npm Request Module | Node.js HTTP Requests Guide
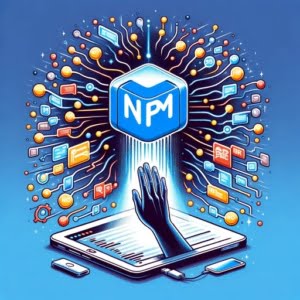
Ensuring seamless communication between our customers dedicated servers and services has always been crucial to us at IOFLOOD. We’ve thoroughly explored managing HTTP requests with the Request library in Node.js in the past which offers powerful features for making HTTP requests. As the requests library has deprecated as of Fen 11th, 2020 updated alternatives have become available, but understanding its usage and alternatives is crucial for modern Node.js applications. To help with this, we’ve gathered our notes and code examples into this guide.
This guide will navigate you through making HTTP requests with Request and introduce you to the new tools on the horizon. Whether you’re a seasoned developer familiar with Request or a newcomer eager to understand the basics and beyond, this journey is for you.
Let’s embark on this adventure together, exploring how Request has shaped the way we interact with the web and how its successors promise to carry on its legacy.
TL;DR: How Do I Use the Request Library in Node.js?
To use the Request library, install it via npm,
npm install request
. Then require the request module in your Node.js script,const request = require('request');
, and use the request function to make a GET request to a URL. However, with its deprecation, consider alternatives like Axios or Node’s native HTTP module for future projects.
Here’s a simple example:
const request = require('request');
request('http://example.com', function (error, response, body) {
console.log(body);
});
# Output:
# The HTML content of example.com
In this example, we use the request
module to make a simple GET request to ‘http://example.com’. The callback function is executed once the request completes, logging the body of the response, typically HTML content, to the console. This demonstrates the ease with which the Request library can be used to perform HTTP requests in Node.js applications.
This is a basic way to use the Request library in Node.js, but there’s much more to learn about making HTTP requests and exploring new, more modern alternatives. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Request NPM
Installing Request in Your Project
Embarking on your Node.js journey, the first step is to incorporate the Request library into your project. This library has been a cornerstone for many developers, simplifying how HTTP requests are made. Although it’s important to note that while Request is deprecated, understanding its usage provides a solid foundation for exploring modern alternatives.
To install Request, you will use npm, Node.js’s package manager. Here’s how:
npm install request
This command fetches the Request library and adds it to your project, making it ready for use.
Making Your First HTTP Request
With Request installed, let’s move on to making a simple HTTP GET request. This example will demonstrate the ease of use that made Request a go-to choice for developers.
const request = require('request');
request('https://api.example.com/data', { json: true }, (error, response, body) => {
if (!error && response.statusCode == 200) {
console.log(body);
}
});
# Output:
# {"data": "Here is some example data"}
In this block, we’re requesting data from ‘https://api.example.com/data’, specifying that we expect the response to be in JSON format by setting { json: true }
. The callback function is triggered once the request completes. If no error occurred and the response’s status code is 200 (indicating success), the body of the response is logged to the console, showing the JSON data received.
This example underscores the simplicity and effectiveness of Request for basic HTTP requests. It’s a testament to why it became such a popular choice among developers for Node.js applications. As we move forward, keep in mind the evolving landscape of HTTP request libraries and the importance of adapting to new tools.
Advanced Request NPM Techniques
Leveraging Streams for Efficient Data Handling
When working with large datasets or continuous data flows, streams in Node.js become incredibly useful. The Request library elegantly supports streaming, allowing for efficient data processing without overwhelming your application’s memory.
Here’s an example of using Request with streams:
const fs = require('fs');
const request = require('request');
let writeStream = fs.createWriteStream('output.txt');
request('http://example.com/file.txt').pipe(writeStream);
writeStream.on('finish', function() {
console.log('File download completed.');
});
# Output:
# File download completed.
In this scenario, data from ‘http://example.com/file.txt’ is streamed directly to a file named ‘output.txt’ in your project directory. This method is particularly efficient for handling large files or data streams, as it minimizes memory usage and ensures data is processed as it’s received.
Mastering POST Requests and JSON Handling
While GET requests are common, POST requests are crucial for sending data to servers. Combining POST requests with JSON data handling showcases the flexibility of Request. Let’s explore how to perform a POST request and handle JSON data.
const request = require('request');
let userData = { name: 'John Doe', email: '[email protected]' };
request.post(
'https://api.example.com/users',
{ json: userData },
function (error, response, body) {
if (!error && response.statusCode == 201) {
console.log('User created:', body);
}
}
);
# Output:
# User created: {"id": "123", "name": "John Doe", "email": "[email protected]"}
This example demonstrates sending a POST request to ‘https://api.example.com/users’ with JSON data containing user information. The { json: userData }
configuration automatically sets the content type to ‘application/json’ and stringifies the userData
object, making the process seamless. The callback function is executed upon successful request completion, indicated by a status code of 201 (Created), confirming the user’s creation on the server.
Both examples illustrate the power and versatility of Request for handling diverse HTTP request scenarios. As you grow more comfortable with these advanced techniques, you’ll find Request an invaluable tool in your Node.js development toolkit.
Exploring Alternatives to Request NPM
Axios: The Promised-Based HTTP Client
In the quest for request npm
alternatives, Axios shines brightly, especially for developers who prefer working with promises. Unlike the callback approach of Request, Axios returns a promise, making it easier to manage asynchronous code.
Here’s how you make a GET request with Axios:
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error:', error);
});
# Output:
# {"data": "Here is some example data"}
In this example, axios.get
is used to fetch data from a URL. The .then
method is employed to handle the response, logging the received data to the console. Should an error occur, .catch
is there to handle it, showcasing Axios’s straightforward error management. The promise-based structure of Axios simplifies handling asynchronous HTTP requests, making code cleaner and more readable.
Got: Streamlined HTTP Requests
Got is another formidable alternative to Request, offering a blend of simplicity and power. It supports both callback and promise interfaces, providing flexibility in handling HTTP requests.
Here’s a snippet demonstrating a simple GET request with Got:
const got = require('got');
(async () => {
try {
const response = await got('https://api.example.com/data');
console.log(JSON.parse(response.body));
} catch (error) {
console.log(error.response.body);
}
})();
# Output:
# {"data": "Here is some example data"}
In this example, got
is utilized within an async function to perform a GET request. The use of await
allows for the asynchronous request to be handled in a synchronous manner, with the response’s body being parsed and logged. This demonstrates Got’s ease of use and its support for modern JavaScript features.
Node.js Native HTTP/HTTPS Module
For those seeking a no-dependency solution, Node.js’s native HTTP and HTTPS modules are perfect. They provide the most direct way to make HTTP requests without external libraries.
A GET request using Node’s HTTPS module looks like this:
const https = require('https');
https.get('https://api.example.com/data', (resp) => {
let data = '';
// A chunk of data has been received.
resp.on('data', (chunk) => {
data += chunk;
});
// The whole response has been received.
resp.on('end', () => {
console.log(JSON.parse(data));
});
}).on("error", (err) => {
console.log("Error: " + err.message);
});
# Output:
# {"data": "Here is some example data"}
This snippet demonstrates making a GET request to an API using the HTTPS module. Data is collected in chunks, then parsed and logged once the entire response is received. This example highlights the module’s direct approach to handling HTTP requests, emphasizing control and minimizing dependencies.
Each of these alternatives to Request offers unique advantages, from promise-based handling in Axios to the dependency-free approach of Node’s native modules. Understanding when and why to use each can greatly enhance your Node.js development experience.
Troubleshooting Requests in NPM
Handling Network Errors Gracefully
When making HTTP requests, encountering network errors is common. Whether you’re using Request or its alternatives, it’s crucial to handle these errors to ensure your application remains robust. Here’s an example of handling network errors with the Request library:
const request = require('request');
request('https://api.example.inaccessible.com/data', function (error, response, body) {
if (error) {
console.error('Network error:', error);
}
console.log(body);
});
# Output:
# Network error: { Error: getaddrinfo ENOTFOUND api.example.inaccessible.com }
In this snippet, a request is made to an inaccessible URL, resulting in a network error. The error object is logged, providing valuable information for troubleshooting. Handling errors in this manner allows your application to respond intelligently to network issues, such as retrying the request or alerting the user.
JSON Parsing Safeguards
Working with JSON data is a staple in modern web development. However, parsing JSON can lead to errors if not done cautiously. Here’s how you can safeguard your JSON parsing with Axios:
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => {
try {
const data = JSON.parse(response.data);
console.log(data);
} catch (error) {
console.error('Parsing error:', error);
}
})
.catch(error => {
console.error('Network error:', error);
});
# Output:
# Parsing error: SyntaxError: Unexpected token o in JSON at position 1
This example demonstrates an attempt to parse the response.data
using JSON.parse
. However, if response.data
is already an object or malformed, a syntax error occurs during parsing. Wrapping the parsing in a try-catch
block allows you to catch and handle these parsing errors effectively, preventing your application from crashing and providing a means to debug the issue.
Both examples underscore the importance of error handling when working with HTTP requests and JSON data. By implementing these troubleshooting strategies, you can create more resilient Node.js applications that can withstand common pitfalls encountered during development.
HTTP Requests Explained in Node.js
The Role of Libraries Like Request
HTTP requests are the backbone of communication in the world of web development. They enable interaction between clients and servers, fetching data, submitting forms, and much more. In Node.js, making these requests requires an understanding of its built-in modules or the use of external libraries for simplified interaction. The Request library emerged as a popular choice, offering a straightforward and intuitive API for making HTTP requests.
Why did developers gravitate towards Request? Its simplicity and ease of use made it a standout choice. For example, to make a basic GET request to fetch data from an API, you could simply do:
const request = require('request');
request('https://api.example.com', function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log('Data fetched successfully:', body);
}
});
# Output:
# Data fetched successfully: {"info": "Here is your data"}
This code snippet illustrates how Request allows for easy HTTP requests, managing the complexities of networking and HTTP protocols behind its simple API. The callback function receives error, response, and body parameters, enabling developers to handle responses and errors efficiently.
The Deprecation of Request
As the web development landscape evolves, so do the tools and libraries we use. Request has been deprecated, not due to a lack of functionality, but as a natural progression towards more modern and versatile solutions. The deprecation of Request marks a shift in the Node.js community towards libraries that support promises and async/await syntax out of the box, offering improved error handling and cleaner code.
The move away from Request doesn’t diminish its impact on Node.js development. It serves as a testament to the library’s role in shaping HTTP request handling in Node.js. As developers, embracing the evolution of these tools allows us to build more robust and efficient applications.
Understanding the fundamentals of HTTP requests and the role of libraries like Request in Node.js equips developers with the knowledge to adapt to new tools and methodologies. This foundation is crucial for navigating the ever-changing landscape of web development, ensuring we’re always leveraging the best tools for our projects.
Expanding Your Node.js Horizons
Integrating HTTP Requests into Larger Applications
HTTP requests are the cornerstone of web interaction, but they’re just the starting point in the vast landscape of Node.js development. Understanding how to use libraries like Request and its modern successors is crucial, but integrating these requests into larger Node.js applications opens a world of possibilities. From web scraping to API consumption, the ability to efficiently handle HTTP requests enables developers to build complex, data-driven applications.
Consider a scenario where you’re consuming data from multiple APIs to aggregate information:
const axios = require('axios');
async function fetchUserData() {
const userResponse = await axios.get('https://api.example.com/users/1');
const postsResponse = await axios.get(`https://api.example.com/users/1/posts`);
console.log('User:', userResponse.data);
console.log('Posts:', postsResponse.data);
}
fetchUserData();
# Output:
# User: {"id": "1", "name": "John Doe"}
# Posts: [{"id": "1", "title": "A New Beginning"}, {"id": "2", "title": "The Node.js Journey"}]
This example demonstrates fetching user data and their posts from an API using Axios, a popular alternative to Request. By leveraging async/await syntax, the code remains clean and easy to understand, showcasing how HTTP requests can be seamlessly integrated into larger applications for data aggregation.
Discovering Related Libraries and Frameworks
The Node.js ecosystem is rich with libraries and frameworks that can enhance your projects and streamline development. Exploring these tools can provide new ways to approach HTTP requests and application architecture.
Further Resources for Node.js Development
To deepen your understanding and expand your toolkit, consider exploring the following resources:
- Node.js Official Documentation: A comprehensive resource for all things Node.js, including guides on HTTP request handling.
Express.js: A fast, unopinionated, minimalist web framework for Node.js, perfect for building APIs and web applications.
NestJS: A progressive Node.js framework for building efficient, reliable, and scalable server-side applications.
These resources provide a wealth of information and tools for enhancing your Node.js projects, from making HTTP requests to building full-fledged web applications. By exploring these further resources, you can continue to grow as a Node.js developer and create more sophisticated and powerful applications.
Recap: HTTP Requests in Node.js
In this comprehensive guide, we’ve explored the intricacies of making HTTP requests in Node.js, with a special focus on the Request library. Despite its deprecation, the Request library has played a pivotal role in simplifying HTTP requests for Node.js developers, serving as a trusted tool for many years.
We began with the basics of installing Request and making your first HTTP request, highlighting the simplicity that made it a popular choice among developers. We then delved deeper into advanced usage, exploring how to handle streams and errors, and how to use Request for more complex scenarios like POST requests and handling JSON data.
As we ventured into the realm of alternatives, we discovered robust libraries like Axios, Got, and Node.js’s native HTTP/HTTPS module. Each offers unique advantages, from promise-based handling in Axios to the dependency-free approach of Node’s native modules, catering to different developer preferences and project requirements.
Library | Feature | Use Case |
---|---|---|
Request | Callback-based, simple syntax | Quick HTTP requests, deprecated |
Axios | Promise-based, interceptors | Modern web apps, API consumption |
Got | Promise & callback, stream support | Versatile HTTP requests, advanced use cases |
Node.js HTTP/HTTPS | No external dependencies | Low-level HTTP requests, full control |
Whether you’re just starting out with HTTP requests in Node.js or seeking to update your toolkit with modern alternatives, this guide aims to provide a thorough understanding of your options. Embracing the evolving landscape of HTTP request handling ensures that your development practices remain efficient and up-to-date.
The journey through HTTP requests in Node.js, from using the Request library to exploring its successors, underscores a key aspect of web development: adaptability. As technologies evolve, so too must our tools and approaches. By staying informed and flexible, developers can continue to build powerful, efficient applications that leverage the best of what the Node.js ecosystem has to offer. Happy coding!