Java Final Keyword: A Detailed Exploration
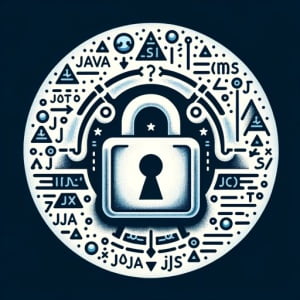
Are you finding the ‘final’ keyword in Java a bit perplexing? You’re not alone. Many developers find themselves in a maze when it comes to understanding this particular keyword. Think of the ‘final’ keyword as a security guard in Java, it locks down variables, methods, and classes, preventing any further changes.
This guide will help you understand and use the ‘final’ keyword effectively in your Java programs. We’ll explore the core functionality of ‘final’, delve into its advanced uses, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the ‘final’ keyword in Java!
TL;DR: What Does ‘final’ Do in Java?
The
final
keyword in Java is used to make a variable constant, prevent a method from being overridden, or prevent a class from being inherited:final int answerToEverything = 42
It’s a powerful tool that can help you control how your code behaves.
Here’s a simple example:
final int MAX_SPEED = 120;
In this example, we declare a constant variable MAX_SPEED
using the ‘final’ keyword. This means that MAX_SPEED
can’t be changed once it’s set to 120.
This is just a basic use of ‘final’ in Java, but there’s much more to learn about this keyword. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of ‘final’ in Java
The ‘final’ keyword in Java is a versatile tool, and its basic uses can be categorized into three main areas: variables, methods, and classes. Let’s explore each of these in detail.
‘final’ with Variables
When you declare a variable as ‘final’, it becomes a constant, meaning its value cannot be changed after it has been initialized. Here’s an example:
final int MAX_AGE = 100;
// MAX_AGE = 101; // This would throw an error
// Output:
// Error: cannot assign a value to final variable MAX_AGE
In this code, MAX_AGE
is a ‘final’ variable and is assigned a value of 100. Any attempt to change this value will result in a compile-time error.
‘final’ with Methods
When applied to methods, ‘final’ prevents a method from being overridden in a subclass. This can be useful when you want a method to always behave in a specific way, regardless of any subclasses that extend the parent class. Here’s an example:
class ParentClass {
final void show() {
System.out.println("This is a final method.");
}
}
class ChildClass extends ParentClass {
// Attempting to override show() would result in a compile-time error
}
// Output:
// Error: show() in ChildClass cannot override show() in ParentClass
// overridden method is final
‘final’ with Classes
When a class is declared as ‘final’, it can’t be extended by any other class. This can be useful when you want to prevent a class from being subclassed. Here’s an example:
final class FinalClass {
// Some methods and variables
}
class SomeClass extends FinalClass { // This would throw an error
// Some methods and variables
}
// Output:
// Error: cannot inherit from final FinalClass
In this code, FinalClass
is declared as ‘final’, so attempting to create a subclass SomeClass
results in a compile-time error.
These are the basic uses of ‘final’ in Java, but there’s much more to learn about this keyword. In the next section, we’ll explore some advanced uses of ‘final’.
Advanced Use of ‘final’ in Java
As you become more comfortable with the ‘final’ keyword, you can start to explore its more sophisticated applications. Let’s delve into using ‘final’ with arrays, parameters, and anonymous classes.
‘final’ with Arrays
When you declare an array as ‘final’, it means you can’t change its reference to point to another array. However, you can modify its elements. Here’s an example:
final int[] FINAL_ARRAY = new int[]{1, 2, 3};
FINAL_ARRAY[0] = 10; // This is allowed
// FINAL_ARRAY = new int[]{4, 5, 6}; // This would throw an error
// Output:
// Error: cannot assign a value to final variable FINAL_ARRAY
In this code, FINAL_ARRAY
is a ‘final’ array. You can modify its elements, as shown by setting FINAL_ARRAY[0]
to 10. However, you can’t make FINAL_ARRAY
point to a different array.
‘final’ with Parameters
‘final’ parameters are parameters declared as ‘final’. A ‘final’ parameter’s value can’t be changed within the method. This can be useful when you want to ensure that a passed parameter isn’t altered. Here’s an example:
void show(final int NUM) {
// NUM = 10; // This would throw an error
}
// Output:
// Error: cannot assign a value to final variable NUM
In this code, NUM
is a ‘final’ parameter in the show
method. Any attempt to change its value within the method results in a compile-time error.
‘final’ with Anonymous Classes
In anonymous classes, variables from the enclosing scope must be declared ‘final’ to be accessed. Here’s an example:
int num = 10;
Runnable r = new Runnable() {
public void run() {
// System.out.println(num); // This would throw an error
}
};
// Output:
// Error: local variables referenced from an inner class must be final or effectively final
In this code, num
is a variable in the scope of the anonymous class Runnable
. To access num
within the run
method, num
must be declared as ‘final’.
These advanced uses of ‘final’ can provide more control and precision in your Java coding. In the next section, we’ll explore alternative approaches to using ‘final’.
Alternatives to ‘final’ in Java
While ‘final’ is a powerful tool in Java, there are alternative approaches to achieve similar results. These alternatives can be particularly useful in certain scenarios. Let’s explore using private methods and composition as alternatives to ‘final’.
Private Methods as an Alternative
One way to prevent a method from being overridden, similar to using ‘final’, is to declare it as private. Private methods are not visible to subclasses and thus cannot be overridden.
class ParentClass {
private void show() {
System.out.println("This is a private method.");
}
}
class ChildClass extends ParentClass {
// Attempting to override show() would result in a compile-time error
}
// Output:
// Error: show() has private access in ParentClass
In this example, the show()
method is private, so it cannot be overridden in ChildClass
. However, using private methods limits the method’s visibility, which may not be desirable in all scenarios.
Composition Over Inheritance
Another alternative to using ‘final’ with classes is to use composition over inheritance. This design principle suggests using composition (combining simple objects to create more complex ones) instead of inheritance to achieve code reuse and flexibility.
class Engine {
void start() {
System.out.println("Engine started.");
}
}
class Car {
private Engine engine = new Engine();
void startEngine() {
engine.start();
}
}
In this example, instead of Car
inheriting from Engine
, Car
has an Engine
. This allows Car
to control access to the Engine
‘s methods and prevents the Engine
class from being subclassed.
While these alternatives can achieve similar results to using ‘final’, they have their own benefits and drawbacks. Understanding these can help you make more informed decisions when designing your Java programs.
Troubleshooting ‘final’ in Java
While ‘final’ is a powerful keyword in Java, it’s not without its complexities. Let’s explore some common issues and their solutions when using ‘final’.
Uninitialized ‘final’ Variables
One common error is declaring a ‘final’ variable without initializing it. ‘final’ variables must be initialized at the time of declaration.
final int MAX_AGE;
// This would throw an error
// Output:
// Error: variable MAX_AGE might not have been initialized
In this code, MAX_AGE
is a ‘final’ variable that is not initialized, resulting in a compile-time error. To fix this, you should initialize MAX_AGE
at the time of declaration.
Overriding ‘final’ Methods
Another common error is attempting to override a ‘final’ method. ‘final’ methods can’t be overridden.
class ParentClass {
final void show() {
System.out.println("This is a final method.");
}
}
class ChildClass extends ParentClass {
void show() { // This would throw an error
System.out.println("This is a child method.");
}
}
// Output:
// Error: show() in ChildClass cannot override show() in ParentClass
// overridden method is final
In this code, show()
in ChildClass
attempts to override the ‘final’ method show()
in ParentClass
, resulting in a compile-time error. To fix this, you should remove the ‘final’ keyword from the method in the parent class if you want it to be overridden.
Extending ‘final’ Classes
A ‘final’ class can’t be extended. Attempting to do so will result in a compile-time error.
final class FinalClass {
// Some methods and variables
}
class SomeClass extends FinalClass { // This would throw an error
// Some methods and variables
}
// Output:
// Error: cannot inherit from final FinalClass
In this code, SomeClass
attempts to extend the ‘final’ class FinalClass
, resulting in a compile-time error. To fix this, you should remove the ‘final’ keyword from the class definition if you want it to be extended.
Remember, understanding these common issues and their solutions can help you use the ‘final’ keyword more effectively in your Java programs.
Java’s Object-Oriented Principles and ‘final’
To fully understand the power and utility of the ‘final’ keyword, it’s crucial to grasp Java’s fundamental object-oriented principles. These principles include inheritance, encapsulation, and polymorphism, all of which interact with ‘final’ in different ways.
Inheritance and ‘final’
Inheritance allows a new class to inherit the members (fields and methods) of an existing class. The ‘final’ keyword can restrict this inheritance. When a class is declared as ‘final’, it can’t be extended. Similarly, a ‘final’ method can’t be overridden by subclasses.
final class FinalClass {
// Some methods and variables
}
class SomeClass extends FinalClass { // This would throw an error
// Some methods and variables
}
// Output:
// Error: cannot inherit from final FinalClass
In this example, SomeClass
attempts to extend the ‘final’ class FinalClass
, resulting in a compile-time error.
Encapsulation and ‘final’
Encapsulation is the technique of making the fields in a class private and providing access to the fields via public methods. If a field is declared public, it can be accessed from anywhere. ‘final’ variables can be used to create constants that are accessible but not modifiable.
public final int MAX_SPEED = 120;
// MAX_SPEED can be accessed but not modified
In this code, MAX_SPEED
is a ‘final’ variable that can be accessed from any class, but its value can’t be changed.
Polymorphism and ‘final’
Polymorphism allows a subclass to behave as its superclass. This is typically achieved by overriding methods in the superclass. However, ‘final’ methods can’t be overridden, which restricts polymorphism.
class ParentClass {
final void show() {
System.out.println("This is a final method.");
}
}
class ChildClass extends ParentClass {
void show() { // This would throw an error
System.out.println("This is a child method.");
}
}
// Output:
// Error: show() in ChildClass cannot override show() in ParentClass
// overridden method is final
In this example, show()
in ChildClass
attempts to override the ‘final’ method show()
in ParentClass
, resulting in a compile-time error.
In conclusion, the ‘final’ keyword plays a significant role in Java’s object-oriented principles. By understanding these principles, you can use ‘final’ more effectively in your Java programs.
Exploring ‘final’ in Larger Java Projects
The ‘final’ keyword isn’t just for small programs or simple scripts. It plays an integral role in larger Java projects as well, providing control and ensuring consistency in your codebase. Let’s delve into its application in broader contexts.
‘final’ and Immutable Classes
Immutable classes, once created, cannot be changed. They provide several benefits such as simplicity of use, thread safety, and potential performance enhancements. The ‘final’ keyword is essential in creating immutable classes in Java, as it prevents the alteration of field values once they’re set.
public final class ImmutableClass {
final private int value;
public ImmutableClass(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
In this example, ImmutableClass
is an immutable class with a ‘final’ field value
. The field can only be set once during object creation and cannot be changed afterwards.
‘final’ in Design Patterns
Design patterns represent solutions to common problems in software design. They can be classified into creational, structural, and behavioral patterns. The ‘final’ keyword often features in these patterns, such as the Singleton pattern, where a ‘final’ instance ensures only one instance of the class exists.
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
In this example, INSTANCE
is a ‘final’ variable in the Singleton
class. It ensures there’s only one Singleton
instance throughout the application.
‘final’ and Concurrency
Concurrency in Java involves executing multiple threads simultaneously. The ‘final’ keyword plays a crucial role in ensuring thread-safety, as ‘final’ variables are safe from data races.
public class ThreadSafeClass {
private final int safeValue;
public ThreadSafeClass(int value) {
this.safeValue = value;
}
public int getSafeValue() {
return safeValue;
}
}
In this example, safeValue
is a ‘final’ variable in the ThreadSafeClass
class. It ensures that the value is thread-safe and won’t be changed by multiple threads simultaneously.
Further Resources for Mastering ‘final’ in Java
To continue your learning journey with the ‘final’ keyword in Java, check out these resources:
- Mastering Variables in Java: Essential Concepts – Understand Java variables easily with clear explanations.
Access Modifiers in Java – Understand using access modifiers in Java for controlling the accessibility of class members.
Array Length in Java – Explore how to use .length in Java to retrieve the size of arrays and strings.
Oracle’s Java Tutorials provide in-depth information about the ‘final’ keyword.
Final Keyword in Java by JavaTPoint provides a detailed exploration of the ‘final’ keyword in Java.
GeeksforGeeks’ ‘final’ keyword guide offers a comprehensive look at ‘final’, complete with examples and explanations.
Wrapping Up: Mastering ‘final’ in Java
In this comprehensive guide, we’ve navigated the intricacies of the ‘final’ keyword in Java, a powerful tool for controlling the behavior of your code.
We started with the basics, learning how to use ‘final’ with variables, methods, and classes. We then dived deeper, exploring more complex uses of ‘final’ with arrays, parameters, and anonymous classes. We also discussed alternative approaches, such as using private methods or composition instead of inheritance, to achieve similar results without using ‘final’.
Along the way, we tackled common challenges you might encounter when using ‘final’, such as uninitialized ‘final’ variables, overriding ‘final’ methods, and extending ‘final’ classes, providing you with solutions and workarounds for each issue.
We also looked at how ‘final’ interacts with Java’s fundamental object-oriented principles, such as inheritance, encapsulation, and polymorphism. Finally, we explored the use of ‘final’ in larger Java projects, discussing its role in creating immutable classes, implementing design patterns, and ensuring thread safety in concurrent programming.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘final’ with Variables | Creates constant variables | Requires initialization at declaration |
‘final’ with Methods | Prevents method overriding | Limits flexibility in subclasses |
‘final’ with Classes | Prevents class extension | Limits code reuse through inheritance |
Private Methods | Prevents method overriding | Limits method visibility |
Composition Over Inheritance | Provides code reuse and flexibility | More complex than inheritance |
Whether you’re just starting out with ‘final’ in Java or you’re looking to deepen your understanding, we hope this guide has given you a comprehensive overview of the ‘final’ keyword and its applications.
With its ability to control the behavior of variables, methods, and classes, ‘final’ is a key tool in any Java developer’s toolbox. Happy coding!