Primitive Data Types in Java: A Detailed Overview
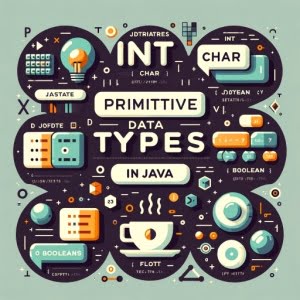
Are you finding it challenging to understand primitive data types in Java? You’re not alone. Many developers, especially beginners, find this topic a bit complex. But, think of Java’s primitive data types as the basic building blocks, akin to Lego pieces, that form the foundation of your Java programs.
These primitive types are the most fundamental data types in Java, and understanding them is crucial to mastering the language. They are the simplest forms of data with which we can start building more complex data structures.
In this guide, we will walk you through each of Java’s eight primitive data types, explaining their uses and characteristics. We’ll cover everything from their size, range of values, and common uses, to more advanced topics like type casting, type promotion, and the use of wrapper classes.
So, let’s dive in and start mastering Java’s primitive data types!
TL;DR: What Are the Primitive Data Types in Java?
Java has eight primitive data types:
byte, short, int, long, float, double, char, and boolean
. Each of these types has a specific size and a specific range of values it can represent.
Here’s a simple example of declaring each type:
byte b = 10;
short s = 500;
int i = 10000;
long l = 150000L;
float f = 5.75f;
double d = 19.99;
char c = 'A';
boolean bool = true;
// Output:
// b = 10
// s = 500
// i = 10000
// l = 150000
// f = 5.75
// d = 19.99
// c = 'A'
// bool = true
In this example, we’ve declared and initialized each of Java’s eight primitive data types. The values assigned are within the range that each type can represent.
This is just a basic introduction to Java’s primitive data types. There’s much more to learn about each type, including their size, range of values, and when to use them. Continue reading for a more detailed exploration of these fundamental building blocks of Java.
Table of Contents
- Exploring Java’s Primitive Data Types
- Diving Deeper: Type Casting, Promotion, and Wrapper Classes
- Deep Dive into Java’s Reference Types
- Troubleshooting Primitive Data Types in Java
- Understanding Data Types in Programming
- Exploring Beyond Primitive Data Types in Java
- Wrapping Up: Mastering Primitive Data Types in Java
Exploring Java’s Primitive Data Types
Java provides eight primitive data types, each with its own size, range of values, and common uses. Let’s explore each of these types in detail.
Byte
The byte
data type in Java is an 8-bit signed two’s complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive).
byte byteValue = 100;
System.out.println(byteValue);
// Output:
// 100
In this example, we’ve declared a byte
variable named byteValue
and assigned it a value of 100. The System.out.println
command prints the value of byteValue
to the console.
Short
The short
data type in Java is a 16-bit signed two’s complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive).
short shortValue = 30000;
System.out.println(shortValue);
// Output:
// 30000
Here, we’ve declared a short
variable named shortValue
and assigned it a value of 30000. The System.out.println
command prints the value of shortValue
to the console.
Int
The int
data type in Java is a 32-bit signed two’s complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive).
int intValue = 2000000;
System.out.println(intValue);
// Output:
// 2000000
In this example, we’ve declared an int
variable named intValue
and assigned it a value of 2000000. The System.out.println
command prints the value of intValue
to the console.
Long
The long
data type in Java is a 64-bit two’s complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive).
long longValue = 3000000000L;
System.out.println(longValue);
// Output:
// 3000000000
Here, we’ve declared a long
variable named longValue
and assigned it a value of 3000000000. The System.out.println
command prints the value of longValue
to the console.
Float
The float
data type in Java is a single-precision 32-bit IEEE 754 floating point. It’s mainly used to save memory in large arrays of floating point numbers.
float floatValue = 3.14f;
System.out.println(floatValue);
// Output:
// 3.14
In this example, we’ve declared a float
variable named floatValue
and assigned it a value of 3.14. The System.out.println
command prints the value of floatValue
to the console.
Double
The double
data type in Java is a double-precision 64-bit IEEE 754 floating point. It’s generally used for decimal values.
double doubleValue = 3.1415926535;
System.out.println(doubleValue);
// Output:
// 3.1415926535
Here, we’ve declared a double
variable named doubleValue
and assigned it a value of 3.1415926535. The System.out.println
command prints the value of doubleValue
to the console.
Char
The char
data type in Java is a single 16-bit Unicode character. It has a minimum value of ‘\u0000’ (or 0) and a maximum value of ‘\uffff’ (or 65,535 inclusive).
char charValue = 'J';
System.out.println(charValue);
// Output:
// J
In this example, we’ve declared a char
variable named charValue
and assigned it a value of ‘J’. The System.out.println
command prints the value of charValue
to the console.
Boolean
The boolean
data type in Java has only two possible values: true
and false
. It’s used for simple flags that track true/false conditions.
boolean booleanValue = true;
System.out.println(booleanValue);
// Output:
// true
Here, we’ve declared a boolean
variable named booleanValue
and assigned it a value of true. The System.out.println
command prints the value of booleanValue
to the console.
Diving Deeper: Type Casting, Promotion, and Wrapper Classes
As you become more comfortable with Java’s primitive data types, you’ll encounter situations that require a more advanced understanding. Let’s explore some of these scenarios.
Type Casting in Java
Type casting is when you assign a value of one primitive data type to another type. In Java, there are two types of casting:
- Widening Casting (automatically) – converting a smaller type to a larger type size
- Narrowing Casting (manually) – converting a larger type to a smaller size type
// Widening Casting
int myInt = 9;
double myDouble = myInt;
System.out.println(myInt);
System.out.println(myDouble);
// Output:
// 9
// 9.0
In the example above, we cast an int
to a double
. Java automatically casts the int
to a double
since a double
is larger than an int
.
// Narrowing Casting
double myDouble = 9.78;
int myInt = (int) myDouble;
System.out.println(myDouble);
System.out.println(myInt);
// Output:
// 9.78
// 9
In this example, we cast a double
to an int
. Since an int
is smaller than a double
, we need to manually cast it using (int)
.
Type Promotion in Java
Type promotion is an automatic conversion of one data type to another. It usually occurs when we perform operations between different types.
int myInt = 5;
double myDouble = 6.2;
double result = myInt + myDouble;
System.out.println(result);
// Output:
// 11.2
In this example, myInt
is automatically promoted to a double
when performing the addition operation with myDouble
. The result is a double
.
Wrapper Classes in Java
Each of Java’s eight primitive data types has a corresponding wrapper class. These classes encapsulate a primitive data type within an object.
// Creating an Integer object
Integer myInt = Integer.valueOf(10);
System.out.println(myInt);
// Output:
// 10
In this example, we use the Integer
wrapper class to create an Integer
object that encapsulates the primitive int
value 10.
Deep Dive into Java’s Reference Types
Java’s primitive types are efficient and easy to use, but they have their limitations. For instance, they cannot be used to call methods because they are not objects. This is where Java’s reference types come into play.
Understanding Reference Types
Reference types in Java are used to create objects and arrays. Unlike primitive types, which hold their values directly, reference types hold the address of the object they refer to.
String str = new String("Hello World");
System.out.println(str);
// Output:
// Hello World
In this example, str
is a reference type. It holds the address of the String
object that contains “Hello World”.
Primitive Types vs. Reference Types
The choice between using primitive types and reference types depends on what you need to do. Primitive types are simpler and faster, while reference types are more flexible and powerful.
int primitive = 5;
Integer reference = new Integer(5);
System.out.println(primitive);
System.out.println(reference);
// Output:
// 5
// 5
In this example, primitive
is a primitive type and reference
is a reference type. Both hold the value 5, but reference
is an object that can be used to call methods.
Performance Considerations
Primitive types are generally faster and use less memory than reference types. This is because reference types need to allocate memory for their object and store the address of the object.
The Use of Null
One key advantage of reference types is that they can be assigned the value null
, representing the absence of a value. Primitive types cannot be null
.
Integer reference = null;
System.out.println(reference);
// Output:
// null
In this example, we assign null
to the Integer
reference type. This would not be possible with an int
primitive type.
Troubleshooting Primitive Data Types in Java
While working with primitive data types in Java, you might encounter some common issues. Let’s discuss these problems and provide some solutions.
Handling Overflow and Underflow
Overflow and underflow situations arise when a value is outside the range for its data type.
byte byteValue = 127;
byteValue++;
System.out.println(byteValue);
// Output:
// -128
In this example, we incremented byteValue
beyond its maximum value. This caused an overflow, and byteValue
wrapped around to its minimum value.
Dealing with Loss of Precision
Loss of precision occurs when a floating-point value is assigned to an integer type.
int intValue = (int) 3.14;
System.out.println(intValue);
// Output:
// 3
In this example, we cast the floating-point number 3.14 to an int
. The decimal part was lost, resulting in a loss of precision.
Avoiding Null Pointer Exceptions
Null pointer exceptions occur when you try to use a reference type that has a null
value.
Integer reference = null;
reference++;
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, we tried to increment a null
reference, which resulted in a NullPointerException
.
To avoid these issues, always ensure that your values are within the correct range for their data type, be aware of the loss of precision when casting between types, and check for null
before using a reference type.
Understanding Data Types in Programming
In programming, data types are an integral concept. They define the kind of data that can be stored and manipulated within a program. Java, like many other programming languages, categorizes its data types into two broad categories: primitive types and reference types.
Primitive Types vs. Reference Types
Primitive types are the most basic data types available within the Java language. They include byte
, short
, int
, long
, float
, double
, char
, and boolean
. These types are predefined by the language and named by a reserved keyword.
On the other hand, reference types are created based on the programmer’s need. They can be used to store collections of data, such as arrays and objects.
int primitiveType = 10; // Primitive type
Integer referenceType = new Integer(10); // Reference type
System.out.println(primitiveType);
System.out.println(referenceType);
// Output:
// 10
// 10
In this example, primitiveType
is a primitive data type, while referenceType
is a reference data type. Both hold the integer value 10, but referenceType
is an object that can be used to call methods.
How Data Types are Handled in Memory
When it comes to memory management, primitive types and reference types are handled differently. Primitive types are stored directly in the stack, while reference types are stored in the heap. The variable in the stack points to the location in the heap where the actual object is stored.
int primitiveType = 10; // Stored directly in the stack
Integer referenceType = new Integer(10); // Stored in the heap
In this example, primitiveType
is stored directly in the stack. In contrast, referenceType
is an address that points to the actual object in the heap.
Understanding the distinction between primitive and reference types, as well as how they are handled in memory, is crucial for efficient programming in Java.
Exploring Beyond Primitive Data Types in Java
Understanding Java’s primitive data types is a fundamental step in mastering the language. However, it’s also the starting point for delving into more complex topics that are crucial for effective programming and system design.
Data Structures and Algorithms
Data structures and algorithms form the backbone of efficient programming. A deep understanding of primitive data types in Java is essential for building and manipulating various data structures like arrays, linked lists, stacks, queues, trees, and graphs. These data structures, in turn, form the basis for creating efficient algorithms.
Java’s Object Class
In Java, every class implicitly or explicitly extends the Object class, making it a superclass of all other classes. Hence, understanding primitive data types is vital for working with the Object class and its methods.
Generics in Java
Generics add stability to your code by allowing you to specify the exact type of your collections at compile time. A solid understanding of Java’s primitive data types is necessary to use generics effectively.
Java’s Collections Framework
The Collections Framework in Java is a unified architecture for representing and manipulating collections. Knowledge of primitive data types is essential to work effectively with different types of collections like List, Set, Map, etc.
Further Resources for Mastering Java’s Primitive Data Types
To deepen your understanding of Java’s primitive data types, consider exploring the following resources:
- Understanding Data Types – Master selecting the appropriate data type for variables in Java programs
Java Long – Explore the Java long data type for representing large integer values.
Boolean Data Type in Java – Learn about boolean expressions and logical operations in Java.
Oracle’s Java Tutorials – Oracle’s official tutorials are a great resource for learning Java.
Geeks for Geeks’ Java Guide offers tutorials on all aspects of Java programming, including primitive data types.
Introduction to Primitive Types in Java by Baeldung explains primitive data types in Java, their memory management, and usage.
Wrapping Up: Mastering Primitive Data Types in Java
In this comprehensive guide, we’ve delved into the core of Java programming – the primitive data types. We’ve dissected each of Java’s eight primitive types, understanding their characteristics, common uses, and potential issues.
We embarked on our journey with the basics, exploring each primitive type from byte
to boolean
. We then delved into more advanced topics, discussing type casting, type promotion, and the use of wrapper classes. Along the way, we tackled common issues you might encounter, such as overflow, underflow, and loss of precision, providing you with solutions and workarounds for each problem.
We also ventured into the world of reference types, understanding their use, performance considerations, and the unique ability to handle null
values. This led us to the distinction between primitive types and reference types in Java, a fundamental concept for any Java programmer.
Data Type | Size | Min Value | Max Value |
---|---|---|---|
byte | 8-bit | -128 | 127 |
short | 16-bit | -32,768 | 32,767 |
int | 32-bit | -2,147,483,648 | 2,147,483,647 |
long | 64-bit | -9,223,372,036,854,775,808 | 9,223,372,036,854,775,807 |
float | 32-bit | 1.4e-45 | 3.4e+38 |
double | 64-bit | 4.9e-324 | 1.8e+308 |
char | 16-bit | ” | ‘’ |
boolean | N/A | false | true |
Whether you’re just starting out with Java or you’re looking to brush up your skills, we hope this guide has given you a deeper understanding of Java’s primitive data types and their importance in your coding journey.
Understanding primitive data types is a fundamental step in mastering Java. With this knowledge, you are well-equipped to tackle more complex topics in Java. Happy coding!