Mastering Java with Code Examples | Beginner to Expert
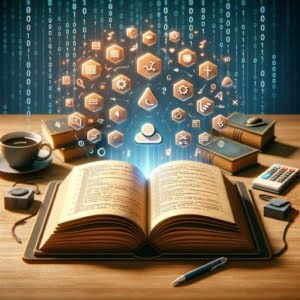
Are you on a quest for practical Java code examples? You’re not alone. Many developers, whether they’re just starting out or looking to expand their knowledge, often find themselves in need of real-world examples to truly grasp a language.
Think of Java code examples as the secret ingredient to mastering Java – they provide a hands-on approach to learning, helping you understand how the syntax and concepts come together to create functional programs.
In this guide, we’ll walk you through a variety of Java code examples, from basic syntax to advanced concepts. Each example will be explained line by line, helping you understand not just the ‘how’, but also the ‘why’. So, whether you’re a beginner looking to get started, or an experienced programmer aiming to brush up on advanced concepts, this guide has got you covered.
Let’s dive in and start mastering Java through practical examples!
TL;DR: How Can I Master Java Syntax and Methods?
The best way to learn Java is with in-depth code examples. This guide provides a variety of Java code examples that cover basic syntax to advanced concepts, such as printing to console:
System.out.println('Text you want to print');
. Here’s a simple example of a Java program that prints text to the screen:
public class ConsolePrint {
public static void main(String[] args) {
System.out.println('Here is some sample text.');
}
}
# Output:
# 'Here is some sample text.'
In this example, we’ve created a basic Java program that prints to the console. This is often used to create the first program that beginners write when learning a new programming language. It’s a simple yet effective way to understand the basic structure of a Java program.
But Java’s capabilities go far beyond this. Continue reading for more detailed examples and advanced concepts.
Table of Contents
Java for Beginners: Writing Basic Programs
Your First Java Program: ‘Hello, World!’
Let’s start with the classic ‘Hello, World!’ program. This simple program is often the first one you’ll write when learning a new programming language. It’s a great way to understand the basic structure of a Java program.
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# Output:
# 'Hello, World!'
In this example, we’ve created a basic Java program that prints ‘Hello, World!’ to the console. This is often the first program that beginners write when learning a new programming language. It’s a simple yet effective way to understand the basic structure of a Java program.
Understanding Basic Data Types in Java
Java has several basic data types that you’ll use frequently when programming. These include integers (int), floating-point numbers (float and double), characters (char), and booleans (boolean).
Here’s an example of how you might declare and initialize these basic data types in Java:
public class BasicDataTypes {
public static void main(String[] args) {
int number = 10;
float floatNumber = 20.0f;
double doubleNumber = 30.0;
char character = 'A';
boolean isJavaFun = true;
System.out.println(number);
System.out.println(floatNumber);
System.out.println(doubleNumber);
System.out.println(character);
System.out.println(isJavaFun);
}
}
# Output:
# 10
# 20.0
# 30.0
# A
# true
This code declares and initializes several variables of different data types, then prints their values to the console. Understanding these basic data types is crucial to programming in Java, as they form the building blocks of more complex data structures and algorithms.
Control Structures: Making Decisions with If-Else Statements
Control structures allow your program to make decisions based on certain conditions. One of the most common control structures in Java is the if-else statement.
Here’s an example of an if-else statement in action:
public class ControlStructures {
public static void main(String[] args) {
int number = 10;
if (number > 5) {
System.out.println('The number is greater than 5.');
} else {
System.out.println('The number is not greater than 5.');
}
}
}
# Output:
# 'The number is greater than 5.'
In this example, the program checks if the variable ‘number’ is greater than 5. If it is, it prints ‘The number is greater than 5.’ to the console. Otherwise, it prints ‘The number is not greater than 5.’ Understanding control structures is key to writing dynamic programs that can respond to different situations.
Simple Class Definitions: Creating Your First Java Class
In Java, everything is an object, and objects are instances of classes. Classes are essentially blueprints for creating objects. They define the properties and behaviors that an object of that class should have.
Here’s an example of a simple class definition in Java:
public class SimpleClass {
int number;
String text;
SimpleClass(int number, String text) {
this.number = number;
this.text = text;
}
void display() {
System.out.println('Number: ' + number);
System.out.println('Text: ' + text);
}
}
public class Main {
public static void main(String[] args) {
SimpleClass simpleClass = new SimpleClass(10, 'Hello, World!');
simpleClass.display();
}
}
# Output:
# 'Number: 10'
# 'Text: Hello, World!'
In this example, we’ve created a simple class called ‘SimpleClass’ with two properties: ‘number’ and ‘text’. We’ve also defined a constructor for the class (the ‘SimpleClass(int number, String text)’ method), which is a special method that’s called when an object of the class is created. Finally, we’ve defined a ‘display()’ method that prints the values of the ‘number’ and ‘text’ properties to the console.
Understanding class definitions is crucial to mastering Java, as it’s an object-oriented programming language where classes and objects form the basis of the code.
Diving Deeper: Intermediate Java Concepts
Leveraging Interfaces in Java
Interfaces in Java are a fundamental aspect of Java programming. They define a contract for classes, specifying a set of methods that a class should implement. Here’s an example of how to define and use an interface in Java:
interface Animal {
void makeSound();
}
class Dog implements Animal {
public void makeSound() {
System.out.println('Woof!');
}
}
class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound();
}
}
# Output:
# 'Woof!'
In this example, we’ve defined an interface named ‘Animal’ with a single method ‘makeSound()’. Then, we’ve created a class ‘Dog’ that implements this interface and provides an implementation for the ‘makeSound()’ method. When we create an instance of ‘Dog’ and call ‘makeSound()’, it prints ‘Woof!’ to the console.
Understanding Inheritance in Java
Inheritance is a fundamental concept in object-oriented programming that allows one class to inherit the properties and methods of another. Here’s an example of inheritance in Java:
class Animal {
void eat() {
System.out.println('The animal eats...');
}
}
class Dog extends Animal {
void bark() {
System.out.println('The dog barks...');
}
}
class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat();
myDog.bark();
}
}
# Output:
# 'The animal eats...'
# 'The dog barks...'
In this example, we’ve created a base class ‘Animal’ with a method ‘eat()’. Then, we’ve created a ‘Dog’ class that extends ‘Animal’, inheriting its methods. The ‘Dog’ class also defines its own method, ‘bark()’. When we create an instance of ‘Dog’ and call ‘eat()’ and ‘bark()’, it prints ‘The animal eats…’ and ‘The dog barks…’ to the console.
Handling Exceptions in Java
Exception handling in Java is a powerful mechanism that allows you to handle runtime errors so that normal flow of the application can be maintained. Here’s an example of how to handle exceptions in Java:
class Main {
public static void main(String[] args) {
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println('Caught an arithmetic exception.');
} finally {
System.out.println('The finally block always executes.');
}
}
}
# Output:
# 'Caught an arithmetic exception.'
# 'The finally block always executes.'
In this example, we’ve used a try-catch-finally block to handle an ArithmeticException
. The code within the try block is executed first. If an exception occurs, the catch block is executed. The finally block is always executed, whether an exception occurred or not.
File I/O in Java
Java provides strong support for reading from and writing to files. Here’s an example of how to write to a file in Java:
import java.io.FileWriter;
import java.io.IOException;
class Main {
public static void main(String[] args) {
try {
FileWriter myWriter = new FileWriter('filename.txt');
myWriter.write('Hello, World!');
myWriter.close();
System.out.println('Successfully wrote to the file.');
} catch (IOException e) {
System.out.println('An error occurred.');
e.printStackTrace();
}
}
}
# Output:
# 'Successfully wrote to the file.'
In this example, we’ve used the FileWriter
class to write data to a file. If the file doesn’t exist, it will be created. If the file does exist, it will be overwritten. If an error occurs during this process, an IOException
will be thrown and can be caught and handled.
Exploring Alternative Approaches in Java
Utilizing Different Data Structures
Java provides a rich set of data structures within its Collections Framework. Depending on your specific needs, you may find one type of data structure more suitable than others. For instance, if you need to store data where the order matters, you might opt for a List. If you need to ensure there are no duplicates, a Set might be the way to go. Here’s an example of how you might use a List and a Set in Java:
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import java.util.HashSet;
class Main {
public static void main(String[] args) {
List<String> myList = new ArrayList<String>();
myList.add('Hello');
myList.add('World');
myList.add('Hello');
System.out.println(myList);
Set<String> mySet = new HashSet<String>();
mySet.add('Hello');
mySet.add('World');
mySet.add('Hello');
System.out.println(mySet);
}
}
# Output:
# [Hello, World, Hello]
# [Hello, World]
In this example, we’ve added the same elements to both a List and a Set. The List preserves the order of elements and allows duplicates, while the Set does not allow duplicate elements.
Implementing Design Patterns
Design patterns are proven solutions to common software design problems. They represent best practices and can significantly improve the quality of your code. For instance, the Singleton pattern ensures that a class has only one instance and provides a global point of access to it. Here’s an example of how you might implement a Singleton in Java:
class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
class Main {
public static void main(String[] args) {
Singleton singleton = Singleton.getInstance();
System.out.println(singleton);
}
}
# Output:
# Singleton@15db9742
In this example, we’ve created a Singleton class that has a private constructor to prevent creating more than one instance. The getInstance()
method returns the single instance of the class, creating it if it doesn’t exist.
Leveraging Third-Party Libraries
Java has a vast ecosystem of third-party libraries that you can use to solve various problems. For instance, if you need to work with JSON data, you might use the Jackson library. Here’s an example of how you might use Jackson to parse JSON in Java:
import com.fasterxml.jackson.databind.ObjectMapper;
class Main {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
String jsonString = '{"name":"John", "age":30}';
try {
Person person = mapper.readValue(jsonString, Person.class);
System.out.println(person);
} catch (Exception e) {
e.printStackTrace();
}
}
}
# Output:
# Person{name='John', age=30}
In this example, we’ve used the Jackson library to parse a JSON string into a Person object. This is just one example of how third-party libraries can simplify your Java code and increase your productivity.
Common Java Troubleshooting and Considerations
Handling Null Pointer Exceptions
One of the most common errors encountered when writing Java code is a Null Pointer Exception. This happens when you try to access a method or property of an object that’s null. Here’s an example of this error and how to handle it:
class Main {
public static void main(String[] args) {
String text = null;
try {
System.out.println(text.length());
} catch (NullPointerException e) {
System.out.println('Caught a NullPointerException.');
}
}
}
# Output:
# 'Caught a NullPointerException.'
In this example, we’ve attempted to call the length()
method on a null string, which throws a NullPointerException
. We’ve used a try-catch block to handle this exception and print a message to the console.
Best Practices for Exception Handling
When handling exceptions, it’s a good practice to catch more specific exceptions before more general ones. This allows you to handle different types of exceptions in different ways. It’s also a good practice to include meaningful messages in your exceptions, which can help with debugging.
Optimization Tips
When writing Java code, it’s important to consider performance. Here are a few tips for optimizing your Java code:
- Use StringBuilder for String Concatenation: If you’re concatenating strings in a loop, use
StringBuilder
instead of the+
operator for better performance. Use Primitive Types When Possible: Primitive types are faster and take up less memory than their wrapper classes.
Avoid Unnecessary Object Creation: Creating objects in Java is expensive in terms of memory and processing power. If you can reuse an existing object instead of creating a new one, you can make your Java code more efficient.
Use Enhanced for Loops: The enhanced for loop, also known as the for-each loop, can make your code cleaner and less prone to errors. It’s especially useful when iterating over collections.
Remember, the best way to learn is to practice. So, don’t be afraid to experiment, make mistakes, and learn from them. Happy coding!
Unraveling Java Fundamentals
Embracing Object-Oriented Programming
Java is an object-oriented programming (OOP) language, which means it revolves around ‘objects’ and ‘classes’. Objects are instances of classes – they’re the things you interact with in your code. Classes, on the other hand, are like blueprints from which objects are created. They define the properties (attributes) and behaviors (methods) of an object.
Here’s a simple example of a class and an object in Java:
public class Car {
String color;
void start() {
System.out.println('The car starts...');
}
}
class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.color = 'Red';
myCar.start();
}
}
# Output:
# 'The car starts...'
In this example, we’ve created a ‘Car’ class with a ‘color’ attribute and a ‘start()’ method. Then, we’ve created an object ‘myCar’ from the ‘Car’ class, set its color to ‘Red’, and called its ‘start()’ method.
Understanding Java’s Memory Model
Java’s memory model is an abstract representation of how Java manages memory. It divides memory into two areas: the stack and the heap. The stack is where primitive types and object references are stored, while the heap is where objects and their instance variables are stored.
Consider this example:
public class Main {
public static void main(String[] args) {
int number = 10; // Stored in the stack
Car myCar = new Car(); // 'myCar' is stored in the stack, the new 'Car' object is stored in the heap
myCar.color = 'Red'; // 'color' is an instance variable of the 'Car' object, so it's stored in the heap
}
}
In this example, the ‘number’ variable is a primitive type, so it’s stored in the stack. The ‘myCar’ variable is an object reference, so it’s also stored in the stack. However, the ‘Car’ object that ‘myCar’ refers to is stored in the heap, along with its ‘color’ instance variable.
Exploring the Java Runtime Environment
The Java Runtime Environment (JRE) is a part of the Java Development Kit (JDK) that contains the Java Virtual Machine (JVM) and the Java class libraries. It’s what allows your Java programs to run.
When you run a Java program, the JVM loads the .class files (bytecode) into memory, verifies the bytecode, interprets it, and then executes it. The Java class libraries provide a standard set of classes for tasks like handling I/O, managing collections of data, networking, and GUI development.
Expanding Horizons: Java in the Real World
Java in Larger Projects
Java is not just a language for small programs or academic exercises. It’s a robust language used in large-scale projects across various industries. From enterprise-level applications to Android apps, from scientific computing to big data analytics, Java’s versatility makes it suitable for a wide range of applications.
// A simple Android activity written in Java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
This is a simple example of an Android activity written in Java. Android, one of the most widely used mobile operating systems, is largely based on Java. If you’re learning Java, developing Android apps could be a great way to apply and expand your skills.
Accompanying Topics in Java Development
When developing in Java, you’ll likely encounter related topics such as unit testing, build tools, and deployment strategies. These are essential skills for any serious Java developer.
- Unit Testing: This involves testing individual components of your program to ensure they work as expected. JUnit is a popular framework for unit testing in Java.
Build Tools: These are used to automate the process of compiling, testing, and packaging your code. Maven and Gradle are widely used build tools in the Java ecosystem.
Deployment Strategies: These are plans for delivering your software to the users. Depending on the nature of your project, you might deploy your Java applications on a server, in the cloud, or directly on the users’ devices.
Further Resources for Mastering Java
If you’re interested in going beyond the basics and mastering Java, here are some resources that you might find helpful:
- Use Cases Explored: Java Tutorial – Understand how to create and use custom Java exceptions.
Java Runner Guide – Learn how to use the Java Runner tool to execute Java programs from the command line.
Java Functions Basics – Explore Java functions for encapsulating reusable code blocks and modular programming.
Oracle’s Java Tutorials cover a wide range of topics, from basics to advanced features.
Baeldung offers a wealth of articles and tutorials on various Java topics, including Spring, REST APIs, and more.
Java Code Geeks features articles, tutorials, code snippets, and open source projects.
Wrapping Up: Practical Code Examples
In this comprehensive guide, we’ve explored Java through practical code examples, from the basic syntax to advanced concepts.
We started with the basics, learning how to write simple Java programs, and understanding basic data types, control structures, and simple class definitions. We then delved into more advanced topics, such as using interfaces, inheritance, exception handling, and file I/O, with each example accompanied by a detailed explanation.
We also discussed alternative ways to solve problems in Java, such as using different data structures, design patterns, or third-party libraries. Each alternative was illustrated with a code example and an explanation of when and why to use it. In addition, we covered common errors or obstacles encountered when writing Java code and their solutions, along with tips for best practices and optimization.
Here’s a quick comparison of the methods and concepts we’ve discussed:
Concept | Use Case | Difficulty Level |
---|---|---|
Basic Syntax | Writing simple programs | Beginner |
Interfaces & Inheritance | Creating complex, scalable programs | Intermediate |
Exception Handling & File I/O | Building robust, error-resilient programs | Intermediate |
Alternative Approaches (Data Structures, Design Patterns) | Solving problems more efficiently | Expert |
We hope this guide, packed with practical Java code examples, has helped you understand and master Java, whether you’re a beginner just starting out or an experienced developer looking to brush up on your skills.
With its balance of simplicity for beginners and power for experts, Java is a versatile language that’s widely used in the industry. Keep practicing, keep coding, and you’ll continue to grow your Java skills. Happy coding!