Python ‘Nonlocal’ | Keyword Usage Guide
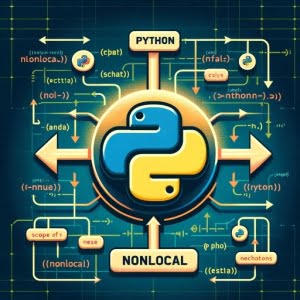
Are you finding it challenging to understand the ‘nonlocal’ keyword in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling nonlocal variables in Python, but we’re here to help.
Think of Python’s nonlocal keyword as a backstage pass – allowing a nested function to access a variable from its enclosing scope, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of mastering the nonlocal keyword in Python, from its basic usage, advanced scenarios, to alternative approaches. We’ll cover everything from the basics of nonlocal variables to more advanced techniques, as well as common issues and their solutions.
Let’s get started!
TL;DR: What is the Nonlocal Keyword in Python?
The ‘nonlocal’ keyword in Python is a statement that allows a nested function to access and modify a variable from its enclosing scope. It’s like a bridge that connects an inner function with an outer function’s variable.
Here’s a simple example:
def outer():
x = 'local'
def inner():
nonlocal x
x = 'nonlocal'
inner()
print(x)
outer()
# Output:
# 'nonlocal'
In this example, we have an outer function and an inner function. The outer function has a local variable x
with the value ‘local’. Inside the inner function, we declare x
as nonlocal, which means it refers to the x
in the outer function. We then change the value of x
to ‘nonlocal’. When we call the inner function inside the outer function, it changes the value of x
in the outer function. So, when we print x
, it outputs ‘nonlocal’.
This is a basic way to use the nonlocal keyword in Python, but there’s much more to learn about handling variable scope in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Python Nonlocal: A Beginner’s Guide
- Nonlocal in Nested Functions: An Intermediate Guide
- Exploring Alternatives to Python Nonlocal
- Troubleshooting Python Nonlocal: Common Issues and Solutions
- Understanding Variable Scope in Python
- Expanding the Scope: Python Nonlocal in Larger Scripts
- Wrapping Up: Mastering the Nonlocal Keyword in Python
Understanding Python Nonlocal: A Beginner’s Guide
The ‘nonlocal’ keyword in Python is primarily used in nested functions. It allows an inner function to access a variable from its immediate outer function. Let’s delve into a simple example to understand how it works.
Consider a nested function where we have an outer function and an inner function. The outer function has a local variable x
, and we want to change the value of x
inside the inner function.
def outer():
x = 'local'
def inner():
nonlocal x
x = 'nonlocal'
inner()
print(x)
outer()
# Output:
# 'nonlocal'
In this example, x
is a local variable in the outer function, initially set to ‘local’. Inside the inner function, we declare x
as nonlocal, which means it refers to the x
in the outer function. We then change the value of x
to ‘nonlocal’. When we call the inner function inside the outer function, it changes the value of x
in the outer function. So when we print x
, it outputs ‘nonlocal’.
This is a basic demonstration of how the ‘nonlocal’ keyword works in Python. It’s a powerful tool that allows us to manipulate the value of variables in the outer function from within an inner function. However, it’s important to use it judiciously, as it can lead to code that is hard to understand and debug if used excessively or inappropriately.
Nonlocal in Nested Functions: An Intermediate Guide
The Python nonlocal keyword becomes even more interesting when we deal with multiple levels of nested functions. It allows an inner function to access a variable from its immediate outer function, not any function outside of that. Let’s explore this with a more complex example.
Consider a scenario with three nested functions: outer, middle, and inner. The outer function has a local variable x
, and we want to change the value of x
from the inner function.
def outer():
x = 'local'
def middle():
x = 'middle'
def inner():
nonlocal x
x = 'inner'
inner()
print('Middle:', x)
middle()
print('Outer:', x)
outer()
# Output:
# Middle: inner
# Outer: local
In this example, x
is a local variable in the outer function and is initially set to ‘local’. Inside the middle function, we have another local variable x
, set to ‘middle’. The inner function declares x
as nonlocal and changes its value to ‘inner’. When we call the inner function inside the middle function, it changes the value of x
in the middle function. So when we print x
in the middle function, it outputs ‘inner’. However, when we print x
in the outer function, it still outputs ‘local’.
This is because the nonlocal keyword in the inner function refers to the x
in the middle function, not the outer function. It shows that the nonlocal keyword allows access to the nearest enclosing scope that is not global, demonstrating the power and flexibility of Python’s scoping rules.
Exploring Alternatives to Python Nonlocal
While the ‘nonlocal’ keyword provides a way to access and modify variables in the nearest enclosing scope that is not global, there are other ways to manage variable scope in Python. Let’s look at two alternative approaches: using global variables and mutable data types.
Using Global Variables
Global variables are accessible throughout the entire program, making them an alternative to nonlocal variables. However, using global variables should be done with caution as they can lead to code that is difficult to understand and debug.
global_var = 'global'
def outer():
def inner():
global global_var
global_var = 'changed'
inner()
print(global_var)
outer()
# Output:
# 'changed'
In this example, we have a global variable global_var
. Inside the inner function, we declare global_var
as global and change its value to ‘changed’. When we call the inner function, it changes the value of the global variable. So when we print global_var
, it outputs ‘changed’.
Using Mutable Data Types
Another approach to access variables from enclosing scopes is to use mutable data types like lists or dictionaries. Since these data types are mutable, changes made inside a function are reflected globally.
def outer():
x = ['local']
def inner():
x[0] = 'nonlocal'
inner()
print(x[0])
outer()
# Output:
# 'nonlocal'
In this example, x
is a list in the outer function, initially set to [‘local’]. Inside the inner function, we change the first element of the list to ‘nonlocal’. When we call the inner function, it changes the value of x
in the outer function. So when we print x[0]
, it outputs ‘nonlocal’.
Both of these methods have their benefits and drawbacks. Global variables can be accessed from anywhere in the program, but they can lead to code that is hard to understand and debug. On the other hand, mutable data types allow changes to be reflected globally, but this might not be the desired behavior in all cases. It’s important to understand these trade-offs and choose the best approach for your specific use case.
Troubleshooting Python Nonlocal: Common Issues and Solutions
Like any other programming concept, using the ‘nonlocal’ keyword in Python can sometimes lead to errors or obstacles. In this section, we’ll discuss some common issues related to the ‘nonlocal’ keyword and their solutions. We’ll also provide some tips for best practices and optimization.
Nonlocal Variable Not Defined
One of the most common errors when using the ‘nonlocal’ keyword is trying to declare a nonlocal variable that does not exist in the enclosing scope. For example:
def outer():
def inner():
nonlocal x
x = 'nonlocal'
inner()
outer()
# Output:
# SyntaxError: no binding for nonlocal 'x' found
In this example, we’re trying to declare x
as nonlocal inside the inner function. However, x
doesn’t exist in the enclosing scope, leading to a SyntaxError.
To fix this error, ensure that the variable you’re declaring as nonlocal exists in the enclosing scope.
Using Nonlocal in the Global Scope
Another common error is trying to use the ‘nonlocal’ keyword in the global scope. The ‘nonlocal’ keyword is meant to be used in nested functions, and using it in the global scope will result in a SyntaxError.
nonlocal x
x = 'nonlocal'
# Output:
# SyntaxError: nonlocal declaration not allowed at module level
In this example, we’re trying to declare x
as nonlocal in the global scope, leading to a SyntaxError. To fix this error, only use the ‘nonlocal’ keyword inside nested functions.
Best Practices and Optimization
When using the ‘nonlocal’ keyword in Python, it’s important to follow some best practices to write clean, efficient, and bug-free code. Here are some tips:
- Only use the ‘nonlocal’ keyword when necessary. If you can achieve the same result without it, it’s usually better to do so to keep your code simple and easy to understand.
Avoid using the ‘nonlocal’ keyword with the same variable name in multiple nested functions. This can lead to confusion and make your code harder to debug.
Always ensure that the variable you’re declaring as nonlocal exists in the enclosing scope to avoid SyntaxErrors.
Understanding Variable Scope in Python
To fully grasp the concept of the ‘nonlocal’ keyword in Python, it’s crucial to understand the idea of variable scope. The scope of a variable refers to the region within the code where a variable is recognized. There are three types of variable scope in Python: local, nonlocal, and global.
Local Scope
A variable declared inside a function has a local scope. It’s only accessible within that function, not outside it.
def greet():
message = 'Hello, world!'
print(message)
greet()
# Output:
# 'Hello, world!'
print(message)
# Output:
# NameError: name 'message' is not defined
In this example, message
is a local variable inside the greet
function. It’s accessible within the greet
function, but trying to access it outside the function results in a NameError.
Nonlocal Scope
As we’ve discussed, the ‘nonlocal’ keyword in Python allows a nested function to access a variable from its immediate outer function.
Global Scope
A variable declared outside all functions has a global scope. It’s accessible throughout the entire program, both inside and outside of functions.
global_message = 'Hello, universe!'
def greet_universe():
print(global_message)
greet_universe()
# Output:
# 'Hello, universe!'
print(global_message)
# Output:
# 'Hello, universe!'
In this example, global_message
is a global variable. It’s accessible both inside the greet_universe
function and outside the function.
Understanding these different types of variable scope in Python is fundamental to effectively using the ‘nonlocal’ keyword and managing variable scope in your Python programs.
Expanding the Scope: Python Nonlocal in Larger Scripts
While we’ve covered the basic and advanced uses of the ‘nonlocal’ keyword in Python, it’s important to understand how it applies in larger scripts or projects. The ‘nonlocal’ keyword often comes into play in more complex code structures, especially when dealing with nested functions and closures.
Closures and Python Nonlocal
Closures in Python are a direct application of the ‘nonlocal’ keyword. A closure is a function object that has access to variables from its enclosing lexical scope, even when the function is called outside that scope. The ‘nonlocal’ keyword is used to modify these variables.
def outer_function(text):
def inner_function():
nonlocal text
text = 'Hello, ' + text
return text
return inner_function
greet = outer_function('world!')
print(greet())
# Output:
# 'Hello, world!'
In this example, outer_function
returns inner_function
, creating a closure. Inside inner_function
, we declare text
as nonlocal, allowing us to modify it. When we call greet()
, it outputs ‘Hello, world!’, demonstrating how the ‘nonlocal’ keyword is used in closures.
Further Resources for Python Nonlocal Mastery
To further your understanding of the ‘nonlocal’ keyword and related topics in Python, here are some additional resources:
- Python Keywords Use Cases Explored – Empower your Python coding journey with comprehensive keyword understanding.
Null Handling with Python’s None Keyword – Explore Python’s approach to null values and how “None” is used.
Python Comment: Enhancing Code Readability – Explore Python’s comment syntax for adding notes to your code.
Python Keywords and Identifiers – Understand the roles of keywords and identifiers in Python programming.
Python’s Official Documentation on the ‘nonlocal’ Keyword dives into toopics on the ‘nonlocal’ keyword.
Python Scopes and Namespaces – Enhance your understanding of Python’s scope and namespaces with this in-depth guide.
These resources provide in-depth information and practical examples that can help you master the use of the ‘nonlocal’ keyword in Python and understand its role in larger scripts and projects.
Wrapping Up: Mastering the Nonlocal Keyword in Python
In this comprehensive guide, we’ve explored the ins and outs of the ‘nonlocal’ keyword in Python, a powerful tool for managing variable scope in nested functions.
We began with the basics, learning how to use the ‘nonlocal’ keyword in a simple nested function scenario. We then delved into more advanced usage, exploring how the ‘nonlocal’ keyword works in multiple levels of nested functions.
We also discussed common issues related to the ‘nonlocal’ keyword and their solutions, providing you with a toolbox of strategies to overcome these challenges.
We also explored alternative approaches to handle variable scope in Python, such as using global variables and mutable data types. Each of these methods has its benefits and drawbacks, and the best choice depends on your specific use case.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Nonlocal | Access to outer function’s variable | Can lead to confusion if overused |
Global | Accessible throughout the program | Can lead to hard-to-debug code |
Mutable Data Types | Changes reflected globally | Might not be the desired behavior in all cases |
Whether you’re just starting out with Python or looking to deepen your understanding of variable scope, we hope this guide has given you a deeper understanding of the ‘nonlocal’ keyword and its role in Python programming.
With the knowledge and strategies you’ve gained from this guide, you’re now well-equipped to use the ‘nonlocal’ keyword effectively in your Python projects. Happy coding!