binarySearch() Java: Locating Elements in Sorted Arrays
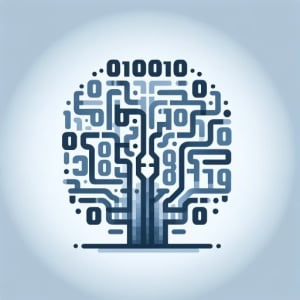
Are you finding it challenging to implement binary search in Java? You’re not alone. Many developers find themselves in a maze when it comes to binary search in Java, but we’re here to help.
Think of binary search as a detective, helping you find the ‘suspect’ (element) in a sorted ‘lineup’ (array) in the most efficient way. It’s a powerful tool that can significantly speed up your search process in sorted arrays.
In this guide, we’ll walk you through the process of implementing and using binary search in Java, from basic use to advanced techniques. We’ll cover everything from the logic behind binary search, how to write the code, to more complex uses and common issues you may encounter.
So, let’s dive in and start mastering binary search in Java!
TL;DR: How Do I Implement Binary Search in Java?
The simplest way to perform a binary search in Java is by using the
Arrays.binarySearch()
method from the Java standard library, with the syntaxint result = Arrays.binarySearch(arrayToSearch, keyToSearch);
.
Here’s a basic example:
int[] arr = {10, 20, 30, 40, 50};
int key = 30;
int result = Arrays.binarySearch(arr, key);
// Output:
// 2
In this example, we’ve created an array arr
and a key key
that we want to find in the array. We then use the Arrays.binarySearch()
method to find the index of the key in the array. The method returns the index of the key, which is 2 in this case.
This is a basic way to use binary search in Java, but there’s much more to learn about implementing and using binary search in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Implementing Binary Search in Java: The Basics
- Binary Search in Java: Advanced Techniques
- Exploring Alternative Approaches to Binary Search in Java
- Troubleshooting Binary Search in Java
- Understanding Binary Search: The Basics
- Broader Applications of Binary Search in Java
- Wrapping Up: Binary Search in Java
Implementing Binary Search in Java: The Basics
Binary search is a divide-and-conquer algorithm that reduces the search space by half at each step. It starts by comparing the middle element of a sorted array with the target value. If the target value matches the middle element, its position in the array is returned. If the target value is less or more than the middle element, the search continues on the lower or upper half of the array, respectively.
Now, let’s dive into how to implement this algorithm in Java.
Step-by-Step Guide to Writing Binary Search Code
Here’s a simple code example of how to implement binary search in Java:
public class BinarySearch {
int binarySearch(int arr[], int x) {
int l = 0, r = arr.length - 1;
while (l <= r) {
int m = l + (r - l) / 2;
// Check if x is present at mid
if (arr[m] == x)
return m;
// If x greater, ignore left half
if (arr[m] < x)
l = m + 1;
// If x is smaller, ignore right half
else
r = m - 1;
}
// if we reach here, then element was not present
return -1;
}
}
// Driver method to test above
public static void main(String args[]) {
BinarySearch bs = new BinarySearch();
int arr[] = {2, 3, 4, 10, 40};
int n = arr.length;
int x = 10;
int result = bs.binarySearch(arr, x);
if(result == -1)
System.out.println("Element not present in array");
else
System.out.println("Element found at index " + result);
}
// Output:
// Element found at index 3
In this example, we’ve created a BinarySearch
class with a binarySearch
method. This method takes an array arr[]
and a target value x
as parameters. It starts by defining the lower (l
) and upper (r
) bounds of the search space. Then, it enters a while loop where it calculates the middle index m
and compares the middle element arr[m]
with the target value x
. Depending on the comparison, it either returns the middle index or adjusts the search space. If the target value is not found, it returns -1.
Binary Search in Java: Advanced Techniques
As you become more comfortable with the basics of binary search in Java, you can start to explore more complex uses. Two such uses include searching in a 2D array and using binary search with custom objects.
Binary Search in 2D Arrays
Implementing binary search on a 2D array is a bit more complex than a 1D array, but it’s still feasible. The idea is to apply binary search on both dimensions of the 2D array.
Here’s an example of how you can implement binary search in a 2D array:
public class BinarySearch2D {
int[] binarySearch(int matrix[][], int target) {
int row = matrix.length;
int col = matrix[0].length;
int low = 0;
int high = row*col - 1;
while(low <= high) {
int mid = low + (high - low) / 2;
int mid_element = matrix[mid/col][mid%col];
if(target == mid_element)
return new int[]{mid/col, mid%col};
else if(target < mid_element)
high = mid - 1;
else
low = mid + 1;
}
return new int[]{-1, -1};
}
}
// Driver method to test above
public static void main(String args[]) {
BinarySearch2D bs2d = new BinarySearch2D();
int matrix[][] = {{1, 3, 5}, {7, 9, 11}, {13, 15, 17}};
int target = 15;
int result[] = bs2d.binarySearch(matrix, target);
if(result[0] == -1)
System.out.println("Element not present in matrix");
else
System.out.println("Element found at index " + Arrays.toString(result));
}
// Output:
// Element found at index [2, 1]
In this example, we’ve created a BinarySearch2D
class with a binarySearch
method. This method takes a 2D array matrix[][]
and a target value target
as parameters. It starts by defining the lower (low
) and upper (high
) bounds of the search space, which is the total number of elements in the matrix. Then, it enters a while loop where it calculates the middle index mid
and the middle element mid_element
. Depending on the comparison between mid_element
and target
, it either returns the indices of mid_element
or adjusts the search space. If the target value is not found, it returns [-1, -1].
Binary Search with Custom Objects
In Java, you can also use binary search with custom objects. The key is to provide a way for the Collections.binarySearch()
method to compare the custom objects. You can do this by making your custom class implement the Comparable
interface or by providing a custom Comparator
.
Here’s how you can implement binary search with custom objects:
import java.util.Arrays;
import java.util.Comparator;
// Custom class
class Point {
int x, y;
// Constructor
public Point(int x, int y) {
this.x = x;
this.y = y;
}
}
// Custom comparator
class MyComparator implements Comparator<Point> {
public int compare(Point p1, Point p2) {
return p1.x - p2.x;
}
}
// Driver method to test above
public static void main(String args[]) {
Point arr[] = {new Point(10, 20), new Point(30, 40), new Point(50, 60)};
Point key = new Point(30, 40);
int result = Arrays.binarySearch(arr, key, new MyComparator());
if(result < 0)
System.out.println("Element not present in array");
else
System.out.println("Element found at index " + result);
}
// Output:
// Element found at index 1
In this example, we’ve created a custom Point
class and a custom MyComparator
class. The Point
class represents points in a 2D space, and the MyComparator
class defines how to compare two Point
objects. We then create an array of Point
objects and use the Arrays.binarySearch()
method with the custom comparator to find the index of a target Point
object in the array.
Exploring Alternative Approaches to Binary Search in Java
In Java, there are several alternative methods you can use to perform a binary search. Two of these methods include using the Collections.binarySearch()
method for lists and implementing an iterative binary search. Let’s delve into these methods.
Binary Search on Lists Using Collections.binarySearch()
The Collections.binarySearch()
method is a powerful tool for performing binary search on lists. This method assumes that the list is already sorted in ascending order.
Here’s an example of how you can use Collections.binarySearch()
:
import java.util.Arrays;
import java.util.List;
import java.util.Collections;
public class BinarySearchList {
public static void main(String[] args) {
List<Integer> list = Arrays.asList(2, 4, 6, 8, 10, 12, 14, 16);
int key = 10;
int result = Collections.binarySearch(list, key);
System.out.println("Element found at index " + result);
}
}
// Output:
// Element found at index 4
In this example, we’ve created a list of integers and used the Collections.binarySearch()
method to find the index of a target integer in the list. The method returns the index of the target integer, which is 4 in this case.
Implementing Iterative Binary Search
An iterative binary search is another alternative approach to recursive binary search. This method uses a while loop instead of recursion to find the target element.
Here’s an example of how you can implement an iterative binary search in Java:
public class IterativeBinarySearch {
int binarySearch(int arr[], int x) {
int l = 0, r = arr.length - 1;
while (l <= r) {
int m = l + (r - l) / 2;
if (arr[m] == x)
return m;
if (arr[m] < x)
l = m + 1;
else
r = m - 1;
}
return -1;
}
}
// Driver method to test above
public static void main(String args[]) {
IterativeBinarySearch ibs = new IterativeBinarySearch();
int arr[] = {2, 3, 4, 10, 40};
int x = 10;
int result = ibs.binarySearch(arr, x);
if(result == -1)
System.out.println("Element not present in array");
else
System.out.println("Element found at index " + result);
}
// Output:
// Element found at index 3
In this example, the binarySearch
method implements an iterative binary search. It takes an array arr[]
and a target value x
as parameters. It uses a while loop to find the index of the target value in the array. If the target value is not found, it returns -1.
As you can see, each of these methods has its own benefits and use cases. The Collections.binarySearch()
method is great for searching in lists, while the iterative binary search can sometimes be easier to understand and debug than a recursive binary search. The best method to use depends on your specific needs and circumstances.
Troubleshooting Binary Search in Java
Even with the best understanding and implementation of binary search in Java, you may still encounter some common issues. Let’s discuss these potential pitfalls and provide solutions to overcome them.
Off-By-One Errors
One of the most common issues you might face when implementing binary search in Java is the infamous off-by-one error. This error typically occurs when you miscalculate the middle index or incorrectly define the boundaries of your search space.
To avoid off-by-one errors, always ensure that your middle index calculation (int m = l + (r - l) / 2;
) is correct, and that your search space boundaries (l
and r
) are appropriately defined and updated.
Unsorted Arrays
Binary search in Java requires the array to be sorted before the search. If your array is not sorted, the binary search algorithm will not work correctly.
Always ensure that your array is sorted in ascending order before performing a binary search. You can use Java’s built-in methods such as Arrays.sort(arr)
to sort an array.
Handling Duplicate Elements
If your array contains duplicate elements, the binary search algorithm may not return the index of the first occurrence of the target element. Instead, it might return the index of any occurrence.
If you need to find the first or last occurrence of a target element in an array with duplicate elements, you may need to modify your binary search algorithm or use a different search algorithm.
Array Index Out of Bounds
Another common issue is the ArrayIndexOutOfBoundsException, which occurs when you try to access an array element using an invalid index. This can happen if your search space boundaries (l
and r
) are incorrectly defined or updated.
Always ensure that your search space boundaries are within the valid range of indices for your array.
Implementing binary search in Java can be tricky, but with these troubleshooting tips and best practices, you can avoid common issues and ensure that your binary search algorithm works correctly.
Understanding Binary Search: The Basics
Before we delve deeper into the implementation of binary search in Java, it’s crucial to understand the fundamentals of the binary search algorithm itself and its time complexity.
The Binary Search Algorithm
Binary search is a fast search algorithm that finds the position of a target value within a sorted array. It works by repeatedly dividing the search interval in half and comparing the target value with the middle element of the current search interval.
If the target value matches the middle element, its position in the array is returned. If the target value is less or more than the middle element, the search continues on the lower or upper half of the array, respectively.
Here’s a simple illustration of how the binary search algorithm works:
// Initial array (sorted)
int[] arr = {2, 3, 4, 10, 40, 55, 78, 99};
// Target value
int key = 40;
// Binary search process
// Step 1: Compare key with middle element (10). Key is larger, so consider right half.
// Step 2: In the right half, compare key with middle element (55). Key is smaller, so consider left half.
// Step 3: In the new left half, compare key with middle element (40). Key matches, so return index.
In this example, the binary search algorithm successfully finds the target value (40) in the sorted array after three steps.
Time Complexity of Binary Search
The time complexity of binary search is O(log n), where n is the number of elements in the array. This is because with each step, the binary search algorithm halves the search space. This division process can be repeated approximately log n times until the search space is reduced to just one element.
The logarithmic time complexity of binary search makes it very efficient for large arrays. For example, for an array with a million elements, binary search can find the target value in just 20 steps in the worst case scenario.
The Importance of Sorting in Binary Search
Binary search relies on the array being sorted in ascending order. This is because the algorithm works by comparing the target value with the middle element and then deciding whether to continue the search on the left or right half of the array. If the array is not sorted, this decision process will not work correctly, and the algorithm may not find the target value even if it’s present in the array.
In conclusion, binary search is a powerful and efficient search algorithm that can significantly speed up the search process in sorted arrays. Understanding the basics of this algorithm and its time complexity is crucial for implementing it correctly and effectively in Java.
Broader Applications of Binary Search in Java
Binary search in Java is not limited to finding elements in arrays. The algorithm’s efficiency and speed make it suitable for a variety of applications, including data analysis and machine learning.
Binary Search in Data Analysis
In data analysis, binary search can be used to quickly find specific data points in large datasets. For instance, if you have a sorted dataset of user activity on a website, you could use binary search to quickly find the activities of a specific user or within a specific time frame.
// Simplified example - finding user activity
UserActivity[] activities = ...; // Sorted array of user activities
UserActivity key = ...; // The target user activity
int index = Arrays.binarySearch(activities, key, new UserActivityComparator());
// Output:
// Index of the target user activity or -1 if not found
In this example, UserActivity
is a custom class that represents user activities, and UserActivityComparator
is a custom comparator that defines how to compare two UserActivity
objects. The Arrays.binarySearch()
method is then used to find the index of a target UserActivity
object in the array.
Binary Search in Machine Learning
In machine learning, binary search can be used in decision trees, which are a type of model used for classification and regression. Each node in the decision tree represents a binary decision, effectively forming a binary search algorithm.
// Simplified example - decision tree node
public class DecisionTreeNode {
DecisionTreeNode leftChild, rightChild;
Decision decision;
public DecisionTreeNode(DecisionTreeNode leftChild, DecisionTreeNode rightChild, Decision decision) {
this.leftChild = leftChild;
this.rightChild = rightChild;
this.decision = decision;
}
}
// Output:
// A node in a decision tree, representing a binary decision
In this example, DecisionTreeNode
is a class that represents a node in a decision tree, and Decision
is a class that represents a binary decision. Each DecisionTreeNode
has a left child, a right child, and a decision, forming a binary search tree structure.
Further Resources for Binary Search in Java
If you’re interested in learning more about binary search in Java and related topics, here are some resources you might find beneficial:
- Java Stream: Enhancing Data Manipulation – Dive into advanced topics like parallelism and concurrency in Java stream processing.
Exploring Java Stream Filter – Master Java stream filter operations for efficient data manipulation and extraction.
Java Merge Sort Algorithm – Learn Java merge sort techniques for efficient sorting of large datasets.
Java Algorithms and Clients – A comprehensive resource that covers various algorithms in Java, including binary search.
Oracle’s Java Tutorials covers a wide range of topics, including collections, generics, and more.
GeeksforGeeks’ Binary Search Guide provides a detailed explanation of binary search, its implementation, and its variations.
Wrapping Up: Binary Search in Java
In this comprehensive guide, we’ve delved into the process of implementing and using binary search in Java, an efficient algorithm for finding elements in sorted arrays.
We started with the basics, learning how to implement binary search using the Arrays.binarySearch()
method from the Java standard library. We then moved onto more advanced techniques, such as performing binary search on 2D arrays and using binary search with custom objects. Along the way, we tackled common issues you might encounter when implementing binary search, such as off-by-one errors and problems with unsorted arrays, providing solutions and best practices for each issue.
We also explored alternative methods for performing binary search in Java, such as using the Collections.binarySearch()
method for lists and implementing an iterative binary search. Here’s a quick comparison of these methods:
Method | Use Case | Complexity |
---|---|---|
Arrays.binarySearch() | Arrays | O(log n) |
Collections.binarySearch() | Lists | O(log n) |
Iterative Binary Search | Custom Implementation | O(log n) |
Whether you’re just starting out with binary search in Java or you’re looking to refine your skills, we hope this guide has helped you understand the intricacies of binary search and its implementation in Java.
Armed with this knowledge, you’re now ready to implement binary search in your Java programs and solve complex problems more efficiently. Happy coding!