Understanding and Using Java Predicate: A Detailed Guide
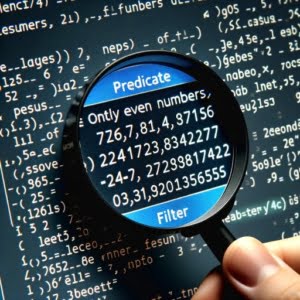
Are you finding it challenging to work with Java Predicates? You’re not alone. Many developers find themselves puzzled when it comes to using Java Predicates, but we’re here to help.
Think of Java Predicates as your personal data detective – they can help you filter or evaluate data efficiently. Whether you’re dealing with collections, streams, or any other form of data, Java Predicates can be a powerful tool in your arsenal.
This guide will walk you through the process of understanding and using Java Predicates effectively, from the basics to more advanced techniques. We’ll cover everything from creating a Predicate, using the test() method, to more complex uses such as chaining Predicates using and(), or(), and negate().
So, let’s dive in and start mastering Java Predicates!
TL;DR: What is a Java Predicate?
A Java Predicate is a functional interface that represents a boolean-valued function of one argument. It is instantiated by using the
Predicate
keyword followed by Constructor and variable name, for example:Predicate<String> lengthIsEven
. It is commonly used to filter data.
Here’s a simple example:
Predicate<String> lengthIsEven = s -> s.length() % 2 == 0;
boolean result = lengthIsEven.test("Hello");
// Output:
// false
In this example, we’ve created a Predicate lengthIsEven
that checks if the length of a string is even. We then test this Predicate with the string “Hello”, which has 5 characters, an odd number. Therefore, the result is false
.
This is just a basic way to use Java Predicates, but there’s so much more to explore. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with Java Predicates
- Chaining Java Predicates: and(), or(), negate()
- Exploring Alternative Data Filtering Techniques
- Troubleshooting Common Java Predicate Issues
- Delving into Java’s Functional Interfaces and Lambda Expressions
- Java Predicates in Larger Applications
- Exploring Related Concepts
- Wrapping Up: Mastering Java Predicates
Getting Started with Java Predicates
Java Predicates are a powerful tool for working with data, especially when you need to filter or evaluate it. Let’s break down how to use them, starting with the basics.
Creating a Java Predicate
A Java Predicate is a functional interface that can be defined using a lambda expression. Here’s an example of a simple Predicate that checks if a string has an even length:
Predicate<String> lengthIsEven = s -> s.length() % 2 == 0;
In this code, lengthIsEven
is a Predicate that takes a string s
and checks if its length is an even number. We use the lambda expression s -> s.length() % 2 == 0
to define this logic.
Using the test() Method
Once we have our Predicate, we can use it to evaluate data using the test()
method. This method takes an input and applies the Predicate’s condition to it. Here’s how we can use our lengthIsEven
Predicate to evaluate a string:
boolean result = lengthIsEven.test("Hello");
System.out.println(result);
// Output:
// false
In this example, we’re testing the string “Hello” with our lengthIsEven
Predicate. Since “Hello” has 5 characters (an odd number), the result is false
.
Filtering Data with Java Predicates
Java Predicates are often used to filter data. For instance, you can use them to filter a collection of items that match a certain condition. Here’s an example of using a Predicate to filter a list of numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
Predicate<Integer> isEven = n -> n % 2 == 0;
List<Integer> evenNumbers = numbers.stream()
.filter(isEven)
.collect(Collectors.toList());
System.out.println(evenNumbers);
// Output:
// [2, 4, 6]
In this example, we’ve created a Predicate isEven
that checks if a number is even. We then use this Predicate to filter our list of numbers and collect the even ones into a new list. The result is a list of the even numbers: [2, 4, 6]
.
Chaining Java Predicates: and(), or(), negate()
As you become more comfortable with Java Predicates, you can start to use more advanced techniques. One such technique is chaining Predicates together using the and()
, or()
, and negate()
methods. These methods allow you to combine multiple Predicates into a single, more complex Predicate.
Chaining with and()
The and()
method allows you to chain two Predicates together. The resulting Predicate will return true
if and only if both of the original Predicates return true
. Here’s an example:
Predicate<String> startsWithJ = s -> s.startsWith("J");
Predicate<String> hasLengthOf5 = s -> s.length() == 5;
Predicate<String> startsWithJAndHasLengthOf5 = startsWithJ.and(hasLengthOf5);
boolean result = startsWithJAndHasLengthOf5.test("Java");
System.out.println(result);
// Output:
// false
In this example, we first define two Predicates: startsWithJ
, which checks if a string starts with “J”, and hasLengthOf5
, which checks if a string has a length of 5. We then chain these two Predicates together using the and()
method to create a new Predicate startsWithJAndHasLengthOf5
. When we test this Predicate with the string “Java”, the result is false
because while “Java” does start with “J”, it does not have a length of 5.
Chaining with or()
The or()
method works similarly to and()
, but it returns true
if either of the original Predicates return true
. Here’s how you might use it:
Predicate<String> startsWithJOrHasLengthOf5 = startsWithJ.or(hasLengthOf5);
boolean result = startsWithJOrHasLengthOf5.test("Java");
System.out.println(result);
// Output:
// true
In this example, we use the or()
method to chain together our startsWithJ
and hasLengthOf5
Predicates. This time, when we test the resulting Predicate with the string “Java”, the result is true
because “Java” does start with “J”, even though it does not have a length of 5.
Negating with negate()
Finally, the negate()
method allows you to invert the result of a Predicate. Here’s an example:
Predicate<String> notStartsWithJ = startsWithJ.negate();
boolean result = notStartsWithJ.test("Java");
System.out.println(result);
// Output:
// false
In this example, we use the negate()
method to create a new Predicate notStartsWithJ
that returns true
if a string does not start with “J”. When we test this Predicate with the string “Java”, the result is false
because “Java” does start with “J”.
Exploring Alternative Data Filtering Techniques
Java offers multiple ways to filter data, not just with Predicates. Let’s explore some alternative approaches and compare them with using Java Predicates.
Filtering with Loops
A simple way to filter data is by using loops. Here’s an example of filtering a list of numbers to only include even numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = new ArrayList<>();
for (Integer number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number);
}
}
System.out.println(evenNumbers);
// Output:
// [2, 4, 6]
In this example, we’re looping through each number in our list and adding it to a new list if it’s even. This approach is straightforward, but it can become cumbersome and less readable as the complexity of the condition increases.
Filtering with Streams (Without Predicates)
Java Streams provide a more declarative way to filter data. Here’s how you can achieve the same result as above using a Stream:
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers);
// Output:
// [2, 4, 6]
In this example, we’re using the filter()
method of the Stream API to filter our list of numbers. The argument to the filter()
method is a lambda expression that defines our condition. This approach is more streamlined and easier to read than using a loop, especially for more complex conditions.
Comparing with Java Predicates
Both of the above methods can be used to filter data, but Java Predicates offer some advantages. Predicates are more flexible because they can be passed around as arguments and returned as results from methods. They can also be combined and reused in different contexts.
For example, once we have a Predicate like isEven
, we can use it in multiple places throughout our code. This is not possible with the conditions defined inside a loop or a Stream’s filter()
method.
In summary, while loops and Streams can be used to filter data in Java, Predicates offer a more flexible and reusable approach, especially for complex conditions and larger applications.
Troubleshooting Common Java Predicate Issues
As with any programming concept, there are potential pitfalls when using Java Predicates. Let’s discuss some common issues and how to avoid them.
NullPointerExceptions with Method References
One common issue is encountering a NullPointerException
when using method references with Predicates. This usually happens when you’re dealing with a collection that contains null
values. Here’s an example:
List<String> strings = Arrays.asList("Hello", null);
Predicate<String> isShortWord = s -> s.length() < 4;
strings.stream()
.filter(isShortWord)
.forEach(System.out::println);
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, we’re trying to filter a list of strings to only include short words. However, our list contains a null
value, which causes a NullPointerException
when we try to call the length()
method on it.
To avoid this issue, you can add an additional check to your Predicate to ignore null
values:
Predicate<String> isShortWord = s -> s != null && s.length() < 4;
strings.stream()
.filter(isShortWord)
.forEach(System.out::println);
// Output:
// (no output)
In this updated example, our isShortWord
Predicate first checks if a string is not null
before trying to get its length. This prevents the NullPointerException
and allows our code to run successfully.
Considerations When Chaining Predicates
When chaining Predicates using and()
, or()
, and negate()
, keep in mind that the order of operations can affect the result. For example, the and()
operation is not commutative when dealing with null
values:
Predicate<String> isNotNull = Objects::nonNull;
Predicate<String> startsWithJ = s -> s.startsWith("J");
Predicate<String> correctOrder = isNotNull.and(startsWithJ);
Predicate<String> incorrectOrder = startsWithJ.and(isNotNull);
System.out.println(correctOrder.test(null)); // false
System.out.println(incorrectOrder.test(null)); // NullPointerException
In this example, correctOrder
checks if a string is not null
before checking if it starts with “J”. This prevents a NullPointerException
from being thrown when we test it with null
. On the other hand, incorrectOrder
tries to check if null
starts with “J” before checking if it’s not null
, which throws a NullPointerException
.
In summary, when using Java Predicates, be mindful of potential NullPointerExceptions
and the order of operations when chaining Predicates.
Delving into Java’s Functional Interfaces and Lambda Expressions
To fully grasp how Java Predicates work, we need to understand the concepts of functional interfaces and lambda expressions in Java, and how Predicates fit into these concepts.
Understanding Functional Interfaces
A functional interface in Java is an interface that contains exactly one abstract method. This concept is a fundamental part of Java’s support for lambda expressions and method references.
Java Predicates are a perfect example of a functional interface. The java.util.function.Predicate
interface contains one abstract method, test()
, which takes an object and returns a boolean.
@FunctionalInterface
public interface Predicate<T> {
boolean test(T t);
}
In this code, T
is the type of the object the Predicate evaluates, and test()
is the method that performs the evaluation and returns the result.
Leveraging Lambda Expressions
Lambda expressions are a feature in Java that allow you to write shorter and more readable code when working with functional interfaces. They provide a concise syntax to create anonymous methods.
When defining a Predicate, we often use lambda expressions. For example, here’s how we might define a Predicate that checks if a number is even:
Predicate<Integer> isEven = n -> n % 2 == 0;
In this code, n -> n % 2 == 0
is a lambda expression. It takes an integer n
and returns true
if n
is even and false
otherwise.
Lambda expressions and functional interfaces are key to understanding and using Java Predicates effectively. They provide a flexible and powerful way to define conditions and evaluate data.
Java Predicates in Larger Applications
Java Predicates are not just useful for small tasks or simple programs. They play a vital role in larger applications, especially in data processing and filtering within business logic.
Imagine you’re developing a large-scale application that deals with a significant amount of data. You need to filter this data based on various complex conditions. Java Predicates can make this task more manageable and your code more readable and maintainable.
For instance, you could define a set of Predicates that each represent a different business rule. You can then combine these Predicates in various ways to implement complex business logic. This approach is not only efficient but also makes your code easier to understand and modify.
Exploring Related Concepts
Java Predicates are part of a larger ecosystem of functional programming features in Java. To get the most out of them, it’s worth exploring related concepts like Java Streams and other functional interfaces.
Java Streams, for example, work hand-in-hand with Predicates to provide powerful and efficient data processing capabilities. Other functional interfaces, like Function
, Supplier
, and Consumer
, can also be used in combination with Predicates to build complex data processing pipelines.
Further Resources for Java Predicate Proficiency
To deepen your understanding of Java Predicates and related concepts, here are some resources you might find helpful:
- IOFlood’s Complete Java Interfaces Guide – Understand the significance of interface segregation in Java.
Java Iterable Interface: Guide – Understand the Java Iterable interface for iterating over collections of elements.
Establishing JDBC Connection in Java – Understand connection pooling and best practices for managing JDBC connections.
Java 8 Lambda Expressions & Streams – A quick start guide by Oracle on lambda expressions and streams in Java 8.
Java 8 Functional Interfaces – An in-depth article by Baeldung that covers various functional interfaces in Java 8.
Java Predicate Documentation – The official Java documentation for the
Predicate
interface.
Wrapping Up: Mastering Java Predicates
In this comprehensive guide, we’ve dived deep into the world of Java Predicates, a powerful tool for data evaluation and filtering in Java.
We kicked off with the basics, learning how to create and utilize Java Predicates, and then using the test()
method to evaluate data. We provided practical examples along the way, showing how to filter data using Predicates.
We then ventured into advanced territory, exploring how to chain Predicates using and()
, or()
, and negate()
. We also discussed alternative approaches to data filtering in Java, comparing the use of loops and Streams with Predicates.
We tackled common issues that you might encounter when using Java Predicates, such as NullPointerExceptions
and order of operations when chaining Predicates, providing you with solutions and considerations for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Reusability | Complexity |
---|---|---|---|
Java Predicates | High | High | Moderate |
Loops | Low | Low | Low |
Streams | Moderate | Moderate | High |
Whether you’re just starting out with Java Predicates or you’re looking to level up your data evaluation and filtering skills, we hope this guide has given you a deeper understanding of Java Predicates and their capabilities.
With their flexibility and reusability, Java Predicates are a powerful tool for data evaluation and filtering in Java. Now, you’re well equipped to enjoy these benefits. Happy coding!