Char Data Type in Java: How to Declare and Use
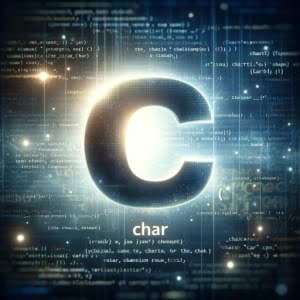
Ever found yourself wrestling with characters in Java? You’re not alone. Many developers find handling chars in Java a bit tricky. Think of a char in Java as a single letter in a word – it represents a single character, and it’s a fundamental part of Java programming.
Chars in Java are versatile and can be used in a variety of ways, from storing simple characters to representing Unicode characters. They are an essential tool in a developer’s toolbox.
This guide will walk you through everything you need to know about working with chars in Java, from declaration to manipulation and conversion. We’ll cover everything from the basics of declaring and using chars, to more advanced techniques such as manipulating and comparing chars, and even alternative approaches.
Let’s dive in and start mastering chars in Java!
TL;DR: How Do I Declare and Use a Char in Java?
To declare and use a char in Java, you use the
char
keyword before a variable name, iechar newChar = 'X';
. You can then manipulate and use this char in various ways in your Java programs.
Here’s a simple example:
char letter = 'A';
System.out.println(letter);
// Output:
// 'A'
In this example, we declare a char variable named ‘letter’ and assign it the value ‘A’. We then print out the value of ‘letter’, which outputs ‘A’.
This is a basic way to declare and use a char in Java, but there’s much more to learn about working with chars in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Declaring and Using Chars in Java: A Beginner’s Guide
- Manipulating and Comparing Chars in Java
- Exploring Alternative Ways to Work with Characters in Java
- Troubleshooting Common Errors with Java Chars
- Understanding Java Char and Unicode
- Applying Java Char in Real-World Programs
- Related Topics for Further Reading
- Wrapping Up: Char Data Type
Declaring and Using Chars in Java: A Beginner’s Guide
In Java, the char keyword is used to declare a character variable. It’s a primitive data type, which means it’s built into the Java language itself. Let’s dive into how to declare and use chars in Java with a simple example.
char myChar = 'J';
System.out.println(myChar);
// Output:
// 'J'
In this example, we declare a char variable named ‘myChar’ and assign it the character ‘J’. The System.out.println(myChar) line then prints out the value of ‘myChar’, which is ‘J’.
It’s important to note that chars in Java are enclosed in single quotes (”), unlike strings which are enclosed in double quotes (“”). This is a key difference between chars and strings in Java.
Advantages and Potential Pitfalls
Chars are incredibly efficient in terms of memory usage. Each char in Java takes up only 2 bytes of memory, making them a great choice when memory usage is a concern.
However, chars in Java can represent a single character only. If you need to work with multiple characters, you’ll need to use a string or an array of chars.
Also, remember that chars in Java are case-sensitive. This means that the char ‘a’ is different from the char ‘A’. Always be mindful of this when working with chars in Java.
Manipulating and Comparing Chars in Java
As you become more familiar with Java char, you’ll find that there’s a lot more you can do beyond simply declaring and printing them. Let’s delve into some of these advanced techniques, such as manipulating and comparing characters.
Char Manipulation in Java
Did you know that you can perform arithmetic operations on chars in Java? This is because chars in Java are, in fact, numeric values representing Unicode characters. Let’s take a look at an example:
char letter = 'A';
letter++;
System.out.println(letter);
// Output:
// 'B'
In this example, we increment the char ‘A’ by 1, which results in the char ‘B’. This is because in the Unicode character set, ‘B’ is one position after ‘A’.
Comparing Chars in Java
Chars can also be compared using relational operators like ==, !=, , =
. Here’s a quick demonstration:
char firstLetter = 'A';
char secondLetter = 'B';
System.out.println(firstLetter < secondLetter);
// Output:
// true
In this code snippet, we compare two chars ‘A’ and ‘B’. Since ‘A’ comes before ‘B’ in the Unicode character set, the expression ‘firstLetter < secondLetter’ returns true.
These are just a few of the many ways you can manipulate and compare chars in Java. As you continue to explore and experiment, you’ll discover even more possibilities!
Exploring Alternative Ways to Work with Characters in Java
While the char data type is a powerful tool for handling single characters in Java, there are alternative approaches that offer additional functionality. Let’s explore two of these: the Character wrapper class and using Strings.
The Character Wrapper Class
Java provides a wrapper class, Character, that encapsulates a char value and provides numerous methods to manipulate and inspect characters. Here’s an example of using the Character class to convert a char to uppercase:
char lowercaseLetter = 'a';
char uppercaseLetter = Character.toUpperCase(lowercaseLetter);
System.out.println(uppercaseLetter);
// Output:
// 'A'
In this example, we use the toUpperCase method of the Character class to convert the lowercase char ‘a’ to an uppercase ‘A’.
Working with Strings
If you need to work with multiple characters, you can use a String, which is essentially a sequence of chars. Here’s an example of declaring a String and accessing a specific char within it:
String word = "Java";
char firstLetter = word.charAt(0);
System.out.println(firstLetter);
// Output:
// 'J'
In this code snippet, we declare a String ‘Java’ and then use the charAt method to access the first character of the String, which is ‘J’.
Benefits, Drawbacks, and Decision-Making Considerations
While these alternative approaches offer additional functionality, they come with their own trade-offs. The Character class provides a wealth of methods for character manipulation, but it also uses more memory than a simple char. Strings offer even more functionality and flexibility, but they are also more memory-intensive and can be slower to process than chars.
Choosing the right approach depends on your specific use case. If you’re dealing with single characters and memory usage is a concern, a char might be the best choice. If you need more advanced character manipulation capabilities, the Character class could be a better fit. And if you’re working with multiple characters, a String might be the way to go.
Troubleshooting Common Errors with Java Chars
Working with chars in Java can sometimes present challenges. Let’s address some common issues you might encounter and provide solutions to overcome them.
Error: Incorrect Declaration of Char
A common mistake when declaring a char in Java is using double quotes instead of single quotes. This will lead to a compile-time error. Here’s an example:
char wrongDeclaration = "A";
// Output:
// error: incompatible types: String cannot be converted to char
To fix this, always remember to use single quotes when declaring a char.
char correctDeclaration = 'A';
Error: Using a Char Like a String
Another common mistake is trying to use a char as if it were a String. For example, trying to use the substring method (which is a String method) on a char will result in an error:
char letter = 'A';
char partOfLetter = letter.substring(0, 1);
// Output:
// error: cannot find symbol
// symbol: method substring(int,int)
// location: variable letter of type char
To fix this, remember that chars in Java can hold a single character only. If you need to work with multiple characters or use String methods, consider using a String instead.
Considerations and Best Practices
When working with chars in Java, it’s important to keep a few key considerations in mind:
- Always use single quotes when declaring a char.
- Remember that chars are case-sensitive.
- Consider the memory usage of chars vs. the Character class or Strings.
- Be aware that chars in Java are actually Unicode values, which allows for manipulation and comparison.
Following these tips and understanding these considerations will help you avoid common pitfalls and write more effective, efficient Java code.
Understanding Java Char and Unicode
Before we delve deeper into the world of chars in Java, let’s take a step back and understand what a char is in Java and how it’s represented in memory.
What is a Char in Java?
In Java, a char is a primitive data type that’s used to represent a single 16-bit Unicode character. It can hold any character, such as a letter, a digit, or a special symbol, from the Unicode character set.
char letter = 'A';
char digit = '1';
char symbol = '$';
System.out.println(letter);
System.out.println(digit);
System.out.println(symbol);
// Output:
// 'A'
// '1'
// '$'
In the above example, we declare three chars: a letter, a digit, and a symbol. We then print out each char, which outputs ‘A’, ‘1’, and ‘$’, respectively.
How is a Char Represented in Memory?
Each char in Java takes up 2 bytes of memory. This is because the char data type in Java is based on the original Unicode specification, which requires 16 bits to represent each character.
The Relationship Between Char and Unicode
The char data type in Java was designed to support the original Unicode specification, which was a 16-bit character encoding. This means that each char in Java can represent a single Unicode character, from U+0000 to U+FFFF.
This allows Java to support a wide range of characters from various world languages, as well as special symbols. It’s one of the reasons why Java is such a powerful and versatile programming language.
Understanding the fundamentals of chars in Java, including what they are and how they’re represented in memory, is crucial to mastering their usage and unlocking their full potential. With this foundation, you’re well-equipped to handle chars in Java and use them to their fullest extent.
Applying Java Char in Real-World Programs
The use of chars in Java extends far beyond simple character representation. In larger Java programs, chars are used in a variety of ways, including string manipulation, file I/O operations, and much more.
For instance, when reading or writing to a text file in Java, you’re essentially dealing with a stream of chars. Understanding how to work with chars is crucial in these scenarios.
Moreover, string manipulation, one of the most common tasks in programming, is essentially the manipulation of chars. Whether you’re reversing a string, converting it to uppercase or lowercase, or removing special characters from it, you’re working with chars.
Related Topics for Further Reading
If you’re interested in diving deeper into the world of Java programming, there are several related topics that you might find interesting:
- String Manipulation: Since strings are sequences of chars, mastering string manipulation is a natural next step after learning about chars.
- File I/O: As mentioned earlier, when you’re reading or writing text files in Java, you’re working with chars.
Further Resources for Mastering Java Char
Here are some resources that offer more in-depth information about chars in Java and related topics:
- Tips and Techniques for Java Primitive Data Types – Learn about available methods for manipulating primitive data types.
Long Data Type in Java – Learn about the range and precision of long integers in Java.
Exploring Characters in Java – Master working with characters and strings in Java applications.
Oracle’s Java Documentation – The official documentation for Java, including a detailed section on chars.
JavaTpoint’s Java Char Tutorial offers an overview of chars in Java, including how to declare, initialize, and use them.
GeeksforGeeks’ Java Char Article provides a deep dive into the char data type in Java, including its background and usage.
Wrapping Up: Char Data Type
In this comprehensive guide, we’ve journeyed through the world of Java char, a fundamental data type used for representing single characters.
We started with the basics, learning how to declare and use chars in Java. We then ventured into more advanced territory, exploring complex operations such as manipulating and comparing chars. We also discussed alternative approaches to working with characters in Java, such as using the Character wrapper class and Strings.
Along the way, we addressed common issues that you might encounter when working with chars in Java, such as incorrect declaration and misuse of chars, providing you with solutions and best practices for each issue.
We also delved into the fundamentals of chars in Java, including what they are, how they’re represented in memory, and their relationship with the Unicode character set. Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Using char | Efficient memory usage, supports Unicode | Limited to single characters |
Using Character class | Provides additional methods for character manipulation | Uses more memory than char |
Using String | Can handle multiple characters, offers extensive functionality | More memory-intensive, slower to process than char |
Whether you’re just starting out with Java char or you’re looking to level up your character handling skills, we hope this guide has given you a deeper understanding of Java char and its capabilities.
With its balance of efficiency, versatility, and support for Unicode, Java char is a powerful tool for character handling in Java. Happy coding!