Python type() Function Guide (With Examples)
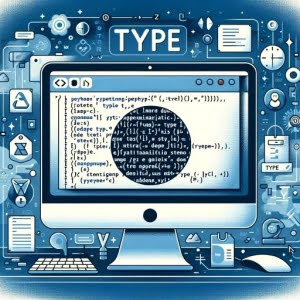
Ever found yourself puzzled, wondering about the ‘type’ of a variable in Python? Just like a seasoned detective, Python’s type()
function is here to help you solve this mystery
In this comprehensive guide, we’ll journey through the intriguing world of Python’s type()
function, exploring everything from its basic usage to advanced techniques. Whether you’re a Python novice or a seasoned coder, this guide will provide valuable insights and practical examples to enhance your Python programming skills.
TL;DR: How Do I Use the type() Function in Python?
The
type()
function in Python is a built-in function used to determine the type of a given object. Here’s a quick example to illustrate its use:
data = 'Hello'
print(type(data))
# Output:
# <class 'str'>
In this example, we used the type()
function to determine that the variable data
, which holds the value ‘Hello’, is of the type str
(string). But there’s much more to the type()
function than just this. Stay tuned for a deeper dive into this function, its various applications, and its role in Python programming.
Table of Contents
- Unraveling the Basics of Python’s type() Function
- Delving Deeper: type() Function with Complex Python Objects
- Exploring Alternatives: The isinstance() Function
- Navigating Challenges: Common Issues with the type() Function
- Python Data Types: The Building Blocks
- Looking Ahead: type() Function in Larger Scripts and Projects
- Further Resources for Python Functions
- Wrapping Up: The Power of Python’s type() Function
Unraveling the Basics of Python’s type() Function
Python’s type()
function is a built-in function that helps you determine the type of an object. This function is particularly useful when you’re working with different kinds of Python objects and you need to understand their type to perform certain operations.
Let’s explore this with a simple example. Suppose we have a variable data
that holds a number, a string, and a list:
number = 5
string = 'Hello'
list_data = [1, 2, 3]
print(type(number))
print(type(string))
print(type(list_data))
# Output:
# <class 'int'>
# <class 'str'>
# <class 'list'>
In this example, we used the type()
function to determine that the variable number
is of type int
(integer), string
is of type str
(string), and list_data
is of type list
. This is the basic usage of the type()
function.
The advantage of using the type()
function is that it allows you to quickly and accurately determine the type of any Python object. This can be particularly useful when debugging your code or when you need to perform type-specific operations.
One potential pitfall to be aware of is that the type()
function will not return the expected results with derived types or subclasses. For instance, if a class B
is derived from a class A
, type()
will return B
and not A
when called with an instance of B
as an argument. We’ll delve more into this in the advanced use section.
Delving Deeper: type() Function with Complex Python Objects
As your Python journey progresses, you’ll encounter more complex objects like lists, dictionaries, and custom classes. The type()
function remains your steadfast companion, helping you identify these types as well.
Let’s consider a Python dictionary and a custom class:
dict_data = {'name': 'John', 'age': 30}
print(type(dict_data))
# Output:
# <class 'dict'>
In this example, we used the type()
function to determine that the variable dict_data
is of the type dict
(dictionary).
Now, let’s create a custom class and use type()
to identify it:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
john = Person('John', 30)
print(type(john))
# Output:
# <class '__main__.Person'>
In this example, we created a custom class Person
and instantiated it as john
. When we used the type()
function, it correctly identified john
as an instance of the Person
class.
These examples demonstrate the versatility of the type()
function. It can handle not only basic data types but also more complex Python objects, making it a valuable tool in your Python toolkit.
Exploring Alternatives: The isinstance() Function
While the type()
function is a powerful tool for identifying Python object types, it’s not the only player in the game. Python also provides the isinstance()
function, which can be a handy alternative for checking an object’s type.
Let’s take an example to understand how isinstance()
works:
number = 5
print(isinstance(number, int))
# Output:
# True
In this example, isinstance()
checks if the variable number
is an instance of the int
(integer) class and returns True
.
Now, let’s compare type()
and isinstance()
using a subclass:
class A:
pass
class B(A):
pass
b = B()
print(type(b) is A)
print(isinstance(b, A))
# Output:
# False
# True
In this example, we have a class A
and a class B
that is a subclass of A
. We create an instance b
of class B
. When we use type()
, it returns False
because type()
only considers the immediate class of an object. However, isinstance()
returns True
because it considers the full class hierarchy. Therefore, isinstance()
can sometimes be more appropriate than type()
when you want to check if an object is an instance of a class or its subclasses.
While the type()
function is a robust tool in Python, you might encounter some issues while using it. One common issue is dealing with NoneType
objects.
The NoneType
is the type of the None
object which represents a lack of value, for example, a function not explicitly returning a value will return None
.
Let’s look at an example:
data = None
print(type(data))
# Output:
# <class 'NoneType'>
In this example, the type()
function correctly identifies None
as a NoneType
.
But what happens when you try to perform an operation on a NoneType
object? Let’s see:
data = None
print(data + 5)
# Output:
# TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
Attempting to add 5
to None
results in a TypeError
. It’s important to handle these cases in your code to avoid such errors. One way to handle this is by using an if
statement to check if a variable is None
before performing an operation:
data = None
if data is not None:
print(data + 5)
else:
print('Invalid operation on NoneType object')
# Output:
# Invalid operation on NoneType object
In this example, we first check if data
is not None
before attempting to add 5
. If data
is None
, we print a message instead. This is one way to troubleshoot and handle potential issues when using the type()
function in Python.
Python Data Types: The Building Blocks
Python is a dynamically typed language. This means that the type of a variable is checked during execution, not at compile time. Understanding Python’s data types is crucial as they form the building blocks of your code.
Python supports several built-in data types, including integers (int
), floating-point numbers (float
), strings (str
), and complex numbers (complex
). It also supports more complex types like lists (list
), dictionaries (dict
), and custom classes (class
).
Let’s explore these data types with examples:
integer = 10
floating_point = 20.5
string = 'Hello, World!'
complex_number = 1+2j
print(type(integer))
print(type(floating_point))
print(type(string))
print(type(complex_number))
# Output:
# <class 'int'>
# <class 'float'>
# <class 'str'>
# <class 'complex'>
In this example, we used the type()
function to identify the types of various Python objects. Understanding these types is important as different data types support different operations.
Python’s dynamic typing means that a variable’s type can change over its lifetime. For instance, a variable that initially holds an integer can later hold a string. This is where the type()
function comes in handy. It allows you to determine the current type of a variable at any point in your code, aiding in debugging and ensuring you perform valid operations on your data.
Looking Ahead: type() Function in Larger Scripts and Projects
Understanding Python’s data types and the type()
function is not just for small scripts or basic Python exercises. It’s also crucial when working on larger scripts or projects. Knowing the type of your data can help you write efficient code, prevent bugs, and make your code easier to understand and maintain.
For instance, let’s consider a scenario where you’re working with a large list of mixed data types. Using the type()
function, you could write a function to process the data differently based on their types:
def process_data(data):
for item in data:
if isinstance(item, str):
print(f'Processing string: {item}')
elif isinstance(item, int):
print(f'Processing integer: {item}')
else:
print(f'Unknown data type: {type(item)}')
data = ['Hello', 5, 10.5]
process_data(data)
# Output:
# Processing string: Hello
# Processing integer: 5
# Unknown data type: <class 'float'>
In this example, the process_data()
function processes each item in the data list differently based on its type. This is just one example of how understanding Python’s data types and the type()
function can be beneficial in larger scripts or projects.
Further Resources for Python Functions
To further enrich your understanding of Python functions, we’ve curated a collection of valuable resources for your consideration:
- Python Built-In Functions Usage: Quick Guide – Discover advanced techniques for working with functions and lambda expressions.
Simplifying Data Summation with sum() in Python – Learn how to calculate sums using the “sum” function in Python.
Zip and Unzip Data in Python with zip() Function – Learn how the “zip” function simplifies data pairing and iteration in Python.
JavaTpoint’s Guide on Python Type Function explains how to use Python’s built-in type function effectively.
Codecademy’s Documentation on Python Sum Function offers a clear explanation of Python’s built-in sum function.
Python in a Nutshell Chapter on Built-in Functions – Explore Python’s built-in functions with this book “Python in a Nutshell”
These resources will provide you with a broader perspective of Python functions, thereby enhancing your expertise as a Python developer.
Wrapping Up: The Power of Python’s type() Function
In this comprehensive guide, we’ve explored the type()
function in Python, a handy built-in function that reveals the type of any Python object. From basic integers and strings to complex data structures like lists and dictionaries, and even custom classes, type()
can handle it all.
We’ve also delved into some common issues you might encounter when using the type()
function, such as dealing with NoneType
objects. We learned how to navigate these challenges and ensure our code runs smoothly.
Beyond the type()
function, we discussed the alternative isinstance()
function. We saw how it differs from type()
, particularly when dealing with subclasses, and how it can sometimes be a more appropriate choice.
Understanding Python’s data types and the type()
function is crucial, not just for small scripts, but also for larger projects. As we move beyond the basics, these concepts continue to play a vital role in writing efficient, bug-free, and maintainable code.
Whether you’re just starting out with Python or looking to deepen your knowledge, we hope this guide has shed some light on the power and versatility of Python’s type()
function.