Running npm Build Scripts | Node.JS Developer’s Guide
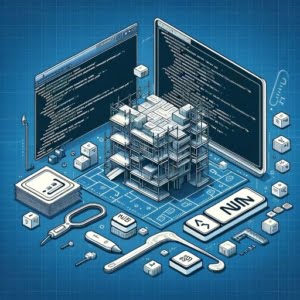
While learning to utilize npm to maintain the Node.js projects here at IOFLOOD, it was challenging to understand the ‘npm run build’ command. However, through testing and research, I’ve gained valuable insights into its role in optimizing project builds. Now, equipped with this knowledge, I’m excited to share our experiences and guide others on how to use npm run build.
This guide will not only explain the ‘npm run build’ command but also provide you with the knowledge to wield it like a pro. We’ll explore its basic usage, tackle more complex scenarios, and even touch upon alternative tools and commands for those looking to expand their development toolkit. So, whether you’re a beginner looking to understand the basics or an experienced developer aiming to refine your build process, you’re in the right place.
Let’s roll up our sleeves and dive into the world of project optimization with ‘npm run build’ together!
TL;DR: What Does ‘npm run build’ Do?
npm run build
executes the ‘build’ script defined in your project’s package.json, preparing the project for production by bundling assets and performing other build steps. To ensure a fresh build use the commandnpm run clean
before the build script to clean the build directory.
Here’s a quick example:
"scripts": {
"build": "webpack --mode production"
}
# Output:
# Successfully created a production build.
In this example, the npm run build
command triggers the build
script in package.json
, which in turn runs webpack --mode production
. This command tells Webpack to bundle your project’s assets for production, optimizing them for speed and efficiency.
This is just the tip of the iceberg. Continue reading for a deeper dive into how to leverage ‘npm run build’ for your projects, including more complex scenarios and troubleshooting common issues.
Table of Contents
First Steps with ‘npm run build’
Embarking on your journey with Node.js and JavaScript, one of the first spells you’ll need to master is the ‘npm run build’ command. This command is your gateway to preparing your project for the grand unveiling – the production stage. Let’s walk through the process step by step, ensuring you have a solid foundation.
Setting Up Your Build Script
Before you can conjure the magic of ‘npm run build’, you need to define a ‘build’ script in your project’s package.json
. This script is where you specify the commands that package your application, optimize it for production, or any other tasks you need to prepare your project. Here’s a simple example to get you started:
"scripts": {
"build": "echo 'Building for production...'
}
In this example, the build
script simply echoes a message. While not particularly useful for real-world applications, it’s a perfect first step to understanding how scripts are run.
Running Your First Build
With your script in place, it’s time to run it. Open your terminal, navigate to your project directory, and execute:
npm run build
# Output:
# > echo 'Building for production...'
# Building for production...
This command triggers the ‘build’ script defined in your package.json
. The output shows the message we specified, indicating the script has run successfully. While this example is simple, it’s a crucial step in understanding the workflow of ‘npm run build’.
Understanding the Outcome
Executing ‘npm run build’ with our simple script demonstrates how npm scripts are triggered and provides a glimpse into the potential of what can be achieved with more complex scripts. As you progress, you’ll replace the echo command with commands that bundle assets, minify code, and perform other essential build steps. The ‘npm run build’ command is a powerful tool in your development toolkit, and mastering it is key to deploying efficient, optimized applications.
Elevate Your Build Process
As you grow more comfortable with the basics of ‘npm run build’, it’s time to explore how this command can be tailored and expanded to fit more complex development needs. By integrating with tools like Webpack and Babel, and utilizing additional flags, you can significantly enhance your build process.
Integrating Webpack
Webpack is a powerful module bundler that can transform, bundle, or package just about any resource or asset. When combined with ‘npm run build’, it can automate and streamline the process of preparing your application for production. Here’s an example of a more sophisticated build script in package.json
that uses Webpack:
"scripts": {
"build": "webpack --mode production --optimize-minimize"
}
This script tells Webpack to run in production mode, which includes optimizations like minification and dead code elimination. The --optimize-minimize
flag further instructs Webpack to minimize the code.
npm run build
# Output:
# Hash: 123abc456def
# Version: webpack 4.44.1
# Time: 5402ms
# Built at: 01/01/2021
# Asset Size Chunks Chunk Names
# bundle.js 1.2 MB 0 [emitted] main
# Entrypoint main = bundle.js
In the output, you’ll notice details about the build process, including the hash, version, build time, and the generated assets. This level of detail is crucial for understanding how your project is compiled and optimized, ensuring you’re delivering the best version of your application to users.
Leveraging Babel
Babel is a JavaScript compiler that lets you use next-generation JavaScript, today. It can be integrated into your build process to transpile ES6+ code down to ES5, ensuring compatibility across browsers. Here’s how you might include Babel in your build script:
"scripts": {
"build": "babel src --out-dir dist --presets=@babel/preset-env"
}
This script compiles the code from the src
directory, outputting the transpiled ES5 code to the dist
directory, using the specified Babel presets for compatibility.
By embracing these advanced tools and techniques, you can transform ‘npm run build’ from a simple command into a comprehensive build solution, capable of handling even the most complex projects with ease.
Exploring Beyond ‘npm run build’
As you advance in your development journey, exploring alternative build tools and commands becomes essential for optimizing your workflow. While ‘npm run build’ is a cornerstone in Node.js and JavaScript development, tools like ‘yarn build’ and task runners such as Gulp or Grunt offer unique advantages in specific scenarios. Let’s delve into these alternatives and understand when and why they might be preferred.
Yarn Build: A Close Cousin
Yarn emerged as a strong competitor to npm, offering faster dependency management and more reliable installations. Its build command, similarly, is a potent alternative to ‘npm run build’. Here’s how you might use it:
yarn build
# Output:
# Done in 0.56s.
The command structure is almost identical, but Yarn prides itself on performance and speed, which can be crucial in large-scale projects or continuous integration environments. The output indicates a successful build, completed in significantly less time.
Gulp/Grunt: Task Runners’ Power
For projects requiring complex build processes, Gulp and Grunt offer more flexibility and power. These task runners allow you to write more sophisticated build scripts, automating tasks beyond bundling and minification. Here’s a Gulp example for a custom build process:
const gulp = require('gulp');
const uglify = require('gulp-uglify');
gulp.task('build', function () {
return gulp.src('src/*.js')
.pipe(uglify())
.pipe(gulp.dest('dist'));
});
gulp build
# Output:
# [16:00:00] Using gulpfile ~/project/gulpfile.js
# [16:00:00] Starting 'build'...
# [16:00:00] Finished 'build' after 800 ms
This script demonstrates Gulp’s ability to read JavaScript files from a source directory, minify them using the uglify
plugin, and output the minified files to a destination directory. The output confirms the successful execution of these tasks, showcasing Gulp’s capability to handle more than just bundling and minification.
Making the Choice
Choosing between ‘npm run build’, ‘yarn build’, and task runners like Gulp or Grunt depends on your project’s needs, the complexity of your build process, and personal or team preferences. While ‘npm run build’ offers simplicity and direct integration with the npm ecosystem, Yarn can provide performance benefits, and task runners offer unparalleled flexibility for complex build tasks. Understanding the strengths of each tool allows you to tailor your build process for optimal efficiency and performance.
Even with a powerful tool like ‘npm run build’, developers can encounter pitfalls and errors. Understanding these common issues and how to address them can save you time and frustration, ensuring a smoother build process.
Missing Scripts Error
One of the most common errors occurs when the ‘build’ script is missing from package.json
. Attempting to run ‘npm run build’ without the necessary script defined will result in an error.
npm run build
# Output:
# npm ERR! missing script: build
This error message is npm’s way of telling you that it can’t find a ‘build’ script in your package.json
. To resolve this, ensure you have correctly defined the ‘build’ script in your project’s package.json
file. This script tells npm what commands to execute when npm run build
is run.
Dependency Issues
Another common issue is failing builds due to missing or incompatible dependencies. This can often be resolved by ensuring all dependencies are correctly installed and up-to-date.
npm install
npm run build
# Output:
# [Success message indicating the build has completed]
Running npm install
ensures that all the project’s dependencies are installed according to the versions specified in package.json
. This step is crucial before attempting to build, as missing dependencies can lead to failed builds.
Environment-Specific Bugs
Sometimes, a build might work perfectly on one developer’s machine but fail on another’s or in a different environment (like production). These issues can often be traced back to differences in global npm packages, node versions, or environment variables.
To mitigate environment-specific bugs, consider using a tool like Docker to containerize your application, ensuring consistency across development, testing, and production environments. Additionally, leveraging nvm
(Node Version Manager) can help manage and sync node versions across different machines.
nvm use
npm run build
# Output:
# [Success message indicating the build has completed in the specified Node environment]
This command sequence ensures you’re using the correct version of Node for your project, as specified in your project’s .nvmrc
file, before running the build command.
Navigating these common issues with ‘npm run build’ not only streamlines your development process but also enhances collaboration across teams by ensuring everyone is working in a consistent environment. Armed with these troubleshooting tips and best practices, you’re better prepared to tackle any build challenges that come your way.
The NPM Ecosystem Unveiled
The Node Package Manager (npm) ecosystem is a cornerstone of modern web development, serving as a vast repository of packages and a powerful tool for managing project dependencies. The command npm run build
sits at the heart of this ecosystem, acting as a bridge between development and production environments.
Understanding package.json
At the core of every npm-based project is the package.json
file. This file serves as the blueprint for your project, detailing everything from dependencies to scripts that automate tasks. Here’s a basic example of a package.json
that includes a build script:
{
"name": "your-project",
"version": "1.0.0",
"scripts": {
"build": "echo 'Building...'
}
}
When the npm run build
command is executed, npm looks for the build
script in package.json
and runs the command specified. In this case, it simply echoes ‘Building…’. While this example is simplified, in real-world applications, the build script would include commands to bundle assets, minify code, and more.
The Significance of Build Steps
Build steps are crucial in web development, transforming the raw code into an optimized, production-ready package. These steps may include transpilation (converting newer JavaScript syntax to older syntax for broader browser compatibility), bundling (combining multiple JavaScript files into one), and minification (reducing file size by eliminating unnecessary characters).
npm run build
# Output:
# Building...
This output is a simplified representation of what happens when a build script is executed. The real power of npm run build
is seen in complex applications where the build process significantly optimizes the application for production use.
Understanding the npm ecosystem, the role of package.json
, and the significance of build steps are fundamental to mastering web development workflows. These components work together to streamline the process from development to deployment, ensuring that developers can focus on creating high-quality code while npm handles the intricacies of package management and build processes.
Expanding ‘npm run build’ Horizons
Mastering the ‘npm run build’ command is just the beginning. The true potential of this command unfolds as you integrate it into broader development practices such as Continuous Integration/Continuous Deployment (CI/CD) pipelines, automated testing, and deployment strategies. Let’s explore how ‘npm run build’ fits into these advanced scenarios, ensuring your projects are not only built efficiently but also delivered seamlessly to your users.
CI/CD Pipelines Integration
Continuous Integration (CI) and Continuous Deployment (CD) are practices designed to automate the testing and deployment of your code. Integrating ‘npm run build’ into CI/CD pipelines ensures that every code change is automatically built and tested, significantly reducing the chances of deployment failures. Here’s an example of a CI configuration snippet that includes ‘npm run build’:
steps:
- name: Install Dependencies
command: npm install
- name: Run Build
command: npm run build
# Output:
# [Success message indicating that the build has completed and is ready for testing or deployment]
In this CI pipeline configuration, npm install
is run to ensure all dependencies are up to date, followed by npm run build
to build the project. The successful execution of these commands is crucial for the next steps in the pipeline, such as automated testing or deployment.
Automated Testing During Build
Automated testing is an essential part of maintaining code quality and stability. By running tests as part of the build process, you can catch and address issues early. Here’s how you might include automated testing in your package.json
:
"scripts": {
"build": "npm run test && webpack --mode production"
}
This script combines running tests (with npm run test
) and the build process in a single step. The &&
ensures that if the tests fail, the build process will not proceed, highlighting the importance of passing tests for a successful build.
Deploying Built Assets
Once your project is built and tested, the next step is deployment. Automating this process ensures that your application is quickly and reliably made available to users. For instance, you might use a script to deploy the built assets to a cloud service:
npm run deploy
# Output:
# [Success message indicating that the application has been deployed to the specified environment]
This command could be part of your CI/CD pipeline, triggered after a successful build and test phase, automating the deployment process and ensuring that your application is always up to date.
Further Resources for NPM Build Mastery
To deepen your understanding and expand your skills, here are three invaluable resources:
- Node.js Official Website: Comprehensive Guides and Documentation – Provides authoritative and comprehensive guides and documentation on Node.js and npm.
Webpack Official Guides: Detailed Tutorials and Configuration Options – Offers in-depth tutorials and various configuration options for Webpack users and developers.
Jenkins Documentation for CI/CD: Insightful Build Process Integration – Shares insights into integrating build processes using Jenkins, the widely-used CI/CD tool.
These resources provide a wealth of information that can help you refine your build processes, integrate with CI/CD pipelines, and ensure your applications are robust, tested, and deployed efficiently.
Wrapping Up: ‘npm run build’ Guide
In this comprehensive guide, we’ve explored the pivotal role of the ‘npm run build’ command in the Node.js and JavaScript development ecosystem. This command is essential for transforming your project into a production-ready package, optimizing it for performance and efficiency.
We began with the basics, explaining how to set up and execute your first ‘npm run build’ command. We then progressed to more complex scenarios, integrating tools like Webpack and Babel to enhance and customize the build process. Along the way, we explored alternative approaches for those looking to expand their toolkit beyond npm, discussing the merits of yarn build and task runners like Gulp or Grunt.
We also tackled common challenges and errors that developers might face when using ‘npm run build’, offering solutions and best practices to ensure a smooth and efficient build process. Furthermore, we delved into the npm ecosystem, understanding the significance of the package.json file and the critical role of build steps in modern web development.
Aspect | Importance | Consideration |
---|---|---|
‘npm run build’ Command | Essential for production readiness | Requires correct script in package.json |
Integration with Tools | Enhances build process | May require additional configuration |
Troubleshooting | Ensures smooth build process | Knowledge of common errors is beneficial |
Whether you’re just starting out with ‘npm run build’ or looking to refine your build process, we hope this guide has provided you with valuable insights and practical knowledge. The ability to efficiently prepare your project for production is a crucial skill in the development world, and mastering ‘npm run build’ is a significant step towards achieving that goal.
With a solid understanding of ‘npm run build’ and its place within the broader development practices, you’re well-equipped to tackle your projects with confidence. Remember, the journey doesn’t stop here; continue to experiment with the command, explore related tools, and always be on the lookout for new practices that can improve your development workflow. Happy coding!