Programming / Coding
28 Aug 2023
Python sleep() | Function Guide (With Examples)
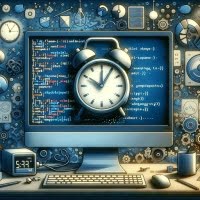
Ever wished for your Python program to take a breather? Just like a hardworking employee, there are moments when your code needs to halt its operations. Whether it’s to simulate user interaction, delay an API call, or simply to let your system catch its breath, the ability to pause can be a game-changer in the
28 Aug 2023
Python Random Module | Usage Guide (With Examples)
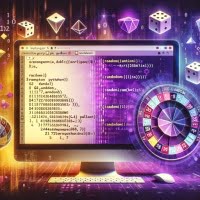
Ever felt puzzled by the concept of randomness in Python? You’re not alone. Just as the outcome of a dice roll adds an element of unpredictability to a board game, Python’s random module introduces a similar sense of chance into your code. This comprehensive guide is designed to help you navigate the labyrinth of Python’s
28 Aug 2023
Learn Flask for Python | Quick Start Guide (With Examples)
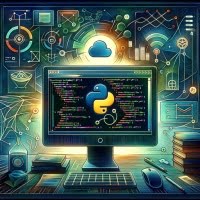
Ever felt overwhelmed by web development in Python? You’re not alone. Web development can be a complex beast, especially when you’re dealing with a powerful language like Python. But, just like a skilled craftsman has a toolbox full of tools, Python developers have Flask. Flask is a lightweight web framework that can help you build
28 Aug 2023
Python String format() Function Guide (With Examples)
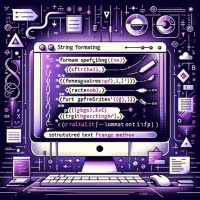
Ever found yourself wrestling with Python string formatting? Fear not, you’re not alone. Python, like a skilled typesetter, offers a multitude of ways to format strings. These tools help make your code cleaner, more readable, and efficient. In this comprehensive guide, we’ll journey through the various methods of string formatting in Python. We’ll start from
28 Aug 2023
Python Requests Module Usage Guide (With Examples)
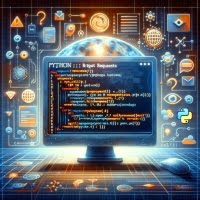
Are you grappling with HTTP requests in Python? Imagine having a seasoned negotiator to help you converse with the world wide web. That’s exactly what the Python requests library does for you. This comprehensive guide is designed to usher you into the world of making HTTP requests in Python using the requests library. We’ll start
28 Aug 2023
Python Regex: Guide to the ‘re’ Library and Functions

Are you wrestling with regular expressions in Python? Fear not, you’re not alone. Regular expressions, or regex, can feel like an impenetrable fortress of complexity. But just like a seasoned detective, Python’s regex module can help you uncover any pattern you’re searching for in a string. This comprehensive guide will take you from a novice
28 Aug 2023
Python Tuple | Data Type Usage Guide (With Examples)
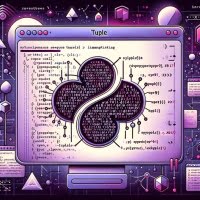
Ever felt like you’re wrestling with a locked suitcase when trying to grasp the concept of tuples in Python? You’re not alone. Tuples, just like a tightly locked suitcase, are immutable in Python, meaning once you’ve packed them, you can’t change what’s inside. They can hold a collection of items, but once created, they remain
28 Aug 2023
Learn Python Write to File | Guide (With Examples)
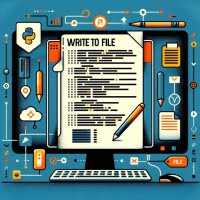
Ever felt like you’re wrestling with your code just to write data to a file in Python? You’re not alone. But the good news is, Python is like a diligent secretary, capable of jotting down data into any file format with ease. Whether you’re a beginner just dipping your toes into Python’s file handling capabilities,
28 Aug 2023
Python Datetime | Module Usage Guide (With Examples)
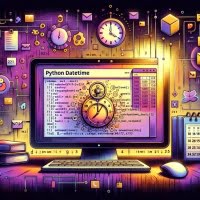
Ever found yourself wrestling with dates and times in Python? Well, you’re not alone. Handling time can be a mind-bending task, especially when dealing with different time zones, formats, and calculations. But fear not! Python’s datetime module is like your personal time machine, designed to help you navigate the complex world of dates, times, and
28 Aug 2023
Python Try / Except: How to Catch Errors (With Examples)
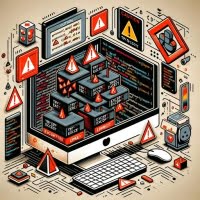
Ever found yourself stuck with error handling in Python? You’re not alone. Python’s try and except blocks function like a safety net, catching errors and ensuring your program runs without hiccups. This comprehensive guide will walk you through the intricacies of using try and except in Python. Whether you’re a beginner or an advanced Python