Exploring Java’s Math Class: Methods and Examples
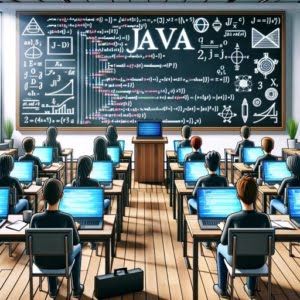
Are you finding it challenging to navigate the Math class in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling mathematical operations in Java, but we’re here to help.
Think of Java’s Math class as a trusty calculator – providing a host of functions to perform mathematical operations, from simple arithmetic to complex trigonometric calculations.
In this guide, we’ll walk you through the key methods of the Math class in Java, from basic to advanced usage. We’ll cover everything from the basics of the Math class to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering the Math class in Java!
TL;DR: How to use the Math Class in Java?
The Math class in Java is a utility class that provides a host of methods for performing mathematical operations. You can call it with the
Math
keyword, followed by the desired method, such as.sqrt(), .abs(), .min(), or .max()
, for exampleint result = Math.abs(-25);
. Whether you need to calculate the square root of a number or find the absolute value of a number, the Math class has got you covered.
Here’s a simple example of using the Math class to calculate the square root of a number:
double result = Math.sqrt(25);
System.out.println(result);
// Output:
// 5.0
In this example, we use the sqrt()
method from the Math class to calculate the square root of 25. The result is then printed to the console, giving us an output of 5.0.
This is just a basic usage of the Math class in Java. There’s a lot more to explore, including more complex methods and alternative approaches. Continue reading for a more detailed guide and advanced usage scenarios.
Table of Contents
Basic Usage of Java’s Math Class
The Math class in Java offers a variety of methods that allow you to perform common mathematical operations. Let’s dive into some of these basic methods and how to use them.
Calculating Square Root with sqrt()
One of the most commonly used methods in the Math class is sqrt()
. This method calculates the square root of a given number. Here’s an example:
double num = 36;
double result = Math.sqrt(num);
System.out.println(result);
// Output:
// 6.0
In this example, we calculate the square root of 36 using Math.sqrt()
, and the result is 6.0.
Finding Absolute Value with abs()
The abs()
method returns the absolute value of the given number. This means it returns the number without any sign (negative or positive). Here’s how you can use it:
int num = -10;
int result = Math.abs(num);
System.out.println(result);
// Output:
// 10
In this example, we find the absolute value of -10 using Math.abs()
, and the result is 10.
Rounding Numbers with round()
The round()
method rounds a floating-point value to the nearest integer. Here’s an example:
float num = 5.6f;
long result = Math.round(num);
System.out.println(result);
// Output:
// 6
In this example, we round the number 5.6 to the nearest integer using Math.round()
, and the result is 6. These basic methods of the Math class in Java form the foundation for more complex mathematical operations you’ll encounter in your programming journey.
Advanced Usage of Java’s Math Class
Beyond the basic methods, the Math class in Java offers more complex methods that can be incredibly useful in various applications. Let’s explore some of them.
Trigonometric Methods: sin()
, cos()
, and tan()
The Math class provides methods to calculate the sine, cosine, and tangent of a given angle in radians. Here’s how you can use them:
double angleInRadians = Math.toRadians(45);
// Calculate sine of the angle
double sinValue = Math.sin(angleInRadians);
System.out.println("Sine: " + sinValue);
// Calculate cosine of the angle
double cosValue = Math.cos(angleInRadians);
System.out.println("Cosine: " + cosValue);
// Calculate tangent of the angle
double tanValue = Math.tan(angleInRadians);
System.out.println("Tangent: " + tanValue);
// Output:
// Sine: 0.7071067811865476
// Cosine: 0.7071067811865476
// Tangent: 1.0
In this example, we first convert an angle of 45 degrees to radians using Math.toRadians()
. Then, we calculate the sine, cosine, and tangent of this angle using Math.sin()
, Math.cos()
, and Math.tan()
respectively.
Power and Logarithmic Methods: pow()
and log()
The pow()
method calculates the power of a number, and the log()
method calculates the natural logarithm (base e) of a number. Here’s an example:
double base = 2;
double exponent = 3;
// Calculate power
double powerResult = Math.pow(base, exponent);
System.out.println("Power: " + powerResult);
// Calculate natural logarithm
double logResult = Math.log(powerResult);
System.out.println("Logarithm: " + logResult);
// Output:
// Power: 8.0
// Logarithm: 2.0794415416798357
In this example, we calculate the power of 2 to the power of 3 using Math.pow()
, and the result is 8.0. Then, we calculate the natural logarithm of 8.0 using Math.log()
, and the result is approximately 2.079.
Exploring Alternatives: The StrictMath Class
While the Math class in Java offers a plethora of methods for mathematical operations, there’s an alternative you might want to consider – the StrictMath
class. This class provides similar functionality to the Math class but adheres more strictly to the IEEE 754 standard.
StrictMath vs Math
Just like the Math class, StrictMath offers methods like sqrt()
, abs()
, round()
, sin()
, cos()
, tan()
, pow()
, and log()
. The key difference lies in the precision of the results.
Let’s look at an example using the sqrt()
method in both classes:
double num = 25;
// Using Math class
double mathSqrtResult = Math.sqrt(num);
System.out.println("Math.sqrt: " + mathSqrtResult);
// Using StrictMath class
double strictMathSqrtResult = StrictMath.sqrt(num);
System.out.println("StrictMath.sqrt: " + strictMathSqrtResult);
// Output:
// Math.sqrt: 5.0
// StrictMath.sqrt: 5.0
In this example, both classes return the same result. However, for more complex calculations, particularly those involving trigonometric functions, the StrictMath class may provide more precise results due to its strict adherence to the IEEE 754 standard.
When to Use StrictMath
If you’re working on applications where precision is critical, such as scientific or financial applications, you might want to consider using StrictMath. However, for most general purposes, the Math class should suffice.
Remember, Java’s Math class and StrictMath class are tools at your disposal. Understanding their differences and knowing when to use each can help you write more effective and accurate Java programs.
Troubleshooting Java’s Math Class
While the Math class in Java is a powerful tool, it’s not without its quirks. Let’s discuss some common issues you might encounter and how to handle them effectively.
Dealing with Precision Errors
One common issue when working with floating-point numbers is precision errors. Here’s an example:
double result = Math.sqrt(2);
System.out.println(result * result);
// Output:
// 2.0000000000000004
In this example, we calculate the square root of 2, then square the result. Ideally, we should get 2, but due to precision errors, we get a slightly different result.
To handle such issues, consider rounding the result to the desired precision using the round()
method.
Handling NaN
and Infinity
Values
The Math class methods can sometimes return NaN
(Not a Number) and Infinity
values. For instance, the sqrt()
method returns NaN
when passed a negative number, and the division operation returns Infinity
when dividing by zero.
double sqrtResult = Math.sqrt(-10);
System.out.println(sqrtResult);
double divResult = 10.0/0.0;
System.out.println(divResult);
// Output:
// NaN
// Infinity
In this example, Math.sqrt(-10)
returns NaN
and 10.0/0.0
returns Infinity
. When dealing with such cases, it’s important to check the input values before performing operations or handle these special values in your code.
Understanding these considerations when using the Math class in Java can help you avoid unexpected results and write more robust code.
Understanding Java’s Math Class
The Math class in Java is an integral part of the Java programming language. It’s a final class (which means it can’t be inherited) and is part of java.lang
package. This class provides a variety of methods that allow you to perform mathematical operations without the need to create an object.
// Example of using Math class
double result = Math.sqrt(16);
System.out.println(result);
// Output:
// 4.0
In this example, we use the sqrt()
method from the Math class to calculate the square root of 16. The result is then printed to the console, giving us an output of 4.0.
The IEEE 754 Standard and Its Relevance
The Math class in Java adheres to the IEEE 754 standard for floating-point arithmetic. This standard was established to create a uniform way of representing and manipulating floating-point numbers across different computing platforms.
The IEEE 754 standard affects how numbers are represented, how rounding is handled, and how special cases like infinity and NaN (Not a Number) are dealt with. For example, when you divide a positive number by zero using the Math class, it returns Infinity
, which is a special floating-point value defined by the IEEE 754 standard.
double result = 10.0/0.0;
System.out.println(result);
// Output:
// Infinity
In this example, we divide 10.0 by 0.0, and the result is Infinity
. This behavior is defined by the IEEE 754 standard and is implemented in the Math class.
Applying Java’s Math Class in Real-World Applications
The Math class in Java isn’t just for academic exercises – it has a wide range of applications in the real world. Let’s explore some of them.
Game Development
In game development, the Math class is often used for creating movements and animations. For instance, the sin()
and cos()
methods can be used to create circular movements, while the random()
method can be used to generate random events.
Scientific Computing
In scientific computing, the Math class is used for complex calculations. Methods like pow()
, sqrt()
, and log()
are commonly used in fields like physics and engineering to perform computations.
Financial Applications
In financial applications, precision is critical. Methods like round()
can be used to round off currency values to the nearest cent. For high-precision calculations, it’s recommended to explore the BigDecimal
class.
BigDecimal bd1 = new BigDecimal("10.25");
BigDecimal bd2 = new BigDecimal("5.75");
BigDecimal result = bd1.add(bd2);
System.out.println(result);
// Output:
// 16.00
In this example, we use the BigDecimal
class for a high-precision addition operation. The result is exactly 16.00, demonstrating the high precision of BigDecimal
.
Further Resources for Mastering Java’s Math Class
To deepen your understanding of the Math class in Java and its applications, here are some resources you might find useful:
- Java Classes Overview – Dive into Java classes, the building blocks of object-oriented programming in Java.
Math.min() in Java – Learn how to find the minimum value among two numbers using Math.min() in Java.
Oracle’s Official Java Documentation provides a detailed explanation of each method in the Math class.
GeeksforGeeks Java Math Class Article provides a comprehensive tutorial on the Math class, complete with code examples.
Baeldung’s Guide to Java’s Math Class provides a deeper dive into the Math class and its methods, including some of the nuances and common pitfalls.
Wrapping Up: Java’s Math Class
In this comprehensive guide, we’ve explored the ins and outs of the Math class in Java, a vital tool for performing a wide range of mathematical operations.
We began with the basics, learning how to use the Math class for simple tasks like calculating the square root of a number or finding the absolute value of a number. We then delved into more advanced usage, exploring complex methods like sin()
, cos()
, tan()
, pow()
, and log()
. Along the way, we tackled common issues that you might encounter when using the Math class, such as dealing with precision errors and handling NaN
and Infinity
values, providing you with solutions and workarounds for each issue.
We also looked at the StrictMath
class, an alternative to the Math class that adheres more strictly to the IEEE 754 standard. Here’s a quick comparison of these classes:
Class | Precision | Ease of Use |
---|---|---|
Math | High | High |
StrictMath | Higher | High |
Whether you’re just starting out with the Math class in Java or you’re looking to level up your mathematical operations skills, we hope this guide has given you a deeper understanding of the Math class and its capabilities.
With its balance of ease of use and precision, the Math class in Java is a powerful tool for any Java developer. Now, you’re well equipped to make the most of it. Happy coding!