Python ‘String’ Data Type: Usage and Manipulation Guide
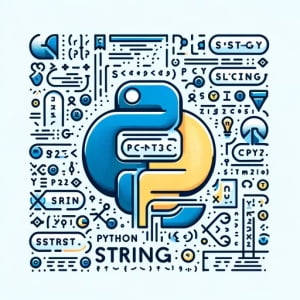
Ever felt like you’re wrestling with Python strings? You’re not alone. Many developers find Python string manipulation a bit daunting. But think of Python strings as a string of pearls – each pearl, a character, forming a beautiful sequence.
In this guide, we’ll walk you through the process of mastering Python strings, from the basics to more advanced techniques. We’ll cover everything from creating strings, accessing and manipulating string elements, to troubleshooting common issues and exploring alternative approaches.
Let’s dive in and start mastering Python strings!
TL;DR: What is a Python String and How Do I Use It?
A Python
string
is a sequence of characters. You can create a string with the syntax,string = 'New String'
. The sequence must be wrapped with''
or""
.
Here’s a simple example:
greeting = 'Hello, World!'
print(greeting)
# Output:
# 'Hello, World!'
In this example, we’ve created a string greeting
that contains the text ‘Hello, World!’. We then print the string to the console, resulting in the output ‘Hello, World!’.
This is just the tip of the iceberg when it comes to Python strings. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Python Strings: The Basics
Python strings are incredibly versatile and easy to use. Let’s start with the basics: creating and accessing elements in Python strings.
Creating a Python String
You can create a Python string by enclosing a sequence of characters in single quotes (”) or double quotes (“”). Here’s an example:
my_string = 'Hello, Python!'
print(my_string)
# Output:
# 'Hello, Python!'
In this example, we’ve created a string my_string
that contains the text ‘Hello, Python!’. We then print the string to the console, resulting in the output ‘Hello, Python!’.
Accessing Elements in Python Strings
Python strings are sequences, which means you can access individual elements in the string using indexing. Python uses zero-based indexing, so the first character in the string is at index 0, the second character is at index 1, and so on. Here’s how you can access elements in a Python string:
my_string = 'Hello, Python!'
print(my_string[0])
print(my_string[7])
# Output:
# 'H'
# 'P'
In the above example, we print the first character (at index 0) and the eighth character (at index 7) of the string my_string
. The output is ‘H’ and ‘P’, respectively.
Python strings are a powerful tool in any developer’s toolkit. However, they do come with some quirks and potential pitfalls, such as immutability (you can’t change individual characters in a string) and the fact that they can sometimes behave in unexpected ways when used with certain functions or in certain contexts. But with a solid understanding of the basics, you’ll be well-equipped to navigate these challenges and make the most of Python strings.
Advanced Uses of Python Strings
As you become more comfortable with Python strings, you’ll discover a plethora of techniques to manipulate and work with them. Let’s explore some of these advanced techniques, such as slicing, concatenation, and using string methods like split()
, replace()
, and more.
Python String Slicing
Slicing allows you to extract a portion of a Python string. It’s a powerful technique that can save you a lot of time and effort. Here’s how you can slice a Python string:
my_string = 'Hello, Python!'
print(my_string[0:5])
# Output:
# 'Hello'
In the above example, we print the first five characters of the string my_string
using slicing. The output is ‘Hello’.
Python String Concatenation
Concatenation allows you to join two or more Python strings together. Here’s how you can concatenate Python strings:
string1 = 'Hello, '
string2 = 'Python!'
print(string1 + string2)
# Output:
# 'Hello, Python!'
In the above example, we join string1
and string2
using the +
operator and print the result. The output is ‘Hello, Python!’.
Python String Methods
Python provides a host of built-in methods to work with strings. Let’s explore split()
and replace()
methods.
The split() Method
The split()
method divides a string into a list where each word is an item. Here’s how you can use split()
:
my_string = 'Hello, Python!'
print(my_string.split())
# Output:
# ['Hello,', 'Python!']
In the above example, we split my_string
into a list of words using the split()
method. The output is a list with two items: ‘Hello,’ and ‘Python!’.
The replace() Method
The replace()
method replaces a specified phrase with another specified phrase. Here’s how you can use replace()
:
my_string = 'Hello, Python!'
print(my_string.replace('Python', 'World'))
# Output:
# 'Hello, World!'
In the above example, we replace ‘Python’ with ‘World’ in my_string
using the replace()
method. The output is ‘Hello, World!’.
Mastering these advanced techniques will allow you to manipulate Python strings effectively, opening up a world of possibilities for your Python scripting.
Alternate Tools for String Manipulation
Python provides a wealth of built-in functionality for string manipulation, but there are also several alternative approaches that can offer more flexibility or efficiency in certain scenarios. Let’s take a look at two of these: regular expressions and third-party libraries.
Regular Expressions in Python
Regular expressions (regex) provide a powerful way to match and manipulate strings based on patterns. Python’s re
module supports regex operations.
Here’s an example where we use a regular expression to find all the words in a string:
import re
my_string = 'Hello, Python! How are you doing?'
words = re.findall(r'\b\w+\b', my_string)
print(words)
# Output:
# ['Hello', 'Python', 'How', 'are', 'you', 'doing']
In the above example, we use the findall()
function from the re
module to find all words in my_string
. The output is a list of words in the string.
Regular expressions can be complex but are extremely powerful. They can handle tasks that are difficult or impossible with standard string methods.
String Manipulation with Third-Party Libraries
There are several third-party libraries available that provide additional functionality for string manipulation. One such library is fuzzywuzzy
, which allows for fuzzy string matching.
Here’s an example of how you can use fuzzywuzzy
to compare two strings:
from fuzzywuzzy import fuzz
string1 = 'Python'
string2 = 'Pyhton'
similarity = fuzz.ratio(string1, string2)
print(similarity)
# Output:
# 83
In the above example, we use the fuzzywuzzy
library to calculate the similarity between string1
and string2
. The output is a score out of 100, with 100 being a perfect match. In this case, the strings ‘Python’ and ‘Pyhton’ have a similarity score of 83.
While third-party libraries like fuzzywuzzy
can provide additional functionality, they also introduce dependencies into your project. It’s important to weigh the benefits against the potential costs of using third-party libraries.
Whether you choose to use regular expressions, third-party libraries, or stick with Python’s built-in string methods, the key is to understand the tools at your disposal and choose the right one for the task at hand.
Error Handling with String Data Types
As with any programming language, working with Python strings can sometimes lead to unexpected issues. Let’s discuss some common challenges and how to solve them.
Dealing with Unicode Errors
Python strings can sometimes throw Unicode errors, especially when working with non-ASCII characters. Here’s how you can handle such errors:
try:
my_string = 'Hello, Python!'
print(my_string.encode('ascii'))
except UnicodeEncodeError:
print('String contains non-ASCII characters.')
# Output:
# b'Hello, Python!'
In the above example, we try to encode a string to ASCII. If the string contains non-ASCII characters, a UnicodeEncodeError
is thrown, and we print a custom error message.
Understanding String Immutability
Python strings are immutable, which means you cannot change individual characters in a string. If you try to do so, you’ll get an error. Here’s an example:
my_string = 'Hello, Python!'
try:
my_string[0] = 'h'
except TypeError:
print('Python strings are immutable.')
# Output:
# Python strings are immutable.
In the above example, we try to change the first character of the string my_string
. Because Python strings are immutable, we get a TypeError
, and we print a custom error message.
Understanding these common issues and how to handle them can help you avoid pitfalls and work more effectively with Python strings.
The Fundamentals of Strings in Python
To truly master Python strings, it’s important to understand the underlying concepts and the string data type in Python.
Understanding Python’s String Data Type
In Python, a string is a sequence of characters. It is a derived data type, meaning it’s composed of more basic data types (characters). Python treats single quotes and double quotes as the same, which means you can use either to create a string.
Here’s an example:
string1 = 'Hello, Python!'
string2 = "Hello, Python!"
print(string1 == string2)
# Output:
# True
In the above example, we create two identical strings using single and double quotes and compare them. The output is True
, which confirms that Python treats single and double quotes as the same.
Python String Methods and Formatting
Python provides a wealth of built-in methods for string manipulation. These methods allow you to transform, search, split, replace, and perform many other operations on strings.
Here’s an example of using the upper()
method to convert a string to uppercase:
my_string = 'Hello, Python!'
print(my_string.upper())
# Output:
# 'HELLO, PYTHON!'
In the above example, we convert my_string
to uppercase using the upper()
method. The output is ‘HELLO, PYTHON!’.
Python also supports several ways to format strings, including f-strings and the format()
method. These techniques allow you to insert variables into strings, align text, set precision, and more.
Here’s an example of using an f-string to insert a variable into a string:
name = 'Python'
greeting = f'Hello, {name}!'
print(greeting)
# Output:
# 'Hello, Python!'
In the above example, we use an f-string to insert the variable name
into the string greeting
. The output is ‘Hello, Python!’.
Understanding these fundamental concepts can help you work more effectively with Python strings and leverage their full power in your Python scripts.
Programming Use Cases of Strings
Python strings are not only a fundamental data type but also a powerful tool that can be used in various scenarios beyond the basic string manipulations.
Python Strings in File Handling
Python strings play a crucial role when it comes to file handling. Whether you’re reading from a file or writing to it, you’re essentially dealing with strings.
Here’s an example of writing a string to a file:
my_string = 'Hello, Python!'
with open('hello.txt', 'w') as f:
f.write(my_string)
# Check the file 'hello.txt' for the output
In the above example, we write the string my_string
to a file named ‘hello.txt’. If you open ‘hello.txt’, you’ll see ‘Hello, Python!’.
Python Strings in Web Scraping
Web scraping is another area where Python strings show their power. When you scrape a web page, you essentially fetch a large string of HTML that you then parse and extract information from.
Here’s an example using the requests
library to fetch a web page and print its HTML:
import requests
response = requests.get('https://www.python.org')
print(response.text)
# Output:
# The HTML of the Python.org homepage
In the above example, we fetch the Python.org homepage and print its HTML. The output is a large string of HTML.
Further Resources for Python String Mastery
For those who want to delve deeper into Python strings, here are some resources that provide more in-depth information:
- IOFlood’s Complete Python Data Types article covers everything from the basic data types like integers, floats, and strings to more complex ones like lists, tuples, sets, and dictionaries.
Click here for our Python Int to String conversion guide, complete with examples and explanations on using different methods.
Python String Formatting Tutorial by IOFlood: Dive into the topic of string formatting in Python.
Python String Documentation: The official Python documentation on strings. A comprehensive resource covering all the built-in string methods.
Python String Formatting Guide: A detailed guide on Real Python about string formatting techniques in Python.
Python Regular Expressions Tutorial: A tutorial on DataCamp about using regular expressions in Python. A great resource for those interested in more advanced string manipulation techniques.
Understanding the versatility of Python strings and their applications in various scenarios like file handling and web scraping will open up a new realm of possibilities for your Python scripting.
Wrap Up: Python String Manipulation
In this comprehensive guide, we’ve explored the fascinating world of Python strings, a fundamental and powerful data type in Python.
We started with the basics of Python strings, understanding how to create and access elements in a string. We then moved on to more advanced techniques, including string slicing, concatenation, and the use of string methods like split()
, replace()
, and more. We also delved into alternative approaches for string manipulation, such as using regular expressions and third-party libraries like fuzzywuzzy
.
Along the journey, we tackled common challenges you might face when working with Python strings, such as Unicode errors and string immutability, providing you with solutions for each issue.
We also explored the relevance of Python strings in various scenarios like file handling and web scraping, opening up a new realm of possibilities for your Python scripting.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Python Built-in Methods | Simple, no additional dependencies | May not handle complex scenarios |
Regular Expressions | Powerful, handles complex scenarios | Higher learning curve |
Third-Party Libraries | Additional functionalities, handles complex scenarios | Introduces extra dependencies |
Whether you’re just starting out with Python strings or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of Python strings and their capabilities.
With their versatility and wide range of applications, Python strings are undoubtedly a powerful tool in any developer’s toolkit. Now, you’re well equipped to master Python strings. Happy coding!