npm Lodash | JavaScript Developer’s Utility Library Guide
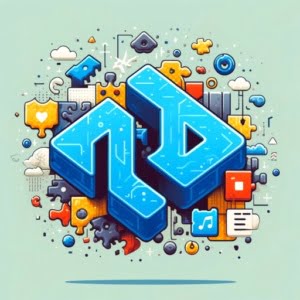
Diving into JavaScript projects but feeling overwhelmed by data manipulation? You’re not alone. Like a Swiss Army knife for developers, Lodash provides a treasure trove of utilities to streamline your code, making tasks that once seemed complex or tedious, manageable and efficient.
When developing software for IOFLOOD, we’ve discovered that incorporating Lodash into npm projects offers simplified data manipulation and improved code maintainability. By sharing our tips and tricks, we aim to empower developers and customers utilizing our dedicated servers to use Lodash effectively in their own projects.
This guide will walk you through integrating Lodash into your npm projects, making your JavaScript journey smoother and more efficient. Whether you’re dealing with arrays, objects, strings, or any other data type, Lodash has a function to simplify the process. By the end of this guide, you’ll see why Lodash is an indispensable tool in modern JavaScript development.
Let’s dive in and learn how to integrate the Lodash utility library into your npm projects.
TL;DR: How Do I Use Lodash in an npm Project?
To kickstart your journey with Lodash in an npm project, begin by installing Lodash using the command
npm install lodash
. Following installation, you can incorporate Lodash into your project by requiring it in your files. For a broad inclusion, useconst _ = require('lodash');
, or, to import specific functions, opt for a more targeted approach likeconst { map } = require('lodash');
.
Here’s a quick example to demonstrate how you might use the map
function to iterate over an array of numbers and double their value:
const _ = require('lodash');
const numbers = [1, 2, 3, 4];
const doubled = _.map(numbers, (number) => number * 2);
console.log(doubled);
# Output:
# [2, 4, 6, 8]
In this snippet, we import Lodash and use its map
function to apply a transformation to each element in the numbers
array, effectively doubling their values. This example showcases the simplicity and power of using Lodash for common data manipulation tasks.
Eager to streamline your JavaScript projects with Lodash? Keep reading for a comprehensive guide on leveraging this utility library to enhance your coding efficiency and simplify complex tasks.
Table of Contents
Simplify Data with Lodash
Embarking on your JavaScript project with npm and Lodash? Let’s start with the basics. The first step is to integrate Lodash into your project, which begins with its installation. Using npm, the Node.js package manager, you can easily add Lodash to your project with a simple command.
Installing Lodash
To install Lodash, open your terminal and run:
npm install lodash
This command fetches the Lodash library from the npm repository and adds it to your project, making its powerful utilities available for use.
Importing Lodash into Your Project
Once Lodash is installed, you’ll need to require it in your JavaScript files to use its functions. Here’s how you can import the entire Lodash library:
const _ = require('lodash');
Utilizing Lodash Functions
With Lodash imported, let’s demonstrate its power with a basic example. Suppose you want to find the maximum number in an array. Instead of writing complex logic, you can use Lodash’s _.max
function:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const maxNumber = _.max(numbers);
console.log(maxNumber);
# Output:
# 5
In this code block, we import Lodash and use its max
function to effortlessly find the highest number in the array. This example underscores Lodash’s ability to simplify data manipulation, allowing you to focus more on the logic of your application rather than on implementing common tasks.
Pros and Cons
While Lodash offers a plethora of functions that simplify data manipulation, it’s important to be mindful of its impact on your project’s bundle size. If Lodash is not tree-shaken properly, it can significantly increase the size of your application’s bundle, affecting load times and performance. However, with proper management and selective imports, you can leverage Lodash’s capabilities without the drawbacks.
Advanced Lodash: Chainability
As you become more familiar with Lodash in your npm projects, you’ll discover the power of its chainability feature. This allows you to perform complex data manipulation in a more elegant and readable manner. But what exactly does chainability mean in the context of Lodash, and how can it transform your coding approach?
Understanding Chainability
Chainability refers to the ability to link multiple function calls together in a single statement, passing the result of one function as the input to the next. Lodash makes this possible with the _.chain()
function, which wraps your data, allowing you to apply several transformations in a sequence.
Chainability in Action
Let’s say you have an array of user objects and you want to perform multiple operations: filter out inactive users, pick specific properties from each user, and then sort them by their age. Here’s how you can achieve this using Lodash’s chainability:
const _ = require('lodash');
const users = [
{ 'user': 'fred', 'age': 48, 'active': false },
{ 'user': 'barney', 'age': 34, 'active': true },
{ 'user': 'pebbles', 'age': 40, 'active': false }
];
const result = _.chain(users)
.filter('active')
.orderBy(['age'], ['asc'])
.map('user')
.value();
console.log(result);
# Output:
# ['barney']
In this example, we start by wrapping our users
array with _.chain()
, then sequentially apply filter
, orderBy
, and map
operations before finally extracting the result with .value()
. The output is the name of the active user sorted by age, showcasing Lodash’s ability to handle complex data manipulations with ease.
Pros and Cons of Advanced Use
The chainability feature of Lodash is both powerful and flexible, allowing you to write more concise and readable code. However, it’s worth noting that for simple tasks, this approach can be overkill and may introduce unnecessary complexity into your code. Additionally, while chainability is a powerful feature, it’s essential to be mindful of its impact on performance and bundle size. Properly tree-shaking Lodash during your build process can help mitigate these concerns, ensuring that only the parts of the library you use are included in your final bundle.
Comparing Lodash vs. Alternatives
When embarking on a JavaScript project, one critical decision is selecting the right utility library. While npm lodash
is a popular choice, it’s essential to weigh it against alternatives like Underscore.js and native JavaScript ES6+ methods. Understanding when to opt for Lodash or pivot to another solution can significantly impact your project’s efficiency and performance.
Lodash and Underscore.js
At first glance, Lodash and Underscore.js appear remarkably similar, offering a range of utilities for common programming tasks. However, Lodash often comes out ahead in terms of performance optimizations and additional features.
Consider a scenario where you need to deep clone an object. Lodash provides an intuitive _.cloneDeep
method, whereas with Underscore.js, you might have to implement a custom solution or rely on a less efficient approach.
const _ = require('lodash');
const object = { 'a': 1, 'b': { 'c': 2 } };
const deepClone = _.cloneDeep(object);
console.log(deepClone);
# Output:
# { 'a': 1, 'b': { 'c': 2 } }
This code block demonstrates how Lodash’s _.cloneDeep
method easily creates a deep copy of an object, preserving nested structures. Such a task is more cumbersome with Underscore.js or native methods, showcasing Lodash’s advantage for complex operations.
Native JavaScript ES6+
With the evolution of JavaScript, many functions previously requiring utility libraries can now be accomplished using native ES6+ features. For example, array manipulation tasks like mapping, filtering, and reducing have native implementations that are both efficient and straightforward.
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(number => number * 2);
console.log(doubled);
# Output:
# [2, 4, 6, 8]
This example showcases the use of the native map
function to double the values in an array. For projects prioritizing modern JavaScript syntax and minimal dependencies, leveraging native ES6+ features for such tasks might be preferable.
Making the Decision
Choosing between Lodash, Underscore.js, and native JavaScript depends on several factors, including project requirements, performance considerations, and team familiarity with the libraries. Lodash is an excellent choice for complex data manipulations and scenarios where performance optimizations are critical. Meanwhile, for projects that can rely on modern browser support and aim for minimal dependency footprints, native JavaScript ES6+ methods offer a compelling alternative.
In summary, while npm lodash
remains a powerful tool in a developer’s arsenal, understanding the landscape of utility libraries and native JavaScript features is crucial for making informed decisions tailored to your project’s needs.
While integrating npm lodash
into your projects can significantly enhance your development experience, there are common pitfalls that you might encounter. Understanding these issues and knowing how to address them is crucial for maintaining the efficiency and reliability of your applications.
Version Conflicts
One of the challenges with using Lodash, or any npm package, is managing version conflicts. It’s possible that different parts of your project or its dependencies require different versions of Lodash, leading to compatibility issues.
To ensure consistency, you can lock the Lodash version in your package.json
file:
"dependencies": {
"lodash": "4.17.21"
}
Specifying a version number helps prevent unexpected updates that could introduce breaking changes or conflicts.
Improper Import Statements
Another common issue is improper import statements, which can lead to modules not being found or unexpected behavior in your code. For example, when importing a specific function from Lodash, ensure you’re using the correct syntax.
Here’s the right way to import the find
function:
const find = require('lodash/find');
This import statement ensures that you’re only importing the find
function, which can help reduce the bundle size of your application.
Bundle Size Concerns
Speaking of bundle size, indiscriminate use of Lodash can lead to bloated application bundles, especially if you’re importing the entire library when only a few functions are needed. To mitigate this, consider importing only the specific functions you need.
For instance, instead of importing the whole Lodash library, you can import just the debounce
function like this:
const debounce = require('lodash/debounce');
This approach significantly reduces the impact on your bundle size, as you’re only including the code necessary for the functions you use.
Best Practices and Optimization Tips
To optimize your use of Lodash in npm projects, follow these best practices:
- Use tree shaking to remove unused code from your bundle, especially if using webpack or another module bundler.
- Regularly audit your project’s dependencies to identify opportunities to reduce the overall size.
- Consider using native JavaScript alternatives for simpler tasks, reserving Lodash for more complex or specialized operations.
By being mindful of these considerations and employing strategic practices, you can leverage the power of npm lodash
without compromising on performance or maintainability.
The Evolution of JavaScript Utilities
The JavaScript ecosystem has evolved significantly over the years, with utility libraries playing a pivotal role in this transformation. Among these, Lodash has emerged as a cornerstone, offering developers an extensive toolkit for handling data manipulation, iteration, and much more. But what sets Lodash apart, and why does it continue to be a go-to choice in the npm realm?
npm: The Catalyst for JavaScript Innovation
npm, standing for Node Package Manager, has been instrumental in the evolution of JavaScript development. It serves as a repository for open-source JavaScript projects, facilitating easy sharing and integration of code. The command npm install lodash
is more than just a way to add Lodash to your project; it symbolizes the ease with which developers can leverage community-driven solutions to enhance their applications.
Consider the following example where we use Lodash to perform a deep comparison between two objects:
const _ = require('lodash');
const object = { 'a': 1 };
const other = { 'a': 1 };
const areEqual = _.isEqual(object, other);
console.log(areEqual);
# Output:
# true
In this code snippet, we use _.isEqual
to check if two objects are deeply equal, a task that can be verbose and error-prone if done with native JavaScript alone. This example illustrates the simplicity and power of Lodash, enabling developers to write more robust and maintainable code.
Why Lodash Stands Out
Lodash’s enduring popularity stems from its commitment to performance, reliability, and a comprehensive suite of functions that cater to a wide range of programming needs. Its functions are meticulously optimized for speed and cover edge cases that might not be immediately obvious, ensuring developers can work confidently with complex data structures and algorithms.
Furthermore, Lodash’s modular design means that developers can import only the functions they need, significantly reducing the impact on bundle size—a crucial consideration in web development. This thoughtful approach to library design, combined with the ease of integration via npm, cements Lodash’s role as an essential tool in the modern JavaScript developer’s toolkit.
In conclusion, the synergy between Lodash and npm exemplifies the dynamic nature of JavaScript development. As the landscape continues to evolve, Lodash remains at the forefront, offering solutions that are both practical and powerful, making it an invaluable resource for developers seeking to navigate the complexities of modern web development.
Expanding Lodash’s Horizons
As your journey with npm lodash
progresses, you’ll find its utility extends far beyond basic array and object manipulations. Lodash’s versatility shines in larger-scale applications, server-side development with Node.js, and when used alongside popular frameworks like React. Let’s explore how Lodash can elevate these advanced scenarios, providing efficiency and elegance to your codebase.
Lodash in Large-Scale Applications
In the context of large-scale applications, managing data becomes increasingly complex. Lodash’s ability to simplify and streamline data manipulation makes it an invaluable asset. For instance, consider the task of filtering a large dataset based on multiple conditions:
const _ = require('lodash');
const users = [
{ 'user': 'barney', 'age': 36, 'active': true },
{ 'user': 'fred', 'age': 40, 'active': false },
{ 'user': 'pebbles', 'age': 1, 'active': true }
];
const activeUsers = _.filter(users, {'active': true, 'age': { '$gt': 30 }});
console.log(activeUsers);
# Output:
# [{ 'user': 'barney', 'age': 36, 'active': true }]
This code snippet demonstrates how Lodash can efficiently filter a dataset based on complex criteria, a common requirement in large-scale applications.
Lodash on the Server Side with Node.js
Lodash’s utility extends to server-side development with Node.js, where its functions can enhance backend logic. For example, debouncing function calls can be crucial in handling high-frequency events, such as API requests:
const _ = require('lodash');
const expensiveCall = _.debounce(() => {
// Perform an expensive operation
}, 1000);
// Simulated high-frequency call
setInterval(expensiveCall, 50);
In this example, _.debounce
ensures that the expensive operation is not called more often than once per second, demonstrating Lodash’s capability to optimize server-side operations.
Enhancing React Development with Lodash
When developing React applications, Lodash can simplify state management and data transformations. Its functions can be particularly useful in handling complex state objects or arrays. Integrating Lodash with React enhances readability and maintainability of the code, allowing for more elegant data manipulation patterns.
Further Resources for Mastering Lodash
To deepen your understanding of Lodash and explore its full potential, consider the following resources:
- Lodash Official Documentation – Comprehensive resource for a deep understanding of Lodash methods and their usage.
Lodash on GitHub – Source code, issue tracking, and latest updates of the Lodash library.
Lodash Cookbook on Leanpub – Practical guide and hands-on examples on how to effectively use Lodash in real-world scenarios.
These resources will provide you with a broader perspective on Lodash’s capabilities and inspire you to leverage its full power in your projects.
Recap: Lodash for npm Projects
In this comprehensive guide, we’ve navigated through the process of integrating Lodash into your npm projects, from the simplicity of adding it to your project to leveraging its vast array of utilities for more efficient and readable code.
We began with the basics, illustrating how to install Lodash using npm and how to import it or specific functions into your project. This foundational knowledge sets the stage for utilizing Lodash to simplify data manipulation tasks, making your JavaScript journey smoother and more efficient.
Moving to more advanced usage, we explored Lodash’s chainability feature, which allows for elegant and complex data manipulation with minimal code. We also discussed the importance of tree-shaking in optimizing your project’s bundle size, ensuring that Lodash enhances your project without unnecessary bloat.
In considering alternative approaches, we compared Lodash with other utility libraries like Underscore.js and native JavaScript ES6+ methods. This comparison highlighted situations where Lodash’s performance optimizations and additional features make it the preferred choice, as well as scenarios where native methods or other libraries might be more appropriate.
Feature | Lodash | Native JavaScript | Underscore.js |
---|---|---|---|
Performance Optimizations | High | Moderate | Moderate |
Feature Set | Extensive | Growing with ES6+ | Broad |
Use Case Flexibility | High | Varies with ES version | Moderate |
Whether you’re just starting out with Lodash in your npm projects or you’re looking to deepen your understanding of its capabilities, we hope this guide has provided you with valuable insights. Lodash remains a potent tool in a developer’s toolkit, simplifying complex tasks and enhancing code readability and maintainability.
With its comprehensive suite of utilities and the ability to integrate seamlessly into your npm projects, Lodash empowers developers to write more efficient and effective JavaScript code. Embrace the power of Lodash and unlock new levels of productivity in your development projects.