Java Serializable Interface: Object Serialization Guide
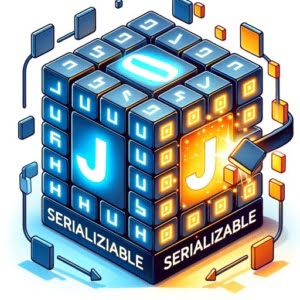
Are you finding it challenging to serialize objects in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like packing a suitcase for a trip, Java serialization allows you to bundle up an object and send it wherever you want. This process is crucial in various scenarios, such as when you need to save an object’s state to a file or send it over the network to a different Java runtime environment.
This guide will walk you through the process of making a class serializable in Java, from the basics to more advanced techniques. We’ll explore Java’s Serializable interface, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java Serializable!
TL;DR: How Do I Make a Class Serializable in Java?
To make a class serializable in Java, you simply implement the
Serializable
interface. This interface marks your class as capable of being serialized and deserialized.
Here’s a basic example:
import java.io.Serializable;
public class MyClass implements Serializable {
// class contents...
}
In this example, we’ve created a class MyClass
and made it implement the Serializable
interface. This simple declaration allows MyClass
to be serialized and deserialized, enabling it to be saved to a file or sent over a network.
This is just the beginning of what you can do with Java Serializable. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Making a Class Serializable in Java
- Serializing and Deserializing Objects
- Serializing Custom Objects
- Handling Object References
- Exploring Other Methods of Serialization
- Troubleshooting Common Serialization Issues
- Understanding Java Serialization
- The Role of the Serializable Interface
- Serialization in Larger Projects
- Related Topics
- Wrapping Up: Java Serializable Interface
Making a Class Serializable in Java
To make a class serializable in Java, it needs to implement the Serializable
interface. This interface is a marker interface, meaning it doesn’t contain any methods for a class to implement. It simply flags the Java Virtual Machine (JVM) that this class can be serialized and deserialized.
Here’s how you can make a simple Person
class serializable:
import java.io.Serializable;
public class Person implements Serializable {
private String name;
private int age;
// Constructor, getters, and setters...
}
In this example, the Person
class implements the Serializable
interface, making it possible to serialize and deserialize Person
objects.
Serializing and Deserializing Objects
Once you have a serializable class, you can now serialize and deserialize its objects. Java provides the ObjectOutputStream
and ObjectInputStream
classes in the java.io
package for this purpose.
Here’s a simple example of serializing and then deserializing a Person
object:
import java.io.*;
public class Main {
public static void main(String[] args) {
Person person = new Person("John", 30);
try {
// Serialize
FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(person);
out.close();
fileOut.close();
// Deserialize
FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Person deserializedPerson = (Person) in.readObject();
in.close();
fileIn.close();
System.out.println("Deserialized Person: " + deserializedPerson.getName());
} catch (IOException i) {
i.printStackTrace();
} catch (ClassNotFoundException c) {
System.out.println("Person class not found");
c.printStackTrace();
}
}
}
// Output:
// Deserialized Person: John
In this example, we first create a Person
object named person
. We then use ObjectOutputStream
to write the person
object to a file named person.ser
. This process is known as serialization.
Next, we use ObjectInputStream
to read the person.ser
file and recreate the Person
object. This process is known as deserialization. The readObject()
method returns an Object
, so we need to cast it back to Person
. Finally, we print the name of the deserialized Person
to the console, which outputs John
.
Serializing Custom Objects
Java serialization goes beyond simple classes and can handle more complex, custom objects. Let’s look at an example where a Person
object has a reference to an Address
object:
import java.io.Serializable;
public class Address implements Serializable {
private String street;
private String city;
// Constructor, getters, and setters...
}
public class Person implements Serializable {
private String name;
private int age;
private Address address;
// Constructor, getters, and setters...
}
In this example, the Person
class contains an Address
object. Both classes implement the Serializable
interface, which is necessary because when a Person
object is serialized, its Address
object is also serialized.
Handling Object References
Java serialization can handle objects with multiple references. When an object is serialized, all of its references are serialized as well.
Let’s look at an example where two Person
objects share the same Address
object:
public class Main {
public static void main(String[] args) {
Address address = new Address("123 Main St", "Springfield");
Person person1 = new Person("John", 30, address);
Person person2 = new Person("Jane", 28, address);
// Serialize and deserialize person1 and person2...
}
}
In this example, person1
and person2
share the same Address
object. When person1
and person2
are serialized, the Address
object is serialized only once. During deserialization, the Address
object is restored only once and shared by person1
and person2
. This is because Java serialization maintains the object graph, preserving the shared references.
This feature is important because it not only reduces the size of the serialized data but also maintains the object relationships in the deserialized objects.
Exploring Other Methods of Serialization
Java provides alternative methods for serialization, such as using the Externalizable
interface or third-party libraries like Gson and Jackson. These offer more control and flexibility compared to the Serializable
interface.
Using the Externalizable
Interface
The Externalizable
interface gives you more control over the serialization process. This interface allows you to define custom write and read methods for your objects.
Here’s an example of how to use the Externalizable
interface:
import java.io.*;
public class Person implements Externalizable {
private String name;
private int age;
// Constructor, getters, and setters...
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeObject(name);
out.writeInt(age);
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
name = (String) in.readObject();
age = in.readInt();
}
}
In this example, we define writeExternal
and readExternal
methods in the Person
class. These methods are called during serialization and deserialization, respectively.
Using Third-Party Libraries
Third-party libraries like Gson and Jackson offer more features and flexibility for serialization. For instance, they can serialize objects to and from JSON, which is a common requirement in modern applications.
Here’s an example of how to use Gson for serialization:
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
Person person = new Person("John", 30);
Gson gson = new Gson();
// Serialize
String json = gson.toJson(person);
System.out.println(json);
// Deserialize
Person deserializedPerson = gson.fromJson(json, Person.class);
System.out.println(deserializedPerson.getName());
}
}
// Output:
// {"name":"John","age":30}
// John
In this example, we use the Gson library to serialize and deserialize a Person
object to and from JSON. The toJson
method serializes the object to a JSON string, and the fromJson
method deserializes the JSON string back to a Person
object.
These alternative methods provide more control and flexibility than the Serializable
interface. However, they also require more work and understanding of the serialization process. Depending on your needs, you might prefer to use the Serializable
interface for its simplicity, or one of these alternative methods for their additional features and control.
Troubleshooting Common Serialization Issues
Like any process in programming, serialization can encounter issues. One common problem with Java serialization is the NotSerializableException
. Let’s discuss how to troubleshoot this and other issues, and explore some best practices for serialization.
Handling NotSerializableException
The NotSerializableException
is thrown when an instance of a class is required to have a Serializable
interface. For example, if you try to serialize an object of a class that doesn’t implement Serializable
, you’ll encounter this exception.
public class MyClass {
// class contents...
}
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
try {
FileOutputStream fileOut = new FileOutputStream("myclass.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(myClass);
out.close();
fileOut.close();
} catch (IOException i) {
i.printStackTrace();
}
}
}
// Output:
// java.io.NotSerializableException: MyClass
In this example, the MyClass
class doesn’t implement Serializable
, so trying to serialize an object of MyClass
throws a NotSerializableException
.
The solution to this issue is simple: make the class implement the Serializable
interface.
public class MyClass implements Serializable {
// class contents...
}
Best Practices for Serialization
Follow these best practices to avoid potential pitfalls during serialization:
- Implement
Serializable
carefully: Remember that once a class implementsSerializable
, its subclasses are also serializable. Be sure that serialization is appropriate for an entire class hierarchy. Use the
transient
keyword for non-serializable fields: If your class has fields that can’t or shouldn’t be serialized (like a Thread or a File), declare them astransient
. This keyword tells the JVM to ignore these fields during serialization.Consider thread safety: If multiple threads can access your serializable class, make sure to synchronize the serialization process to prevent inconsistent object state.
Use version control with
serialVersionUID
: When a serializable class is modified, it’s a good practice to update aserialVersionUID
field in the class. This field helps the deserialization process to verify that a loaded class and a serialized object are compatible.
Understanding Java Serialization
Serialization is a mechanism of converting the state of an object into a byte stream. It’s essentially a way to ‘flatten’ an object into a format that can be stored or transmitted and then reconstructed later. This process is crucial in several contexts, such as storing data on disk, transmitting data over a network, or caching objects in memory.
But why is serialization important? Imagine you’re working on a multiplayer online game. The game’s state, including players’ positions, scores, and game settings, needs to be consistently updated and shared between the server and clients. Serialization allows you to convert these complex game states into a format that can be easily transmitted over the network.
public class GameState implements Serializable {
private List<Player> players;
private GameSettings settings;
// Constructor, getters, and setters...
}
public class Main {
public static void main(String[] args) {
GameState gameState = new GameState(players, settings);
// Serialize and transmit gameState...
}
}
In this example, the GameState
class, which includes a list of Player
objects and a GameSettings
object, is made serializable. This allows the game state to be easily transmitted between the server and clients.
The Role of the Serializable
Interface
In Java, the Serializable
interface plays a key role in serialization. It’s a marker interface, meaning it does not contain any methods. When a class implements this interface, it tells the Java Virtual Machine (JVM) that objects of this class can be serialized and deserialized.
The Serializable
interface is part of the java.io
package, and it works hand in hand with ObjectOutputStream
and ObjectInputStream
, the core classes in Java’s serialization API.
Implementing Serializable
is as simple as adding implements Serializable
to your class declaration. Once implemented, you can use ObjectOutputStream.writeObject()
to serialize an object and ObjectInputStream.readObject()
to deserialize an object.
Serialization in Larger Projects
Serialization plays a crucial role in larger projects, especially in distributed systems and caching mechanisms. It allows data to be converted into a format that can be easily stored or transmitted.
Serialization in Distributed Systems
In distributed systems, data needs to be shared between different systems or components. Serialization allows complex objects to be converted into a byte stream, which can be easily transmitted over a network.
public class DistributedSystem {
// Send object over network
public void sendObject(Object obj, ObjectOutputStream out) throws IOException {
out.writeObject(obj);
}
// Receive object from network
public Object receiveObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
return in.readObject();
}
}
In this example, the sendObject
method serializes an object and sends it over a network, and the receiveObject
method receives a serialized object from the network and deserializes it.
Serialization in Caching
Caching mechanisms often use serialization to store data. Serialized objects can be stored in memory or on disk, allowing data to be quickly accessed when needed.
public class Cache {
private Map<String, byte[]> cache = new HashMap<>();
// Store object in cache
public void storeObject(String key, Object obj) throws IOException {
ByteArrayOutputStream byteOut = new ByteArrayOutputStream();
ObjectOutputStream out = new ObjectOutputStream(byteOut);
out.writeObject(obj);
cache.put(key, byteOut.toByteArray());
}
// Retrieve object from cache
public Object retrieveObject(String key) throws IOException, ClassNotFoundException {
byte[] bytes = cache.get(key);
ByteArrayInputStream byteIn = new ByteArrayInputStream(bytes);
ObjectInputStream in = new ObjectInputStream(byteIn);
return in.readObject();
}
}
In this example, the storeObject
method serializes an object and stores it in a cache, and the retrieveObject
method retrieves a serialized object from the cache and deserializes it.
Related Topics
If you’re interested in learning more about Java serialization, you might want to explore related topics such as Java I/O, networking, and multithreading. These topics provide a deeper understanding of how Java handles data and can help you better understand and use serialization.
Further Resources for Java Serialization
- Java Interface: Tips and Techniques – Understand how interfaces enable loose coupling between components.
Java Iterator Interface Guide – Understand the Java Iterator interface for traversing elements in collections
JDBC Usage in Java – Master JDBC usage for database operations such as querying and updating data.
Oracle’s Java Tutorials: Serialization: A comprehensive guide on Java serialization from the creators of Java.
Baeldung’s Guide to Java Deserialization includes how to handle different types of objects.
InfoWorld’s Understand the Java Serialization API explains the Java Serialization API, including numerous code examples.
Wrapping Up: Java Serializable Interface
In this comprehensive guide, we’ve delved into the world of Java Serializable, a key tool in Java for object serialization.
We embarked on our journey with the basics, learning how to make a class serializable and how to serialize and deserialize objects. We then ventured into more advanced territory, handling the serialization of custom objects and those with multiple references.
We also explored alternative approaches to serialization, such as using the Externalizable
interface or third-party libraries like Gson and Jackson. Each of these methods offers its own advantages, giving you a range of options depending on your specific needs.
Along the way, we tackled common challenges you might encounter during serialization, such as the NotSerializableException
, and provided solutions to help you overcome these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Control | Flexibility | Complexity |
---|---|---|---|
Serializable | Moderate | Moderate | Low |
Externalizable | High | High | High |
Gson/Jackson | High | High | Moderate |
Whether you’re just starting out with Java Serializable or you’re looking to level up your serialization skills, we hope this guide has given you a deeper understanding of Java Serializable and its capabilities.
With its balance of control, flexibility, and simplicity, Java Serializable is a powerful tool for object serialization in Java. Now, you’re well equipped to handle any serialization task that comes your way. Happy coding!