Python os.path Module: Complete Guide
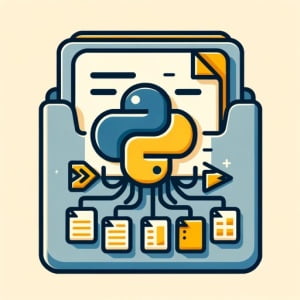
Are you finding it challenging to handle file and directory paths in Python? You’re not alone. Many developers find themselves in a maze when it comes to navigating the file system in Python, but there’s a module that can make this process a breeze.
Think of Python’s os.path module as a seasoned explorer – it’s a handy tool that can help you navigate the file system with ease. It provides functions for common pathname manipulations, making it a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using os.path in Python, from its basic operations to more advanced techniques. We’ll cover everything from simple file and directory manipulations to handling different operating systems and their path conventions.
So, let’s dive in and start mastering Python’s os.path module!
TL;DR: How Do I Use the os.path Module in Python?
The os.path module in Python is used for common pathname manipulations. It provides a set of functions that can be used to manipulate file and directory paths in a way that is independent of the underlying operating system.
Here’s a simple example:
import os
print(os.path.join('/home', 'user', 'documents'))
# Output:
# '/home/user/documents'
In this example, we’re using the os.path.join
function to concatenate several path components with the appropriate operating system separator. The result is a string representing a path that points to a user’s documents directory.
This is just a basic usage of the os.path module in Python. There’s much more to learn about this module, including more advanced techniques and alternative approaches. So, keep reading for a more detailed guide on using the os.path module in Python.
Table of Contents
- Navigating Python os.path: Beginner’s Guide
- Advanced Techniques with Python’s os.path
- Exploring Alternative Approaches: Python’s pathlib Module
- Troubleshooting Common Issues with Python’s os.path
- Python os.path: Understanding the Fundamentals
- Python os.path: Beyond the Basics
- Wrapping Up: Mastering Python’s os.path Module
The os.path module in Python is a handy tool for manipulating file and directory paths. It provides several functions that can be utilized to perform common tasks related to file paths. Let’s start with some basic usage examples.
Joining Paths with os.path.join
One of the most commonly used functions in the os.path module is os.path.join()
. This function is used to combine one or more path names into a single path. Here’s a basic example:
import os
path = os.path.join('/home', 'user', 'documents')
print(path)
# Output:
# '/home/user/documents'
In this example, os.path.join()
concatenates the given path components with the appropriate operating system separator, resulting in a string that represents a path to the user’s documents directory.
Getting the Base Name with os.path.basename
The os.path.basename()
function returns the base name of the pathname path
. This is the second element of the pair returned by passing path
to the function os.path.split()
. Here’s a basic example:
import os
path = '/home/user/documents'
basename = os.path.basename(path)
print(basename)
# Output:
# 'documents'
In this example, os.path.basename()
returns the base name ‘documents’ from the path string.
These are just a few examples of the basic functions provided by the os.path module in Python. As you can see, this module is a powerful tool for manipulating file and directory paths. However, like any tool, it must be used correctly to avoid potential pitfalls, such as incorrectly joining paths or retrieving the wrong path component.
Advanced Techniques with Python’s os.path
As you become more comfortable with the os.path module, you can start to explore some of its more complex functions. Let’s delve into some intermediate-level uses of os.path.
Splitting and Joining Paths
The os.path
module provides functions to split a pathname into a pair (head, tail) and to join two or more pathname components.
import os
path = '/home/user/documents/myfile.txt'
head, tail = os.path.split(path)
print('Head:', head)
print('Tail:', tail)
# Output:
# Head: /home/user/documents
# Tail: myfile.txt
In this example, os.path.split()
splits the path into two parts: the head (/home/user/documents
) and the tail (myfile.txt
). This can be useful when you need to manipulate individual parts of a path.
Checking if a Path Exists
The os.path
module provides a function to check if a certain path exists. This can be useful in many situations, such as before trying to open a file.
import os
path = '/home/user/documents/myfile.txt'
exists = os.path.exists(path)
print('Exists:', exists)
# Output:
# Exists: True
In this example, os.path.exists()
checks if the path exists and returns a boolean value (True
if the path exists, False
otherwise).
Finding the Size of a File
The os.path
module also provides a function to find the size of a file. Here’s an example:
import os
path = '/home/user/documents/myfile.txt'
size = os.path.getsize(path)
print('Size:', size)
# Output:
# Size: 1024
In this example, os.path.getsize()
returns the size of the file in bytes. This can be useful when you need to monitor the size of files, such as for logging or cleanup tasks.
By leveraging these advanced functions, you can perform more complex operations on file and directory paths in Python. Remember, the key is to understand what each function does and how to use it effectively in your code.
Exploring Alternative Approaches: Python’s pathlib Module
While the os.path module is a powerful tool for manipulating file and directory paths, Python also offers an alternative approach: the pathlib module. This module provides an object-oriented interface for dealing with filesystem paths.
A Glimpse at Python’s pathlib Module
The pathlib module was introduced in Python 3.4 and has since become a popular alternative to os.path for working with file paths. It provides a more intuitive method to handle file system paths. Here’s a basic example:
from pathlib import Path
p = Path('/home/user/documents')
print(p)
# Output:
# /home/user/documents
In this example, we’re creating a Path object that represents the path to the user’s documents directory. The Path object can be used just like a string in most contexts, and it also provides several useful methods and properties.
Comparing pathlib and os.path
While both os.path and pathlib can be used to manipulate file and directory paths, they offer different approaches. The os.path module provides a set of functions for pathname manipulations, while pathlib offers an object-oriented interface.
For example, to join two paths together, you would use os.path.join()
with os.path, and the /
operator with pathlib:
import os
from pathlib import Path
# os.path
path1 = os.path.join('/home', 'user', 'documents')
print(path1)
# pathlib
path2 = Path('/home') / 'user' / 'documents'
print(path2)
# Output:
# /home/user/documents
# /home/user/documents
In this example, both os.path.join()
and the /
operator result in the same path string. However, the pathlib approach can be more intuitive and easier to read, especially when dealing with complex paths.
The choice between os.path and pathlib often comes down to personal preference and the specific requirements of your project. Both modules provide a robust set of tools for working with file and directory paths in Python.
Troubleshooting Common Issues with Python’s os.path
While Python’s os.path module is a powerful tool for manipulating file and directory paths, it’s not without its quirks. Here, we’ll discuss some common issues you may encounter when using os.path and provide solutions and workarounds.
Dealing with Different Operating Systems
One of the main challenges when working with file paths is dealing with different operating systems. Different operating systems have different conventions for file paths. For instance, Windows uses backslashes (\
) while Unix-based systems like Linux and macOS use forward slashes (/
).
Fortunately, os.path provides a set of functions that handle these differences for you. For example, os.path.join()
uses the appropriate path separator for the current operating system:
import os
path = os.path.join('home', 'user', 'documents')
print(path)
# Output on Unix-based systems:
# home/user/documents
# Output on Windows:
# home\user\documents
In this example, os.path.join()
uses the appropriate path separator for the current operating system, ensuring that your code works correctly on different platforms.
However, if you’re reading a path from a string, you might still encounter issues. For instance, a path written for Windows might not work correctly on a Unix-based system. In such cases, you can use os.path.normpath()
to convert a pathname to the normal form for the current operating system:
import os
path = os.path.normpath('home\user\documents')
print(path)
# Output on Unix-based systems:
# home/user/documents
# Output on Windows:
# home\user\documents
In this example, os.path.normpath()
converts the backslashes in the path to forward slashes on Unix-based systems, ensuring that the path works correctly regardless of the operating system.
By understanding these issues and knowing how to handle them, you can make your Python scripts more robust and portable.
Python os.path: Understanding the Fundamentals
Before we delve deeper into the os.path module, it’s important to understand how Python handles file and directory paths and why it’s crucial to handle paths correctly in your Python scripts.
How Python Handles File and Directory Paths
In Python, file and directory paths are just strings that represent the location of a file or directory in the file system. These strings can be manipulated using standard string operations. For example, you might concatenate two strings to join two path components together:
path = '/home/user' + '/documents'
print(path)
# Output:
# /home/user/documents
However, this approach can be error-prone. For instance, you might forget to include a path separator, or you might include too many. Moreover, different operating systems use different path separators, which can lead to compatibility issues.
The Role of Python’s os.path Module
This is where the os.path module comes in. It provides a set of functions that abstract these details away, allowing you to manipulate file and directory paths in a way that is independent of the underlying operating system.
For example, the os.path.join()
function takes care of the path separator for you:
import os
path = os.path.join('/home/user', 'documents')
print(path)
# Output:
# /home/user/documents
In this example, os.path.join()
automatically inserts the appropriate path separator between the two path components.
The Importance of Handling Paths Correctly
Handling file and directory paths correctly is crucial for the reliability and portability of your Python scripts. If you handle paths incorrectly, your script might work on your machine but break on others. By using the os.path module, you can ensure that your scripts work correctly regardless of the operating system.
In essence, understanding the fundamentals of file and directory paths in Python and the role of the os.path module is key to writing robust and portable Python scripts.
Python os.path: Beyond the Basics
The os.path module is not just for simple file and directory manipulations. Its functions are fundamental in larger scripts or projects, such as web applications or data analysis scripts, where handling file and directory paths correctly is crucial for the reliability and portability of the code.
File I/O and os.path
When working with file I/O in Python, the os.path module becomes particularly important. Whether you’re reading data from CSV files in a data analysis script or writing logs to a file in a web application, you’ll likely need to handle file paths.
Here’s an example of how you might use os.path when opening a file:
import os
path = os.path.join('/home/user', 'documents', 'myfile.txt')
with open(path, 'r') as file:
content = file.read()
print(content)
# Output:
# (The contents of myfile.txt)
In this example, we’re using os.path.join()
to construct the path to a file, which we then open and read.
Exploring File Metadata with os.path
The os.path module also provides functions to retrieve file metadata, such as the file’s size and modification time. This can be useful in a variety of scenarios, from cleaning up old files to monitoring changes in a directory.
Here’s an example of how you might use os.path to retrieve file metadata:
import os
path = os.path.join('/home/user', 'documents', 'myfile.txt')
size = os.path.getsize(path)
mtime = os.path.getmtime(path)
print('Size:', size)
print('Last modified:', mtime)
# Output:
# Size: 1024
# Last modified: 1632960473.0
In this example, we’re using os.path.getsize()
to retrieve the size of the file and os.path.getmtime()
to retrieve the file’s last modification time.
Further Resources for Mastering Python’s os.path
If you’re interested in learning more about Python’s os.path module and related topics, Click Here! This guide explains environment variables and how “os” allows you to access and modify them.
To further your OS and file handling knowledge, here are some other resources that you might find useful:
- Python File Creation – Dive into the world of file creation and understand file modes and permissions.
Closing Files in Python – Explore the importance of closing files in Python and preventing resource leaks.
Python’s official os.path documentation provides a comprehensive overview of the os.path module and its functions.
Python’s official pathlib documentation is a great resource if you’re interested in exploring the pathlib module.
Real Python’s Python file reading and writing guide provides a practical introduction to file I/O in Python, including os.path usage.
Wrapping Up: Mastering Python’s os.path Module
In this comprehensive guide, we’ve unraveled the intricacies of Python’s os.path module, a powerful tool for manipulating file and directory paths in Python.
We began with the basics, learning how to use os.path’s functions for common pathname manipulations, such as joining and splitting paths. We then ventured into more advanced territory, exploring complex uses of os.path, such as checking if a path exists and finding the size of a file.
Along the way, we tackled common challenges you might face when using os.path, such as dealing with different operating systems and their path conventions, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handling file and directory paths in Python, introducing Python’s pathlib module, an object-oriented interface for dealing with filesystem paths.
Here’s a quick comparison of these methods:
Method | Flexibility | Ease of Use | Object-Oriented |
---|---|---|---|
os.path | High | Moderate | No |
pathlib | High | High | Yes |
Whether you’re just starting out with os.path or you’re looking to level up your file handling skills in Python, we hope this guide has given you a deeper understanding of os.path and its capabilities.
With its balance of flexibility and ease of use, os.path is a powerful tool for handling file and directory paths in Python. Now, you’re well equipped to navigate the file system with ease. Happy coding!