Python If Not | How To Use Advanced Python Conditionals
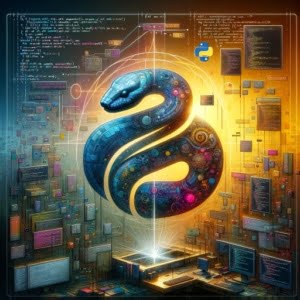
Python programming has revolutionized the tech landscape with its simplicity, versatility, and power, making it a go-to language for beginners and seasoned professionals alike. Used in diverse fields from web development to data science, Python’s appeal lies in its straightforward syntax and logical constructs. A key part of these constructs is the ‘if not’ statement, a tool that adds depth to your code by allowing you to test the opposite of a condition.
This article aims to provide a comprehensive understanding of the ‘if not’ statement in Python. We will dissect its structure, explore its interaction with different data types, and showcase its versatility through practical examples. Whether you’re a Python novice or an experienced coder wanting to refresh your knowledge, this article has something for you. Let’s get started!
TL;DR: What is the ‘if not’ statement in Python?
The ‘if not’ statement in Python is a logical construct that tests the opposite of a given condition. If the condition is not met, the code under this statement gets executed. It’s a handy tool for enhancing code logic and readability. For more advanced usage, tips, and tricks, continue reading the article.
name = ""
if not name:
print("Name is empty.")
In this simple example, the ‘if not’ statement checks if the ‘name’ variable is empty and prints a message if it is.
Table of Contents
Understanding Python “If Not”
In Python, ‘if not’ is a logical construct that flips the outcome of a condition. It’s a fusion of the ‘if’ statement and the ‘not’ operator, both of which are integral to Python programming. The ‘if’ statement in Python tests a specific condition and executes a block of code based on the result. The ‘not’ operator, conversely, is a logical operator that returns True if the condition is False, and vice versa.
When these two elements unite, they form the ‘if not’ statement, which tests the inverse of a condition. Essentially, it permits the execution of a block of code when a particular condition is not met. Consider the following code for a clearer understanding:
name = ""
if not name:
print("Name is empty.")
In this instance, the ‘if not’ statement checks if the variable ‘name’ is empty. If it is (i.e., the condition is True), it executes the print statement.
While using ‘if not’ in combination with other logical operators such as ‘and’ and ‘or’, it’s crucial to use parentheses to ensure the correct order of operations. For instance:
name = "John"
age = 15
if not (name == "John" and age > 18):
print("Access denied.")
In this code snippet, the ‘if not’ statement checks if the user is not John or is under 18. If either condition is met, the print statement is executed.
Here is a table summarizing the different combinations of ‘if not’ with ‘and’ and ‘or’ operators:
Condition | Result |
---|---|
if not (True and True) | False |
if not (True and False) | True |
if not (False and True) | True |
if not (False and False) | True |
if not (True or True) | False |
if not (True or False) | False |
if not (False or True) | False |
if not (False or False) | True |
The ‘if not’ statement is a potent tool in Python programming. It simplifies complex logical expressions and enhances code readability by reversing the output of an ‘if’ statement. Grasping its syntax and usage is key to writing efficient and effective Python code.
Delving into Boolean Operators in Python
Boolean operators in Python are instrumental in building logical conditions. There are three main Boolean operators: ‘not’, ‘and’, and ‘or’. Each operator serves a unique purpose.
The ‘not’ operator is a unary operator that flips the truth value of the operand. For instance, if x is True, ‘not x’ is False, and vice versa.
x = True
print(not x) # Outputs: False
The ‘and’ operator returns True only if both operands are true. If either operand (or both) is False, ‘and’ returns False.
x = True
y = False
print(x and y) # Outputs: False
The ‘or’ operator returns True if either operand (or both) is true. If both operands are False, ‘or’ returns False.
x = True
y = False
print(x or y) # Outputs: True
Understanding the precedence of these operators is essential when working with them. The ‘not’ operator has the highest precedence, followed by ‘and’, and then ‘or’. This means that in a complex expression, ‘not’ will be evaluated first, followed by ‘and’, and then ‘or’.
The ‘if not’ statement can be utilized in tandem with these operators to evaluate complex conditions. For example, you can use ‘if not’ to verify if a user is not logged in or if their session has expired.
logged_in = False
session_expired = True
if not logged_in or session_expired:
print("Please log in or renew your session.")
In this scenario, the code block will be executed if the user is not logged in or if their session has expired.
Boolean operators can be employed to formulate more intricate conditions and expressions in Python. They bolster the logic of your code, enabling you to manage a wide array of scenarios. Gaining proficiency in using these operators is a crucial step towards mastering Python programming.
Exploring Conditional Statements in Python
Conditional statements are the backbone of Python programming, dictating the flow of your program by enabling it to make decisions based on specific conditions.
Python offers three types of conditional statements: ‘if’, ‘else’, and ‘elif’.
The ‘if’ statement tests a specific condition. If the condition is true, the code within the ‘if’ block executes. If not, the code is bypassed.
x = 10
if x > 5:
print("x is greater than 5")
In this scenario, as x is indeed greater than 5, the print statement within the ‘if’ block executes.
The ‘else’ statement specifies a block of code to be executed if the condition in the ‘if’ statement is not met.
x = 10
if x > 15:
print("x is greater than 15")
else:
print("x is not greater than 15")
Here, since x is not greater than 15, the print statement within the ‘else’ block executes.
The ‘elif’ statement (short for ‘else if’) specifies a new condition to be tested if the previous condition was not met.
x = 10
if x > 15:
print("x is greater than 15")
elif x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
In this example, as x is not greater than 15 but is greater than 5, the print statement within the ‘elif’ block executes.
The ‘not’ keyword, when used with these statements, reverses the result of a condition. For instance, you can use ‘if not’ to check if a list is empty.
my_list = []
if not my_list:
print("The list is empty")
In this code snippet, the ‘if not’ statement checks if ‘my_list’ is empty. If it is (i.e., the condition is True), it executes the print statement.
Here is a table summarizing the behavior of ‘if not’ with different data types:
Data Type | Condition | Result |
---|---|---|
Numbers | if not 0 | True |
Numbers | if not 1 | False |
Strings | if not “” | True |
Strings | if not “Hello” | False |
Lists | if not [] | True |
Lists | if not [1, 2, 3] | False |
Here is an example of using ‘if not’ with the ‘else’ statement:
my_list = []
if not my_list:
print("The list is empty")
else:
print("The list is not empty")
The ‘not’ keyword is a powerful tool for manipulating and evaluating the outcome of Boolean expressions. It can make your conditional statements more efficient and your code more readable. Understanding how to use it effectively is a key step in mastering Python programming.
Utilizing ‘If Not’ with Python Data Types
The ‘if not’ statement in Python is an incredibly adaptable tool, capable of working with a variety of data types. It interacts with numbers, strings, lists, tuples, dictionaries, sets, and ranges, marking it as an indispensable tool for Python programmers.
Let’s delve deeper into how ‘if not’ operates with these different data types.
- Numbers: When dealing with numbers, ‘if not’ verifies whether the value is zero (0). If it is, the condition becomes True.
x = 0
if not x:
print("x is zero")
- Strings: For strings, ‘if not’ examines whether the string is empty. If it is, the condition becomes True.
str = ""
if not str:
print("The string is empty")
- Lists, Tuples, Dictionaries, Sets, and Ranges: In the case of these iterable data types, ‘if not’ assesses whether the iterable is empty. If it is, the condition becomes True.
my_list = []
if not my_list:
print("The list is empty")
Comprehending how ‘if not’ interacts with different data types is vital for crafting efficient Python code. It empowers you to verify if an iterable is not empty, if a specific condition is not met, or if a variable holds a false or zero value. This positions ‘if not’ as a robust tool for controlling the execution of your code based on the value or state of your data.
By mastering the use of ‘if not’ with different data types, you can elevate your coding skills, crafting more efficient and effective Python programs. The power and utility of ‘if not’ are immense—it truly is a must-have tool in every Python programmer’s arsenal.
Further Resources for Python Conditionals
To broaden your knowledge of Python conditional statements, here are some handpicked resources that could be particularly helpful:
- Python If Statement: Code Control Logic – Explore real-world projects and applications of Python’s if statements.
Python’s Walrus Operator – Master the art of streamlining assignments with the walrus operator in Python.
Python Ternary Operator – Simplify conditionals with ternary operators and understand their role in conditional assignments.
Python’s official documentation provides a detailed explanation of assignment expressions.
Python’s official tutorial on control flow tools discusses how the walrus operator can be used in control flow.
The Official Python Documentation: Boolean Operations provides an overview of booleans and operators in Python.
By studying these resources and deepening your understanding of conditional statements in Python, you’re paving the way to becoming a more skilled Python developer.
Conclusion: Python ‘If Not’ summary
Through our journey, we’ve unraveled the significant role of the ‘if not’ statement in Python programming. Its simplicity belies its power, offering a streamlined way to test the inverse of a condition. Regardless of whether you’re dealing with numbers, strings, or various iterable data types, ‘if not’ stands as a versatile tool that bolsters the logic of your code.
When used alongside other Boolean operators, ‘if not’ can be employed to create complex conditions, thereby enhancing the functionality of your programs. Its ability to interact with different data types extends its utility, allowing you to control code execution based on the emptiness of data or false value.
Unlock the full potential of Python with this comprehensive cheat sheet to Python Syntax.
As you continue to refine your Python skills, remember the power and versatility of ‘if not’. It’s more than just a statement—it’s a testament to Python’s accessibility and flexibility as a programming language. And just like a gate that only opens when a specific condition is not met, ‘if not’ is your gatekeeper to efficient coding in Python.