Checking in Bash If File Exists | Simple Scripting Methods
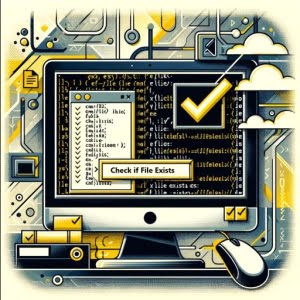
When ensuring consistent behavior in our automation scripts at IOFLOOD, we required simple methods to implement bash if file exists checks. By checking whether a bash file exists or not, we can conditionally execute commands with confidence. To help our bare metal servers customers enhance their scripting capabilities, we have decided to share our processes and methods in today’s article.
In this guide, we’ll walk you through the methods to check in bash if file exists, from the basic use to more advanced techniques. We’ll cover everything from using the ‘-f’ test operator in an ‘if’ statement, to handling more complex scenarios, and even exploring alternative approaches.
Let’s dive in and start mastering ‘bash check if file exists’!
TL;DR: How Do I Bash Check If File Exists?
In bash, you can check if a file exists by using the
'-f'
test operator in an'if'
statement, with the syntax,if [ -f /path/to/file ]
. This operator returns true if the file exists and is a regular file.
Here’s a simple example:
if [ -f /path/to/file ]; then
echo 'File exists.'
else
echo 'File does not exist.'
fi
# Output:
# 'File exists.' if the file is present
# 'File does not exist.' if the file is not present
In this example, we use the ‘-f’ test operator inside an ‘if’ statement to check if a file exists at the specified path. If the file is present, the script echoes ‘File exists.’ If not, it echoes ‘File does not exist.’
This is a basic way to check if a file exists in bash, but there’s much more to learn about file handling in bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basics: Bash If File Exists Checks
The ‘-f’ test operator is a fundamental tool in bash scripting for checking if a file exists. It’s used in an ‘if’ statement and returns true if the file exists and is a regular file. Here’s how you can use it:
filename='/path/to/file'
if [ -f $filename ]; then
echo 'The file exists.'
else
echo 'The file does not exist.'
fi
# Output:
# 'The file exists.' if the file is present
# 'The file does not exist.' if the file is not present
In this example, we first define a variable filename
to store the path of the file we want to check. We then use the ‘-f’ test operator inside an ‘if’ statement. If the file exists at the specified path, the script echoes ‘The file exists.’ If not, it echoes ‘The file does not exist.’
Pros and Cons of ‘-f’ Test Operator
The ‘-f’ test operator is straightforward and easy to use, making it ideal for beginners. It’s a quick way to check if a file exists and is a regular file.
However, it’s worth noting that the ‘-f’ test operator only checks for regular files. It will return false for directories or special files (like device files). If you need to check for directories or special files, you’ll need to use other test operators, which we’ll discuss in the advanced use section.
Advanced Check if File Exists Bash
As you get more comfortable with bash scripting, you might find yourself needing to perform more complex file checks. Let’s explore a couple of scenarios where you might need to go beyond the basic ‘-f’ operator.
Checking for Multiple Files
Sometimes, you might need to verify the existence of several files. You can accomplish this by using a for-loop along with the ‘-f’ operator. Here’s an example:
filenames=('/path/to/file1' '/path/to/file2' '/path/to/file3')
for filename in ${filenames[@]}; do
if [ -f $filename ]; then
echo "$filename exists."
else
echo "$filename does not exist."
fi
done
# Output:
# '/path/to/file1 exists.' if file1 is present
# '/path/to/file2 does not exist.' if file2 is not present
# '/path/to/file3 exists.' if file3 is present
In this example, we define an array filenames
that contains the paths of the files we want to check. We then loop through each filename in the array. For each filename, we use the ‘-f’ operator to check if the file exists.
Checking for Specific Types of Files
The ‘-f’ operator checks if a file exists and is a regular file. But what if you need to check for a specific type of file? Bash provides several test operators for this purpose:
- ‘-d’ checks if a file is a directory.
- -h’ or ‘-L’ checks if a file is a symbolic link.
- ‘-p’ checks if a file is a named pipe (FIFO).
Here’s an example of checking if a file is a directory:
filename='/path/to/directory'
if [ -d $filename ]; then
echo "$filename is a directory."
else
echo "$filename is not a directory."
fi
# Output:
# '/path/to/directory is a directory.' if the directory is present
# '/path/to/directory is not a directory.' if the directory is not present
Pros and Cons of Advanced Use
Checking for multiple files or specific types of files can make your bash scripts more versatile. However, these methods can also be more complex and might be overkill for simple scripts. Always choose the right tool for the job.
Alternates: Shell Check if File Exists
As an expert bash scripter, you might be interested in exploring alternative methods to check if a file exists. Two such methods include using the ‘test’ command and the ‘-e’ test operator.
The ‘test’ Command
The ‘test’ command is a built-in bash command that allows you to perform various checks, including file existence checks. It’s a more explicit way of performing the same checks as the ‘[ ]’ syntax.
Here’s an example of using the ‘test’ command to check if a file exists:
filename='/path/to/file'
test -f $filename && echo 'File exists.' || echo 'File does not exist.'
# Output:
# 'File exists.' if the file is present
# 'File does not exist.' if the file is not present
In this example, we use the ‘test’ command with the ‘-f’ operator to check if a file exists at the specified path. The ‘&&’ operator executes the ‘echo ‘File exists.” command if the test command succeeds (i.e., the file exists). The ‘||’ operator executes the ‘echo ‘File does not exist.” command if the test command fails (i.e., the file does not exist).
The ‘-e’ Test Operator
The ‘-e’ test operator checks if a file exists, regardless of the type of file. This can be useful if you need to check for any type of file, including directories and special files.
Here’s an example of using the ‘-e’ operator to check if a file exists:
filename='/path/to/file'
if [ -e $filename ]; then
echo 'File exists.'
else
echo 'File does not exist.'
fi
# Output:
# 'File exists.' if the file is present
# 'File does not exist.' if the file is not present
In this example, we use the ‘-e’ operator inside an ‘if’ statement to check if a file exists at the specified path. If the file exists, the script echoes ‘File exists.’ If not, it echoes ‘File does not exist.’
Advantages and Disadvantages of Alternative Approaches
The ‘test’ command and the ‘-e’ operator offer more flexibility than the ‘-f’ operator. The ‘test’ command is more explicit, which can make your scripts easier to read and understand. The ‘-e’ operator checks for any type of file, which can be useful in certain scenarios.
However, these methods can also be more complex and might be overkill for simple scripts. As always, choose the right tool for the job.
Error Tips: Bash File Exists Checks
When using bash to check if a file exists, you may encounter a few common issues. Let’s discuss these potential pitfalls and how to navigate them.
Syntax Errors
Bash is sensitive to syntax, and a misplaced space or missing bracket can lead to errors. For example, the following code will produce a syntax error:
filename='/path/to/file'
if [-f $filename ]; then
echo 'File exists.'
else
echo 'File does not exist.'
fi
# Output:
# bash: [-f: command not found
In this example, there’s no space between the opening bracket and ‘-f’. Bash interprets '[-f'
as a command, which it can’t find, leading to a syntax error. To fix this, add a space after the opening bracket:
if [ -f $filename ]; then
echo 'File exists.'
else
echo 'File does not exist.'
fi
# Output:
# 'File exists.' if the file is present
# 'File does not exist.' if the file is not present
Permission Issues
Sometimes, you might have the correct syntax, but still can’t check if a file exists. This could be due to permission issues. If your script doesn’t have read permission for the file or execute permission for the directory containing the file, it can’t check if the file exists.
In such cases, you might need to change the file permissions using the ‘chmod’ command, or run the script with a user that has the necessary permissions.
Tips for Troubleshooting
When troubleshooting, the ‘set -x’ command can be your best friend. This command prints each command to the terminal before it’s executed, which can help you identify where things are going wrong.
Remember, practice makes perfect. The more you work with bash, the more comfortable you’ll become with its syntax and idiosyncrasies.
Scripting Bash File Exists Explained
Before we dive deeper into file checks, let’s take a moment to understand the structure of a bash script and the use of conditional statements in bash. This will help us better understand the concepts underlying the file existence check.
The Anatomy of a Bash Script
A bash script is a plain text file that contains a series of commands. These commands are a sequence of orders that a user can write manually but are often automated in scripts for reusability. Here’s a basic structure of a bash script:
#!/bin/bash
# This is a comment
echo 'Hello, world!'
# Output:
# 'Hello, world!'
In this example, #!/bin/bash
is called a shebang. It tells the system that this script should be executed with bash. Comments start with a ‘#’, and ‘echo’ is a command that outputs its argument.
Conditional Statements in Bash
Conditional statements allow a script to perform different actions depending on whether a certain condition is true or false. The ‘if’ statement is a basic form of conditional statement in bash. Here’s an example:
if [ 10 -gt 5 ]; then
echo '10 is greater than 5.'
fi
# Output:
# '10 is greater than 5.'
In this example, the ‘if’ statement checks if 10 is greater than 5. If the condition is true, it echoes ’10 is greater than 5.’
When checking if a file exists in bash, we use the ‘if’ statement together with a test operator, such as ‘-f’. This allows the script to perform different actions depending on whether a file exists or not.
How to Bash Script Check if File Exists
Checking if a file exists is a fundamental operation in bash scripting, but it’s just the tip of the iceberg. As you delve deeper into bash scripting, you’ll find that file existence checks play a crucial role in larger scripts and projects.
For instance, in a backup script, you might need to check if a backup file already exists before creating a new one. In a log processing script, you might need to check if a log file exists before trying to read from it. In these scenarios, knowing how to check if a file exists in bash is essential.
Beyond Bash If File Exists Checks
As you continue to expand your bash scripting skills, you might want to explore related concepts. For instance, you could learn more about file handling in bash, such as reading from and writing to files. You could also delve into other conditional statements in bash, such as ‘case’ statements and ‘while’ loops.
Understanding these concepts will give you a more in-depth understanding of bash scripting and enable you to write more complex and powerful scripts.
Resources for Bash Scripting Tutorials
To help you on your journey, here are some resources that offer more information and tutorials on bash scripting:
- GNU Bash Manual: This is the official manual for bash. It’s comprehensive and in-depth, making it a great resource for anyone serious about mastering bash scripting.
Bash Scripting Tutorial by Ryan’s Tutorials: This tutorial is beginner-friendly and covers a wide range of topics, from basic commands to file handling and conditional statements.
Advanced Bash-Scripting Guide: This guide is for advanced users. It covers complex topics like arrays, regular expressions, and process management in detail.
Recap: Bash Check If File Exists
In this comprehensive guide, we’ve explored how to check if a file exists in bash, covering the command usage from basic to advanced levels, and even delving into alternative approaches.
We began with the basics, demonstrating how to use the ‘-f’ test operator in an ‘if’ statement to check if a file exists. We then ventured into more advanced territory, discussing how to check for multiple files and specific types of files. We also explored alternative methods for file checks, such as using the ‘test’ command and the ‘-e’ test operator.
Along the way, we addressed common issues that you might encounter when checking if a file exists in bash, such as syntax errors and permission issues, providing solutions and workarounds for each problem.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘-f’ Test Operator | Simple, checks if file is a regular file | Only checks for regular files |
‘test’ Command | Explicit, versatile | Can be more complex |
‘-e’ Test Operator | Checks for any type of file | Can be overkill for simple scripts |
Whether you’re a beginner just starting out with bash scripting or an expert looking to brush up on your skills, we hope this guide has given you a deeper understanding of how to check if a file exists in bash.
The ability to check if a file exists is a fundamental operation in bash scripting, and it’s a skill that will serve you well in many scripting scenarios. Now, you’re well equipped to handle file checks in your bash scripts. Happy scripting!