Java Float Keyword Explained: Usage and Examples
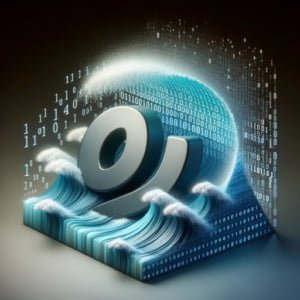
Are you finding it challenging to understand the float data type in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling float in Java, but we’re here to help.
Think of Java’s float as a lightweight container – a container that can hold decimal numbers with ease. It’s a crucial part of Java that plays a significant role in various programming tasks.
In this guide, we’ll walk you through the ins and outs of the float data type in Java, from its basic usage to advanced topics. We’ll cover everything from declaring and initializing float variables, handling precision and rounding errors, to alternative approaches and best practices.
Let’s dive in and start mastering Java float!
TL;DR: What is the ‘Float’ Keyword in Java?
In Java, the float keyword identifies a data type that can hold decimal numbers, created with the
float
keyword before the variable name, and must have an ‘f’ after the value, such asfloat num = 1.11f
. The ‘f’ after the value tells Java that the value is a float.
Here’s a simple example of declaring and initializing a float variable in Java:
float num = 1.11f;
In this example, we declare a float variable named ‘num’ and initialize it with the value 1.11. The ‘f’ at the end of the number is necessary because Java interprets decimal numbers as double by default. The ‘f’ suffix tells Java that this is a float.
This is just the tip of the iceberg when it comes to using float in Java. There’s much more to learn about precision, rounding errors, and alternative approaches. So, let’s dive deeper!
Table of Contents
- Understanding the Float Data Type in Java
- Harnessing Float for Mathematical Operations
- Precision and Rounding Errors in Float
- Best Practices When Using Float
- Exploring the Double Data Type in Java
- Float vs Double: Which to Use?
- Handling Common Issues with Java Float
- Understanding Floating-Point Numbers and the IEEE 754 Standard
- Applying Java Float in Real-World Programming
- Wrapping Up:
Understanding the Float Data Type in Java
In Java, float is a primitive data type. It’s designed to hold decimal numbers, making it a crucial tool in any programmer’s toolkit. But before we dive deeper, let’s understand some key properties of float.
A float in Java:
- Is a single-precision 32-bit IEEE 754 floating point.
- Has a size of 4 bytes.
- Can hold values ranging from 1.4e-45 to 3.4e+38.
- Is precise up to 6-7 decimal places.
Now, let’s see a simple example of declaring and initializing a float variable in Java:
float num = 3.14f;
System.out.println(num);
// Output:
// 3.14
In this example, we declare a float variable named ‘num’ and initialize it with the value 3.14. You might notice the ‘f’ at the end of 3.14. This is because Java interprets decimal numbers as double by default. The ‘f’ suffix tells Java that this is a float, not a double.
This basic understanding of float will help you navigate more complex scenarios as we move to more advanced topics.
Harnessing Float for Mathematical Operations
Java’s float data type is not limited to just storing decimal numbers. It can also be used for various mathematical operations such as addition, subtraction, multiplication, and division. Let’s look at an example:
float num1 = 3.14f;
float num2 = 2.73f;
float result = num1 + num2;
System.out.println(result);
// Output:
// 5.87
In this example, we’ve added two float numbers together. The operation is straightforward and behaves just like you’d expect.
Precision and Rounding Errors in Float
However, it’s essential to understand that float has its limitations, particularly when it comes to precision and rounding errors. This is due to the way floating-point numbers are represented in memory according to the IEEE 754 standard.
Let’s look at an example that demonstrates this:
float num1 = 1.2345678f;
float num2 = 2.2345678f;
float result = num1 + num2;
System.out.println(result);
// Output:
// 3.4691358
As you can see, the result is not exactly 3.4691356, as you might expect. This discrepancy is due to the limited precision of the float data type.
Best Practices When Using Float
To mitigate these issues, it’s best to use float only when the precision provided is sufficient for your needs. For high-precision arithmetic, consider using the double data type or the BigDecimal class in Java, which we’ll discuss later in this guide.
Exploring the Double Data Type in Java
While the float data type is a powerful tool, Java provides another data type for holding decimal numbers: the double. The double data type is similar to float, but it provides more precision.
A double in Java:
- Is a double-precision 64-bit IEEE 754 floating point.
- Has a size of 8 bytes.
- Can hold values ranging from 4.9e-324 to 1.8e+308.
- Is precise up to 15 decimal places.
Let’s see a simple example of declaring and initializing a double variable in Java:
double num = 3.14;
System.out.println(num);
// Output:
// 3.14
In contrast to float, you don’t need to add any suffix when declaring a double. This is because Java interprets decimal numbers as double by default.
Float vs Double: Which to Use?
The choice between float and double depends on the precision you need for your calculations. If you’re working with numbers that require high precision, such as in scientific calculations, it’s better to use double. On the other hand, if you’re dealing with numbers that don’t require a high degree of precision, such as in graphics programming, using float can save memory.
Here’s a comparison of float and double in action:
float floatNum = 1.2345678f;
double doubleNum = 1.2345678;
System.out.println(floatNum);
System.out.println(doubleNum);
// Output:
// 1.2345678
// 1.2345678
In this example, both floatNum and doubleNum hold the same value. However, if we were to use a longer decimal number, the difference in precision would become apparent.
Handling Common Issues with Java Float
While using float in Java, you may encounter a few common issues. The most prevalent of these are precision loss and rounding errors. Let’s delve into these problems and their solutions.
Dealing with Precision Loss
As we’ve mentioned before, float in Java is precise up to 6-7 decimal places. But what happens when we need more precision? Precision loss occurs. Consider the following example:
float num = 1.23456789f;
System.out.println(num);
// Output:
// 1.2345679
In this example, we’ve tried to store a number with 8 decimal places in a float variable. But when we print it out, we see that the number has been rounded to 7 decimal places. This is a classic example of precision loss.
Rounding Errors in Float
Another common issue with float is rounding errors. This happens due to the way floating-point numbers are represented in memory, which can sometimes lead to unexpected results. Here’s an example:
float num1 = 1.2345678f;
float num2 = 0.0000002f;
float result = num1 + num2;
System.out.println(result);
// Output:
// 1.2345679
In this example, we’re adding a very small number to our float number. The result should be slightly larger than our original number, but instead, it’s rounded to the same value. This is a rounding error.
Best Practices for Using Float
To avoid these issues, it’s crucial to understand when to use float and when to consider alternatives. As a rule of thumb, use float when you don’t need high precision and want to save memory. For high-precision calculations, consider using double or the BigDecimal class in Java.
Understanding Floating-Point Numbers and the IEEE 754 Standard
Before we delve further into the intricacies of the float data type in Java, it’s crucial to understand the concept of floating-point numbers and the IEEE 754 standard that governs them.
Floating-Point Numbers: A Primer
In the simplest terms, floating-point numbers are real numbers that have a decimal point. They can be positive, negative, or zero. In Java, float and double are the two primitive data types used to represent these numbers.
The IEEE 754 Standard Explained
The IEEE 754 standard is a widely adopted standard for floating-point arithmetic established by the Institute of Electrical and Electronics Engineers (IEEE). It defines how floating-point numbers are represented in memory, as well as rules for rounding, overflow, underflow, and several special values (such as Infinity and NaN).
In the context of Java’s float, it’s a single-precision 32-bit IEEE 754 floating point. This means it uses 1 bit for sign, 8 bits for exponent, and 23 bits for the fraction.
Let’s see a simple example of this representation:
float num = 1.5f;
// In memory, 1.5 is represented as follows:
// Sign: 0 (as it's a positive number)
// Exponent: 127 (bias for single-precision)
// Fraction: 10011001100110011001101 (binary representation of 1.5)
This deep understanding of floating-point numbers and the IEEE 754 standard is crucial for mastering the use of float in Java and troubleshooting any issues that might arise.
Applying Java Float in Real-World Programming
The float data type in Java is not just a theoretical concept. It finds extensive use in real-world Java programming, especially in areas where handling decimal numbers is crucial.
Float in Graphics Programming
In graphics programming, float is often used to represent coordinates, dimensions, and other properties. The precision provided by float is usually sufficient for these applications, and using float instead of double can save memory.
Float in Physics Simulations
Physics simulations often involve calculations with real numbers. Here, float can be used to represent quantities such as mass, velocity, and force. However, due to the issues of precision and rounding errors, double might be a better choice for high-precision simulations.
Float in Financial Calculations
In financial calculations, accuracy is paramount. While you could use float for simple calculations, it’s generally not recommended due to the risk of precision loss. Instead, consider using the BigDecimal class in Java, which provides arbitrary-precision arithmetic.
import java.math.BigDecimal;
BigDecimal num1 = new BigDecimal("1.23456789");
BigDecimal num2 = new BigDecimal("0.00000001");
BigDecimal result = num1.add(num2);
System.out.println(result);
// Output:
// 1.23456790
In this example, we’re using BigDecimal to add two numbers with high precision. As you can see, the result is accurate to the last decimal place.
Further Resources for Mastering Java Float
To deepen your understanding of the float data type in Java and its applications, consider exploring the following resources:
- IOFlood’s Article on Java Primitive Data Types explores the char data type for representing single characters in Java.
Double Data Type in Java – Understand the increased precision and range of double compared to float.
Working with Booleans in Java – Master using boolean for conditional statements and decision-making in Java code.
The official Java documentation provides a comprehensive overview of all data types in Java, including float.
Baeldung’s Guide to Java’s BigDecimal is an excellent resource for understanding high-precision arithmetic in Java.
GeeksforGeeks’ Article on floating point representation explains the IEEE 754 standard in detail, which is crucial for understanding the behavior of float.
Wrapping Up:
In this comprehensive guide, we’ve navigated the ins and outs of the float data type in Java, from its basic usage to advanced topics.
We began with understanding the concept of float in Java, including its size, range, and precision. We saw how to declare and initialize a float variable and discussed the importance of the ‘f’ suffix. We then delved into more advanced uses, discussing how to use float for various mathematical operations and the issues of precision and rounding errors.
We explored alternative approaches, introducing the double data type in Java, which is also used for holding decimal numbers but with more precision. We discussed the differences between float and double, and when to use each one.
We tackled common issues related to using float in Java, such as precision loss and rounding errors, and provided solutions and workarounds. We also discussed best practices when using float in Java.
Finally, we went beyond the basics, discussing the application of float in real-world Java programming, such as graphics, physics simulations, and financial calculations.
Here’s a quick comparison of float and double in Java:
Data Type | Precision | Size | Range |
---|---|---|---|
Float | 6-7 decimal places | 4 bytes | 1.4e-45 to 3.4e+38 |
Double | 15 decimal places | 8 bytes | 4.9e-324 to 1.8e+308 |
Whether you’re just starting out with Java’s float or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the float data type in Java and its applications.
With its balance of precision and memory efficiency, float is a powerful tool for handling decimal numbers in Java. Happy coding!