Using ‘NPM install –Save’ | Easy Project Dependency Setup
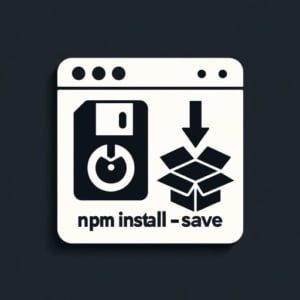
Ever wondered how to keep track of your project’s dependencies efficiently? Like a meticulous librarian, the npm install [package] --save
command helps you organize your project’s library. This article will walk you through using npm install [package] --save
, and how it helps depedency management.
When developing software for IOFLOOD, we ensure our dependency records are consistently accurate by automating the package.json file updates. After learning how to utilize npm install [package] --save
to achieve this, we’ve compiled this together our tips and tricks to aid our bare metal cloud server customers, and other developers, facing similar challenges, on using npm install –save to automatically update the package.json file.
This guide will walk you through the essentials of using npm install –save, a command that not only installs your desired packages but also ensures they’re recorded in your project’s package.json. This is crucial for managing project dependencies effectively, allowing you and your team to have a consistent development environment.
Let’s dive in and simplify dependency management in your Node.js projects with the power of ‘npm install –save’.
TL;DR: What Does npm –save Do?
The
npm install [package] --save
command is used to install a package and save it as a dependency in your project’s package.json file. This ensures that anyone working with your project can install all its dependencies with a single command.
Here’s a quick example:
npm install lodash --save
# Output:
# + [email protected]
# added 1 package in 0.596s
In this example, the npm install lodash --save
command not only installs the lodash library but also adds it to the dependencies list in the package.json file. This is crucial for ensuring that your project remains consistent across different development environments.
Keep reading for more detailed instructions, advanced uses, and troubleshooting tips.
Table of Contents
Basic Use of npm –save
Step-by-Step Dependency Management
When embarking on a Node.js project, one of the first steps is setting up your project’s dependencies. These are the external packages your project needs to function correctly. The npm install [package] --save
command streamlines this process by installing these packages and directly adding them to your project’s package.json
file. Here’s how you can do it:
npm install express --save
# Output:
# + [email protected]
# added 1 package in 1.275s
In this example, we installed express
, a fast, unopinionated, minimalist web framework for Node.js. By using --save
, npm automatically adds express
to the dependencies section of your package.json
file. This is crucial because it ensures that anyone else working on the project can install all necessary packages with a simple npm install
command, fostering a consistent development environment across all team members.
Benefits and Pitfalls
The primary benefit of using npm install [package] --save
is the automatic update of your package.json
file, which helps in maintaining a clear record of all project dependencies. This can significantly simplify project setup for new developers and contribute to smoother project handovers.
However, it’s essential to be mindful of potential pitfalls. One common issue is the inadvertent installation of outdated or incompatible versions of packages. To mitigate this risk, always ensure that you’re installing the latest version of a package or a version that’s known to be compatible with your project.
Advanced npm –save Techniques
Specifying Package Versions
In the world of software development, ensuring that your project uses the correct version of a package is critical for maintaining stability and compatibility. With npm install [package] --save
, you can specify the exact version of a package you wish to install. This is particularly useful when you want to avoid automatically updating to newer versions that might introduce breaking changes. Here’s how you do it:
npm install [email protected] --save
# Output:
# + [email protected]
# added 1 package in 2.674s
In this code block, we specified the version 16.13.1
of the React library. By doing so, we ensure that this specific version is added to our project’s package.json
file under dependencies. This practice is vital for projects that require a specific version of a library to function correctly or to maintain compatibility with other dependencies.
Understanding Semantic Versioning
Semantic versioning, or SemVer, is a versioning scheme that reflects changes in a package with version numbers in the format of MAJOR.MINOR.PATCH
. It’s a system designed to convey meaning about the underlying changes.
- MAJOR version when you make incompatible API changes,
- MINOR version when you add functionality in a backwards-compatible manner, and
- PATCH version when you make backwards-compatible bug fixes.
Understanding SemVer is crucial for managing dependencies effectively, as it helps you make informed decisions about updating packages.
Utilizing –save-exact
For projects where stability is paramount, and you need to lock down the version of a package, npm install [package] --save-exact
is an invaluable option. This command installs the package and records the exact version in your package.json
file without the ^
prefix, which npm uses by default to indicate that minor updates are acceptable.
npm install lodash --save-exact
# Output:
# + [email protected]
# added 1 package in 0.596s
This example demonstrates the installation of lodash
using --save-exact
, ensuring that [email protected]
is the exact version recorded in the package.json
. This approach is recommended for projects where even minor updates could potentially introduce instability or compatibility issues.
By mastering these advanced npm –save options, developers can significantly enhance the stability and reliability of their projects.
Exploring npm –save Alternatives
npm –save-dev for Development Dependencies
While npm install [package] --save
is essential for adding packages to your project’s dependencies, there are scenarios where a package is only needed during development. This is where npm install [package] --save-dev
comes into play. It adds the package to the devDependencies
section of your package.json
, ensuring that your production environment remains lightweight.
npm install eslint --save-dev
# Output:
# + [email protected]
# added 1 package in 1.045s
In this example, we installed eslint
, a tool for identifying and reporting on patterns found in ECMAScript/JavaScript code, with --save-dev
. This command ensures that eslint
is listed under devDependencies
, which is crucial for development but not required in production environments.
npm ci for Clean Installs
For scenarios where you need to ensure a clean, consistent setup, npm ci
comes in handy. This command is especially useful in continuous integration (CI) environments. It disregards the existing node_modules
folder and installs dependencies afresh based on the package.json
and package-lock.json
files, ensuring consistency across installations.
npm ci
# Output:
# added 1050 packages in 10.123s
This command doesn’t directly relate to npm install [package] --save
but is an essential part of managing dependencies in a professional development workflow, ensuring that all developers and the production environment are working with the exact same set of dependencies.
Comparing –save, –save-dev, and –save-optional
Understanding when to use --save
, --save-dev
, and --save-optional
can significantly impact your project’s efficiency and performance. --save-optional
is another variant that adds the package as an optional dependency, which means your project can run without it, but it’s available if needed.
--save
: Adds the package todependencies
, necessary for running the application.--save-dev
: Adds the package todevDependencies
, used only for development.--save-optional
: Adds the package as anoptionalDependency
, not essential but available if needed.
Choosing the right command depends on your project’s specific needs and can make a significant difference in both development and production environments.
Troubleshooting npm –save Issues
Resolving EPERM Errors
One common challenge developers encounter with npm install [package] --save
involves EPERM (Error PERMission) errors. This issue typically occurs on Windows systems and is related to permission conflicts when attempting to modify files or directories.
To resolve EPERM errors, you can try running your command prompt or terminal as an administrator. If the issue persists, clearing the npm cache with npm cache clean --force
can also help. Here’s an example of clearing the cache:
npm cache clean --force
# Output:
# Cache cleaned successfully
After executing this command, attempt to run your npm install [package] --save
command again. Clearing the cache removes any corrupted or incomplete data, potentially resolving the EPERM error.
Handling package.json Conflicts
Conflicts in the package.json
file can arise when multiple developers work on the same project or when merging branches. These conflicts typically occur in the dependencies or scripts sections and can prevent successful package installation.
To avoid package.json
conflicts, regularly commit and push changes to your repository. In case of a conflict, manually review the conflicting lines and decide which version to keep. Tools like Git have built-in mechanisms to help identify and resolve these conflicts.
Managing Version Mismatches
Version mismatches happen when the installed version of a package does not meet the project’s specified requirements. This can lead to runtime errors or unexpected behavior.
An effective way to manage version mismatches is by using the npm list
command to check installed packages against those listed in your package.json
:
npm list
# Output:
# project-name@version
# ├─┬ dependency1@version
# │ └── sub-dependency@version
This command provides a tree view of your project’s dependencies, allowing you to identify discrepancies. If a mismatch is found, consider updating the package version in your package.json
or reinstalling the correct version using npm install [package] --save
with the specific version number.
By understanding and applying these troubleshooting tips and considerations, developers can navigate common issues encountered with npm install [package] --save
and maintain a smooth, efficient workflow.
npm Essentials for Developers
Understanding npm
At its core, npm stands for Node Package Manager. It’s the cornerstone of managing packages in Node.js environments, allowing developers to install, share, and control dependencies in their projects efficiently. npm acts as a conduit between developers and the vast repository of Node.js packages, facilitating easy access to thousands of modules and tools to enhance project development.
The Role of package.json
Every Node.js project begins with a package.json
file. Think of it as the blueprint of your project. It outlines everything from the project’s metadata, scripts, and, most importantly, its dependencies. Dependencies are external packages your project needs to function correctly. Here’s a simple package.json
example:
{
"name": "example-project",
"version": "1.0.0",
"description": "A sample Node.js project",
"main": "index.js",
"dependencies": {
"express": "^4.17.1"
}
}
In this snippet, the package.json
file specifies that our project depends on the express
package. The versioning follows semantic versioning, indicated by the ^
, allowing minor updates but not major ones that could break compatibility.
npm Commands Impact
Understanding npm commands and their impact on project management is crucial. For instance, when you run an npm install
command, npm looks at the package.json
file and installs the versions of the packages listed under dependencies. This ensures that anyone working on the project can set up their development environment with the correct versions of each package, thus maintaining consistency across the board.
The npm install [package] --save
command specifically, when used during package installation, automatically updates the package.json
file, adding the new package to the list of dependencies. This automatic update is vital for keeping track of which packages and versions your project depends on, ensuring that all developers are working with the same set of tools.
By delving into these fundamentals, developers gain a solid foundation for navigating and utilizing npm effectively, ensuring efficient project setup and management.
npm –save in the Ecosystem
The Bigger Picture of npm Commands
The npm install [package] --save
command is a fundamental part of the npm ecosystem, but it’s just one piece of the puzzle. Understanding how it fits within the broader context of npm commands can significantly enhance your Node.js development practices.
Keeping Up with Package Versioning
Package versioning is crucial in software development. The npm update
command helps you keep your dependencies up to date. Unlike npm install [package] --save
which adds new dependencies, npm update
checks for newer versions of the packages you already have and updates them accordingly.
npm update lodash
# Output:
# + [email protected] updated 1 package in 0.596s
This command updates the lodash
package to its latest version, ensuring your project benefits from the latest features and bug fixes. It’s an essential command for maintaining project health.
npm Audit for Security
Security is a paramount concern in development. npm audit
scans your project for vulnerabilities and suggests fixes. It’s a proactive measure to safeguard your project against potential security threats.
npm audit
# Output:
# found 0 vulnerabilities
# in 1050 scanned packages
The npm audit
command provides a security report, highlighting any vulnerabilities found in your project’s dependencies. It’s an invaluable tool for maintaining the integrity of your project.
Further Resources for npm –save Mastery
To deepen your understanding of npm install [package] --save
and its place within Node.js development, here are three recommended resources:
- npm Documentation: The official npm documentation is an exhaustive resource covering all npm commands, including
npm install [package] --save
,npm update
, andnpm audit
. Node.js Guides: These guides provide insights into Node.js itself, helping you understand the environment in which npm operates.
The npm Blog: For the latest updates, features, and best practices in npm, the official npm blog is a valuable resource.
By exploring these resources, you can enhance your proficiency with npm install [package] --save
and stay abreast of the latest developments in Node.js and npm.
Recap: npm –save Usage Guide
In this comprehensive guide, we’ve explored the pivotal role of the npm install [package] --save
command in managing project dependencies efficiently. This command not only installs the desired packages but also ensures they are accurately listed in your project’s package.json
, fostering a consistent development environment.
We began with the basics, showing how npm install [package] --save
simplifies adding dependencies to your project. We then navigated through advanced usage, including specifying package versions and understanding semantic versioning, to maintain project stability.
Further, we delved into alternative approaches like npm install [package] --save-dev
for development dependencies and npm ci
for clean slate installations. These techniques offer flexibility in managing different types of dependencies and ensure that your project setup remains clean and consistent.
Command | Usage | Benefit |
---|---|---|
npm install [package] --save | Install and add to dependencies | Ensures project consistency |
npm install [package] --save-dev | Install and add to devDependencies | Keeps production light |
npm ci | Clean install based on package-lock.json | Guarantees consistency across environments |
Whether you’re just starting out with npm or looking to refine your dependency management strategy, we hope this guide has equipped you with the knowledge to use npm install [package] --save
effectively.
The ability to manage dependencies accurately is a cornerstone of modern software development. With the insights from this guide, you’re now better prepared to ensure your Node.js projects are as dependable and up-to-date as possible. Happy coding!