Using Python Raise() Function to Throw Exceptions
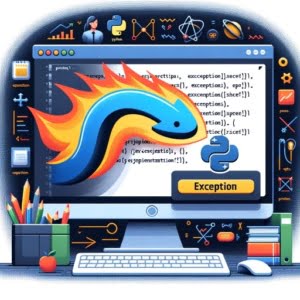
While coding in Python, you might encounter unexpected errors, or ‘exceptions’. Exceptions in Python are events that occur when your program encounters an error during its execution. They are pivotal in Python programming as they assist in identifying and handling errors efficiently.
But what if you want to throw an exception intentionally? There are many reasons to do this, and today we’ll go over the why what and how of throwing exceptions.
In this comprehensive guide, we will navigate through the intricate world of exceptions in Python. We will begin by understanding what exceptions are, followed by learning how to throw, handle, and even create your own exceptions.
So, fasten your seatbelts and get ready to delve deep into the intriguing world of Python exceptions!
TL;DR: How do you throw exceptions in Python?
Throwing exceptions in Python can be done using the
raise
statement. This can help halt execution when a specific undesired condition is met and provides you with a way to output a clear error message. You can even create your own custom exceptions by creating a new class and inheriting from the baseException
class.
# throwing an exception
try:
raise Exception("This is a custom error message.")
except Exception as e:
print(str(e))
# creating a custom exception
class MyCustomException(Exception):
pass
try:
raise MyCustomException("This is a custom exception.")
except MyCustomException as e:
print(str(e))
# Output:
# This is a custom error message.
# This is a custom exception.
Table of Contents
Raising Exceptions in Python
The raise
keyword in Python acts as the flare gun of your code, triggering an exception whenever it’s encountered. When the Python interpreter stumbles upon the raise
statement, the normal flow of the program is interrupted, and control is handed over to the nearest enclosing exception handler.
Consider this simple example:
x = -1
if x < 0:
raise Exception('Sorry, no numbers below zero')
In this code snippet, if x is less than zero, the raise
keyword triggers an Exception with the message ‘Sorry, no numbers below zero’.
Raising Specific Built-In Exceptions
Python is equipped with a plethora of built-in exceptions that you can utilize to indicate different types of errors. For instance, you can raise a TypeError
if the data type of a variable isn’t what you expected, or a ValueError
if a function argument is of the correct type but carries an invalid value.
Here’s an example of raising a TypeError
:
def greet(name):
if not isinstance(name, str):
raise TypeError('name must be a string')
print(f'Hello, {name}!')
greet(123) # This will raise a TypeError
In the code above, the greet
function expects a string argument. If you pass a non-string argument, it raises a TypeError
.
Understanding exception propagation is fundamental in Python exception handling. When an exception is raised without an exception handler in the current function or method, the exception is passed up to the calling function. This propagation continues until an exception handler is found. If no handler is found, the program terminates.
The Influence of Exceptions on Program Flow
The primary impact of exceptions is their ability to alter the normal flow of a program. When an exception is raised, the current operation is halted, and the program attempts to find an appropriate exception handler to deal with the situation.
If no suitable handler is found, the program will terminate. This ability to control program flow is a powerful tool, but it also means that exceptions need to be handled with care to prevent unwanted program termination.
Employing ‘try’ and ‘except’ Blocks
Having grasped how to raise exceptions in Python, let’s shift our focus to managing these exceptions. Managing exceptions is akin to equipping your code with a safety net for potential errors, ensuring you know how to react when they arise.
In Python, try
and except
blocks serve as the primary tools to catch and manage exceptions. The try
block houses the code that might trigger an exception, while the except
block contains the code that executes when an exception occurs.
Consider this basic example:
try:
x = 1 / 0
except ZeroDivisionError:
x = 0
print('Divided by zero. Setting x to 0.')
In the above code, the try
block attempts to divide 1 by 0, which triggers a ZeroDivisionError
. The except
block catches this exception and manages it by setting x
to 0 and printing an informative message.
‘else’ and ‘finally’ in Exception Management
Beyond try
and except
, Python offers else
and finally
clauses to facilitate more nuanced exception handling.
The else
clause comes into play if the try
block doesn’t trigger an exception, while the finally
clause executes regardless of the circumstances, making it the perfect place for cleanup actions.
Here’s an illustration:
try:
x = 1 / 2
except ZeroDivisionError:
print('Divided by zero.')
else:
print('No exceptions raised.')
finally:
print('This gets executed no matter what.')
In this code, since the try
block doesn’t trigger an exception, the else
clause executes, printing ‘No exceptions raised.’ Subsequently, the finally
clause executes, printing ‘This gets executed no matter what.’
Cleanup actions, such as closing files or releasing resources, are vital in exception management. These actions are typically performed in the
finally
clause to ensure they are executed regardless of whether an exception is raised. This practice ensures your program doesn’t leave any loose ends, even when facing errors.
Managing Specific Exceptions
Python allows you to manage specific exceptions by using multiple except
blocks. Each except
block manages a specific type of exception, enabling you to customize your exception management for different error scenarios.
try:
x = 1 / 'a'
except ZeroDivisionError:
print('Divided by zero.')
except TypeError:
print('Invalid operand type.')
In the above code, the try
block triggers a TypeError
, which the second except
block catches and manages.
When dealing with exceptions, it’s crucial to catch and manage specific exceptions rather than catching all exceptions. This strategy enables you to respond appropriately to different types of errors. Furthermore, avoid using exception handling as a regular flow control tool; exceptions should be reserved for unexpected events.
Built-In Exceptions in Python
Python comes equipped with a variety of built-in exceptions that symbolize different types of errors.
Here is a table summarizing some common built-in exceptions in Python:
Exception | Description |
---|---|
Exception | Base class for all built-in exceptions, excluding StopIteration , GeneratorExit , KeyboardInterrupt , and SystemExit . Can be utilized to catch all exceptions. |
ArithmeticError | Base class for exceptions raised for arithmetic errors such as OverflowError , ZeroDivisionError , and FloatingPointError . |
IOError | Triggered when an I/O operation fails. For instance, the print statement or the open() function when trying to open a non-existent file. |
ImportError | Triggered when an import statement fails to find the module definition or when a from ... import fails to find a name that is to be imported. |
Designing Custom Exceptions in Python
While Python’s arsenal of built-in exceptions covers a broad spectrum of error scenarios, there might be instances when you need to define your own exceptions. This is where the concept of custom exceptions comes into the picture.
Custom exceptions are user-defined exceptions that you formulate to signal specific error conditions in your code. They prove to be particularly beneficial when you want to raise an exception that doesn’t seamlessly fit into Python’s built-in exceptions.
Crafting a custom exception in Python is a straightforward process. All you need to do is define a new class that inherits from the Exception
class or one of its subclasses. Here’s an example:
class CustomError(Exception):
pass
try:
raise CustomError('This is a custom error')
except CustomError as e:
print(e)
In this snippet, we define a new exception type named CustomError
that inherits from the Exception
class. We then raise and catch this custom exception in a try
/except
block.
When creating custom exceptions, it’s advisable to provide meaningful names for your exceptions and to define a docstring for each exception that explains when it should be raised. It’s also a good practice to define custom exceptions in a separate module or file, especially if they’re used across multiple modules.
Advanced Topics & Resources
While we’ve journeyed through the core concepts of exceptions in Python, there’s a vast landscape yet to be explored. Let’s dive deeper into some advanced topics and resources that can further bolster your understanding and application of exceptions in Python.
Exception Chaining & Re-raising Exceptions
Python 3 introduced the concept of exception chaining, a mechanism that allows one exception to be raised in the except
or finally
block of another exception. This proves to be useful when you intend to add additional context or information to an exception before passing it on.
Here’s an example:
try:
1 / 0
except ZeroDivisionError as e:
raise RuntimeError('A division by zero occurred') from e
In this snippet, when the ZeroDivisionError
is raised, we catch it and raise a RuntimeError
with additional information. The from e
clause connects the original ZeroDivisionError
to our RuntimeError
.
You can also re-raise the last exception that was active in the current scope using the raise
statement with no argument, like so:
try:
1 / 0
except ZeroDivisionError:
print('A division by zero occurred. Reraising the exception...')
raise
In this snippet, after catching the ZeroDivisionError
and printing a message, we re-raise the same exception using raise
.
Exceptions in Test-Driven Development
In test-driven development (TDD), you pen down tests for your code before you write the code itself. Exceptions play a pivotal role in this process. By testing for the occurrence of specific exceptions, you can ensure your code behaves as expected under exceptional conditions.
Here’s how you might use exceptions in test-driven development using Python’s unittest module. This example asserts that a ValueError is raised when trying to convert a string to an integer.
import unittest
def convert_to_int(input_data):
if not isinstance(input_data, int):
raise ValueError("Input data must be of type int.")
return int(input_data)
class TestConversion(unittest.TestCase):
def test_convert_to_int_raises_value_error(self):
with self.assertRaises(ValueError):
convert_to_int('a string')
if __name__ == '__main__':
unittest.main()
Here, convert_to_int
is the function we’re developing and testing. We’re writing a test test_convert_to_int_raises_value_error
that asserts our function should raise a ValueError when we try to pass a string to this function.
Additional Resources for Learning About Exceptions in Python
For a Complete Guide on the Python testing ecosystem and understand where Pytest fits in, Click Here!
And for those keen to delve deeper into the world of exceptions in Python, here are some other resources that you might find insightful:
- Python ImportError: Dealing with “No Module Named” Issues – Explore common scenarios where this error occurs and find solutions.
Python ValueError: Handling and Avoiding Invalid Input – Learn how and where ValueErrors occur and discover best practices for resolution.
Errors and Exceptions in Python – Python’s official tutorial on understanding and handling errors and exceptions.
Handling Exceptions in Python with Real Python – A comprehensive guide by Real Python explaining how to handle exceptions in Python effectively.
Built-in Exceptions in Python – The official Python documentation detailing the built-in exceptions provided by the Python language.
Concluding Thoughts
Throughout this guide, we’ve navigated Python exceptions, starting from a basic understanding of what they are, to learning how to throw, handle, and even craft your own exceptions.
We’ve discussed that exceptions are not merely error messages – they’re potent tools that can help us steer the flow of our programs and fortify them against potential pitfalls. We’ve seen the power of the raise
keyword in triggering exceptions, and how try
and except
blocks enable us to catch and manage them.
But this isn’t the end of the road. The realm of Python exceptions is vast and ripe for exploration. So, keep experimenting, keep coding, and most importantly, don’t let exceptions intimidate you. Embrace them, understand them, and harness them to your advantage to become a more proficient Python developer.