What is Maven? | A Java Project Management Guide
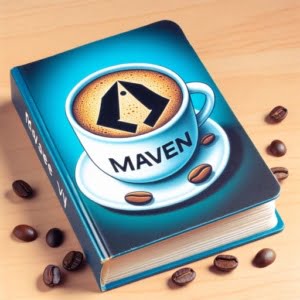
Ever wondered what Maven is in the world of Java? Think of it as a handy toolbox that helps Java developers manage projects more efficiently. It’s like a Swiss army knife, equipped with tools that can handle everything from compiling code to managing dependencies and even generating project documentation.
This guide will help you understand what Maven is, how it works, and why it’s an essential tool for Java developers. We’ll explore Maven’s core functionality, delve into its advanced features, and even discuss common issues and their solutions. So, let’s dive in and start mastering Maven in Java!
TL;DR: What is Maven in Java?
Maven is a powerful project management tool used in Java development. The commands can be utilized by preceding them with
mvn
, for example:mvn compile
. It helps with project build automation, dependency management, and documentation. For example, you can use Maven to compile your Java code, run tests, and generate a project website.
Here’s a simple example of how Maven can be used to compile Java code:
mvn compile
In this example, the mvn compile
command is used to compile the Java code in your project. Maven automatically takes care of finding dependencies, compiling the code, and handling any other tasks necessary to build your project.
This is just a basic introduction to Maven in Java, but there’s much more to learn about this powerful tool. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
Maven in Java: Basic Use for Beginners
Maven is a versatile tool in Java development, offering a range of features that simplify project management. Let’s delve into some of these features.
Maven for Build Automation
Maven automates the build process for Java projects. It compiles your source code, runs tests, generates documentation, and packages the code into a JAR file. Here’s an example of how you can use Maven to compile your Java code:
mvn compile
In this example, the mvn compile
command is used to compile the Java code in your project. Maven automatically takes care of finding dependencies, compiling the code, and handling any other tasks necessary to build your project.
Maven for Dependency Management
Maven also manages dependencies for your project. It automatically downloads necessary libraries and plugins from the internet, which are defined in your project’s POM (Project Object Model) file. Here’s a basic example of a Maven project in Java with a pom.xml
file:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany.app</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
In this pom.xml
file, we have defined a dependency on JUnit, which is a popular testing framework in Java. When you build your project with Maven, it will automatically download JUnit and make it available for your project to use.
Maven for Documentation
Finally, Maven can also generate documentation for your project using the mvn javadoc:javadoc
command. This command generates API documentation for your Java code in HTML format, which can be easily shared and viewed by others. The documentation is generated from Javadoc comments in your code, so make sure to document your code thoroughly!
Maven’s Advanced Features
As you gain more experience with Maven, you’ll discover that its capabilities go far beyond basic build automation and dependency management. Let’s explore some of these advanced features.
Creating Multi-Module Projects
Maven can manage multi-module projects, which are projects that consist of multiple sub-projects (modules). Each module has its own pom.xml
file, but they are all tied together by a parent pom.xml
file. This feature is particularly useful for large projects that need to be broken down into smaller, more manageable parts.
Here’s an example of a multi-module project structure:
my-app
├── module-1
│ └── pom.xml
├── module-2
│ └── pom.xml
└── pom.xml
In this example, my-app
is the parent project, and module-1
and module-2
are the sub-projects (modules). Each module has its own pom.xml
file, and the parent project’s pom.xml
file ties them all together.
Customizing the Build Process
Maven’s build process can be customized using plugins. Plugins provide additional functionality, such as the ability to compile code from different languages, generate reports, or create web applications. You can add a plugin to your project by adding it to your pom.xml
file.
Here’s an example of how to add the Maven Compiler Plugin to your pom.xml
file:
<project>
...
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
...
</project>
In this example, the Maven Compiler Plugin is added to the project, and it’s configured to compile the project’s Java code using Java version 1.8. When you run mvn compile
, Maven will use this plugin to compile your code.
These are just a few examples of the advanced features that Maven offers. As you continue to explore Maven, you’ll discover many more features that can help you manage your Java projects more effectively.
Maven vs. Other Java Build Tools
While Maven is a powerful tool for managing Java projects, it’s not the only tool available. Other popular Java build tools include Ant and Gradle. Let’s compare these tools and discuss when you might want to use each one.
Maven vs. Ant
Ant is another popular build tool in the Java world. Unlike Maven, which emphasizes convention over configuration, Ant is highly configurable and does not impose a specific project structure. This can give you more flexibility, but it can also make your build scripts more complex and harder to maintain.
Here’s an example of a simple Ant build script:
<project name="MyProject" default="dist" basedir=".">
<description>
simple example build file
</description>
<!-- set global properties for this build -->
<property name="src" location="src"/>
<property name="build" location="build"/>
<property name="dist" location="dist"/>
<target name="init">
<!-- Create the build directory structure used by compile -->
<mkdir dir="${build}"/>
</target>
<target name="compile" depends="init" description="compile the source">
<!-- Compile the java code from ${src} into ${build} -->
<javac srcdir="${src}" destdir="${build}"/>
</target>
<target name="dist" depends="compile" description="generate the distribution">
<!-- Create the distribution directory -->
<mkdir dir="${dist}/lib"/>
<!-- Put everything in ${build} into the MyProject-${DSTAMP}.jar file -->
<jar jarfile="${dist}/lib/MyProject-${DSTAMP}.jar" basedir="${build}"/>
</target>
<target name="clean" description="clean up">
<!-- Delete the ${build} and ${dist} directory trees -->
<delete dir="${build}"/>
<delete dir="${dist}"/>
</target>
</project>
This Ant build script compiles Java source code, packages the compiled code into a JAR file, and cleans up the build and distribution directories.
Maven vs. Gradle
Gradle is a newer build tool that combines the best features of Maven and Ant. Like Maven, it uses convention over configuration, making it easy to get started with. Like Ant, it’s highly configurable, allowing you to customize your build process to fit your needs. Additionally, Gradle uses a Groovy-based DSL (domain-specific language) for its build scripts, which can be more readable and expressive than XML.
Here’s an example of a simple Gradle build script:
apply plugin: 'java'
repositories {
jcenter()
}
dependencies {
testCompile 'junit:junit:4.12'
}
This Gradle build script applies the Java plugin, specifies jcenter()
as the repository for dependencies, and adds a dependency on JUnit for testing.
In conclusion, while Maven is a powerful tool for managing Java projects, Ant and Gradle offer alternative approaches that may be better suited to your specific needs. The best tool for you depends on your project’s requirements and your personal preferences.
Troubleshooting Maven: Common Issues and Solutions
While Maven is a powerful tool, like any software, you may encounter issues during its use. Let’s explore some common problems and their solutions.
Dependency Issues
One common issue when using Maven is problems with dependency management. For instance, you might encounter a MissingArtifactException
if Maven cannot find a dependency.
Here’s an example of what this error might look like:
[ERROR] Failed to execute goal on project my-app: Could not resolve dependencies for project com.mycompany.app:my-app:jar:1.0-SNAPSHOT: Could not find artifact junit:junit:jar:4.13 in central (https://repo.maven.apache.org/maven2)
In this example, Maven is trying to download the JUnit dependency from the Maven Central Repository, but it can’t find the specified version (4.13). To fix this issue, you can check if the version number is correct or if the dependency is available in the repository.
Build Failures
Another common issue is build failures. For example, you might encounter a CompilationFailureException
if there’s a problem with your source code.
Here’s an example of what this error might look like:
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3.8.1:compile (default-compile) on project my-app: Compilation failure
[ERROR] /path/to/project/src/main/java/com/mycompany/app/App.java:[14,17] cannot find symbol
[ERROR] symbol: class Scanner
[ERROR] location: class com.mycompany.app.App
In this example, the Java compiler can’t find the Scanner
class, which is likely because of a missing import statement in the source code. To fix this issue, you can add the missing import statement or correct any other errors in your source code.
These are just a few examples of the issues you might encounter when using Maven. Remember, the key to effective troubleshooting is understanding the problem, so always read error messages carefully and use them to guide your investigation.
The Role of Build Tools in Java Development
Build tools are a fundamental part of Java development. They automate the process of converting source code into executable applications, making the development process more efficient and manageable.
Why are Build Tools Important?
Without a build tool, developers would have to manually compile each Java file, manage all the libraries and dependencies, run tests, and package the application for distribution. This manual process is not only time-consuming but also prone to human error.
Build tools automate these tasks, ensuring consistency and reliability in the build process. They also provide a standardized structure, making it easier for developers to understand and manage their projects.
Evolution of Build Tools: From Make to Maven
The concept of build tools isn’t new. It dates back to the 1970s with the creation of Make
, a build automation tool for C. Make
was revolutionary for its time, but it lacked features needed for larger software projects.
In the Java world, Ant
was the first major build tool, introduced in 2000. Ant
provided more flexibility than Make
and was platform-independent, making it popular among Java developers. However, Ant
scripts were often complex and difficult to manage.
Maven
was introduced in 2004 to address some of the shortcomings of Ant
. Unlike Ant
, which is primarily a build tool, Maven
is a project management tool. It introduced a convention-over-configuration approach, providing a standard project structure and lifecycle. This standardization made Maven
projects easier to understand and manage, especially for large projects.
Here’s an example of the lifecycle phases in a Maven
build:
mvn compile # compiles your project's source code
mvn test # runs tests on your compiled source code
mvn package # packages your project into a JAR or WAR file
mvn install # installs the package into your local repository
In this example, each command triggers a different phase of the Maven
build lifecycle. Maven
automatically handles the tasks associated with each phase, such as compiling source code, running tests, packaging the application, and installing the package.
In conclusion, build tools play a crucial role in Java development, automating complex tasks and providing a standardized structure for projects. Maven
, with its powerful project management features, has become a vital tool for many Java developers.
Maven’s Role in Larger Java Projects and the Broader Java Ecosystem
Maven’s significance stretches beyond individual Java projects. In larger projects and the broader Java ecosystem, Maven’s influence is even more pronounced.
Maven in Larger Java Projects
In larger Java projects, Maven’s project management features really shine. Its ability to handle multi-module projects is particularly valuable. Large projects often consist of multiple, interconnected modules, each with its own dependencies and build requirements. Maven’s multi-module project support simplifies managing such projects, ensuring consistent builds across all modules.
Maven in the Broader Java Ecosystem
Beyond individual projects, Maven also plays a crucial role in the broader Java ecosystem. The Maven Central Repository, maintained by the Maven project, is the largest repository of Java libraries and modules in the world. Developers across the globe use this repository to share and access Java libraries, making it a vital resource for the Java community.
Further Resources for Mastering Maven
Want to learn more about Maven and its role in Java development? Here are some resources that can help you deepen your understanding:
- Quick Dive on Java Packages – Explore topics such as package access modifiers and protected members.
Integrating Lombok with Maven – Learn the dependencies and plugins required to configure Lombok in Maven-based Java applications.
Maven Central Repository – Discover the default repository for storing and distributing Java libraries.
Apache Maven Project offers comprehensive documentation, tutorials, and guides on all things Maven.
Baeldung’s Guide to Maven covers everything from basic concepts to advanced topics in Maven.
Maven: The Complete Reference: offers an in-depth look at Maven’s features and capabilities.
Wrapping Up: Maven in Java
In this comprehensive guide, we’ve delved into the world of Maven, a powerful project management tool in Java. We’ve demystified what Maven is, explored its features, and highlighted its pivotal role in Java development.
We began with the basics, discussing how Maven automates build processes, manages dependencies, and generates documentation. We then ventured into more advanced territory, exploring Maven’s capabilities in handling multi-module projects and customizing the build process.
Along the way, we tackled common challenges you might encounter when using Maven, such as dependency issues and build failures, providing you with solutions and best practices for each issue. We also compared Maven with other build tools like Ant and Gradle, giving you a comprehensive overview of the different tools available for Java project management.
Here’s a quick comparison of these tools:
Tool | Flexibility | Standardization | Project Management Features |
---|---|---|---|
Maven | Moderate | High | High |
Ant | High | Low | Moderate |
Gradle | High | High | High |
Whether you’re just starting out with Maven or you’re looking to level up your Java project management skills, we hope this guide has given you a deeper understanding of Maven and its capabilities.
With its balance of standardization, flexibility, and powerful project management features, Maven is an essential tool for any Java developer. Now, you’re well equipped to harness the power of Maven in your Java projects. Happy coding!