Does Python Null Exist? A ‘None’ Python Keyword How-To
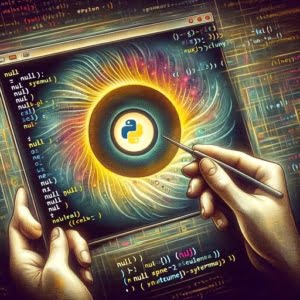
Navigating the world of programming languages, you may have found yourself puzzled by the concept of ‘null’ values. This puzzle becomes even more intriguing when you step into the realm of Python and encounter its unique ‘None’ concept. If you’ve ever questioned the role of ‘None’ in Python, you’re in good company.
Python stands apart from many other programming languages by not having a conventional null value. Instead, it uses ‘None’. This might seem a little baffling, especially for programmers transitioning from languages like JavaScript or C, where null is a standard feature.
In this blog post, we will demystify ‘None’ in Python. We aim to delve into what it is, its usage, and how it contrasts with the traditional null values in other programming languages. Our goal is to equip you with a thorough understanding of ‘None’ in Python. So, when you next come across ‘None’ in your Python code, you’ll have a clear idea of its purpose.
Does Python have a ‘null’? Let’s embark on this exploration to discover the answer!
TL;DR: What is ‘None’ in Python?
‘None’ in Python is a special data type that represents the absence of a value or a null value. It is an object of its own datatype, the NoneType. We can assign ‘None’ to any variable, but you can not create other NoneType objects. For more in-depth understanding, along with tips and tricks, continue reading the article.
# Example of 'None' in Python
var = None
print(type(var))
# Outputs: <class 'NoneType'>
Table of Contents
‘None’ as Python’s Null Equivalent
In numerous programming languages, such as Java or C++, ‘null’ is a value that symbolizes the lack of a value. However, Python deviates from this norm by not having a conventional ‘null’ value. Instead, it employs ‘None’. You can perceive ‘None’ as Python’s unique approach to defining nothingness or emptiness. It’s the value that gets assigned to a variable if it doesn’t hold any other value.
The Role of ‘None’ in Efficient Python Coding
‘None’ holds a pivotal position in Python programming. It contributes to maintaining the integrity of the code and enhancing your code’s efficiency. For instance, if a function doesn’t explicitly return a value, it defaults to returning ‘None’. This feature can be especially beneficial when debugging, as you can check if a function is returning ‘None’ to ascertain if it’s executing as expected.
Assigning ‘None’ to Variables
In Python, ‘None’ is a powerful tool that often signifies the absence of a value. It’s akin to a placeholder for something that doesn’t exist yet. For instance, if you have a variable that you want to declare without assigning a value at the moment, ‘None’ comes in handy. Here’s an illustration of how you can do it:
variable = None
In this scenario, the variable exists, but it doesn’t hold any value. You could visualize it as an empty box waiting to be filled.
Example of assigning ‘None’ to a variable:
variable = None
print(variable)
# Outputs: None
‘None’ as a Default Parameter
One of the common uses of ‘None’ is in function definitions as a default parameter. This is especially useful when you want to make a parameter optional. The following example illustrates this concept:
def greet(name=None):
if name is None:
print('Hello, world!')
else:
print(f'Hello, {name}!')
In this function, if no argument is passed for the name parameter, the function will print ‘Hello, world!’. However, if you do pass an argument, it will use that to greet you.
This is especially helpful because of problems that can occur when assigning a mutable data type as a default argument. None avoids these problems.
Using the Identity Operator to Check for ‘None’
When verifying if a variable is ‘None’, it’s crucial to use the identity operator ‘is’ rather than the equality operator '=='
. This is because ‘is’ checks if two variables point to the same object, whereas '=='
checks if the variables are equal. Here’s an example of how to use the ‘is’ operator to check for ‘None’:
if variable is None:
print('The variable is None')
In Python, ‘None’ is a singleton object, implying there’s only one instance of it. So when you’re checking for ‘None’, you’re essentially verifying if the variable points to this unique ‘None’ instance.
Dealing with Missing Values Using ‘None’
‘None’ can also be employed to handle missing values in data. For instance, if you’re working with a list of data where some values are missing, you can use ‘None’ to represent these missing values. This approach can enhance the robustness of your code as it can handle and recognize these missing values.
Example of using ‘None’ to handle missing values:
data = [1, 2, None, 3, None, 4]
for i in data:
if i is None:
print('Missing value')
else:
print(i)
# Outputs: 1, 2, 'Missing value', 3, 'Missing value', 4
‘None’ in Python is a versatile tool that can enhance the flexibility of your code. Whether you’re assigning ‘None’ to variables, using it as a default parameter, checking for ‘None’ with the identity operator, or handling missing values, ‘None’ is a crucial component of Python programming.
Exploring NoneType: Python’s Exclusive Datatype for ‘None’
In Python, ‘None’ is not merely a value or a placeholder for no value; it’s a data type in its own right, known as NoneType. This characteristic sets ‘None’ apart. You can confirm this by using the type() function:
print(type(None))
The output will be:
<class 'NoneType'>
This output indicates that ‘None’ is indeed a class of its own in Python.
Distinguishing ‘None’ from Other Null Representations
It’s vital to understand that ‘None’ is not the same as other null representations in Python, such as 0, False, or an empty string (”). While these values might symbolize emptiness or the absence of value in a logical sense, they are not equivalent to ‘None’. For instance, if you examine the truthiness of these values, you’ll find that ‘None’ is always considered False, whereas others may vary based on the context.
Value | Truthiness |
---|---|
None | False |
0 | False |
” (empty string) | False |
‘0’ (string containing a single zero) | True |
‘False’ (string containing the word False) | True |
print(bool(None)) # Outputs: False
print(bool(0)) # Outputs: False
print(bool('')) # Outputs: False
‘None’ in Python is a unique entity. It’s not just an absence of value, but a datatype of its own. It’s also a singleton object, which means all variables assigned as ‘None’ point to the same object. Understanding ‘None’ is a fundamental requirement for efficient and effective Python programming.
Return Statements and ‘None’
A unique feature of Python is that if a function doesn’t explicitly return a value, it implicitly returns ‘None’. This can be advantageous in certain situations. For instance, you might have a function that performs an operation and doesn’t require to return a value. In such a case, you can omit the return statement, and Python will automatically return ‘None’. Here’s an illustration:
def function():
print('Hello, world!')
result = function()
print(result) # Outputs: None
In this example, the function function()
lacks a return statement, so it returns ‘None’.
Advanced Use of “None” keyword
Utilizing ‘None’ as Flags
‘None’ can also function as a flag to denote special conditions in your program. For example, you might have a function that processes a list of items. If the function encounters an error while processing the list, it could return ‘None’ to signify that something went wrong. Here’s an illustration:
Example of using ‘None’ as a flag:
def process_items(items):
for item in items:
if not process_item(item): # If processing fails
return None # Return None to indicate failure
return True # Return True if all items were processed successfully
items = [1, 2, 3, 'error', 4]
print(process_items(items))
# Outputs: None
In this example, the function process_items()
returns ‘None’ if it fails to process an item.
‘None’ in User-Defined Objects
In user-defined objects, ‘None’ can be used to indicate the absence of a value or a relationship. For instance, you might have a Node
class in a linked list, where ‘None’ is used to mark the end of the list. Here’s an example:
class Node:
def __init__(self, data=None, next_node=None):
self.data = data
self.next_node = next_node
In this example, the next_node
attribute is set to ‘None’ to indicate that there’s no subsequent node.
Sentinel Value as an Alternative for ‘None’
Python also permits the use of a unique sentinel value as a substitute for ‘None’. This can provide more control and allow you to test special conditions in your program. Here’s an example:
Example of using a unique sentinel value as a substitute for ‘None’:
# Define a unique sentinel value
sentinel = object()
def function(parameter=sentinel):
if parameter is sentinel:
print('No argument was passed')
else:
print('An argument was passed')
function()
# Outputs: 'No argument was passed'
function('Hello')
# Outputs: 'An argument was passed'
In this example, the unique sentinel value is used as the default value for the parameter
argument. If no argument is passed, the function checks if parameter
is the sentinel value, indicating that no argument was passed.
In conclusion, ‘None’ is a versatile tool in Python that can be used in various advanced scenarios, such as in return statements, as flags, in custom objects, and as a sentinel value. Understanding these use cases can facilitate you in writing more efficient and effective Python code.
Delving into the Id Function
Every object in Python is assigned a unique identifier that remains constant throughout the object’s lifetime. This identifier is an integer and can be accessed using the id()
function. This function gains significant importance when working with ‘None’, as ‘None’ is a singleton in Python, meaning all instances of ‘None’ share the same id. Here’s how you can utilize the id()
function:
print(id(None)) # Outputs: the id of None
In this example, the id()
function returns the unique identifier of ‘None’.
Understanding the Getattr Function
Python’s getattr()
function facilitates accessing an object’s attribute. This function proves especially useful when dealing with ‘None’, as it allows for safe access to attributes that may or may not exist. If the attribute doesn’t exist and a default value is provided, getattr()
will return that default value. If no default value is provided and the attribute doesn’t exist, getattr()
will raise an AttributeError
. Here’s an illustration:
Example of using the getattr()
function:
class Test:
attribute = 'Hello'
test = Test()
print(getattr(test, 'attribute', 'Default')) # Outputs: 'Hello'
print(getattr(test, 'non_existent', 'Default')) # Outputs: 'Default'
print(getattr(None, 'attribute', 'Default')) # Outputs: 'Default'
In this example, the getattr()
function attempts to access the ‘attribute’ of ‘None’. Since ‘None’ doesn’t possess an ‘attribute’, getattr()
returns the default value.
Employing the Id and Getattr Functions with NoneType
The id()
and getattr()
functions offer a deeper level of control when handling null values in Python. By leveraging these functions, you can distinguish between different objects and safely access attributes, even when dealing with ‘None’. This proves particularly beneficial in debugging and error handling, as it enables you to identify ‘None’ and handle missing attributes gracefully.
Further Resources for Python Keywords
To enable you to delve deeper into Python Keywords, we’ve gathered a selection of online resources:
- Python Keywords: Strengthening Your Python Skills – Python keyword essentials: essential components for coding mastery.
Handling Null Values with Python None – Learn about Python’s “None” keyword, indicating the absence of a value.
Understanding the nonlocal Keyword in Python – Learn Python’s “nonlocal” keyword for modifying variables.
Async IO in Python – Gain a comprehensive understanding of asynchronous IO in Python with this in-depth tutorial.
Python Lambda Expressions – Learn the usage and techniques of lambda expressions with this simple guide.
Python Keywords – Familiarize yourself with Python’s reserved keywords in this straightforward tutorial.
Utilize these resources to advance your command over Python Keywords and become a more proficient Python programmer.
Conclusion
In conclusion, Python offers a plethora of functions and techniques for handling null values. By understanding and employing these functions, you can craft more robust and effective Python code. Remember, ‘None’ in Python isn’t merely an absence of value; it’s a first-class citizen that can be passed as an argument, returned from a function, and assigned to a variable, making ‘None’ a potent tool in Python programming.
In Python, ‘None’ is not merely an absence of value. It’s a separate entity, a datatype in its own right, and a first-class citizen capable of being passed as an argument, returned from a function, and assigned to a variable. It represents Python’s unique methodology for indicating null or nothingness, and it holds a pivotal role in Python programming.
From assigning ‘None’ to variables and employing it as a default parameter, to managing missing values and using it in return statements, ‘None’ is a versatile tool that can enhance your code’s flexibility and efficiency. It’s also a singleton in Python, implying that all instances of ‘None’ point to the same object. This allows for the use of the identity operator ‘is’ to check for ‘None’.
For both novices and Python gurus, our Python Cheat Sheet is an indispensable asset.
Understanding ‘None’ is vital for any Python programmer. It’s a foundational aspect of Python that underpins many facets of the language. Thus, the next time you encounter ‘None’ in your Python code, remember, it’s not merely a placeholder for no value, but a potent tool that can assist you in crafting superior Python code.