Java BigDecimal Class: Guide to Precise Math Operations
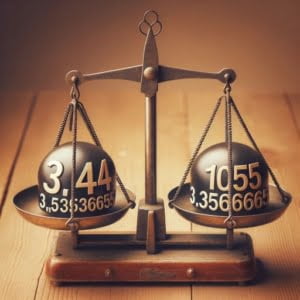
Are you wrestling with handling large precision numbers in Java? You’re not alone. Many developers find themselves in a fix when it comes to dealing with precision in Java, but there’s a tool that can come to your rescue.
Like a mathematical wizard, BigDecimal in Java is a powerful tool that can help you handle numbers with large precision. It provides control over the precision and scale of your numbers and can be a lifesaver when dealing with complex calculations.
This guide will walk you through the ins and outs of using BigDecimal in Java, from basic usage to advanced techniques. We’ll cover everything from creating and manipulating BigDecimal objects, to dealing with precision and rounding issues, and even exploring alternative approaches.
So, let’s dive in and start mastering BigDecimal in Java!
TL;DR: How Do I Use BigDecimal in Java?
BigDecimal is used in Java to handle numbers with large precision. You can create a BigDecimal object and perform operations like addition, subtraction, multiplication, and division with high precision.
Here’s a simple example:
BigDecimal bd = new BigDecimal("0.1");
bd = bd.add(new BigDecimal("0.2"));
System.out.println(bd);
// Output:
// 0.3
In this example, we create a BigDecimal object bd
with the value of 0.1. We then add 0.2 to bd
using the add
method. The result is printed out, and as expected, we get 0.3. This is a basic usage of BigDecimal in Java, but there’s much more to it.
Dive in for a more detailed exploration of BigDecimal in Java, including advanced usage scenarios and alternative approaches.
Table of Contents
Harnessing BigDecimal: Basic Usage
BigDecimal is a class in Java that provides operations for arithmetic (addition, subtraction, multiplication, division), scale manipulation, rounding, comparison, hashing, and format conversion. It can handle very large and very small floating point numbers with great precision.
Let’s see how we can use it in a basic scenario:
BigDecimal number1 = new BigDecimal("10.35");
BigDecimal number2 = new BigDecimal("15.60");
BigDecimal sum = number1.add(number2);
System.out.println("Sum: " + sum);
// Output:
// Sum: 25.95
In this case, we create two BigDecimal objects, number1
and number2
, with the values of 10.35 and 15.60 respectively. We then add these two numbers together using the add
method and print out the result. The output, as expected, is 25.95.
The advantage of using BigDecimal comes from its precision handling. If you were to perform the same operation using double
or float
, you might not get a precise result due to the way floating-point arithmetic works in computers. BigDecimal eliminates this issue, giving you precise and consistent results every time.
However, it’s important to note that BigDecimal can be slower compared to double
or float
due to the high precision arithmetic, so it’s a trade-off between precision and performance. It’s also important to remember to use the String constructor for BigDecimal to avoid precision issues.
BigDecimal: Tackling Complex Operations
As we dive deeper into the world of BigDecimal in Java, we encounter more complex operations like multiplication, division, and handling rounding errors. Let’s explore these with a few examples.
Multiplication with BigDecimal
Multiplication is as straightforward as addition. Let’s multiply two BigDecimal numbers:
BigDecimal number1 = new BigDecimal("10.5");
BigDecimal number2 = new BigDecimal("2.3");
BigDecimal product = number1.multiply(number2);
System.out.println("Product: " + product);
// Output:
// Product: 24.15
In this example, we multiply number1
and number2
using the multiply
method. The result is 24.15, as expected.
Division and Rounding with BigDecimal
Division can be a bit tricky because it might produce a non-terminating decimal. In such cases, you need to specify a rounding mode. Here’s an example:
BigDecimal number1 = new BigDecimal("10");
BigDecimal number2 = new BigDecimal("3");
BigDecimal quotient = number1.divide(number2, 2, RoundingMode.HALF_UP);
System.out.println("Quotient: " + quotient);
// Output:
// Quotient: 3.33
In this case, we’re dividing 10 by 3, which would result in a non-terminating decimal. To handle this, we use the divide
method that accepts two additional parameters – the scale and the rounding mode. The scale is the number of digits to the right of the decimal point. The rounding mode is the strategy to follow when the number can’t be represented precisely. We use RoundingMode.HALF_UP
which is the common rounding mode that you learned in school.
Using BigDecimal for complex operations ensures precision and gives you control over the scale and rounding behavior. However, it’s important to choose the rounding mode carefully based on your specific use case to avoid unexpected results.
Exploring Alternatives to BigDecimal
While BigDecimal is a powerful tool for precision handling in Java, it’s not the only method available. In this section, we’ll explore alternative approaches, such as using the Math class or third-party libraries.
Precision Handling with the Math Class
Java’s Math class provides methods for performing basic numeric operations. Let’s look at an example:
double number1 = 10.35;
double number2 = 15.60;
double sum = Math.round((number1 + number2) * 100.0) / 100.0;
System.out.println("Sum: " + sum);
// Output:
// Sum: 25.95
In this example, we use the Math.round
method to round the result of the addition operation to two decimal places. This approach can handle basic precision requirements but can be cumbersome for more complex scenarios.
Leveraging Third-Party Libraries
Third-party libraries like Apache Commons Math and JScience provide advanced mathematical and statistical functions. They can be a good alternative if you need more advanced features than what BigDecimal or Math class provides.
Here’s an example of using Apache Commons Math for precision handling:
// Note: This requires importing Apache Commons Math library
Precision.round((0.1 + 0.2), 2);
// Output:
// 0.3
In this example, we use the Precision.round
method from Apache Commons Math library to round the result of the addition operation to two decimal places.
Method | Advantages | Disadvantages |
---|---|---|
BigDecimal | High precision, Control over rounding | Slower performance |
Math class | Built-in, No external dependencies | Cumbersome for complex scenarios |
Third-party libraries | Advanced features, High precision | External dependencies, May be overkill for simple scenarios |
Choosing the right method depends on your specific requirements. If you need high precision and control over rounding, BigDecimal is the way to go. For basic operations, the Math class might be sufficient. If you need advanced mathematical functions, consider using a third-party library.
Troubleshooting BigDecimal: Common Issues and Solutions
While using BigDecimal in Java provides many benefits, it’s not without its challenges. Let’s delve into some common issues you might encounter and how to resolve them.
Precision Errors
One common issue is precision errors. For instance, you might expect a certain number of decimal places but get more or less. This is often due to not setting the scale correctly when performing operations.
BigDecimal number1 = new BigDecimal("1.2");
BigDecimal number2 = new BigDecimal("2.2");
BigDecimal result = number1.subtract(number2);
System.out.println(result);
// Output:
// -1.0000000000000002220446049250313080847263336181640625
In this example, the output has many more decimal places than expected. The solution is to set the scale when performing the operation:
BigDecimal result = number1.subtract(number2).setScale(2, RoundingMode.HALF_UP);
System.out.println(result);
// Output:
// -1.00
Rounding Issues
Another common issue is rounding errors. When dividing BigDecimal numbers, you might see an ArithmeticException
if the division doesn’t produce a finite decimal. The solution is to specify a rounding mode when performing the division:
BigDecimal number1 = new BigDecimal("10");
BigDecimal number2 = new BigDecimal("3");
try {
BigDecimal result = number1.divide(number2);
System.out.println(result);
} catch (ArithmeticException e) {
System.out.println("Error: Non-terminating decimal");
}
// Output:
// Error: Non-terminating decimal
In this example, the division of 10 by 3 doesn’t produce a finite decimal, resulting in an exception. The solution is to specify a rounding mode:
BigDecimal result = number1.divide(number2, 2, RoundingMode.HALF_UP);
System.out.println(result);
// Output:
// 3.33
By being aware of these common issues and knowing how to handle them, you can use BigDecimal effectively in your Java programs.
Understanding Numerical Precision in Java
Before diving into BigDecimal, it’s crucial to understand numerical precision and how Java handles it. Precision is the number of digits in a number. In the context of programming, precision is important when dealing with numbers that require a high degree of accuracy, such as financial calculations or scientific computations.
How Java Handles Precision
Java provides several ways to handle numerical precision. Primitive types like float
and double
can represent numbers with decimal points, but they have limitations with precision and can lead to inaccurate results.
float number1 = 1.2f;
float number2 = 2.2f;
float result = number1 - number2;
System.out.println(result);
// Output:
// -0.9999999
In this example, subtracting 2.2 from 1.2 using float
gives us -0.9999999 instead of -1. This is due to the way floating-point numbers are represented in binary, leading to precision loss.
The Role of BigDecimal in Precision Handling
This is where BigDecimal comes into play. BigDecimal is a class in Java that provides operations for arithmetic (addition, subtraction, multiplication, division), scale manipulation, rounding, comparison, hashing, and format conversion. It can handle very large and very small floating point numbers with great precision, making it a popular choice for high-precision calculations.
BigDecimal number1 = new BigDecimal("1.2");
BigDecimal number2 = new BigDecimal("2.2");
BigDecimal result = number1.subtract(number2);
System.out.println(result);
// Output:
// -1.0
In this example, subtracting 2.2 from 1.2 using BigDecimal gives us the correct result, -1.0. This demonstrates the advantage of using BigDecimal for precision handling in Java.
BigDecimal in Real-World Applications
BigDecimal isn’t just a theoretical concept, it has practical applications in many areas where precision is paramount.
Financial Calculations
Financial applications often require high precision to handle money calculations accurately. A small rounding error can lead to significant problems. BigDecimal provides the precision necessary for these calculations.
BigDecimal price = new BigDecimal("999.99");
BigDecimal taxRate = new BigDecimal("0.05"); // 5% tax
BigDecimal tax = price.multiply(taxRate);
tax = tax.setScale(2, RoundingMode.HALF_UP);
System.out.println("Tax: " + tax);
// Output:
// Tax: 50.00
In this example, we calculate the tax on a price using a 5% tax rate. BigDecimal ensures the result is precise to the penny.
Scientific Computing
Scientific computations often involve very large or very small numbers, and precision is critical. BigDecimal can handle these numbers with great precision, making it suitable for scientific computing.
Exploring Related Concepts
If you’re interested in diving deeper, you might want to explore related concepts like floating point arithmetic and numerical analysis. Understanding these concepts can give you a broader view of how numerical precision is handled in computing.
Further Resources for Mastering BigDecimal
For more info on Math and Numbers in Java, we recommend reviewing our Java Math Class Guide by Clicking Here!
And for even more information on BigDecimal class, here are some additional resources:
- Exploring Math.min() Function in Java – Discover how Math.min() simplifies finding the smallest value in Java programming.
Exploring Absolute Value Concept in Java – Discover how Math.abs() simplifies handling absolute value in Java.
Oracle’s Official BigDecimal Documentation – A comprehensive resource for understanding BigDecimal’s methods and.
Baeldung’s Guide on BigDecimal – A practical guide that covers many usage scenarios of BigDecimal.
BigDecimal Class in Java by GeeksforGeeks provides detailed explanations about the BigDecimal class in Java.
Wrapping Up: BigDecimal in Java
In this comprehensive guide, we’ve delved into the world of BigDecimal in Java, a powerful tool for handling numbers with large precision. We’ve uncovered its capabilities, from basic usage to advanced techniques, and explored how to troubleshoot common issues.
We began with the basics, learning how to create BigDecimal objects and perform simple arithmetic operations with high precision. We then ventured into more advanced territory, dealing with complex operations like multiplication, division, and handling rounding errors. Along the way, we tackled common challenges such as precision errors and rounding issues, providing solutions and workarounds for each.
Furthermore, we explored alternative approaches to precision handling, such as using the Math class or third-party libraries. We compared these methods, giving you a sense of the broader landscape of tools for precision handling in Java.
Method | Precision | Performance | Complexity |
---|---|---|---|
BigDecimal | High | Moderate | Moderate |
Math class | Low | High | Low |
Third-party libraries | High | Varies | High |
Whether you’re just starting out with BigDecimal or you’re looking to level up your precision handling skills, we hope this guide has given you a deeper understanding of BigDecimal and its capabilities.
With its balance of precision, performance, and complexity, BigDecimal is a powerful tool for numerical calculations in Java. Now, you’re well equipped to tackle any precision-related challenges. Happy coding!