc -w "<B>l" -u
# Output:
# ["Banana", "Blueberry"]
In this command, the -w "<B>l" -u
part is the filter. It selects only the elements that start with ‘B’.
Tool | Pros | Cons |
---|---|---|
jq | Powerful, flexible, widely used | Can be complex for beginners |
underscore-cli | Simplifies complex tasks, rich feature set | Not as flexible as jq, less community support |
jtc | Rich feature set, good for complex tasks | Less intuitive syntax, less community support |
These alternative methods can be useful in certain scenarios, but it’s important to understand their limitations. While they can simplify certain tasks, they might not be as flexible or powerful as jq. Therefore, it’s recommended to use them as complementary tools to jq, rather than as replacements.
Common Issues and Solutions with jq Arrays
Working with jq arrays is generally straightforward, but you might occasionally encounter challenges. Let’s discuss some common issues and their solutions.
Syntax Errors
jq is quite particular about its syntax. A misplaced comma or a missing bracket can cause a syntax error. For instance, the following command will result in a syntax error:
echo '["Apple", "Banana", "Cherry"' | jq '.[]'
# Output:
# parse error: Unfinished JSON term at EOF at line 2, column 0
The issue here is the missing closing bracket in the array. To fix it, ensure your JSON is correctly formatted.
Unexpected Output
Sometimes, the output from a jq command might not be what you expected. For instance, consider the following command:
echo '["Apple", "Banana", "Cherry"]' | jq '.[3]'
# Output:
# null
The output is null
because there is no fourth element in the array (remember, array indexing starts at 0). To avoid this, ensure you’re accessing an index that exists in the array.
Dealing with Complex JSON Structures
Working with complex JSON structures can be challenging. For instance, accessing an array nested within multiple objects can be tricky. Here’s how you can do it:
echo '{"fruits": {"tropical": ["Mango", "Pineapple", "Papaya"]}}' | jq '.fruits.tropical[1]'
# Output:
# "Pineapple"
In this command, .fruits.tropical[1]
is used to access the second element of the tropical
array, which is nested within the fruits
object.
Remember, practice makes perfect. The more you work with jq and JSON, the more comfortable you’ll become with handling arrays and other data structures.
Understanding JSON and jq’s Approach to Arrays
To truly master jq arrays, we need to understand the fundamentals of JSON and the role arrays play in this data format.
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
The Role of Arrays in JSON
In JSON, an array is an ordered collection of values. An array can contain multiple values, and these values can be of various types, including strings, numbers, objects, or even other arrays. Here’s an example of a JSON array:
[
"Apple",
"Banana",
"Cherry"
]
In this example, the array contains three string values: “Apple”, “Banana”, and “Cherry”.
jq’s Approach to Handling JSON Arrays
jq provides a powerful and flexible way to work with JSON arrays. It allows you to perform a wide range of operations on arrays, from basic tasks like creating, accessing, and modifying arrays to more complex tasks like filtering, mapping, and reducing arrays.
For instance, here’s how you can filter an array in jq to select only the elements that start with the letter ‘A’:
echo '["Apple", "Banana", "Cherry"]' | jq '.[] | select(startswith("A"))'
# Output:
# "Apple"
In this command, the select(startswith("A"))
part is the filter. It selects only the elements that start with ‘A’.
Understanding these fundamentals of JSON and jq’s approach to handling arrays will help you better understand and use the various array operations in jq.
Expanding Your jq Array Skills
Understanding and mastering jq arrays is a valuable skill, but it’s just the tip of the iceberg. The power of jq extends far beyond arrays and can be a game-changer in larger scripts or projects.
Integrating jq in Larger Projects
jq’s array handling abilities can be incredibly useful in larger scripts or projects. Whether you’re working on data analysis, automation scripts, or web development, jq can help you manipulate and process JSON data efficiently.
For instance, you might need to extract specific data from a large JSON file or transform JSON data into a different format. With jq, these tasks become simple and straightforward.
Exploring Related Concepts
As you continue your journey with jq, consider exploring related concepts like JSON data manipulation and command-line processing. Understanding these concepts will not only enhance your jq skills but also broaden your overall programming and scripting abilities.
For example, you might learn how to use jq in combination with other command-line tools like curl
to fetch and process data from APIs, or how to use jq in your programming language of choice to handle JSON data.
Further Resources for jq Arrays
To help you continue learning and mastering jq arrays, here are some additional resources:
- jq Manual: The official manual for jq is a comprehensive resource that covers all aspects of jq, including arrays.
jq Cookbook: This cookbook provides a collection of recipes for solving common tasks with jq. It’s a great resource for learning by doing.
Learn jq With jq.play: This interactive tool allows you to experiment with jq commands and see the results in real-time. It’s a great way to learn jq and experiment with different commands.
By exploring these resources and practicing your skills, you’ll be well on your way to becoming a jq array master!
Wrapping Up: jq Arrays for JSON Manipulation
In this comprehensive guide, we’ve journeyed through the world of jq, a powerful command-line tool for manipulating JSON data, with a special focus on handling arrays.
We started with the basics, learning how to create, access, and modify arrays using jq. We then ventured into more advanced territory, exploring complex operations like filtering, mapping, and reducing arrays. Along the way, we tackled common challenges you might face when using jq, such as syntax errors and unexpected output, providing you with solutions for each issue.
We also looked at alternative approaches to handling arrays in jq, comparing jq with other command-line tools and third-party libraries. Here’s a quick comparison of these tools:
Tool | Pros | Cons |
---|---|---|
jq | Powerful, flexible, widely used | Can be complex for beginners |
underscore-cli | Simplifies complex tasks, rich feature set | Not as flexible as jq, less community support |
jtc | Rich feature set, good for complex tasks | Less intuitive syntax, less community support |
Whether you’re just starting out with jq or you’re looking to level up your JSON manipulation skills, we hope this guide has given you a deeper understanding of jq and its capabilities for handling arrays.
With its balance of power and flexibility, jq is a valuable tool for anyone dealing with JSON data. Happy data wrangling!