Java Applet: Guide to Embedding Web Applications
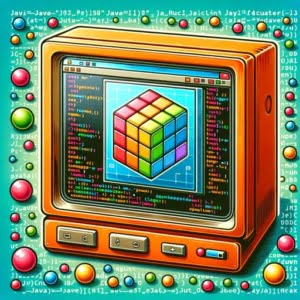
Have you ever been curious about how to create interactive web content using Java? You’re not alone. Many developers find the process of creating dynamic web content a bit challenging, but there’s a tool that can make this process a breeze.
Think of a Java Applet as a puppet master, capable of bringing your webpage to life. It’s a small application written in Java that can be embedded in a webpage, providing a versatile and handy tool for various tasks.
This guide will walk you through the basics of Java Applets, from creation to deployment. We’ll explore everything from the basics to more advanced techniques, as well as alternative approaches. So, let’s dive in and start mastering Java Applets!
TL;DR: What is a Java Applet?
A Java Applet is a small application written in Java that can be embedded in a webpage. To start using an applet, you must use the import statements,
import java.applet.Applet;
. You can then create a class that extends from Applet:
import java.awt.Graphics;public class HelloWorld extends Applet {}
It’s a powerful tool that allows you to create dynamic and interactive web content.
Here’s a simple example of a Java Applet:
import java.applet.Applet;
import java.awt.Graphics;
public class HelloWorld extends Applet {
public void paint(Graphics g) {
g.drawString("Hello world!", 50, 25);
}
}
In this example, we’ve created a basic Java Applet named ‘HelloWorld’. This Applet displays the text ‘Hello world!’ at the coordinates (50, 25) on the screen. The paint
method is a built-in function in the Applet class that allows us to draw strings, lines, shapes and more.
This is just a basic introduction to Java Applets. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Creating Your First Java Applet
- Embedding Java Applet in a Webpage
- Understanding How Java Applet Works
- Advantages and Pitfalls of Java Applets
- Interactive Java Applets
- Complex Calculations in Java Applets
- Best Practices for Advanced Java Applets
- Exploring Alternatives to Java Applets
- Troubleshooting Common Java Applet Issues
- Java Programming: The Backbone of Applets
- Java Applets: Interaction with Webpages and Browsers
- Java Applets in Modern Web Development
- Wrapping Up: Java Applets
Creating Your First Java Applet
Creating a Java Applet is straightforward. Let’s start by writing a basic Java Applet and understanding its structure.
import java.applet.Applet;
import java.awt.Graphics;
public class FirstApplet extends Applet {
public void paint(Graphics g) {
g.drawString("My First Java Applet!", 50, 25);
}
}
In this code, we’ve created an Applet named ‘FirstApplet’. The paint
method is used to draw the string ‘My First Java Applet!’ at the coordinates (50, 25).
Embedding Java Applet in a Webpage
Once your Applet is ready, it’s time to embed it into a webpage. This is done using the “ tag in your HTML code.
<applet code="FirstApplet.class" width="300" height="200"></applet>
This will display your Java Applet within a 300×200 pixel area on your webpage.
Understanding How Java Applet Works
The magic of a Java Applet lies in its lifecycle, which consists of initialization, starting, stopping, and destruction. This lifecycle is managed by calling the Applet’s methods in a specific order.
init()
: This method is called when the Applet is first loaded. It’s typically used for one-time operations such as loading images or sounds.start()
: This method is called afterinit()
, and also whenever the Applet is restarted. It’s typically used to resume animations or threads.stop()
: This method is called when the webpage containing the Applet is no longer on the screen. It’s used to suspend animations or other resource-intensive operations.destroy()
: This method is called when the Applet is about to be unloaded. It’s used to perform cleanup operations.
Advantages and Pitfalls of Java Applets
Java Applets offer several advantages. They enable you to create interactive web content, they’re platform-independent, and they have access to powerful Java APIs.
However, Java Applets also come with some potential pitfalls. They require the Java plugin to be installed and enabled in the user’s browser, and they can pose security risks if not properly sandboxed. Also, as of 2018, many modern browsers have dropped support for Java Applets due to these security concerns.
Interactive Java Applets
Java Applets aren’t limited to displaying static content. They can also interact with users and perform complex calculations. Let’s create an Applet that takes user input and displays it.
import java.applet.Applet;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JTextField;
public class InteractiveApplet extends Applet implements ActionListener {
JTextField input;
String display = "";
public void init() {
input = new JTextField(20);
add(input);
input.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
display = input.getText();
repaint();
}
public void paint(Graphics g) {
g.drawString(display, 20, 100);
}
}
This Applet creates a text field and listens for user input. When the user hits enter, the text they entered is displayed on the Applet. The repaint()
method is called to update the display.
Complex Calculations in Java Applets
Java Applets can also perform complex tasks, such as mathematical calculations. Here’s an example of an Applet that calculates the factorial of a number.
import java.applet.Applet;
import java.awt.Graphics;
public class FactorialApplet extends Applet {
int fact(int n) {
if (n == 1)
return 1;
else
return n * fact(n-1);
}
public void paint(Graphics g) {
int result = fact(5);
g.drawString("Factorial of 5 is " + result, 50, 30);
}
}
In this Applet, the fact
method calculates the factorial of a number using recursion. The result is then displayed on the Applet.
Best Practices for Advanced Java Applets
When creating advanced Java Applets, remember to keep user experience in mind. Make sure your Applet is responsive and doesn’t freeze the browser while performing complex tasks. Use threading if necessary to perform heavy operations in the background. Also, always validate user input to prevent security vulnerabilities.
Exploring Alternatives to Java Applets
While Java Applets have been a staple in web development, there are alternative approaches to creating interactive web content. Two common alternatives are JavaScript and Flash.
JavaScript: The Modern Approach
JavaScript has become the de facto language for client-side web programming. It’s supported by all modern browsers without the need for plugins.
document.getElementById('demo').innerHTML = 'Hello, JavaScript!';
In this JavaScript example, we’re changing the content of an HTML element with the id ‘demo’ to ‘Hello, JavaScript!’.
Flash: The Legacy Approach
Flash was once a popular choice for creating interactive web content. However, it’s less common now due to security concerns and lack of support on mobile devices.
trace('Hello, Flash!');
In this Flash (ActionScript) example, we’re printing ‘Hello, Flash!’ to the debug console.
Java Applets vs JavaScript vs Flash
Feature | Java Applet | JavaScript | Flash |
---|---|---|---|
Plugin required | Yes | No | Yes |
Mobile support | No | Yes | No |
Security | High (if sandboxed) | High | Low |
Browser support | Low | High | Low |
Performance | High | Varies | High |
While every tool has its place, JavaScript is generally the recommended choice for modern web development due to its wide support and versatility. However, for complex applications requiring high performance, Java Applets (or WebAssembly, a newer alternative) may still be a valid choice.
Troubleshooting Common Java Applet Issues
While Java Applets can be powerful tools, they can also present challenges. Here, we’ll discuss some common issues you might encounter when creating Java Applets, along with solutions and workarounds.
Security Restrictions
Java Applets run in a restricted environment, or ‘sandbox’, for security reasons. This can limit their functionality.
For example, an unsigned Applet can’t access the client’s file system. If your Applet needs to perform such operations, you’ll need to sign it with a digital certificate.
// This will throw a security exception in an unsigned Applet
File file = new File("test.txt");
Compatibility Issues
Java Applets require the Java Runtime Environment (JRE) to be installed on the client’s machine. This can cause compatibility issues, as not all users will have the correct version of the JRE installed.
One solution is to use the deployJava.js
script provided by Oracle. This script checks the client’s JRE version and prompts them to update if necessary.
<script src="https://www.java.com/js/deployJava.js"></script>
<script>
deployJava.runApplet({code: 'MyApplet.class', archive: 'MyApplet.jar'}, {}, '1.6');
</script>
In this example, deployJava.runApplet
will only run the Applet if the client has JRE version 1.6 or higher installed.
Tips for Developing Java Applets
When developing Java Applets, keep these tips in mind:
- Always test your Applet in multiple browsers and JRE versions to ensure compatibility.
- Use the Java Console for debugging. The console can display error messages, exceptions, and other useful information.
- Keep security in mind. Always validate user input and avoid performing sensitive operations in an Applet.
Java Programming: The Backbone of Applets
Java, a robust, object-oriented programming language, is the foundation of Java Applets. Its ‘Write Once, Run Anywhere’ principle ensures that Java programs, including Applets, can run on any device that has a Java Runtime Environment (JRE).
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
// Output:
// Hello, World!
In this simple Java program, we’ve defined a class named ‘HelloWorld’. Inside this class, we have a main
method which is the entry point for any Java program. This method prints ‘Hello, World!’ to the console.
Java Applets: Interaction with Webpages and Browsers
Java Applets are designed to be embedded into webpages. When a user visits a webpage containing an Applet, the Applet is automatically downloaded and run in the JRE installed on the user’s machine.
Applets interact with webpages through the Document Object Model (DOM). The DOM represents the structure of the webpage, and Applets can use it to manipulate the webpage’s content.
import netscape.javascript.JSObject;
public class DOMManipulationApplet extends Applet {
public void init() {
JSObject window = JSObject.getWindow(this);
JSObject document = (JSObject) window.getMember("document");
document.call("write", new Object[] {"Hello, DOM!"});
}
}
// Output on webpage:
// Hello, DOM!
In this example, the Applet uses the JSObject
class to access the webpage’s DOM and write ‘Hello, DOM!’ to it.
Java Applets also interact with the user’s browser. The browser provides the Applet with an environment to run in, handles network connections, and manages security. In return, the Applet can provide the user with interactive content, such as games, calculators, or even chat applications.
Java Applets in Modern Web Development
While Java Applets were once a go-to tool for creating interactive web content, their relevance in the modern web development landscape has diminished. The rise of JavaScript, HTML5, and CSS3 has led to native browser technologies that can deliver interactive experiences without the need for plugins like the Java Runtime Environment.
Despite this, Java Applets still have their place. They can be useful in specific scenarios where Java’s robustness and performance are needed. Furthermore, they remain a valuable teaching tool for understanding web programming concepts.
Comparing Java Applets to Modern Technologies
Modern web technologies like JavaScript, HTML5, and CSS3 have largely replaced Java Applets for creating interactive web content. These technologies are natively supported by all modern browsers, removing the need for plugins.
However, Java Applets can still be useful in certain situations. For example, they can be used to create complex applications that require the robustness and performance of the Java programming language.
Further Resources for Mastering Java Applets
For those interested in exploring Java Applets further, here are some resources that provide deeper insights:
- IOFlood’s Java Web Development Article – Explore the latest trends and techniques in Java web development.
Selenium with Java Overview – Learn about Selenium WebDriver for automating web browser interactions using Java.
Exploring JavaServer Faces – Explore JSF’s features for the development of web applications with reusable UI components.
Oracle’s Java Tutorials: A comprehensive guide to Java programming, including a section on Applets.
GeeksforGeeks’ Java Applet Tutorial covers the basics of Java Applets, including their lifecycle and graphics programming.
Java2s Applet Tutorials covers various Applet topics, from graphics to event handling.
Wrapping Up: Java Applets
In this comprehensive guide, we’ve delved into the world of Java Applets, exploring their use in creating dynamic, interactive web content.
We started with the basics, understanding what a Java Applet is and how to create one. We then dived into more advanced usage scenarios, learning how to create interactive Applets and perform complex calculations. We also discussed the lifecycle of a Java Applet, a crucial concept for understanding how Applets work.
Along the way, we tackled common challenges you might encounter when creating Java Applets, from security restrictions to compatibility issues. We provided solutions and workarounds for each issue, equipping you with the knowledge to overcome these hurdles.
We also explored alternative approaches to creating interactive web content, comparing Java Applets with JavaScript and Flash. Here’s a quick comparison of these methods:
Method | Plugin Required | Mobile Support | Security | Browser Support |
---|---|---|---|---|
Java Applet | Yes | No | High (if sandboxed) | Low |
JavaScript | No | Yes | High | High |
Flash | Yes | No | Low | Low |
Whether you’re a beginner just starting out with Java Applets or an experienced developer looking for a refresher, we hope this guide has provided a comprehensive overview of Java Applets and their place in web development.
Java Applets, despite their diminished popularity, still offer a robust and powerful tool for certain web development tasks. With this guide, you’re now equipped to harness the power of Java Applets in your web development journey. Happy coding!