The NPM Registry | Node.js Package Docs, Tips and Tricks
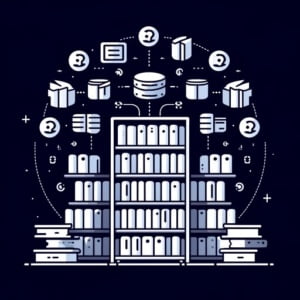
While testing Node.js packages for use at IOFlood, I’ve become familiar with utilizing the npm registry. Understanding the nuances of this essential tool ensures our projects are properly integrated and efficiently managed. To help others facing similar challenges, I have gathered all of my notes into this reference guide.
This guide serves as your map to understanding and utilizing the npm registry, an essential tool for any JavaScript developer. From the basics of finding and installing packages to mastering advanced features, we’ll cover everything you need to navigate this vast resource confidently.
Let’s embark on this journey together, unlocking the full potential of the npm registry to supercharge your development workflow.
TL;DR: What is the npm registry?
The npm registry is a public collection of packages of open-source code for Node.js, front-end web apps, mobile apps, routers, and other needs of the JavaScript community. In a standard installation of npm, the default registry is set to https://registry.npmjs.org/. You can search the registry for apps with,
npm search package-name
and install them withnpm install package-name
.
Here’s a quick example on how to install from the registry:
npm install lodash
# Output:
# + [email protected]
# added 1 package in 0.752s
In this simple example, we use the npm install
command to add the lodash package, a utility library that provides modular methods to improve the efficiency of JavaScript code, to our project. This command fetches the package from the npm registry and adds it to the project’s dependencies, showcasing the registry’s role as a vast repository of JavaScript tools and libraries.
Ready to dive deeper? Continue reading for a more comprehensive exploration of the npm registry, including advanced uses and troubleshooting tips.
Table of Contents
Getting Started with npm
Finding and Installing Packages
The npm registry is a treasure trove for developers, acting as a central hub for open-source packages that can significantly enhance your projects. Understanding how to effectively search for and install these packages is the first step in leveraging the power of npm.
Searching for a Package
Finding the right package can sometimes feel like looking for a needle in a haystack, but with npm’s search functionality, it’s more like using a highly sophisticated metal detector. Let’s look at how to search for a package directly from the command line:
npm search express
# Output:
# NAME | DESCRIPTION | AUTHOR | DATE | VERSION | KEYWORDS
# express | Fast, unopinionated, | TJ Holowaychuk | 2020-06-05 | 4.17.1 | web framework, express
In this example, we used the npm search
command to look for the Express framework, a popular choice for building web applications. The output provides a concise summary of the package, including its description, author, version, and keywords, making it easier to decide if it’s the right fit for your project.
Installing a Package
Once you’ve found a package that meets your needs, installing it is straightforward. Here’s how you can add a package to your project:
npm install moment
# Output:
# + [email protected]
# added 1 package in 1.245s
In this code block, we demonstrate installing the ‘moment’ package, a widely used library for parsing, validating, manipulating, and displaying dates and times in JavaScript. This simple command fetches the package from the npm registry and integrates it into your project, showcasing the registry’s role as an indispensable resource for developers.
Understanding these basic commands opens up a world of possibilities, allowing you to easily incorporate a vast array of tools and libraries into your projects.
Elevating Your npm Skills
Publishing Your Own Package
Once you’ve mastered finding and installing packages from the npm registry, the next step in your npm journey could involve contributing back to the community by publishing your own package. This process turns your solutions into accessible tools for developers worldwide, fostering collaboration and innovation.
Creating a New Package
Before you can publish, you need to create a package. Ensure you have a package.json file in your project, which npm uses to manage your project’s dependencies and scripts among other configurations.
npm init
# Output:
# This utility will walk you through creating a package.json file.
This command initializes a new npm package in your project directory, prompting you to enter details such as the package’s name, version, and description. It’s the first step towards making your project publishable on the npm registry.
Publishing to npm
After setting up your package, publishing it to the npm registry is straightforward. Ensure you’re logged into your npm account and use the following command:
npm publish
# Output:
# + [email protected]
This command uploads your package to the npm registry, making it available for installation by millions of developers. It’s a rewarding step that contributes to the open-source community, allowing others to benefit from your work.
Managing Package Versions
Proper version management is crucial for the ongoing success of your package. npm adheres to semantic versioning, or semver, which helps developers understand the impact of updates at a glance.
Updating Your Package
When you make improvements or fixes to your package, updating its version before publishing is essential. Use the npm version
command to bump up your package version according to semver rules:
npm version patch
# Output:
# v1.0.1
This command increments the patch version of your package, indicating that the changes are backward-compatible bug fixes. It’s an important practice that keeps the npm ecosystem stable and reliable for users.
Setting Up a Private npm Registry
For organizations looking to manage their internal packages securely, setting up a private npm registry is a valuable option. It allows you to control package access, ensuring that only authorized users can install or publish packages.
While npm offers private packages and organizations for this purpose, third-party services and self-hosted solutions also provide similar functionalities. Choosing the right approach depends on your organization’s specific needs and resources.
Through advanced use of the npm registry, developers can significantly enhance their workflows, contribute to the open-source community, and manage internal resources more effectively. These practices not only improve your projects but also support the growth and security of the broader JavaScript ecosystem.
Exploring Beyond npm
Yarn: A Strong Contender
While npm is the go-to registry for many developers, Yarn has emerged as a powerful alternative, especially in scenarios requiring faster package installations and improved reliability. Both npm and Yarn serve the JavaScript community by managing project dependencies, but they do so with slightly different approaches.
Yarn vs. npm: Performance and Features
Yarn introduced the concept of a lock file from the beginning, which ensured that an installed package would remain the same on every machine. npm later introduced a similar feature with package-lock.json
. Here’s how you can start a new project with Yarn:
yarn init
# Output:
# question name (your-project-name):
This command initializes a new project, similar to npm init
. The interactive session will ask you for project details, mirroring npm’s approach but with Yarn’s unique features.
Yarn’s cache mechanism and parallel package downloading significantly speed up the installation process, a boon for developers working on large projects or with limited internet bandwidth.
Transitioning Between Registries
Switching between npm and Yarn, or vice versa, is straightforward, thanks to the interoperability efforts from both teams. If you’re considering a switch, or need to use both for different projects, understanding how to transition smoothly is vital.
Migrating to Yarn from npm
If you decide to switch to Yarn, migrating your project is a simple process. Start by installing Yarn globally on your system. Then, in your project directory, run the following command to create a yarn.lock
file from your existing package-lock.json
or npm-shrinkwrap.json
file:
yarn import
# Output:
# [1/4] Resolving packages...
# [2/4] Fetching packages...
# Success!
This command analyzes your npm lockfile and generates an equivalent yarn.lock
file, ensuring that your dependencies remain consistent. It’s a crucial step for maintaining project stability during the transition.
Exploring alternative package managers and registries like Yarn offers a broader perspective on managing project dependencies. Each tool has its strengths, and understanding these can help you choose the right one for your project’s needs. Whether you stick with npm or venture into using Yarn, the goal is to optimize your development workflow for efficiency and reliability.
Authentication Errors
Authentication errors can be a common stumbling block when using the npm registry, especially when trying to publish a package or access private packages. These errors typically indicate a problem with your npm login credentials or token.
To resolve authentication issues, first, ensure you’re logged into npm with the correct account. You can reauthenticate using the following command:
npm login
# Output:
# Username: (your-username)
# Password: (your-password)
# Email: (your-email)
This command prompts you for your npm account details, effectively resetting your login session. Successful authentication is crucial for package publishing and accessing restricted packages, making this a vital step in troubleshooting.
Package Not Found Errors
Encountering a ‘package not found’ error can be frustrating. This error often occurs if the package name is misspelled or if the package has been deprecated and removed from the registry.
To verify the existence of a package, you can search the npm registry directly from your terminal:
npm search package-name
# Output:
# NAME | DESCRIPTION | AUTHOR | DATE | VERSION | KEYWORDS
If the package appears in the search results, double-check the spelling in your npm install
command. If it doesn’t appear, the package may no longer be available, and you might need to look for an alternative.
Optimizing npm Registry Use
Maximizing the efficiency of interacting with the npm registry can significantly enhance your development workflow. One way to achieve this is by configuring npm to save exact versions of packages. This practice can help prevent unexpected changes due to version updates in dependencies.
To configure npm to save exact versions, use the following command:
npm config set save-exact=true
This command adjusts your npm configuration to ensure that when you install a package, npm records the exact version in your package.json
file. It’s a simple yet effective way to maintain consistency and reliability across development environments.
Navigating the npm registry’s potential pitfalls with the right knowledge and tools can transform these challenges into opportunities for learning and growth. By understanding how to troubleshoot common issues and optimize your use of the registry, you can streamline your development process and focus on creating impactful JavaScript applications.
Overview of the npm Registry
The Birth of npm
The npm registry, standing for Node Package Manager, began as a conversation starter among developers looking for a streamlined way to share code modules in the early days of Node.js. It quickly evolved from a simple package manager into a comprehensive registry, hosting a vast array of JavaScript libraries and applications.
The Early Days
When Node.js was first introduced, it brought along the challenge of managing dependencies in a growing ecosystem. The npm registry was created in 2010 to address this need, providing a centralized repository for Node.js packages. Here’s a glimpse into adding a package to your project in the early days of npm:
npm install request
# Output:
# + [email protected]
# added 1 package in 2.5s
In this example, the npm install
command adds the ‘request’ package, a popular HTTP client, to the project. This command fetches the package from the npm registry and adds it to the project’s dependencies, showcasing the registry’s initial role as a simple yet powerful tool for developers.
npm’s Evolution
Over the years, the npm registry has grown exponentially, both in terms of the number of packages available and its features. It has introduced features such as package version management, private packages, and organizational management tools, significantly expanding its capabilities.
The Growth Phase
The npm registry’s growth mirrored the JavaScript ecosystem’s expansion, becoming an indispensable tool for developers. The introduction of npm install --save
in later versions made it easier to manage project dependencies:
npm install lodash --save
# Output:
# + [email protected]
# added 1 package from 1 contributor and audited 1 package in 1.752s
This command not only installs the lodash library but also adds it to the project’s package.json
file under dependencies, illustrating the registry’s evolution into a more sophisticated package management system.
The npm Registry Today
Today, the npm registry is more than just a repository for JavaScript packages. It’s a comprehensive ecosystem supporting the JavaScript community’s needs, from open-source projects to large-scale enterprise applications. Its role in facilitating code sharing and collaboration has cemented its place as a cornerstone of modern web development.
Understanding the npm registry’s history and evolution provides valuable context for its current capabilities and importance. It highlights the registry’s foundational role in the JavaScript ecosystem and its continuous adaptation to meet developers’ growing needs.
Modern Usage of npm Registry
Integrating npm with CI/CD Pipelines
The npm registry plays a pivotal role in automating and enhancing development workflows, especially when integrated with Continuous Integration/Continuous Deployment (CI/CD) pipelines. This integration ensures that every code commit is automatically tested and, if the tests pass, deployed, making npm packages an integral part of the development, testing, and deployment phases.
Consider this example of a CI pipeline step that installs dependencies from the npm registry before running tests:
npm install && npm test
# Output:
# audited 100 packages in 2s
# found 0 vulnerabilities
# Running tests...
# Tests passed!
In this scenario, npm install
fetches the necessary packages from the npm registry, ensuring the application has all its dependencies. Following this, npm test
runs the project’s tests. The output indicates a successful dependency audit and test pass, showcasing the seamless integration of npm into CI/CD pipelines. This automation not only streamlines the development process but also enhances code quality and deployment reliability.
npm’s Role in Software Development Practices
The npm registry has significantly influenced software development practices by promoting code reuse and sharing. It has fostered a culture of collaboration within the JavaScript community, allowing developers to build upon each other’s work, leading to faster development cycles and more robust applications.
Further Resources for npm Registry Mastery
To deepen your understanding of the npm registry and how to leverage it in your projects, consider exploring the following resources:
- npm Documentation: The official npm documentation provides comprehensive guides on using npm, from basic commands to advanced features.
Node.js Guides: These guides offer insights into Node.js and npm, covering various topics that cater to both beginners and experienced developers.
The npm Blog: Stay updated with the latest news, updates, and insights from the npm team, covering everything from security advisories to feature announcements.
Exploring these resources will not only enhance your skills but also keep you informed about the latest trends and best practices in using the npm registry. Whether you’re a beginner or an experienced developer, there’s always something new to learn in the ever-evolving JavaScript ecosystem.
Recap: npm Registry Reference Guide
In this comprehensive guide, we’ve ventured through the intricate world of the npm registry, an indispensable tool for JavaScript developers. From the fundamentals of finding and installing packages to the nuances of publishing and managing them, the npm registry stands as a pivotal point in modern web development.
We began with the basics, illustrating how to search for and install packages, a fundamental skill for any developer. This initial step opens the door to a vast repository of tools and libraries, ready to be leveraged in your projects.
Moving forward, we delved into the realm of publishing your packages, a significant milestone for contributing back to the community. We explored how to manage package versions and set up a private npm registry, essential practices for maintaining project integrity and security.
We also navigated through alternative package managers like Yarn, offering insights into the broader ecosystem and how to smoothly transition between different tools without disrupting your workflow.
Feature | npm | Yarn |
---|---|---|
Package Installation Speed | Moderate | Fast |
Lockfile | package-lock.json | yarn.lock |
Parallel Installation | No | Yes |
In wrapping up, we addressed common troubleshooting issues and optimization techniques, ensuring you’re well-equipped to tackle any challenges that arise. The npm registry, with its comprehensive features and vast repository, is more than just a package manager; it’s a catalyst for innovation and collaboration in the JavaScript community.
Whether you’re a beginner looking to grasp the basics or an experienced developer aiming to refine your mastery of npm, this guide serves as a valuable resource. The npm registry’s role in streamlining development processes and fostering community contributions cannot be overstated. Happy coding!