Programming / Coding
22 Aug 2023
Python Int to String Conversion Guide (With Examples)
In this in-depth guide, we will explore the process of converting an integer to a string in Python. Irrespective of whether you’re a Python novice, a programming student, or a seasoned developer wishing to brush up your Python skills, this guide aims to provide clear, concise instructions, supplemented with practical examples and use cases. So,
22 Aug 2023
Iterate a Dictionary in Python: Guide (With Examples)
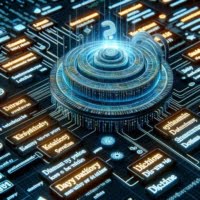
Today, we’re set to decode the mystery of Python dictionaries and the art of iterating through them. If you’ve been puzzled about how to loop through a dictionary or wish to broaden your Python knowledge, you’re at the right spot. Python dictionaries, a type of data structure, store data as key-value pairs. Their versatility is
22 Aug 2023
How To Find a Square Root in Python
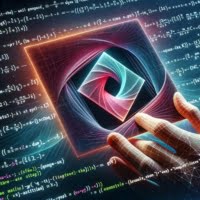
Ever wondered how to calculate square roots in Python? Python, with its powerful and versatile nature, simplifies this process significantly. This article is a comprehensive guide to understanding and calculating square roots in Python. By the end of this guide, you’ll be proficient in computing square roots using various methods in Python. So, let’s embark
22 Aug 2023
Python String to Int Conversion Guide (With Examples)
Ever stumbled upon a scenario where you need to perform a mathematical operation on data, but it’s in string format? Python comes to your rescue here. Its user-friendly and flexible data type conversion simplifies your data manipulation tasks. This article provides a comprehensive guide on converting a string to an integer in Python. We’ll guide
22 Aug 2023
Converting a List to a String in Python
Lists and strings are among the most frequently used data types in Python. A list is a collection of items, which can be of varying types, while a string is a sequence of characters. The need for converting a list into a string is common, particularly when dealing with data manipulation and processing tasks. In
21 Aug 2023
Python Dataclass | Funadmentals, Usage, and Examples
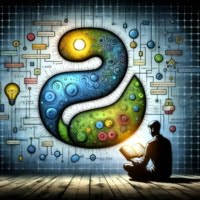
As a type of class specifically designed for storing data, Python dataclasses are a hidden gem in Python’s toolbox that can make your life as a programmer much easier. This comprehensive guide aims to equip you with the knowledge and skills to effectively use Python dataclasses in your projects. By the end of this post,
21 Aug 2023
Python isinstance() Function Guide (With Examples)
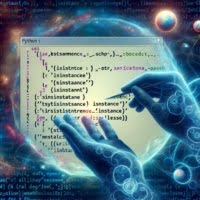
Have you ever been curious about Python’s isinstance() function? At its core, isinstance() is a type-checking function, verifying the type of an object in Python and ensuring your code behaves as expected. But why is type checking crucial, and how does isinstance() contribute to better Python code? This article will demystify isinstance(), guiding you through
21 Aug 2023
Python filter() Function Guide (With Examples)
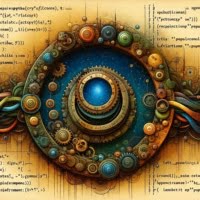
Imagine you’re in a vast desert, and you’re looking for hidden gold nuggets. It’s a daunting task, isn’t it? But what if you could easily separate the gold from the dirt? In Python, the filter function helps you sift through massive amounts of data, separating the gold nuggets from the dirt and rocks. This guide
21 Aug 2023
Python input() Function Guide (With Examples)
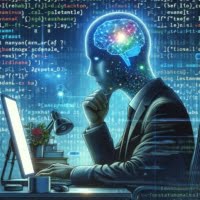
Have you ever been curious about how to make your Python programs more interactive? The answer lies in a single, potent function – input(). This function is your key to user interaction in Python, enabling your programs to receive user data and respond in kind. From a simple program that greets the user by name
21 Aug 2023
Using Python Assert to Write Bug Free Code
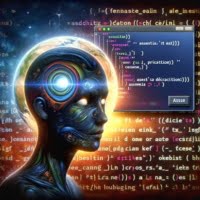
Ever wondered how to use Python’s assert statement? This seemingly modest keyword is actually a powerhouse in Python programming, offering the ability to fortify your code, enhance readability, and streamline debugging. The assert statement serves as a crucial debugging aid in Python. It tests a specific condition within your code, and if the condition is