Python Walrus Operator: Syntax, Usage, Examples
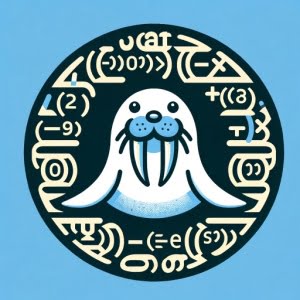
Are you finding the walrus operator in Python a bit puzzling? You’re not alone. Many developers find themselves scratching their heads when it comes to this unique operator. But, just like a walrus uses its tusks to break through ice, Python’s walrus operator can break through complex expressions, making your code more concise and readable.
Think of Python’s walrus operator as a multitasking hero – it allows you to assign values to variables as part of an expression, thereby reducing the lines of code and enhancing readability.
In this guide, we’ll walk you through the ins and outs of the walrus operator in Python, from its basic usage to more advanced applications. We’ll cover everything from the fundamentals of the walrus operator to its practical use cases, as well as alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering the Python walrus operator!
TL;DR: What is the Walrus Operator in Python?
The walrus operator
:=
, also known as the assignment expression operator, is a new operator introduced in Python 3.8. It allows you to assign values to variables as part of an expression, thereby simplifying your code and making it more readable.
Here’s a simple example:
some_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
if (n := len(some_list)) > 10:
print(f'The list has {n} elements.')
# Output:
# 'The list has 11 elements.'
In this example, we’re using the walrus operator :=
to assign the length of some_list
to the variable n
as part of the if
statement. This allows us to use n
in the print statement that follows, thereby reducing the need for an extra line of code to assign n
beforehand.
This is just a basic introduction to the walrus operator in Python. Continue reading for a more in-depth exploration of its usage and benefits.
Table of Contents
- Simplifying Code with the Walrus Operator: A Beginner’s Guide
- Advanced Python Walrus Operator: List Comprehensions and While Loops
- Alternative Approaches to the Python Walrus Operator
- Troubleshooting the Python Walrus Operator
- Python Operators: The Building Blocks
- The Walrus Operator: A Unique Addition to Python
- Advanced Python: Walrus Operator in Larger Projects
- Wrapping Up: Mastering the Python Walrus Operator
Simplifying Code with the Walrus Operator: A Beginner’s Guide
The walrus operator, also known as the assignment expression operator, is a powerful tool that can simplify your code by combining assignment and comparison in a single line. It’s like a two-in-one tool that not only assigns values to variables but also evaluates the expression at the same time.
Let’s look at a basic example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
if (n := len(my_list)) > 10:
print(f'The list has {n} elements.')
# Output:
# 'The list has 11 elements.'
In this example, n := len(my_list)
is the walrus operator in action. It assigns the length of my_list
to the variable n
and then evaluates whether n
is greater than 10. If the condition is true, it prints ‘The list has {n} elements.’
This is a simple but powerful way to streamline your code, making it more concise and readable. However, like any tool, the walrus operator should be used with care. Overuse can lead to code that is difficult to understand and maintain. So, while the walrus operator is a powerful tool, it’s important to use it judiciously and appropriately.
Advanced Python Walrus Operator: List Comprehensions and While Loops
The walrus operator is not just for simple assignments and conditions. It can also be used in more complex scenarios, such as list comprehensions and while loops. Let’s dive deeper into its advanced usage.
Walrus Operator in List Comprehensions
List comprehensions are a powerful feature of Python that allow you to create lists in a very concise way. The walrus operator can be used in list comprehensions to make them even more efficient.
Consider the following example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
filtered_list = [(n := x) for x in my_list if n % 2 == 0]
print(filtered_list)
# Output:
# [2, 4, 6, 8, 10]
In this example, we’re using the walrus operator to assign the value of x
to n
and then check if n
is even. If it is, n
is added to the new list filtered_list
. This allows us to create a filtered list in just one line of code.
Walrus Operator in While Loops
The walrus operator can also be used in while loops to both assign and check a condition in the same line. Let’s see an example:
import random
while (number := random.randint(0, 10)) != 5:
print(f'The number {number} is not 5')
# Output:
# 'The number 3 is not 5'
# 'The number 7 is not 5'
# 'The number 2 is not 5'
# 'The number 5 is not 5'
In this example, we’re using the walrus operator to assign a random number between 0 and 10 to the variable number
and then check if number
is not equal to 5. If number
is not 5, the loop continues and prints ‘The number {number} is not 5’. The loop stops when number
is 5.
These examples show how the walrus operator can be used in more complex scenarios to make your code more efficient and readable. However, it’s important to remember that the walrus operator should be used judiciously. Overuse can lead to code that is difficult to understand and maintain.
Alternative Approaches to the Python Walrus Operator
While the walrus operator is a powerful tool, it’s not the only way to assign values to variables as part of an expression. There are several other techniques and operators that can accomplish the same task. Understanding these alternatives can help you make more informed decisions about which approach is best for your specific situation.
Traditional Assignment and Comparison
Before the introduction of the walrus operator, developers would typically use separate lines for assignment and comparison. Let’s look at an example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
n = len(my_list)
if n > 10:
print(f'The list has {n} elements.')
# Output:
# 'The list has 11 elements.'
In this example, we first assign the length of my_list
to n
, and then we check if n
is greater than 10. Although this approach takes an extra line of code, it can be easier to understand for beginners and is less prone to errors.
Using Ternary Operator
The ternary operator is another approach that can be used to assign values to variables as part of an expression. Here’s how it works:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
n = len(my_list) if (len(my_list) > 10) else 0
print(f'The list has {n} elements.')
# Output:
# 'The list has 11 elements.'
In this example, we’re using the ternary operator to assign the length of my_list
to n
if the length of my_list
is greater than 10. Otherwise, n
is assigned 0. This approach is more concise than the traditional assignment and comparison, but it can be more difficult to understand for beginners.
Each of these alternatives has its own benefits and drawbacks. The traditional assignment and comparison is easier to understand but takes more lines of code. The ternary operator is more concise but can be more difficult to understand. The walrus operator strikes a balance between these two, offering conciseness and readability. However, it’s important to remember that the best approach depends on your specific situation and personal preference.
Troubleshooting the Python Walrus Operator
Like any other programming feature, the Python walrus operator can sometimes lead to errors or obstacles. Understanding these common issues and their solutions can help you write more efficient and error-free code.
Unparenthesized Assignment
One common error when using the walrus operator is forgetting to enclose the assignment in parentheses. This can lead to unexpected results. Let’s look at an example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
if n := len(my_list) > 10:
print(f'The list has {n} elements.')
# Output:
# 'The list has True elements.'
In this example, we forgot to enclose n := len(my_list)
in parentheses. As a result, n
is assigned the result of the comparison len(my_list) > 10
, which is a boolean value. To avoid this error, always enclose the assignment in parentheses:
if (n := len(my_list)) > 10:
Using the Walrus Operator in Older Python Versions
The walrus operator was introduced in Python 3.8. If you’re using an older version of Python, you’ll get a syntax error when trying to use the walrus operator. To avoid this error, make sure to update to Python 3.8 or later.
Overusing the Walrus Operator
While the walrus operator can simplify your code, overusing it can lead to code that is difficult to understand and maintain. It’s important to use the walrus operator judiciously and only when it improves the readability of your code.
In conclusion, understanding these common issues and their solutions can help you avoid errors and write more efficient code with the Python walrus operator. Always remember to enclose assignments in parentheses, use Python 3.8 or later, and use the walrus operator judiciously.
Python Operators: The Building Blocks
Before we dive deeper into the walrus operator, let’s take a step back and understand the concept of operators in Python. Operators are special symbols in Python that carry out arithmetic or logical computation. They are the building blocks of any Python program and are used to manipulate data and variables.
There are several types of operators in Python, including arithmetic operators (like +
, -
, *
, /
), comparison operators (like ==
, !=
, <
, >
), logical operators (like and
, or
, not
), and assignment operators (like =
, +=
, -=
). The walrus operator (:=
) is a type of assignment operator, also known as the assignment expression operator.
The Walrus Operator: A Unique Addition to Python
The walrus operator was introduced in Python 3.8 as a new type of assignment operator. Unlike other assignment operators, the walrus operator allows you to assign values to variables as part of an expression. This means you can assign a value to a variable and evaluate an expression in the same line of code.
Here’s a simple example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
if (n := len(my_list)) > 10:
print(f'The list has {n} elements.')
# Output:
# 'The list has 11 elements.'
In this example, n := len(my_list)
is the walrus operator in action. It assigns the length of my_list
to the variable n
and then evaluates whether n
is greater than 10. If the condition is true, it prints ‘The list has {n} elements.’
This unique ability of the walrus operator to combine assignment and evaluation in a single expression makes it a powerful tool for simplifying and streamlining your Python code.
Advanced Python: Walrus Operator in Larger Projects
The walrus operator can be a powerful tool in larger scripts or projects. Its ability to simplify expressions and reduce lines of code can make your code more efficient and easier to read. However, it’s important to remember that the walrus operator is just one tool in the Python toolbox. There are many other operators and functions that often accompany the walrus operator in typical use cases.
For example, the walrus operator can be used in conjunction with the for
loop and the if
statement to filter elements in a list. Here’s an example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
filtered_list = [(n := x) for x in my_list if n % 2 == 0]
print(filtered_list)
# Output:
# [2, 4, 6, 8, 10]
In this example, we’re using the walrus operator to assign the value of x
to n
and then check if n
is even. If it is, n
is added to the new list filtered_list
. This allows us to create a filtered list in just one line of code.
Further Resources for Mastering Python Operators
If you’re interested in learning more about the walrus operator and other Python operators, here are a few resources that might help:
- Python If Statements – Learn how to troubleshoot and debug code with if statements for error resolution.
Using “if not” in Python – A guide to negation and cheking absence of values in conditionals using “if not”.
Python’s Boolean Operations – An overview on using boolean variables and expressions to create logic in Python programs.
Python’s official documentation on assignment expressions provides a detailed explanation of the walrus operator’s syntax and usage.
Real Python’s Guide to Python operators offers a comprehensive overview of all types of operators in Python.
Python’s official tutorial on control flow tools discusses how the walrus operator can be used in conjunction with other control flow tools.
Wrapping Up: Mastering the Python Walrus Operator
In this comprehensive guide, we’ve delved into the depths of the Python walrus operator, a powerful tool for simplifying and streamlining your code.
We began with the basics, understanding the purpose and functionality of the walrus operator. We then explored its basic usage, learning how it can combine assignment and comparison in a single line of code. We ventured into more advanced territory, examining how the walrus operator can be used in complex scenarios like list comprehensions and while loops.
Along the way, we tackled common challenges that you might encounter when using the walrus operator, such as unparenthesized assignments and compatibility with older Python versions, providing you with solutions to overcome these hurdles.
We also looked at alternative approaches to the walrus operator, comparing it with traditional assignment and comparison methods, and the ternary operator.
Here’s a quick comparison of these methods:
Method | Readability | Efficiency | Ease of Use |
---|---|---|---|
Walrus Operator | High | High | Moderate |
Traditional Assignment and Comparison | Moderate | Low | High |
Ternary Operator | Low | High | Low |
Whether you’re just starting out with the Python walrus operator or you’re looking to level up your Python skills, we hope this guide has given you a deeper understanding of the walrus operator and its capabilities.
With its unique ability to combine assignment and evaluation in a single expression, the Python walrus operator is a powerful tool for efficient and readable coding. Happy coding!