Webpack & NPM | Setup and Integration Guide
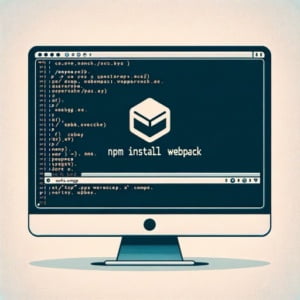
At IOFLOOD, we needed to find a way to efficiently bundle our project files, especially when working with JavaScript applications. We’ve found that using Webpack with npm provides the solution we needed. That’s why we’ve put together a guide on how to use Webpack with npm. By following our straightforward instructions, you’ll be equipped to manage and bundle your project files, ensuring smooth project deployment.
This guide will navigate you through the basics to advanced techniques of integrating webpack with npm, ensuring a smooth development process. Whether you’re just starting out or looking to refine your workflow, this article aims to provide you with a comprehensive understanding of how these powerful tools work together to streamline your projects.
Let’s simplify the process of bundling JavaScript files together and enhance our project workflows!
TL;DR: How Do I Use Webpack with NPM?
To utilize Webpack with npm, begin by installing Webpack locally with the command
npm install --save-dev webpack
. This must be installed within your project.
For example:
npm install --save-dev webpack
Then, you can run webpack using npx or by adding it as a script in your package.json. This basic setup is your entry into the world of modern web development, leveraging webpack’s powerful bundling capabilities in conjunction with npm’s vast repository of packages.
Here’s a quick example of adding a webpack script to your package.json
:
"scripts": {
"build": "webpack"
}
After adding the script, you can run webpack through npm by executing:
npm run build
# Output:
# This will execute the webpack build process based on your `webpack.config.js` settings.
This is just the beginning of what you can achieve with webpack and npm. Continue reading for more detailed setup, configuration, and advanced usage scenarios.
Table of Contents
The Basics: Webpack & NPM
Embarking on your journey with webpack and npm begins with a few simple steps. This section will guide you through installing webpack using npm, creating your first webpack configuration file, and running your first build. Each of these steps is a building block in mastering web development with these powerful tools.
Installing Webpack
The first step is to install webpack along with webpack-cli (the command line interface for webpack), which allows you to run webpack commands. Execute the following command in your project’s root directory:
npm install --save-dev webpack webpack-cli
# Output:
# + webpack@
# + webpack-cli@
This command installs webpack and webpack-cli as development dependencies in your project, ensuring they are only used in the development environment. The output indicates the successful installation of webpack and its CLI with their respective versions.
Creating a Webpack Configuration File
Once webpack is installed, the next step is to create a basic webpack.config.js
file. This file tells webpack how to behave and process your project’s files.
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
}
};
In this configuration, entry
specifies the entry point file of your application, while output
defines where to output the bundled files and what to name them. path.resolve
ensures that the absolute path is used, which is crucial for avoiding errors in the webpack build process.
Running Your First Webpack Build
With the webpack configuration file in place, you can now run your first build. This process compiles your source code into a single bundled file that can be served to your users.
npx webpack
# Output:
# asset bundle.js 1.54 MiB [emitted] (name: main)
# ./src/index.js 1 KiB [built]
# webpack 5.x.x compiled successfully
This output shows that webpack has successfully created a bundle.js
file from your src/index.js
. The size of the bundled file and the successful compilation message indicate that your setup is correct and ready for further development.
Understanding these basic steps of using webpack with npm not only sets the foundation for more advanced concepts but also empowers you to streamline your web development process effectively.
Elevate Your Webpack Game
As you grow more comfortable with the basics of webpack and npm, it’s time to explore the more advanced features that can significantly enhance your development workflow. This section delves into loaders, plugins, and optimization techniques that are crucial for managing complex projects efficiently.
Utilizing Loaders
Loaders in webpack transform and bundle different types of files. They are powerful tools for incorporating preprocessors like Sass or Babel into your workflow. Here’s how you can add a CSS loader to your webpack.config.js
:
module.exports = {
module: {
rules: [
{
test: /\.css$/,
use: ['style-loader', 'css-loader']
}
]
}
};
This configuration allows webpack to process .css
files, injecting them into the DOM with the style-loader
and interpreting @import
and url()
like import/require()
with the css-loader
.
Integrating Plugins
Plugins offer a wide range of functionalities, from bundle optimization to environment variable injection. Here’s an example of adding the HtmlWebpackPlugin, which simplifies the creation of HTML files to serve your bundles:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html'
})
]
};
This plugin automatically generates an HTML file with your bundle injected, a vital step for web apps that rely on complex webpack configurations.
Streamlining Builds with NPM Scripts
Leveraging npm scripts can simplify the execution of webpack builds and other tasks. Instead of remembering complex command line instructions, you can define scripts in your package.json
:
"scripts": {
"build": "webpack --mode production",
"dev": "webpack serve --open --mode development"
}
With these scripts, running npm run build
will trigger a production build, optimizing your bundle for deployment, while npm run dev
starts a development server and opens your project in the browser, enhancing your development experience.
Understanding and applying these intermediate-level webpack and npm features will not only make your projects more robust but also significantly improve your productivity as a developer.
Exploring Beyond Webpack
While webpack is a powerful tool for module bundling in web development, it’s not the only player in the game. The npm ecosystem is rich with alternative tools that might better suit specific project needs. Understanding when to opt for webpack or pivot to another tool can significantly impact your project’s efficiency and performance.
Webpack vs. Parcel
Parcel prides itself on being a ‘zero configuration’ bundler, which can be a game-changer for projects requiring a quick setup. Here’s a basic command to get started with Parcel:
npm install --save-dev parcel-bundler
# Output:
# + parcel-bundler@<version>
After installation, you can run Parcel on your entry file without any configuration:
npx parcel src/index.html
# Output:
# Server running at http://localhost:1234
# Built in 8.78s.
This simplicity contrasts with webpack’s configuration-driven approach, making Parcel an excellent choice for projects where rapid development and simplicity are key.
Webpack vs. Rollup
Rollup, on the other hand, is known for its efficient handling of ES modules, making it ideal for libraries and applications prioritizing modern JavaScript. Here’s how you might set up Rollup:
npm install --save-dev rollup
# Output:
# + rollup@<version>
Rollup’s focus on ES module syntax allows for smaller bundles and faster loading times, a critical factor for performance-sensitive applications.
Choosing the Right Tool
The decision between webpack, Parcel, and Rollup often comes down to the specific needs of your project. Webpack’s extensive plugin system and loader support make it a versatile choice for complex applications. However, for projects where simplicity and speed are more critical, Parcel’s zero-configuration approach or Rollup’s efficiency with ES modules might be more suitable.
In the npm ecosystem, the choice of tooling is vast, and each project’s unique requirements will guide that choice. By understanding the strengths and limitations of webpack and its alternatives, developers can make informed decisions that best suit their project needs.
Troubleshooting Webpack and NPM
Even with a solid understanding of webpack and npm, developers may encounter hurdles that can disrupt the development workflow. This section covers common pitfalls, errors, and their solutions to ensure your projects run smoothly.
Version Conflicts
One of the frequent issues developers face is version conflicts between webpack and its loaders or plugins. This can result in unexpected behavior or build failures. To check your webpack version and ensure compatibility, use the following command:
npm list webpack
# Output:
# webpack@<your-version>
Understanding the version of webpack you’re working with helps in troubleshooting compatibility issues. It’s crucial to consult the documentation of any plugins or loaders you’re using to ensure they support your webpack version.
Configuration Errors
A misconfigured webpack.config.js
can lead to several issues, from failed builds to incorrect bundle outputs. One common mistake is incorrect path configurations. Here’s an example of how to set up your output path correctly:
const path = require('path');
module.exports = {
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
}
};
# Output:
# Correctly configured output path ensures your bundled files are stored in the desired directory.
This configuration ensures that webpack outputs the bundled files to the dist
directory in your project. The path.resolve
method is used to avoid path-related errors by providing an absolute path.
Performance Issues
Webpack builds can become slow as projects grow, affecting development speed. One way to improve build performance is by using the cache
option, which caches the generated webpack modules and chunks to improve rebuild speed.
module.exports = {
cache: {
type: 'filesystem'
}
};
# Output:
# Enabling caching can significantly reduce build times during development.
Enabling file system caching tells webpack to store build information on the disk. This can drastically reduce build times, especially for large projects, by reusing previously computed module information.
Addressing these common issues not only ensures a smoother development process but also enhances the efficiency and reliability of your web development projects. By adopting these best practices, developers can mitigate the challenges associated with using webpack and npm together.
Web Development and Webpack
In the realm of modern web development, understanding the core concepts of module bundling and package management is paramount. This knowledge not only empowers developers to streamline their development workflows but also enhances the performance and efficiency of the applications they build.
The Role of Webpack
Webpack serves as a module bundler, a tool that compiles modules with dependencies into static assets. It’s designed to manage and understand the relationships between modules, transforming them into browser-friendly formats. Here’s a basic example of how webpack bundles modules:
import { add } from './math.js';
console.log(add(2, 3));
// Output:
// 5
In this code block, webpack would bundle the math.js
module along with the main file, ensuring that all dependencies are resolved and compiled into a single file. This process significantly reduces the number of HTTP requests required to load a web application, leading to faster loading times.
Understanding NPM
NPM, or Node Package Manager, plays a crucial role in package management. It allows developers to share and reuse code, and it’s an essential tool for installing and managing dependencies in modern web development. An example command to install a package using npm is:
npm install lodash
# Output:
# + lodash@<version>
This command installs the lodash
library, a utility library offering a wide range of functions for JavaScript developers. NPM handles the downloading, installation, and management of such packages, making it easier for developers to incorporate external libraries and tools into their projects.
Webpack and NPM: A Synergistic Relationship
Webpack and npm complement each other in the web development ecosystem. Webpack’s module bundling capabilities, combined with npm’s extensive package management features, streamline the development process. This synergy allows developers to efficiently manage project dependencies and bundle them into optimized, production-ready assets.
The evolution of these tools has significantly influenced the web development landscape, shifting the focus towards modular, maintainable, and high-performance web applications. Understanding the fundamentals of webpack and npm is not just about mastering the tools; it’s about embracing a philosophy of efficient web development that leverages the strengths of these powerful technologies.
Further Usage Cases of Webpack
As your journey with webpack and npm progresses, integrating these tools with other technologies can unlock even greater potential in your web development projects. This section explores how webpack can be combined with Babel, React, and TypeScript to create robust, scalable, and modern web applications.
Integrating Webpack with Babel
Babel is a JavaScript compiler that allows you to use next-generation JavaScript, today. By integrating Babel with webpack, you can ensure your JavaScript code is compatible across all browsers. Here’s a basic setup for using Babel with webpack:
npm install --save-dev babel-loader @babel/core @babel/preset-env
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
}
]
}
};
This configuration allows webpack to process your JavaScript files with Babel, transforming ES6 and beyond into a format that can be understood by older browsers. The result is a more inclusive web experience for users across different platforms and devices.
Leveraging React with Webpack and NPM
React is a popular JavaScript library for building user interfaces. Combining React with webpack enhances your development workflow by bundling your React components and assets efficiently. Here’s how you can set up React with webpack:
npm install --save-dev react react-dom
This command installs React and ReactDOM as development dependencies, allowing you to start building React components within your webpack-managed project.
TypeScript: Adding Types to JavaScript
TypeScript brings static typing to JavaScript, improving developer productivity and code quality. Integrating TypeScript with webpack is straightforward, thanks to TypeScript’s compatibility with the npm ecosystem. Here’s a simple TypeScript setup with webpack:
npm install --save-dev typescript ts-loader
module.exports = {
module: {
rules: [
{
test: /\.ts$/,
use: 'ts-loader',
exclude: /node_modules/
}
]
}
};
This setup enables webpack to handle TypeScript files, compiling them into JavaScript that browsers can interpret, thereby combining the power of static typing with webpack’s bundling capabilities.
Further Resources for Webpack and NPM Mastery
To deepen your understanding and skills in using webpack and npm, here are three valuable resources:
- The official Webpack Documentation provides comprehensive guides, concepts, and API references.
NPM Documentation is an excellent resource for understanding package management, scripts, and more.
TypeScript and Webpack – This guide offers insights on integrating TypeScript with webpack.
Exploring these resources will not only enhance your proficiency with webpack and npm but also equip you with the knowledge to integrate these tools with other technologies in your web development stack.
Wrap Up: NPM Webpack Usage Guide
In this comprehensive guide, we’ve navigated through the essential steps to effectively use webpack with npm, highlighting the significance of these tools in modern web development. From the initial setup to exploring advanced features, our journey has equipped you with the knowledge to streamline your development process.
We began with the basics, installing webpack with npm and crafting a simple webpack.config.js
file. This foundation enabled us to run our first webpack build, an important milestone in understanding how webpack transforms and bundles our project’s files.
npm install --save-dev webpack webpack-cli
# Output:
# webpack and webpack-cli installed
This command installs webpack and its CLI as development dependencies, marking the first step in our webpack journey. The importance of this step cannot be overstated, as it sets the stage for all subsequent webpack operations.
Moving forward, we delved into more complex configurations, leveraging loaders and plugins to handle various file types and enhance our project’s functionality. We also explored how npm scripts can simplify the execution of webpack builds, making our workflow more efficient.
module.exports = {
plugins: [
new HtmlWebpackPlugin({ template: './src/index.html' })
]
};
# Output:
# HtmlWebpackPlugin simplifies the creation of HTML files
This snippet demonstrates adding the HtmlWebpackPlugin to our webpack configuration, automating the generation of an HTML file for our project. It’s a clear example of how plugins can significantly improve our development process.
We also considered alternative tools available through npm, weighing the pros and cons of webpack against other module bundlers. This comparison is crucial for understanding when webpack is the best tool for the job and when another tool might better suit a project’s needs.
Tool | Flexibility | Ease of Use | Feature Set |
---|---|---|---|
Webpack | High | Moderate | Extensive |
Parcel | Moderate | High | Moderate |
Rollup | Moderate | Moderate | Focused |
As we wrap up, it’s clear that mastering webpack and npm is about more than just learning commands and configurations. It’s about understanding how these tools fit into the larger picture of web development, enabling us to build more efficient, scalable, and maintainable applications.
Whether you’re just starting out or looking to deepen your understanding of webpack and npm, this guide has laid the groundwork for you to explore these tools further. The journey of learning never truly ends, but with the foundation we’ve built together, you’re well-equipped to tackle the challenges and opportunities of modern web development. Happy coding!