Python’s Random Number Generator: Complete Guide
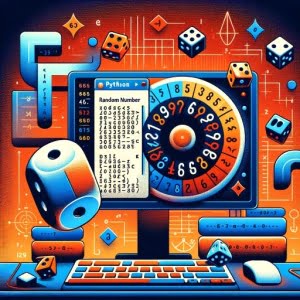
Ever wondered how to generate random numbers in Python? It’s not as complicated as it might seem. Python, like a digital magician, can conjure up a variety of random numbers with just a few commands.
This article will guide you through the entire process, from the basic use to advanced techniques, of Python’s random number generation capabilities. Whether you’re a beginner just starting out with Python or an intermediate user looking to expand your knowledge, this guide has something for you.
Stay with us as we unravel the magic of random numbers in Python, complete with practical code examples and their expected outputs. Let’s dive in!
TL;DR: How Do I Generate Random Numbers in Python?
Python’s built-in
random
module allows you to generate random numbers. Here’s a simple example:
import random
print(random.randint(1, 10))
# Output:
# A random integer between 1 and 10
In the example above, we first import the random
module. Then, we use the randint
function from this module to generate a random integer between 1 and 10. Each time you run this code, Python will print a different random number in this range.
If you’re curious about generating random numbers in Python or want to learn more about advanced usage scenarios, keep reading. We have a lot more to share!
Table of Contents
- Generating Random Numbers: The Basics
- Advanced Random Number Generation in Python
- Alternative Methods for Random Number Generation
- Common Issues and Solutions in Random Number Generation
- Understanding Randomness and Pseudo-Randomness in Python
- Expanding the Horizon: Random Numbers in Action
- Further Resources for Python Modules
- Wrapping Up: The Magic of Random Numbers in Python
Generating Random Numbers: The Basics
Python’s random
module is a built-in library that allows us to generate random numbers. Before we can use it, we need to import it into our script. Here’s how you do that:
import random
With the random
module imported, we can now use the randint
function to generate a random integer within a specified range. Here’s an example:
import random
print(random.randint(1, 10))
# Output:
# A random integer between 1 and 10
In this example, randint(1, 10)
will generate a random integer between 1 and 10, both inclusive. Each time you run this code, you’ll get a different number within this range.
The random
module is a powerful tool with many functions. However, it’s important to note that the numbers it generates are pseudo-random. This means they are generated by a deterministic process and are not truly random. For most applications, pseudo-random numbers are sufficient. But for tasks that require high levels of unpredictability, such as cryptography, you might need to use other methods, which we’ll discuss later in this guide.
Advanced Random Number Generation in Python
Python’s random
module goes beyond generating random integers. It can also generate random floats, choose random elements from a list, and shuffle a list randomly. Let’s explore these features.
Generating Random Floats
To generate a random float, you can use the random()
function. This function returns a random float number between 0.0 and 1.0. Here’s an example:
import random
print(random.random())
# Output:
# A random float between 0.0 and 1.0
Choosing Random Elements from a List
The random
module can also select a random element from a list using the choice()
function. Here’s how you can do it:
import random
my_list = ['apple', 'banana', 'cherry']
print(random.choice(my_list))
# Output:
# 'apple' or 'banana' or 'cherry'
Shuffling a List Randomly
If you need to shuffle the elements in a list randomly, you can use the shuffle()
function. This function reorders the elements in the list in place, meaning that no new list is created. Here’s an example:
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
# Output:
# A shuffled version of [1, 2, 3, 4, 5]
These advanced features of the random
module allow us to perform a wide range of tasks involving random number generation in Python. Remember, the numbers generated by this module are pseudo-random, which means they’re suitable for many applications but not all. For tasks requiring higher levels of unpredictability, other methods may be more appropriate, as we’ll discuss in the next section.
Alternative Methods for Random Number Generation
While Python’s built-in random
module is powerful and versatile, there are alternative methods for generating random numbers that offer additional capabilities. Let’s explore two of these alternatives: the numpy
library and the secrets
module.
Generating Random Numbers with Numpy
The numpy
library is a popular choice for numerical operations in Python, including random number generation. Its random
module can generate arrays of random numbers and supports various probability distributions.
Here’s how you can generate a random integer within a range using numpy
:
import numpy as np
print(np.random.randint(1, 10))
# Output:
# A random integer between 1 and 10
And here’s how you can generate an array of random floats:
import numpy as np
print(np.random.rand(5))
# Output:
# An array of 5 random floats between 0.0 and 1.0
Secure Random Numbers with Secrets
For tasks requiring high levels of unpredictability, such as cryptography, Python’s secrets
module is a good choice. It generates random numbers suitable for security-sensitive applications.
Here’s how you can generate a random integer within a range using secrets
:
import secrets
print(secrets.randbelow(10))
# Output:
# A random integer less than 10
The numpy
library and the secrets
module offer more options and flexibility for random number generation in Python. However, they may be overkill for simple tasks where the built-in random
module is sufficient. Your choice of method should depend on your specific needs and the nature of your project.
Common Issues and Solutions in Random Number Generation
While Python’s random
module is versatile and easy to use, it’s not without its quirks. Let’s discuss some common issues you might encounter when generating random numbers in Python and how to address them.
Pseudo-Randomness and Predictability
The random
module generates pseudo-random numbers. This means they are generated by a deterministic process and are not truly random. If you need to generate truly random numbers for applications like cryptography, you might need to use the secrets
module or an external service.
Here’s how you can generate a random integer using the secrets
module:
import secrets
print(secrets.randbelow(10))
# Output:
# A random integer less than 10
In the example above, the randbelow
function generates a random integer that is less than the number provided.
Reproducibility with Random Seed
Sometimes, you might want to reproduce the same sequence of random numbers for debugging purposes. You can do this by setting a seed value with the seed()
function before generating random numbers.
Here’s an example:
import random
random.seed(1)
print([random.randint(1, 10) for _ in range(5)])
# Output:
# [3, 10, 2, 5, 2]
In this example, we set the seed to 1. This means that every time we run this code, we will get the same sequence of random numbers.
Remember, while Python’s random
module is powerful and versatile, it might not be the best tool for every task. Depending on your specific needs, you might need to use alternative methods like the numpy
library or the secrets
module.
Understanding Randomness and Pseudo-Randomness in Python
To fully grasp the concept of random number generation in Python, it’s important to understand the difference between randomness and pseudo-randomness.
What is Randomness?
In simple terms, randomness implies unpredictability. In a truly random sequence of numbers, there is no pattern that can be used to predict the next number in the sequence.
Pseudo-Randomness in Python
Python’s random
module generates pseudo-random numbers. These numbers appear random and unpredictable, but they are generated by a deterministic process. If you know the process and its initial state (also known as the seed), you can predict all the numbers.
Here’s an example of generating pseudo-random numbers with a fixed seed in Python:
import random
random.seed(1)
print([random.randint(1, 10) for _ in range(5)])
# Output:
# [3, 10, 2, 5, 2]
In this example, we set the seed to 1 using the seed()
function. The sequence of random numbers generated by this code will be the same every time you run it.
The Underlying Algorithm of Python’s random
Module
Python’s random
module uses the Mersenne Twister algorithm to generate pseudo-random numbers. This algorithm is known for its long period (the sequence of numbers before it starts repeating) and high-quality random numbers.
In conclusion, while Python’s random
module doesn’t generate truly random numbers, it generates high-quality pseudo-random numbers that are sufficient for most applications. For tasks requiring truly random numbers, such as cryptography, other methods like the secrets
module or an external service may be more appropriate.
Expanding the Horizon: Random Numbers in Action
The application of random number generation isn’t limited to creating unpredictable sequences. It has a wide range of uses across various fields, including game development, cryptography, and data science.
Game Development
In game development, random numbers can add unpredictability and replayability. They can be used to generate random game scenarios, character attributes, or loot drops. Here’s a simple example of generating a random character attribute:
import random
character_strength = random.randint(1, 10)
print(character_strength)
# Output:
# A random integer between 1 and 10
In this example, the character’s strength attribute is a random number between 1 and 10.
Cryptography
In cryptography, random numbers are essential for creating keys that are hard to predict. Python’s secrets
module, which we discussed earlier, is designed for generating cryptographically strong random numbers.
Data Science
In data science, random numbers are used for tasks such as random sampling, bootstrapping, and Monte Carlo simulations. Python’s numpy
library, which we also discussed earlier, is particularly useful for these tasks due to its ability to generate arrays of random numbers.
Random number generation is a fundamental concept that often accompanies other topics in Python programming.
Further Resources for Python Modules
For more in-depth information about these related topics, you might want to check out the following guides:
- Python Modules Logic Simplified – Explore modules for working with regular expressions and data serialization.
Random Number Generation in Python: A Quick Guide – Explore Python’s “random” module for random number generation
Interacting with External Commands in Python covers subprocess management, input/output streams, and error handling.
Python Generate Random Number – A JavaTpoint tutorial that guides you through generating random numbers in Python.
Python NumPy Random – Learn about the NumPy random module to generate random numbers in Python with W3Schools’ guide.
Python’s Math Module – Explore Python’s math module with W3Schools’ quick reference guide.
Wrapping Up: The Magic of Random Numbers in Python
To recap, we’ve explored the various aspects of random number generation in Python. We started with the basics, learning how to generate random integers using Python’s built-in random
module. We then moved onto more advanced topics, such as generating random floats, selecting random elements from a list, and shuffling a list randomly.
We also discussed common issues you might encounter when using the random
module and how to address them. We learned that the random
module generates pseudo-random numbers, which are sufficient for many applications but not all. For tasks requiring truly random numbers, we explored alternatives such as the secrets
module and the numpy
library.
Here’s a quick comparison of the methods we discussed:
Method | Use Case | Example |
---|---|---|
random.randint() | Generate a random integer within a range | random.randint(1, 10) |
random.random() | Generate a random float between 0.0 and 1.0 | random.random() |
random.choice() | Select a random element from a list | random.choice(my_list) |
random.shuffle() | Shuffle a list randomly | random.shuffle(my_list) |
numpy.random.randint() | Generate a random integer within a range using numpy | np.random.randint(1, 10) |
numpy.random.rand() | Generate an array of random floats using numpy | np.random.rand(5) |
secrets.randbelow() | Generate a random integer less than a certain number using secrets | secrets.randbelow(10) |
We hope this guide has helped you master the art of random number generation in Python. Remember, the method you choose should depend on your specific needs and the nature of your project. Happy coding!