Java Modulus/Modulo: Your Guide to Remainder Operations
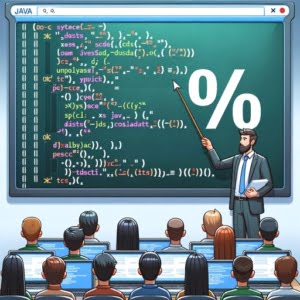
Ever found yourself puzzled with the modulus operator in Java? You’re not alone. Many developers find themselves in a similar situation when it comes to understanding and using the modulus operator in Java. Think of Java’s modulus operator as a skilled mathematician – it performs modulus operations to find the remainder of a division, a fundamental operation in many programming tasks.
In this guide, we’ll walk you through the process of using the modulus operator in Java, from the basics to more advanced techniques. We’ll cover everything from simple modulus operations to its use in loops and with negative numbers, as well as alternative approaches and troubleshooting common issues.
Let’s dive in and start mastering the modulus operator in Java!
TL;DR: How Do I Use the Modulus Operator in Java?
The modulus operator in Java is used to find the remainder of a division operation. It’s represented by the
'%'
symbol. For instance, to find the remainder of 10 divided by 3, you would useint result = 10 % 3;
.
Here’s a simple example:
int result = 10 % 3;
System.out.println(result);
# Output:
# 1
In this example, we use the modulus operator to find the remainder of 10 divided by 3. The result is 1, which is printed to the console.
This is just a basic use of the modulus operator in Java. There’s much more to learn about using this operator in different contexts and scenarios. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding the Modulus Operator in Java
- Modulus Operator: Advanced Scenarios
- Exploring Alternatives to the Modulus Operator
- Troubleshooting Common Issues with Java’s Modulus Operator
- Mathematical Foundation of Modulus Operation
- Modulus Operation in Java
- Expanding the Use of Modulus Operator in Java
- Wrapping Up: Mastering the Modulus Operator in Java
Understanding the Modulus Operator in Java
The modulus operator, represented as %
, is a mathematical operator that’s used to find the remainder of a division operation. In Java, it’s often used to solve problems where you need to divide two integers and are interested in the remainder.
Let’s take a look at a basic example:
int dividend = 25;
int divisor = 7;
int result = dividend % divisor;
System.out.println(result);
# Output:
# 4
In this example, we’re dividing 25 by 7. The quotient is 3 and the remainder is 4. The modulus operator %
returns this remainder.
Advantages of the Modulus Operator
The modulus operator is incredibly useful in a variety of scenarios. For instance, it can be used to determine if a number is even or odd (any number % 2 will return 0 for even numbers and 1 for odd numbers). It’s also handy in scenarios where you need to cycle through a range of values.
Potential Pitfalls
While the modulus operator is simple to use, there are a few things to keep in mind. First, the modulus operator can only be used with integers – using it with floating-point numbers can lead to unexpected results. Second, be mindful of the possibility of a ArithmeticException
being thrown when the divisor is 0.
Modulus Operator: Advanced Scenarios
While the modulus operator is straightforward in its basic use, there are more complex scenarios where it becomes invaluable. Let’s explore a couple of these scenarios: using the modulus operator in loops and with negative numbers.
Modulus in Loops
The modulus operator is often used in loops, especially when we want to perform an action at regular intervals. Here’s an example of how you can use it to print a message every five iterations in a loop:
for (int i = 1; i <= 20; i++) {
if (i % 5 == 0) {
System.out.println("Reached a multiple of 5: " + i);
}
}
# Output:
# Reached a multiple of 5: 5
# Reached a multiple of 5: 10
# Reached a multiple of 5: 15
# Reached a multiple of 5: 20
In this example, i % 5 == 0
checks if the current iteration number is a multiple of 5. If it is, it prints a message.
Modulus with Negative Numbers
When dealing with negative numbers, the modulus operator can yield results that might initially seem surprising. Let’s take a look:
int result = -10 % 3;
System.out.println(result);
# Output:
# -1
Here, the modulus operation -10 % 3
results in -1
. This is because the sign of the result is the same as the dividend (the number being divided).
Exploring Alternatives to the Modulus Operator
While the modulus operator is a powerful tool, sometimes you might need a different approach to solve your problem. Java provides a few alternatives that can accomplish similar tasks. Let’s take a look at these alternatives and understand when to use them.
The Math.floorMod Method
Java’s Math library provides a method called floorMod
which can be used as an alternative to the modulus operator. This method also returns the remainder of a division operation, but it behaves differently when working with negative numbers.
Here’s an example:
int result = Math.floorMod(-10, 3);
System.out.println(result);
# Output:
# 2
In this example, Math.floorMod(-10, 3)
returns 2
, whereas -10 % 3
would have returned -1
. This is because floorMod
rounds the quotient towards negative infinity, while the modulus operator rounds towards zero.
The Benefits and Drawbacks
The floorMod
method can be beneficial when you’re working with negative numbers and you want the result to always be positive. However, it’s important to note that floorMod
is slower than the modulus operator, so if performance is a concern, the modulus operator might be a better choice.
Making the Right Decision
When deciding between the modulus operator and floorMod
, consider the nature of your problem and the data you’re working with. If you’re dealing with positive numbers or you want the sign of the result to match the dividend, the modulus operator is the way to go. If you’re working with negative numbers and you want a positive result, floorMod
might be a better choice.
Troubleshooting Common Issues with Java’s Modulus Operator
While the modulus operator in Java is quite straightforward, you might encounter some common errors or obstacles when using it. Let’s discuss some of these issues and their solutions.
Division by Zero
One of the most common errors when using the modulus operator is trying to divide by zero. This will result in an ArithmeticException
being thrown. Here’s an example:
try {
int result = 10 % 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
# Output:
# Cannot divide by zero!
In this example, we’re trying to divide 10 by 0, which is mathematically undefined. Java throws an ArithmeticException
, which we catch and print a custom error message.
Using Modulus with Non-Integers
Another common issue is trying to use the modulus operator with non-integer numbers. While Java does allow this, it can lead to unexpected results due to the way floating-point numbers are represented internally. Here’s an example:
double result = 10.5 % 3.2;
System.out.println(result);
# Output:
# 1.1
In this example, we’re using the modulus operator with floating-point numbers. The result might not be what you expect due to the precision issues inherent with floating-point numbers.
Best Practices and Optimization
When using the modulus operator, it’s best to stick to integer numbers to avoid precision issues. Also, always check if your divisor is zero before performing a modulus operation to prevent an ArithmeticException
. Remember, while alternatives like Math.floorMod
can be useful in certain scenarios, they are slower than the modulus operator, so use them judiciously.
Mathematical Foundation of Modulus Operation
Before diving deeper into the programming aspect, it’s essential to understand the mathematical concept of ‘modulus’. In mathematics, the modulus operation finds the remainder after the division of one number by another (called the divisor).
For instance, if we divide 10 by 3, the quotient is 3 and the remainder is 1. The number ‘1’ is the modulus of 10 divided by 3.
int modulus = 10 % 3;
System.out.println(modulus);
# Output:
# 1
In this code block, we’re applying the modulus operation in Java. The %
operator performs the modulus operation and the result, ‘1’, is printed out.
Modulus Operation in Java
In Java, the modulus operation is implemented using the %
operator. This operator returns the remainder of a division operation. It’s a binary operator that requires two operands: the dividend (the number to be divided) and the divisor (the number by which the dividend is divided).
The modulus operator in Java can be used with both integers and floating-point numbers. However, it’s most commonly used with integers, as using it with floating-point numbers can lead to unexpected results due to precision issues.
Expanding the Use of Modulus Operator in Java
The modulus operator in Java is not just limited to simple arithmetic operations. It’s an integral part of many larger programs and projects, especially those involving mathematical computations, data analysis, algorithms, and more.
Modulus in Real-World Applications
In real-world applications, the modulus operator is often used in conjunction with other operators and methods. For instance, it’s frequently used in algorithms to hash data into a specific range of values. It can also be used in cryptography, random number generation, and even in creating certain types of animations in game development.
Accompanying Operators and Methods
The modulus operator often comes into play with other operators and methods. For instance, the division operator /
is closely related, as both are used in division operations but yield different results. The Math.floorDiv
method, which rounds the quotient towards negative infinity, is another method related to the modulus operation.
Further Resources for Mastering Java Operators
If you’re interested in learning more about the modulus operator and other related operators or methods in Java, here are a few resources to explore:
- IOFlood’s Guide to Operators in Java – Understand how Java handles overflow and underflow with arithmetic operators.
Expression Concepts in Java – Understand the arithmetic, relational, logical, and assignment expressions in Java.
The .equals Method Usage – Explores how the “.equals()” method differs from the
"=="
operator for comparing Java objects.Oracle’s Java Documentation contains in-depth information about all the operators and methods in Java.
GeeksforGeeks’ Java Operators Article provides an overview of operators in Java, including the modulus operator.
JavaTpoint’ Java Tutorials covers various topics, including the modulus operator.
Wrapping Up: Mastering the Modulus Operator in Java
In this comprehensive guide, we’ve delved into the world of Java’s modulus operator, exploring its uses, benefits, and potential pitfalls.
We began with the basics, understanding how to use the modulus operator in simple arithmetic operations. We then ventured into more complex scenarios, such as using the modulus operator in loops and with negative numbers. We also discussed common issues that you might encounter when using the modulus operator, such as division by zero and precision issues with non-integer numbers, and provided solutions to these challenges.
We also explored alternative approaches to the modulus operation, like the Math.floorMod
method, and compared their benefits and drawbacks. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Modulus Operator | Fast, straightforward | Can lead to unexpected results with non-integers |
Math.floorMod Method | Always returns a positive result, handles negative numbers well | Slower than the modulus operator |
Whether you’re a beginner just starting to learn Java or a seasoned developer looking to brush up on your skills, we hope this guide has given you a deeper understanding of the modulus operator in Java and its applications.
Understanding and effectively using the modulus operator is a valuable skill in Java programming. With the knowledge you’ve gained from this guide, you’re well-equipped to use this operator in your future projects. Happy coding!