Java
13 Nov 2023
Java Synchronized Keyword: Usage, Tips, and Alternatives
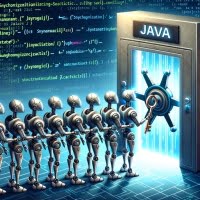
Are you finding it challenging to work with Java’s ‘synchronized’ keyword? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze. Think of Java’s ‘synchronized’ keyword as a traffic cop – managing the flow of threads in Java, ensuring that only one thread can access
13 Nov 2023
Insertion Sort in Java: Implementation Guide
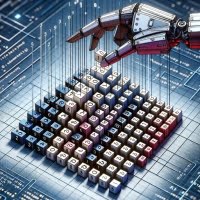
Are you finding it challenging to implement the Insertion Sort algorithm in Java? You’re not alone. Many developers find themselves puzzled when it comes to sorting algorithms, but we’re here to help. Think of the Insertion Sort as a meticulous librarian, carefully placing each book (or in our case, data element) in its rightful place.
13 Nov 2023
LinkedHashMap | Guide to Java’s Hybrid Data Structure
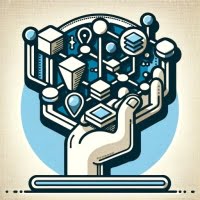
Are you finding it challenging to understand and use LinkedHashMap in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this hybrid data structure, but we’re here to help. Think of LinkedHashMap as a bridge – a bridge that combines the best of HashMap and LinkedList. It’s a powerful tool
13 Nov 2023
Array vs ArrayList: Comparing Java Array Data Structures
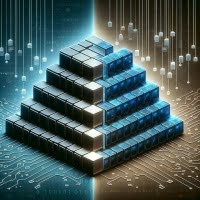
Ever found yourself tangled in the choice between Array and ArrayList in Java? You’re not alone. Many developers find themselves in a similar predicament. Think of Array and ArrayList as two different tools in your Java toolkit, each with its own strengths and weaknesses. Choosing the right tool for the job can make your coding
13 Nov 2023
Selection Sort Java: Guide to Sorting Array Elements
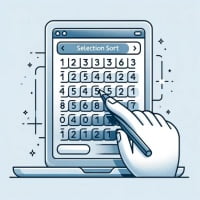
Ever found yourself grappling with implementing selection sort in Java? You’re not alone. Many developers find the selection sort algorithm a bit tricky to implement. Think of selection sort as a meticulous organizer, efficiently sorting your data in a systematic manner. This guide will walk you through the process of implementing selection sort in Java,
13 Nov 2023
Java Optional: Managing Nullable Object References
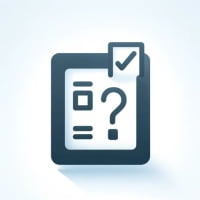
Ever found yourself grappling with null pointer exceptions in Java? You’re not alone. Many developers find themselves in this predicament, but there’s a tool that can help you avoid these pitfalls. Think of Java Optional as a safety net – a safety net that can save you from the perils of null pointer exceptions. It’s
13 Nov 2023
parseInt() Java Method: From Strings to Integers
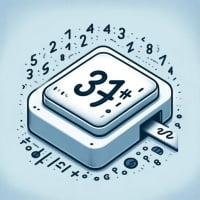
Are you finding it challenging to convert strings to integers in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze. Like a skilled mathematician, Java’s parseInt method can transform a string of digits into a usable integer. These integers can then be used
13 Nov 2023
Using JDoodle: Your Online Compiler and Editor
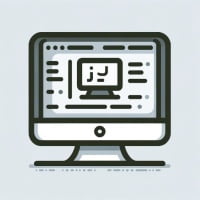
Ever found yourself needing to compile and run a code snippet quickly, but dread the idea of setting up a local development environment? You’re not alone. Many developers find themselves in this situation, but there’s a tool that can make this process a breeze. Think of JDoodle as your online compiler and editor – a
13 Nov 2023
binarySearch() Java: Locating Elements in Sorted Arrays
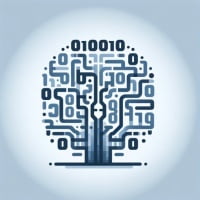
Are you finding it challenging to implement binary search in Java? You’re not alone. Many developers find themselves in a maze when it comes to binary search in Java, but we’re here to help. Think of binary search as a detective, helping you find the ‘suspect’ (element) in a sorted ‘lineup’ (array) in the most
13 Nov 2023
Int to String Java Conversion: Methods and Examples
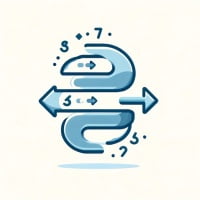
Are you finding it challenging to convert integers to strings in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this task, but there’s a solution. Think of Java as a skilled interpreter, capable of translating integers into strings. It provides various methods to perform this conversion, each with its