Java’s Math.abs() Function: Usage Guide with Examples
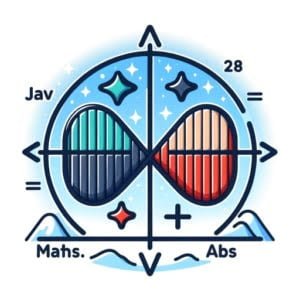
Are you grappling with negative numbers in your Java code? You’re not alone. Many developers find themselves in a bind when dealing with negative numbers, but there’s a function that can turn things around.
Just like a compass always points north, Java’s Math.abs() function always points to the positive. It’s a reliable tool that can help you navigate the sea of numbers in your Java programs, ensuring you always land on positive shores.
This guide will help you understand and use the Math.abs() function effectively in your Java programs. We’ll explore its basic usage, delve into more advanced applications, and even discuss alternative approaches. So, let’s embark on this journey and start mastering Math.abs() in Java!
TL;DR: How Do I Use the math.abs function in Java?
You can use the
Math.abs()
function to get the absolute value of a number in Java, wit the syntaxint a = Math.abs(-1);
. This function returns the absolute value of the argument, effectively eliminating any negative sign.
Here’s a simple example:
int a = Math.abs(-10);
System.out.println(a);
// Output:
// 10
In this example, we’re using the Math.abs() function to get the absolute value of -10. The function returns 10, which is the positive equivalent of -10. We then print the result, which outputs ’10’.
This is just a basic usage of the Math.abs() function in Java. There’s much more to learn about this function, including handling floating-point numbers and large integers. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Math.abs() in Java
- Handling Floating-Point Numbers and Large Integers with Math.abs()
- Exploring Alternatives to Math.abs()
- Troubleshooting Common Issues with Math.abs()
- Understanding the Concept of Absolute Value
- The Importance of Math.abs() in Java
- Math.abs() and Its Relevance in Larger Projects
- Exploring Related Functions: Math.max() and Math.min()
- Wrapping Up: Math.abs()
Understanding the Basics of Math.abs() in Java
The Math.abs() function in Java is a built-in method that returns the absolute value of the given argument. In simpler terms, it converts a negative number into a positive one. This function is a part of the Java Math class and is widely used in mathematical calculations in the programming world.
Let’s take a look at a basic example of how this function works:
int num = -50;
int result = Math.abs(num);
System.out.println("The absolute value is " + result);
// Output:
// The absolute value is 50
In this example, we have a negative number -50
stored in the variable num
. We then use the Math.abs() function to get the absolute value of num
, which is 50
. The function effectively removes the negative sign from -50
, turning it into 50
. We then print the result, which outputs ‘The absolute value is 50’.
This basic usage of the Math.abs() function is particularly useful when you need to perform calculations that require positive numbers, or when you want to compare the magnitudes of numbers without considering their signs.
Handling Floating-Point Numbers and Large Integers with Math.abs()
The Math.abs() function in Java isn’t just limited to basic integers. It can also handle floating-point numbers and large integers, making it a versatile tool for a variety of mathematical operations.
Working with Floating-Point Numbers
Let’s take a look at how Math.abs() handles floating-point numbers:
double num = -15.65;
double result = Math.abs(num);
System.out.println("The absolute value is " + result);
// Output:
// The absolute value is 15.65
In this example, we’re dealing with a negative floating-point number -15.65
. When we pass this number to the Math.abs() function, it returns 15.65
, effectively removing the negative sign. This is particularly useful when you’re working with decimal numbers and need to ensure they’re positive.
Dealing with Large Integers
Math.abs() can also handle large integers effectively. Here’s an example:
long num = -12345678910L;
long result = Math.abs(num);
System.out.println("The absolute value is " + result);
// Output:
// The absolute value is 12345678910
In this scenario, we have a large negative integer -12345678910L
. When we use the Math.abs() function on this number, it returns 12345678910
, once again removing the negative sign. This shows that Math.abs() can handle large numbers just as efficiently as smaller ones.
These examples illustrate the versatility of the Math.abs() function in Java, making it a valuable tool for developers dealing with a wide range of numerical data.
Exploring Alternatives to Math.abs()
While Math.abs() is a powerful tool in Java for dealing with negative numbers, there are other approaches and techniques that can accomplish the same task. Understanding these alternatives can provide you with more flexibility when writing your code.
Using Conditional Statements
One alternative approach is to use a conditional statement to check if a number is negative, and if so, multiply it by -1
to make it positive. Here’s an example:
int num = -20;
if (num < 0) {
num = num * -1;
}
System.out.println("The absolute value is " + num);
// Output:
// The absolute value is 20
In this code, we’re using an if
statement to check if the number is less than zero. If it is, we multiply it by -1
to convert it to a positive number. This approach gives you more control over the code but can be more verbose than using Math.abs().
Utilizing Ternary Operator
Another alternative is to use the ternary operator, which can make your code more concise. Here’s how you can do it:
int num = -30;
num = (num < 0) ? -num : num;
System.out.println("The absolute value is " + num);
// Output:
// The absolute value is 30
In this example, we’re using the ternary operator to check if the number is less than zero. If it is, we multiply it by -1
; otherwise, we leave it as it is. This approach is more compact than using an if
statement but may be less readable for some developers.
While these alternative approaches can accomplish the same task as Math.abs(), they come with their own benefits and drawbacks. Conditional statements provide more control but can be verbose, while the ternary operator is more concise but may be less readable. Depending on the specific needs of your code, you may choose to use these alternatives over Math.abs().
Troubleshooting Common Issues with Math.abs()
While Math.abs() is a straightforward and powerful function in Java, there are a few common issues that you might encounter when using it. Understanding these issues and their solutions can help you write more robust and error-free code.
Handling NaN and Infinity
One common issue occurs when you pass NaN
(Not a Number) or Infinity
to the Math.abs() function. Let’s see what happens:
double num = Double.NaN;
double result = Math.abs(num);
System.out.println("The absolute value is " + result);
// Output:
// The absolute value is NaN
In this example, we’re passing NaN
to the Math.abs() function. The function returns NaN
, which might not be the expected behavior. The same issue occurs when you pass Infinity
to the function.
To avoid this issue, you should always ensure that the number you’re passing to Math.abs() is a valid finite number.
Dealing with Negative Zero
Another issue arises when you pass negative zero (-0.0
) to the Math.abs() function. Here’s what happens:
double num = -0.0;
double result = Math.abs(num);
System.out.println("The absolute value is " + result);
// Output:
// The absolute value is 0.0
In this scenario, we’re passing -0.0
to the Math.abs() function. The function returns 0.0
, effectively removing the negative sign. While this might not be an issue in most cases, it’s something to keep in mind if you’re dealing with operations where the sign of zero matters.
By understanding these common issues with Math.abs(), you can write more robust code and avoid unexpected behavior in your Java programs.
Understanding the Concept of Absolute Value
Before we delve deeper into the usage of Math.abs() in Java, it’s important to understand the fundamental concept it’s based on – the absolute value.
In mathematics, the absolute value of a number is its distance from zero on the number line, regardless of the direction. This means that whether the number is positive or negative, its absolute value is always positive or zero. The absolute value is a critical concept in mathematics and has significant applications in various fields, including programming.
Absolute Value in Programming
In programming, the concept of absolute value is just as crucial. It allows programmers to deal with numerical data without worrying about their sign. This is particularly useful in scenarios where the magnitude of a number is more important than its sign.
For instance, if you’re developing a program to calculate the difference in temperature, the sign of the difference might not matter. Whether it’s +10
degrees or -10
degrees, the magnitude of the change is the same: 10
degrees. This is where the absolute value comes in handy.
The Importance of Math.abs() in Java
In Java, the Math.abs() function is the built-in method used to calculate the absolute value of a number. It’s a part of the Java Math class and is widely used in mathematical calculations in Java programs.
Math.abs() plays a crucial role in handling negative numbers in Java. It allows developers to easily convert negative numbers to positive, simplifying calculations and making the code more readable.
Here’s a simple example:
int num = -15;
int result = Math.abs(num);
System.out.println("The absolute value is " + result);
// Output:
// The absolute value is 15
In this example, we’re using Math.abs() to calculate the absolute value of -15
. The function returns 15
, effectively removing the negative sign. This shows how Math.abs() can be used to handle negative numbers in Java.
In essence, the Math.abs() function in Java brings the concept of absolute value from mathematics into the world of programming, providing developers with a powerful tool to handle numerical data effectively.
Math.abs() and Its Relevance in Larger Projects
The Math.abs() function in Java isn’t just useful for handling individual numbers; it has significant relevance in larger projects and scenarios as well.
For instance, in data analysis projects, you might need to calculate the absolute differences between data points. In game development, you might use it to calculate distances between game objects. And in financial applications, it can be used to calculate absolute returns on investments.
Exploring Related Functions: Math.max() and Math.min()
In addition to Math.abs(), Java provides other useful mathematical functions that often accompany it in typical use cases. Two such functions are Math.max() and Math.min().
Math.max() returns the maximum of two numbers, while Math.min() returns the minimum. These functions can be particularly useful in scenarios where you need to compare numbers or find the highest or lowest value.
Here’s a simple example:
int num1 = 10;
int num2 = 20;
int max = Math.max(num1, num2);
int min = Math.min(num1, num2);
System.out.println("Max: " + max);
System.out.println("Min: " + min);
// Output:
// Max: 20
// Min: 10
In this example, we’re using Math.max() and Math.min() to find the maximum and minimum values, respectively, between 10
and 20
. The functions return 20
and 10
, respectively.
Further Resources for Java Math Functions
If you’re interested in learning more about the Math.abs() function and other related functions in Java, here are some useful resources:
- Tips and Techniques for Using Math Class in Java – Learn how to handle exceptions and edge cases with Java’s Math class.
Using Math.min() Method in Java – Explore the Math.min() method in Java for efficient comparison of numerical values.
Math.max() in Java – Learn how to determine the maximum value among two numbers using Math.max() in Java.
Java Math class documentation includes detailed information about Math.abs() and other functions.
Java Programming tutorials by Oracle cover a wide range of Java topics, including the use of mathematical functions.
Java Math.abs Method Examples by GeeksforGeeks provides thorough insight into the Math.abs method in Java.
Wrapping Up: Math.abs()
In this comprehensive guide, we’ve journeyed through the world of Math.abs() in Java, a powerful function that can turn negative numbers into positive ones.
We began with the basics, learning how to use Math.abs() to get the absolute value of an integer. We then delved into more advanced usage, exploring how Math.abs() can handle floating-point numbers and large integers. We’ve also discussed common issues that you might encounter when using Math.abs(), such as handling NaN
, Infinity
, and negative zero, and provided solutions to help you overcome these challenges.
Moreover, we’ve looked at alternative approaches to getting absolute values, such as using conditional statements and the ternary operator. These alternatives provide more flexibility and control over your code, depending on your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Math.abs() | Simple, supports all number types | May return unexpected results with NaN , Infinity , and -0.0 |
Conditional Statements | More control, can handle special cases | More verbose |
Ternary Operator | More concise | May be less readable for some developers |
Whether you’re just starting out with Math.abs() in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Math.abs() and its capabilities.
With its simplicity and versatility, Math.abs() is a powerful tool for handling negative numbers in Java. Now, you’re well equipped to navigate the sea of numbers in your Java programs. Happy coding!