Python input() Function Guide (With Examples)
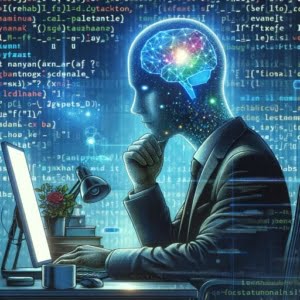
Have you ever been curious about how to make your Python programs more interactive? The answer lies in a single, potent function – input()
.
This function is your key to user interaction in Python, enabling your programs to receive user data and respond in kind. From a simple program that greets the user by name to a complex data-entry system, the
input()
function is a tool you can’t do without.
In this comprehensive guide, We’ll journey through the input()
functions basic usage, plunge into more advanced topics, and even tackle common issues you might face. Whether you’re a Python novice or a seasoned programmer seeking a refresher, this guide is for you.
TL;DR: What is Python’s input()
function?
Python’s
input()
function is a built-in function that allows a program to interact with the user by receiving user input. It reads a line from the input (usually user input), converts it into a string, and returns that string. For example:
name = input("Enter your name: ")
print("Hello, " + name)
In this example, the program displays the message ‘Enter your name: ‘, waits for the user to type something, and then prints out a greeting that includes whatever the user typed. For more advanced methods, background, tips and tricks, etc., read on!
Table of Contents
Python Input Basic Usage
Python’s input()
function is a built-in function that opens the door to user interaction in your program. It’s a fundamental concept in programming, adding dynamism and interactivity to your code. But what exactly is the input()
function, and how does it operate in Python?
Imagine Python’s input()
function as a conversation starter. It initiates a dialogue with the user, awaiting their response. In technical terms, the input()
function in Python reads a line from input (usually user input), converts it into a string, and returns that string.
The syntax for the input()
function is pretty straightforward:
input([prompt])
Here, the prompt
is a string that is displayed on the standard output (usually your console) without a trailing newline. It serves as a cue to the user, indicating that the program is ready for their input.
If the
prompt
argument is left out, the function still waits for input, but without any preceding message.
Let’s unravel this with a simple example of using the input()
function:
name = input("Enter your name: ")
print("Hello, " + name)
In this example, the program will display the message ‘Enter your name: ‘, patiently wait for the user to type something, and then print out a greeting that includes whatever the user typed.
An important thing to remember about the
input()
function is that it always returns the user input as a string. If you want to work with a different data type, you’ll need to convert the string into that data type.
Python Input: Advanced Use Cases
Having grasped the basics of Python’s input()
function, we can now venture into more advanced territories.
In this section, we’ll discuss the input()
function’s interaction with different data types, illustrate its use in complex scenarios, delve into error handling, and even shed light on some potential security risks.
Juggling Different Data Types
As we’ve already noted, the input()
function treats all user input as a string. But what if you need the user to input a number, a list, or some other data type? In such scenarios, you’ll need to perform a conversion from the string returned by the input()
function into the desired data type. Here’s an example of how you can do this:
# Input a number
num = int(input("Enter a number: "))
print("You entered: ", num)
In this example, we use the int()
function to convert the user’s input into an integer.
If you wished to convert the input into a float or a list, you could use the float()
or eval()
functions, respectively. Can you think of a case where you would need this kind of conversion in your project?
Example of converting user input into a float and a list:
# Input a float
num = float(input("Enter a float number: "))
print("You entered: ", num)
# Input a list
lst = eval(input("Enter a list (for example [1,2,3]): ")) # User must input list format, we are using eval to evaluate the list
print("You entered: ", lst)
So let’s say the user enters 2.5
for float and [4,5,6]
for the list. The output will be:
Enter a float number: 2.5
You entered: 2.5
Enter a list (for example [1,2,3]): [4,5,6]
You entered: [4,5,6]
Please note that using
eval
function can be risky as it can evaluate any python code. It’s fine for simple examples or personal use but in production code or anywhere with untrusted input, it should be avoided or used with extreme care. It’s always better to validate the user input.
Input in a Loop
The input()
function is a versatile tool that can be employed in a variety of complex scenarios. For instance, you might use it in a loop to continuously ask for user input until the user decides to quit. Here’s an example:
while True:
response = input("Enter 'q' to quit: ")
if response == 'q':
break
In this example, the program will keep asking the user to enter ‘q’ to quit until the user does so. This can be particularly useful in programs that require continuous user interaction until a specific condition is met.
Graceful Error Handling
When working with user input in Python, it’s important to handle errors gracefully. If you’re asking the user to input a number and they input a string, your program will crash if it tries to convert that string into a number.
To prevent this, you can use a try-except block to catch the error and handle it appropriately:
try:
num = int(input("Enter a number: "))
except ValueError:
print("That's not a number!")
In this example, if the user inputs something that can’t be converted into a number, the program will catch the ValueError
and print a helpful message instead of crashing. This is a great way to ensure a smooth user experience even when the user makes a mistake.
Security Risks and Mitigations
While the input()
function is a powerful tool, it can pose a security risk if not handled carefully. If you’re using the eval()
function to convert user input into a list or some other data type, the user could potentially enter malicious code that gets executed by your program.
Sanitize String
To mitigate this risk, it’s best to avoid using eval()
with user input whenever possible. If you absolutely need to use eval()
, make sure to sanitize the user input to remove any potentially harmful code.
Example of sanitizing user input:
import re
# Input a string
s = input("Enter a string: ")
# Sanitize the string
s = re.sub('[^\w\s-]', '', s)
print("Sanitized string: ", s)
Don’t Forget Input Validation
Finally, let’s reinforce the importance of input validation. When you’re working with user input, it’s crucial to validate the input to ensure it’s in the correct format and within the expected range. This not only helps prevent errors and security risks, but also ensures that your program behaves as expected.
Example of validating user input:
while True:
response = input("Enter a number: ")
if response.isdigit():
print("You entered: ", int(response))
break
else:
print("That's not a number! Please try again.")
Now that we’ve explored the advanced usage of Python’s input()
function, let’s move on to troubleshooting common issues.
Troubleshooting Python Input Issues
Working with Python’s input()
function can sometimes feel like navigating a maze. Errors pop up, limitations are encountered, and debugging can feel like a dead end. But fear not! We’ve got a map to guide you through.
Resolving ValueError
When using the input()
function, you might stumble upon some common errors. One such error is a ValueError
. This sneaky error appears when you attempt to convert the user’s input into a certain data type, but the input is not in the correct format.
For example, trying to convert an input into an integer when the user enters a string, leads to a ValueError
. To outsmart this error, you can use a try-except block to catch it and handle it gracefully.
Example:
try:
num = int(input("Enter a number: "))
print("You entered: ", num)
except ValueError:
print("That's not a number!")
Handling TypeError
Another common error is a TypeError
, which occurs when you try to perform an operation on the user’s input that is not supported for the data type of the input.
For instance, if you attempt to add a number to the user’s input without first converting the input into a number, you’ll encounter a TypeError
.
The solution? Make sure to convert the user’s input into the correct data type before performing operations on it.
Example:
try:
s = input("Enter a string: ")
print("You entered: ", s + 10)
except TypeError:
print("Can't add a number to a string!")
Debugging With Print()
When debugging issues with the input()
function, print statements are your best friends. They can help you check the value and data type of the user’s input.
Example of using print statements for debugging:
s = input("Enter a string: ")
print("Debug: s = ", s)
num = input("Enter a number: ")
print("Debug: num = ", num)
Input() Limitations and Alternatives
While the input()
function is a powerful tool for getting user input, it has its limitations.
For instance, it always returns the input as a string, which can be inconvenient if you’re working with other data types. And it doesn’t provide any built-in functionality for validating the user’s input.
If you need more advanced features, consider alternatives like the argparse
module for command-line input or a library like curses
for more complex user interfaces.
Argparse Library
The argparse
library is a module in Python’s standard library that makes it easy to write user-friendly command-line interfaces. The argparse module can handle positional and optional arguments, provide usage and help messages, and even check for the correct types and ranges of the arguments.
# argparse module
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("--name", help="Enter your name")
args = parser.parse_args()
print("Hello, " + args.name)
An example of how to use the argparse
library is provided above. In this example, you define an argument "--name"
that the user can specify when they run your script from the command line. The argparse library will then capture this argument as a string, which you can then use in your script.
This makes the argparse
library a useful tool when you’re writing scripts that need to accept various options or arguments from the user, when you need control over the data type of the input, or when you want to display help messages to the user.
Curses Library
The curses
library is a tool to create text user interfaces in a terminal. This library provides functions for creating windows, handling user input, and controlling the cursor.
# curses library
import curses
stdscr = curses.initscr()
stdscr.addstr("Enter your name: ")
stdscr.refresh()
name = stdscr.getstr().decode()
stdscr.addstr("
Hello, " + name)
stdscr.refresh()
An example of how to use the curses
library is provided above. In this example, you initialize a standard screen, display a message prompting the user for input, and then capture and display the user’s input.
Please remember close the curses session with
curses.endwin()
when you’re finished with it, to ensure the terminal remains in a usable state.
With these features, the curses
library provides much greater control over the user interface than the input()
function. If you’re creating more complex user interfaces that need to capture various types of user input, or require more control over the display in the terminal, the curses
library could be a great tool to consider.
User Input in the Programming World
User input isn’t just a Python thing—it’s a cornerstone of programming, regardless of the language. Let’s zoom out and view user input from a broader programming perspective.
Be it a simple command-line tool prompting for parameters, a web form gathering user information, or a sophisticated graphical user interface (GUI) responding to clicks and keystrokes, user input is integral to the functioning of programs.
Programming is essentially about problem-solving and task automation. Many of these tasks are interactive, requiring user inputs.
Python’s Input Function vs. Other Languages
Different programming languages have different ways of handling user input. Python keeps it simple with the input()
function. It reads a line from input, converts it into a string, and returns that string. Languages like Java or C++, on the other hand, have their own methods of getting user input, which can be more complex and require more coding.
For instance, in Java, you’d need to use a Scanner
object to get user input, like this:
Scanner scanner = new Scanner(System.in);
String name = scanner.nextLine();
In C++, you’d use the cin
object, like this:
std::string name;
std::cin >> name;
Comparatively, Python’s
input()
function is much simpler and more straightforward, making Python an excellent choice for beginners and projects that require swift and easy user interaction.
Summary of Input methods:
Language | Method of Getting User Input |
---|---|
Python | name = input("Enter your name: ") |
Java | Scanner scanner = new Scanner(System.in); String name = scanner.nextLine(); |
C++ | std::string name; std::cin >> name; |
Other Python Functions for User Interaction
Python has more to offer for user interaction beyond the input()
function. Functions like print()
for outputting data to the user, open()
for file operations, and socket()
for network communication, among others, enable your program to interact with the user or the external world in diverse ways, adding to your program’s versatility.
For instance, the print()
function can display messages to the user, exhibit computation results, or assist in debugging your code. The open()
function facilitates file operations, enabling your program to read data from files or write data into them. And the socket()
function empowers your program to communicate over the network, sending and receiving data from other computers.
Further Resources for Python Functions
To help you delve deeper into the world of Python functions, the following resources have been specially curated for you:
- Python Built-In Functions Fundamentals Unveiled – Explore built-in functions for file handling and I/O operations.
Dynamic Attribute Access with getattr() in Python – Dive into attribute access, default values, and dynamic property retrieval.
Type Checking with Python isinstance() Function – Discover Python’s “isinstance” function for type checking and validation.
Python Programming Video Guide – Explore Python programming with this instructive video guide.
A Guide to User Input in Python – Explains Python user input interaction with this article by Towards Data Science.
Codecademy’s Python Input Function Documentation provides a user-friendly explanation of Python’s built-in input function.
By taking time to explore these materials, you will enhance your understanding and mastery of Python functions.
Final Words:
As we wrap up our comprehensive guide, we’ve navigated the vast landscape of user input in Python. We’ve dissected the input()
function, unraveled its basic usage, ventured into more advanced territories, and even addressed error handling and security concerns.
However, the input()
function is just the tip of the iceberg. User input is a cornerstone in programming, influencing how programs operate and interact with the user. We’ve compared how different programming languages handle user input, stressed the significance of input validation in secure programming, and discussed some alternative ways to capture user interactions.
So, the next time you’re working on a Python project, remember the power of the input()
function. Use it to make your program more interactive, validate the user input to make your program more secure, and handle errors gracefully to make your program more robust. Here’s to your journey in Python programming. Happy coding!